在Python中导入文件的主要方法包括使用内置的open
函数、利用pandas
库、通过csv
模块、借助json
模块和使用pickle
模块等。使用内置的open
函数是最常见的方式之一,适用于读取文本文件。以下将详细介绍如何使用open
函数导入文件。
使用open
函数时,首先需要确定文件的路径,然后指定打开模式(如读取、写入等)。读取文件内容后,可以进行相应的处理,最后别忘了关闭文件。
例如,读取一个文本文件的基本步骤如下:
# 打开文件
file = open('example.txt', 'r')
读取文件内容
content = file.read()
处理文件内容
print(content)
关闭文件
file.close()
这样做的好处是非常直接且适用于大多数简单的文件读取操作。接下来,我将详细介绍在Python中导入文件的其他方法。
一、使用open
函数
open
函数是Python内置的文件操作函数之一,适用于多种类型的文件操作,如读取、写入和追加等。以下是一些常见的使用场景:
1、读取文本文件
读取文本文件是最常见的文件操作之一。可以通过指定模式'r'
来打开文件进行读取。
with open('example.txt', 'r') as file:
content = file.read()
print(content)
使用with
语句可以确保文件在使用完毕后自动关闭,避免文件句柄未关闭导致的资源泄露问题。
2、逐行读取文件
有时候,文件内容较大,需要逐行读取进行处理,可以使用readline
或readlines
方法。
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
3、写入文件
使用open
函数也可以写入文件,通过指定模式'w'
或'a'
来创建或追加文件内容。
with open('example.txt', 'w') as file:
file.write("Hello, World!")
二、利用pandas
库
pandas
是一个强大的数据分析库,特别适合处理结构化数据,如CSV文件、Excel文件等。
1、读取CSV文件
pandas
提供了简便的方法来读取CSV文件,并将其转换为DataFrame。
import pandas as pd
df = pd.read_csv('example.csv')
print(df.head())
2、读取Excel文件
同样,pandas
也支持读取Excel文件。
df = pd.read_excel('example.xlsx')
print(df.head())
3、写入CSV文件
可以使用to_csv
方法将DataFrame写入CSV文件。
df.to_csv('output.csv', index=False)
三、通过csv
模块
Python内置的csv
模块专门用于处理CSV文件。
1、读取CSV文件
使用csv.reader
可以读取CSV文件,并逐行处理。
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
2、写入CSV文件
使用csv.writer
可以将数据写入CSV文件。
with open('output.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['name', 'age'])
writer.writerow(['Alice', 30])
四、借助json
模块
json
模块用于处理JSON格式的数据。
1、读取JSON文件
使用json.load
可以将JSON文件内容解析为Python对象。
import json
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
2、写入JSON文件
使用json.dump
可以将Python对象写入JSON文件。
data = {'name': 'Alice', 'age': 30}
with open('output.json', 'w') as file:
json.dump(data, file)
五、使用pickle
模块
pickle
模块用于序列化和反序列化Python对象,适用于保存和恢复复杂的数据结构。
1、序列化对象
使用pickle.dump
可以将Python对象序列化并写入文件。
import pickle
data = {'name': 'Alice', 'age': 30}
with open('data.pkl', 'wb') as file:
pickle.dump(data, file)
2、反序列化对象
使用pickle.load
可以从文件中读取并反序列化Python对象。
with open('data.pkl', 'rb') as file:
data = pickle.load(file)
print(data)
六、处理大文件
处理大文件时,需要注意内存的使用,可以通过分块读取文件来避免内存溢出。
1、逐行读取大文件
逐行读取大文件可以有效地控制内存使用。
with open('large_file.txt', 'r') as file:
for line in file:
process(line) # 处理每一行
2、分块读取大文件
可以通过指定块大小来分块读取大文件。
def read_in_chunks(file_object, chunk_size=1024):
while True:
data = file_object.read(chunk_size)
if not data:
break
yield data
