Python提高爬虫访问速度的方法有很多,例如使用异步编程、使用多线程、多进程、优化网络请求、使用高效的解析库、减少不必要的操作、使用代理、缓存和分布式爬虫等。其中,使用异步编程是一个非常有效的方法。异步编程可以在处理 I/O 操作时不阻塞主线程,这样可以在等待网络响应的同时进行其他的任务,从而提高爬虫的访问速度。
一、使用异步编程
异步编程是一种高效处理 I/O 操作的方法,特别适用于网络爬虫。Python 中的 asyncio
库可以实现异步编程,通过将网络请求设为异步任务,可以在等待响应的同时处理其他任务,从而大幅提高爬虫的访问速度。
1.1 异步编程基础
Python 的 asyncio
库是实现异步编程的基础。下面是一个简单的示例,展示了如何使用 asyncio
进行异步操作:
import asyncio
async def fetch_data():
print("Start fetching data...")
await asyncio.sleep(2)
print("Data fetched")
async def main():
await asyncio.gather(fetch_data(), fetch_data(), fetch_data())
asyncio.run(main())
在这个例子中,fetch_data
函数是一个异步函数,会在调用 await asyncio.sleep(2)
时暂停 2 秒钟,但在这 2 秒钟内,其他的 fetch_data
调用仍然可以继续进行。
1.2 使用 aiohttp
进行异步网络请求
aiohttp
是一个支持异步 HTTP 请求的库,可以与 asyncio
一起使用。下面是一个使用 aiohttp
实现异步网络请求的示例:
import aiohttp
import asyncio
async def fetch(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
async def main():
urls = ["http://example.com", "http://example.org", "http://example.net"]
tasks = [fetch(url) for url in urls]
responses = await asyncio.gather(*tasks)
for response in responses:
print(response)
asyncio.run(main())
在这个例子中,fetch
函数使用 aiohttp
发送 HTTP 请求,并在获取响应后返回响应内容。main
函数中创建了多个异步任务,并使用 asyncio.gather
并行执行这些任务。
二、使用多线程、多进程
多线程和多进程是提高爬虫访问速度的另一种有效方法。通过并行执行多个任务,可以充分利用系统资源,从而提高爬虫的效率。
2.1 多线程
Python 中的 threading
库可以实现多线程。下面是一个使用多线程进行网络请求的示例:
import threading
import requests
def fetch(url):
response = requests.get(url)
print(response.text)
urls = ["http://example.com", "http://example.org", "http://example.net"]
threads = [threading.Thread(target=fetch, args=(url,)) for url in urls]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
在这个例子中,fetch
函数使用 requests
库发送 HTTP 请求,并打印响应内容。然后,创建了多个线程,每个线程执行一个 fetch
任务。
2.2 多进程
Python 中的 multiprocessing
库可以实现多进程。下面是一个使用多进程进行网络请求的示例:
import multiprocessing
import requests
def fetch(url):
response = requests.get(url)
print(response.text)
urls = ["http://example.com", "http://example.org", "http://example.net"]
processes = [multiprocessing.Process(target=fetch, args=(url,)) for url in urls]
for process in processes:
process.start()
for process in processes:
process.join()
在这个例子中,fetch
函数与多线程示例中的 fetch
函数相同,但这里使用 multiprocessing.Process
创建了多个进程,每个进程执行一个 fetch
任务。
三、优化网络请求
优化网络请求是提高爬虫访问速度的关键。通过减少不必要的请求、使用持久连接、设置合理的超时时间等,可以显著提高爬虫的效率。
3.1 使用持久连接
持久连接可以减少建立连接的开销,从而提高爬虫的访问速度。requests
库的 Session
对象支持持久连接:
import requests
session = requests.Session()
urls = ["http://example.com", "http://example.org", "http://example.net"]
for url in urls:
