要修改文件编码格式,可以使用Python中的open
函数、read
和write
方法来读取和写入文件,并使用指定的编码格式。 例如,可以通过以下步骤来修改文件的编码格式:读取原始文件的内容、将其解码为Unicode字符串、然后使用新的编码格式将其写入新的文件。下面详细介绍其中的一个方法。
使用open
函数读取和写入文件内容
- 首先,使用
open
函数打开原始文件,并指定其编码格式(例如'utf-8'
)。 - 读取文件内容到内存中。
- 关闭原始文件。
- 使用
open
函数创建一个新文件,并指定目标编码格式(例如'utf-16'
)。 - 将内存中的内容写入新文件,自动转换为指定的编码格式。
- 关闭新文件。
以下是一个示例代码:
# 打开原始文件并读取内容
with open('source_file.txt', 'r', encoding='utf-8') as source_file:
content = source_file.read()
打开目标文件并写入内容
with open('target_file.txt', 'w', encoding='utf-16') as target_file:
target_file.write(content)
通过这种方法,我们可以轻松地修改文件的编码格式。接下来,我们将深入探讨其他方法和相关的编码知识。
一、文件编码基础知识
在处理文件编码格式之前,了解一些基本的编码知识是非常重要的。文件编码决定了如何将字符数据转换为字节数据,并从字节数据中还原字符数据。常见的编码格式包括UTF-8
、UTF-16
和ISO-8859-1
等。
1、字符编码简介
字符编码是一种将字符映射到数字(字节序列)的规则。不同的字符编码标准支持不同的字符集和编码方式。例如:
- ASCII:一种早期的字符编码标准,只支持128个字符,主要用于英语字符。
- UTF-8:一种变长字符编码,支持所有Unicode字符,常用于互联网和文本文件。
- UTF-16:另一种Unicode字符编码,使用16位或32位来表示字符,常用于Windows和Java平台。
- ISO-8859-1:一种单字节编码标准,支持西欧字符集。
2、Python中的编码支持
Python内置了对多种字符编码的支持,可以通过open
函数的encoding
参数来指定文件的编码格式。常见的编码格式包括:
'utf-8'
:UTF-8编码'utf-16'
:UTF-16编码'latin-1'
:ISO-8859-1编码'ascii'
:ASCII编码
了解了文件编码的基础知识后,我们将探讨如何使用Python来修改文件的编码格式。
二、修改文件编码格式的方法
1、使用open
函数
正如前面提到的,可以使用open
函数读取和写入文件来修改文件的编码格式。以下是一个更详细的示例,展示了从UTF-8编码转换为UTF-16编码:
def convert_encoding(source_path, target_path, source_encoding, target_encoding):
# 打开原始文件并读取内容
with open(source_path, 'r', encoding=source_encoding) as source_file:
content = source_file.read()
# 打开目标文件并写入内容
with open(target_path, 'w', encoding=target_encoding) as target_file:
target_file.write(content)
示例调用
convert_encoding('source_file.txt', 'target_file.txt', 'utf-8', 'utf-16')
在这个示例中,我们定义了一个函数convert_encoding
,它接受源文件路径、目标文件路径、源文件编码和目标文件编码作为参数,并执行编码转换。
2、使用codecs
模块
Python的codecs
模块提供了更灵活的字符编码处理功能。可以使用codecs.open
函数来读取和写入文件。以下是一个示例:
import codecs
def convert_encoding_with_codecs(source_path, target_path, source_encoding, target_encoding):
# 打开原始文件并读取内容
with codecs.open(source_path, 'r', encoding=source_encoding) as source_file:
content = source_file.read()
# 打开目标文件并写入内容
with codecs.open(target_path, 'w', encoding=target_encoding) as target_file:
target_file.write(content)
示例调用
convert_encoding_with_codecs('source_file.txt', 'target_file.txt', 'utf-8', 'utf-16')
使用codecs
模块可以更好地处理不同编码之间的转换。