with open('large_file.txt', 'r') as file:
for chunk in read_in_chunks(file):
process(chunk) # 处理每一个块
七、处理二进制文件
有时候需要处理二进制文件,如图像、音频等,可以通过指定模式'rb'
或'wb'
来读取或写入二进制文件。
1、读取二进制文件
读取二进制文件时,需要使用'rb'
模式。
with open('image.png', 'rb') as file:
data = file.read()
# 处理二进制数据
2、写入二进制文件
写入二进制文件时,需要使用'wb'
模式。
with open('output.png', 'wb') as file:
file.write(data)
八、文件路径管理
在处理文件时,通常需要管理文件路径,可以使用os
和pathlib
模块来简化路径管理。
1、使用os
模块
os
模块提供了多种路径处理函数。
import os
获取当前工作目录
cwd = os.getcwd()
print(cwd)
连接路径
path = os.path.join(cwd, 'example.txt')
print(path)
2、使用pathlib
模块
pathlib
模块提供了面向对象的路径处理方式。
from pathlib import Path
获取当前工作目录
cwd = Path.cwd()
print(cwd)
连接路径
path = cwd / 'example.txt'
print(path)
九、处理压缩文件
有时候需要处理压缩文件,可以使用zipfile
和tarfile
模块。
1、处理ZIP文件
使用zipfile
模块可以读取和写入ZIP文件。
import zipfile
解压ZIP文件
with zipfile.ZipFile('example.zip', 'r') as zip_ref:
zip_ref.extractall('extracted')
压缩文件
with zipfile.ZipFile('output.zip', 'w') as zip_ref:
zip_ref.write('example.txt')
2、处理TAR文件
使用tarfile
模块可以读取和写入TAR文件。
import tarfile
解压TAR文件
with tarfile.open('example.tar', 'r') as tar_ref:
tar_ref.extractall('extracted')
压缩文件
with tarfile.open('output.tar', 'w') as tar_ref:
tar_ref.add('example.txt')
十、处理配置文件
配置文件通常用于存储程序的配置信息,可以使用configparser
模块来处理INI格式的配置文件。
1、读取配置文件
使用configparser
模块可以读取INI格式的配置文件。
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
获取配置项
database = config['database']
host = database['host']
port = database['port']
print(f"Host: {host}, Port: {port}")
2、写入配置文件
可以使用configparser
模块将配置信息写入INI文件。
config = configparser.ConfigParser()
config['database'] = {'host': 'localhost', 'port': '5432'}
with open('config.ini', 'w') as configfile:
config.write(configfile)
十一、处理XML文件
XML文件是一种常见的数据交换格式,可以使用xml.etree.ElementTree
模块来处理XML文件。
1、读取XML文件
使用ElementTree
模块可以解析XML文件。
import xml.etree.ElementTree as ET
tree = ET.parse('example.xml')
root = tree.getroot()
遍历XML树
for child in root:
print(child.tag, child.attrib)
2、写入XML文件
可以使用ElementTree
模块创建和写入XML文件。
root = ET.Element("root")
child = ET.SubElement(root, "child")
child.text = "Hello, World!"
tree = ET.ElementTree(root)
tree.write("output.xml")
十二、处理YAML文件
YAML文件是一种人类可读的数据序列化格式,可以使用pyyaml
库来处理YAML文件。
1、读取YAML文件
使用pyyaml
库可以读取YAML文件。
import yaml
with open('example.yaml', 'r') as file:
data = yaml.safe_load(file)
print(data)
2、写入YAML文件
可以使用pyyaml
库将数据写入YAML文件。
data = {'name': 'Alice', 'age': 30}
with open('output.yaml', 'w') as file:
yaml.safe_dump(data, file)
十三、处理HDF5文件
HDF5文件是一种用于存储和组织大量数据的文件格式,可以使用h5py