response = session.get(url)
print(response.text)
在这个例子中,使用 requests.Session
创建了一个会话对象,并使用该会话对象发送多个请求,从而实现持久连接。
3.2 设置合理的超时时间
设置合理的超时时间可以避免长时间等待无响应的请求,从而提高爬虫的效率:
import requests
urls = ["http://example.com", "http://example.org", "http://example.net"]
for url in urls:
try:
response = requests.get(url, timeout=5)
print(response.text)
except requests.exceptions.Timeout:
print(f"Request to {url} timed out")
在这个例子中,使用 timeout
参数设置超时时间为 5 秒。如果请求在 5 秒内未响应,将抛出 requests.exceptions.Timeout
异常。
四、使用高效的解析库
选择高效的解析库可以显著提高爬虫的性能。常用的解析库包括 BeautifulSoup
、lxml
和 html5lib
等。
4.1 使用 lxml
lxml
是一个高效的解析库,性能优于 BeautifulSoup
。下面是一个使用 lxml
解析 HTML 的示例:
from lxml import etree
import requests
url = "http://example.com"
response = requests.get(url)
html = response.text
tree = etree.HTML(html)
titles = tree.xpath("//title/text()")
for title in titles:
print(title)
在这个例子中,使用 etree.HTML
解析 HTML 文本,并使用 xpath
提取标题。
4.2 使用 BeautifulSoup
与 lxml
结合
BeautifulSoup
提供了简单易用的接口,而 lxml
提供了高效的解析性能。可以将两者结合使用:
from bs4 import BeautifulSoup
import requests
url = "http://example.com"
response = requests.get(url)
html = response.text
soup = BeautifulSoup(html, "lxml")
titles = soup.find_all("title")
for title in titles:
print(title.get_text())
在这个例子中,使用 BeautifulSoup
提供的接口,并指定解析器为 lxml
,从而结合了两者的优点。
五、减少不必要的操作
减少不必要的操作可以提高爬虫的效率,例如避免重复请求、精简数据处理等。
5.1 避免重复请求
在爬取数据时,避免重复请求可以节省时间和资源。可以使用集合或数据库记录已访问的 URL:
import requests
visited_urls = set()
urls = ["http://example.com", "http://example.org", "http://example.net"]
for url in urls:
if url not in visited_urls:
response = requests.get(url)
print(response.text)
visited_urls.add(url)
在这个例子中,使用集合 visited_urls
记录已访问的 URL,避免重复请求。
5.2 精简数据处理
在处理数据时,尽量精简操作,避免复杂的计算和冗余的步骤。例如,在解析 HTML 时,只提取必要的数据:
from bs4 import BeautifulSoup
import requests
url = "http://example.com"
response = requests.get(url)
html = response.text
soup = BeautifulSoup(html, "lxml")
titles = soup.find_all("title")
for title in titles:
print(title.get_text())
在这个例子中,只提取了标题数据,避免了不必要的解析和处理。
六、使用代理
使用代理可以避免 IP 被封禁,从而提高爬虫的稳定性和访问速度。
6.1 免费代理
可以使用免费代理,但免费代理通常不稳定,速度较慢:
import requests
proxies = {
"http": "http://proxy.example.com:8080",
"https": "https://proxy.example.com:8080",
}
url = "http://example.com"
response = requests.get(url, proxies=proxies)
print(response.text)
在这个例子中,使用 proxies
参数指定代理服务器。
6.2 付费代理
付费代理通常更加稳定和快速,可以提高爬虫的访问速度:
import requests
proxies = {
"http": "http://username:password@proxy.example.com:8080",
"https": "https://username:password@proxy.example.com:8080",
}