3、处理大文件
当处理大文件时,可能无法将整个文件内容一次性读入内存。此时,可以逐行读取文件,并逐行写入目标文件。以下是一个示例:
def convert_large_file_encoding(source_path, target_path, source_encoding, target_encoding):
# 打开原始文件
with open(source_path, 'r', encoding=source_encoding) as source_file:
# 打开目标文件
with open(target_path, 'w', encoding=target_encoding) as target_file:
# 逐行读取和写入
for line in source_file:
target_file.write(line)
示例调用
convert_large_file_encoding('large_source_file.txt', 'large_target_file.txt', 'utf-8', 'utf-16')
这种方法可以节省内存,并适用于处理大文件的编码转换。
4、使用第三方库chardet
在某些情况下,可能不知道源文件的编码格式。可以使用第三方库chardet
来自动检测文件的编码格式。以下是一个示例:
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
return result['encoding']
def convert_encoding_with_detection(source_path, target_path, target_encoding):
# 检测源文件编码
source_encoding = detect_encoding(source_path)
print(f"Detected source encoding: {source_encoding}")
# 转换编码
convert_encoding(source_path, target_path, source_encoding, target_encoding)
示例调用
convert_encoding_with_detection('unknown_encoding_file.txt', 'converted_file.txt', 'utf-16')
在这个示例中,我们使用chardet
库来检测源文件的编码,然后进行编码转换。
三、常见问题和解决方法
1、编码错误和异常处理
在处理文件编码转换时,可能会遇到各种编码错误,例如UnicodeDecodeError
和UnicodeEncodeError
。这些错误通常是由于源文件包含不符合指定编码格式的字符。可以使用异常处理来捕获和解决这些错误。以下是一个示例:
def convert_encoding_with_error_handling(source_path, target_path, source_encoding, target_encoding):
try:
# 打开原始文件并读取内容
with open(source_path, 'r', encoding=source_encoding, errors='replace') as source_file:
content = source_file.read()
# 打开目标文件并写入内容
with open(target_path, 'w', encoding=target_encoding) as target_file:
target_file.write(content)
except UnicodeDecodeError as e:
print(f"UnicodeDecodeError: {e}")
except UnicodeEncodeError as e:
print(f"UnicodeEncodeError: {e}")
示例调用
convert_encoding_with_error_handling('source_file_with_errors.txt', 'target_file.txt', 'utf-8', 'utf-16')
在这个示例中,我们使用errors='replace'
选项来替换无法解码的字符,避免解码错误。
2、处理不同平台的换行符
不同平台使用不同的换行符,例如Windows使用\r\n
,Unix和Linux使用\n
,而旧版Mac使用\r
。在进行文件编码转换时,可能需要处理这些换行符。可以使用Python的os.linesep
来获取当前平台的换行符,或者使用str.replace
方法来统一处理换行符。以下是一个示例:
import os
def convert_encoding_with_newlines(source_path, target_path, source_encoding, target_encoding):
# 打开原始文件并读取内容
with open(source_path, 'r', encoding=source_encoding) as source_file:
content = source_file.read()