库来处理HDF5文件。
1、读取HDF5文件
使用h5py
库可以读取HDF5文件。
import h5py
with h5py.File('example.h5', 'r') as file:
data = file['dataset'][:]
print(data)
2、写入HDF5文件
可以使用h5py
库将数据写入HDF5文件。
import numpy as np
data = np.random.random((100, 100))
with h5py.File('output.h5', 'w') as file:
file.create_dataset('dataset', data=data)
十四、处理数据库文件
数据库文件通常用于存储结构化数据,可以使用sqlite3
模块来处理SQLite数据库文件。
1、读取数据库文件
使用sqlite3
模块可以读取SQLite数据库文件。
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute('SELECT * FROM users')
rows = cursor.fetchall()
for row in rows:
print(row)
conn.close()
2、写入数据库文件
可以使用sqlite3
模块将数据写入SQLite数据库文件。
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute('INSERT INTO users (name, age) VALUES (?, ?)', ('Alice', 30))
conn.commit()
conn.close()
十五、处理音频文件
音频文件是一种常见的多媒体文件格式,可以使用wave
和pydub
库来处理音频文件。
1、读取音频文件
使用wave
模块可以读取WAV格式的音频文件。
import wave
with wave.open('example.wav', 'rb') as file:
params = file.getparams()
frames = file.readframes(params.nframes)
print(params)
2、写入音频文件
可以使用wave
模块将数据写入WAV格式的音频文件。
with wave.open('output.wav', 'wb') as file:
file.setnchannels(1)
file.setsampwidth(2)
file.setframerate(44100)
file.writeframes(frames)
十六、处理图像文件
图像文件是一种常见的多媒体文件格式,可以使用PIL
(Python Imaging Library)和opencv
库来处理图像文件。
1、读取图像文件
使用PIL
库可以读取图像文件。
from PIL import Image
image = Image.open('example.png')
image.show()
2、写入图像文件
可以使用PIL
库将图像数据写入文件。
image.save('output.png')
十七、处理视频文件
视频文件是一种常见的多媒体文件格式,可以使用opencv
库来处理视频文件。
1、读取视频文件
使用opencv
库可以读取视频文件。
import cv2
cap = cv2.VideoCapture('example.mp4')
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
cv2.imshow('frame', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
2、写入视频文件
可以使用opencv
库将视频数据写入文件。
fourcc = cv2.VideoWriter_fourcc(*'XVID')
out = cv2.VideoWriter('output.avi', fourcc, 20.0, (640, 480))
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
out.write(frame)
cap.release()
out.release()
通过以上多种方法,可以在Python中导入和处理不同类型的文件。选择合适的方法和工具,可以高效地完成文件操作任务。
相关问答FAQs:
在Python中导入文件需要注意哪些常见的步骤和技巧?
在导入文件时,确保文件路径正确是至关重要的。如果文件在当前工作目录中,可以直接使用相对路径。如果文件在其他目录中,使用绝对路径更为安全。此外,使用import
语句时,需确保文件中没有语法错误,这会导致导入失败。为了避免命名冲突,建议使用唯一的模块名称。
Python支持导入哪些类型的文件?
Python可以导入多种类型的文件,包括.py文件(Python模块)、.csv文件(数据文件)、.json文件(JSON数据格式)以及其他文本和二进制文件。对于非Python文件,可以使用相应的库,例如pandas
用于处理CSV文件,json
库用于解析JSON数据。
如何在导入文件时处理可能出现的错误?
在导入文件时,常见的错误包括ModuleNotFoundError
和FileNotFoundError
。为了解决这些问题,可以使用try...except
语句来捕捉异常,并提供友好的错误信息。此外,确保PYTHONPATH环境变量正确设置,以便Python能够找到需要导入的模块或文件。
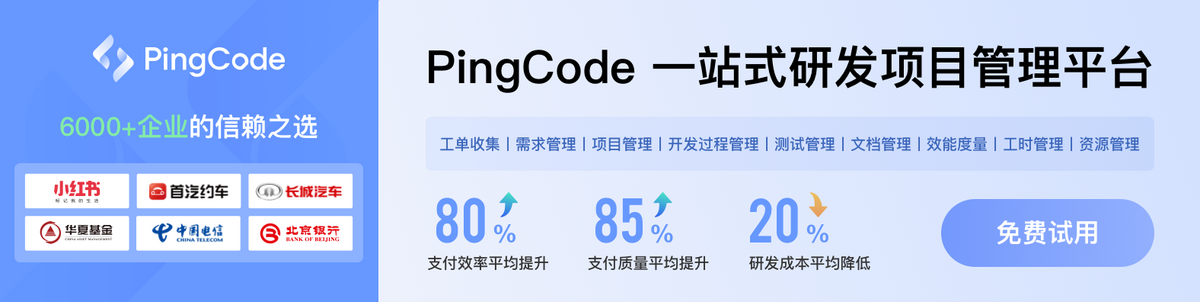