url = "http://example.com"
response = requests.get(url, proxies=proxies)
print(response.text)
在这个例子中,使用 proxies
参数指定付费代理服务器,并提供用户名和密码进行身份验证。
七、缓存
缓存可以减少重复请求,提高爬虫的效率。可以使用内存缓存或磁盘缓存。
7.1 内存缓存
内存缓存可以使用字典或第三方库,例如 cachetools
:
import requests
import cachetools
cache = cachetools.LRUCache(maxsize=100)
def fetch(url):
if url in cache:
return cache[url]
response = requests.get(url)
cache[url] = response.text
return response.text
urls = ["http://example.com", "http://example.org", "http://example.net"]
for url in urls:
print(fetch(url))
在这个例子中,使用 cachetools.LRUCache
实现了一个简单的内存缓存,避免了重复请求。
7.2 磁盘缓存
磁盘缓存可以使用第三方库,例如 requests-cache
:
import requests
import requests_cache
requests_cache.install_cache("my_cache")
urls = ["http://example.com", "http://example.org", "http://example.net"]
for url in urls:
response = requests.get(url)
print(response.text)
在这个例子中,使用 requests_cache
实现了一个磁盘缓存,将请求结果缓存到磁盘上。
八、分布式爬虫
分布式爬虫可以通过多个节点并行爬取数据,提高爬虫的效率。常用的分布式爬虫框架包括 Scrapy
和 PySpider
。
8.1 使用 Scrapy
Scrapy
是一个功能强大的爬虫框架,支持分布式爬取和多种扩展:
import scrapy
class ExampleSpider(scrapy.Spider):
name = "example"
start_urls = ["http://example.com", "http://example.org", "http://example.net"]
def parse(self, response):
yield {"title": response.xpath("//title/text()").get()}
在命令行中运行:
scrapy runspider example_spider.py -o output.json
在这个例子中,定义了一个简单的 Scrapy
爬虫,爬取多个 URL 并提取标题数据。
8.2 使用 PySpider
PySpider
是另一个功能强大的爬虫框架,支持 Web 界面和分布式爬取:
from pyspider.libs.base_handler import *
class Handler(BaseHandler):
crawl_config = {}
@every(minutes=24 * 60)
def on_start(self):
self.crawl("http://example.com", callback=self.index_page)
def index_page(self, response):
for each in response.doc("a[href^='http']").items():
self.crawl(each.attr.href, callback=self.detail_page)
def detail_page(self, response):
return {"title": response.doc("title").text()}
在这个例子中,定义了一个简单的 PySpider
爬虫,爬取多个页面并提取标题数据。
九、总结
提高 Python 爬虫访问速度的方法有很多,包括使用异步编程、使用多线程、多进程、优化网络请求、使用高效的解析库、减少不必要的操作、使用代理、缓存和分布式爬虫等。在实际应用中,可以根据具体需求和场景,选择合适的方法和技术组合,来最大化爬虫的效率和性能。无论选择哪种方法,都需要注意爬取的合法性和道德规范,遵守网站的爬取规则和隐私政策。
相关问答FAQs:
如何优化Python爬虫的性能以提高访问速度?
为了优化Python爬虫的性能,可以考虑使用异步编程(如使用asyncio
和aiohttp
库)来并发发送请求,减少等待时间。此外,合理使用连接池和设置请求头信息也有助于提升速度。确保遵循网站的爬虫协议,避免被封禁。
使用哪些库可以提升Python爬虫的访问速度?
常用的库包括Scrapy
、requests
和aiohttp
。Scrapy
提供了强大的异步处理能力,能够并行地处理多个请求,而aiohttp
则专注于异步HTTP请求。选择适合项目需求的库,可以显著提升爬虫的访问效率。
如何处理爬虫中的请求限制问题以提高访问速度?
面对请求限制,可以通过设置合理的请求间隔、使用代理IP池和动态User-Agent来规避。这些方法不仅可以降低被封禁的风险,还能在一定程度上提高爬虫的访问速度。确保遵循robots.txt文件的规则,尊重网站的访问限制。
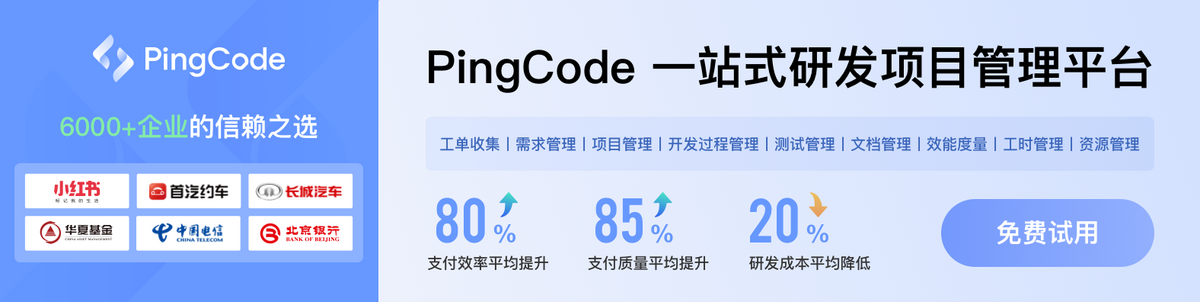