# 统一换行符
content = content.replace('\r\n', '\n').replace('\r', '\n')
content = content.replace('\n', os.linesep)
# 打开目标文件并写入内容
with open(target_path, 'w', encoding=target_encoding) as target_file:
target_file.write(content)
示例调用
convert_encoding_with_newlines('source_file.txt', 'target_file.txt', 'utf-8', 'utf-16')
在这个示例中,我们统一了换行符,确保在不同平台上处理文件时不会出现问题。
3、批量处理文件
在某些情况下,可能需要批量处理多个文件的编码转换。可以使用Python的os
和glob
模块来遍历文件夹中的所有文件,并进行编码转换。以下是一个示例:
import os
import glob
def batch_convert_encoding(source_folder, target_folder, source_encoding, target_encoding):
# 创建目标文件夹
if not os.path.exists(target_folder):
os.makedirs(target_folder)
# 遍历源文件夹中的所有文件
for source_file_path in glob.glob(os.path.join(source_folder, '*')):
# 获取文件名
file_name = os.path.basename(source_file_path)
# 构造目标文件路径
target_file_path = os.path.join(target_folder, file_name)
# 转换编码
convert_encoding(source_file_path, target_file_path, source_encoding, target_encoding)
示例调用
batch_convert_encoding('source_folder', 'target_folder', 'utf-8', 'utf-16')
在这个示例中,我们遍历源文件夹中的所有文件,并将它们的编码转换为目标编码。这个方法非常适用于批量处理文件的编码转换。
四、总结
修改文件编码格式是处理文本文件时常见的任务。通过了解字符编码的基础知识,并使用Python中的open
函数、codecs
模块和第三方库chardet
,我们可以轻松地完成文件编码转换。在处理大文件、异常处理、换行符和批量处理文件时,也有相应的方法和技术。
1、主要方法总结
- 使用
open
函数读取和写入文件内容,进行编码转换。 - 使用
codecs
模块提供更灵活的字符编码处理。 - 使用
chardet
库自动检测文件编码。 - 处理大文件时逐行读取和写入文件。
- 处理编码错误和异常。
- 统一不同平台的换行符。
- 批量处理多个文件的编码转换。
2、实际应用
在实际应用中,修改文件编码格式可以帮助我们在不同平台和应用程序之间共享和处理文本文件。无论是处理日志文件、配置文件、数据文件还是其他文本文件,了解和掌握文件编码转换的技术都将提高我们的工作效率和数据处理能力。
希望这篇文章能帮助你更好地理解和应用Python来修改文件编码格式。通过掌握这些技术,你将能够更高效地处理各种文本文件,并在不同的应用场景中灵活应对编码问题。
相关问答FAQs:
如何判断一个文件的当前编码格式?
在处理文件编码格式之前,了解文件的当前编码是非常重要的。可以使用Python的chardet
库来检测文件的编码。通过读取文件的部分内容,该库能够提供一个最有可能的编码建议。这对于确保在转换编码时不会丢失或破坏数据非常有用。
在Python中修改文件编码的常用方法是什么?
可以使用Python内置的open()
函数来读取和写入文件。通过指定encoding
参数,可以轻松地将文件从一种编码转换为另一种。例如,读取一个UTF-8编码的文件并将其保存为ISO-8859-1编码,可以使用以下代码:
with open('input_file.txt', 'r', encoding='utf-8') as infile:
content = infile.read()
with open('output_file.txt', 'w', encoding='iso-8859-1') as outfile:
outfile.write(content)
这种方法简单直接,适合大多数情况。
修改文件编码时需要注意哪些事项?
在修改文件编码时,要确保目标编码格式能够支持原始内容中的所有字符。例如,UTF-8能够支持多种字符集,而某些其他编码(如ASCII或ISO-8859-1)可能无法处理特定的Unicode字符。此外,处理较大的文件时,需要考虑内存的使用情况,采用分块读取和写入的方式可以有效避免内存不足的问题。
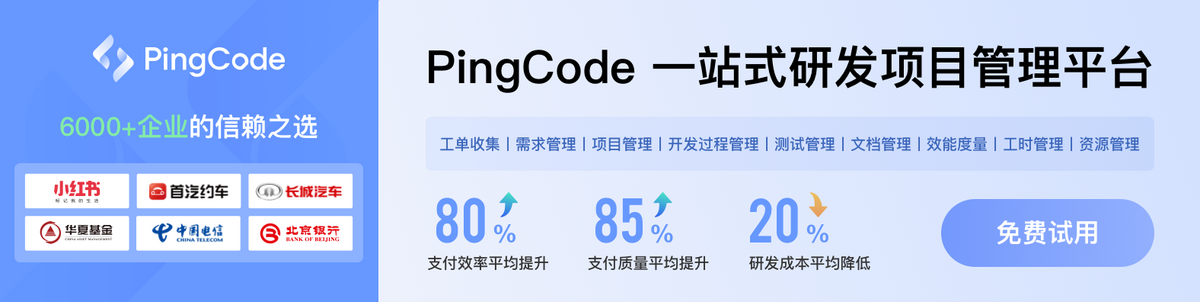