Python输出到txt文件的方法包括使用open函数、使用with语句管理文件上下文、使用write方法写入数据、使用writelines方法写入列表数据、使用print函数重定向输出到文件。
使用open
函数和write
方法是最常见的方式。使用open
函数可以打开一个文件,然后用write
方法将内容写入文件中。例如:
with open('output.txt', 'w') as file:
file.write('Hello, World!')
在这段代码中,open
函数以写模式('w')打开文件output.txt
,如果文件不存在,它将被创建。如果文件已经存在,它的内容将被清空。with
语句确保了文件在使用完毕后自动关闭,避免资源泄露。
接下来,我们将详细介绍Python中输出到txt文件的各种方法和使用场景。
一、使用open函数和write方法
1、基本用法
在Python中,打开文件的基本语法是使用open
函数。open
函数的第一个参数是文件名,第二个参数是模式。常用的模式有:
'r'
:以只读模式打开文件,文件必须存在。'w'
:以写入模式打开文件,如果文件存在会清空文件内容,如果文件不存在则创建一个新文件。'a'
:以追加模式打开文件,文件不存在则创建新文件。'b'
:以二进制模式打开文件(可以与其他模式结合使用,如'rb'
、'wb'
)。
例如:
file = open('output.txt', 'w')
file.write('Hello, World!')
file.close()
2、上下文管理器with
使用with
语句可以确保文件在使用完毕后自动关闭,这是推荐的方式:
with open('output.txt', 'w') as file:
file.write('Hello, World!')
3、写入多行
如果需要写入多行文本,可以使用多次write
方法:
with open('output.txt', 'w') as file:
file.write('Hello, World!\n')
file.write('This is a new line.\n')
二、使用writelines方法
1、基本用法
writelines
方法可以将一个字符串列表写入文件。每个字符串必须包含换行符:
lines = ['Hello, World!\n', 'This is a new line.\n']
with open('output.txt', 'w') as file:
file.writelines(lines)
2、自动添加换行符
如果字符串列表没有包含换行符,可以使用列表解析添加换行符:
lines = ['Hello, World!', 'This is a new line.']
with open('output.txt', 'w') as file:
file.writelines([line + '\n' for line in lines])
三、使用print函数重定向输出
1、基本用法
print
函数可以通过file
参数重定向输出到文件:
with open('output.txt', 'w') as file:
print('Hello, World!', file=file)
2、格式化输出
可以使用print
函数的sep
和end
参数控制输出格式:
with open('output.txt', 'w') as file:
print('Hello,', 'World!', sep=' ', end='\n', file=file)
四、文件模式详解
1、只读模式 'r'
以只读模式打开文件,如果文件不存在会报错:
try:
with open('output.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print("File not found.")
2、写入模式 'w'
以写入模式打开文件,会清空文件内容:
with open('output.txt', 'w') as file:
file.write('New content.')
3、追加模式 'a'
以追加模式打开文件,新内容会被添加到文件末尾:
with open('output.txt', 'a') as file:
file.write('Appended content.\n')
4、读写模式 'r+'
以读写模式打开文件,可以读取和写入:
with open('output.txt', 'r+') as file:
content = file.read()
print('Original content:', content)
file.write('New content.')
5、二进制模式 'b'
以二进制模式打开文件:
with open('output.txt', 'wb') as file:
file.write(b'Binary content.\n')
五、处理大文件
1、逐行读取
读取大文件时,避免一次性读取整个文件,可以逐行读取:
with open('large_file.txt', 'r') as file:
for line in file:
process(line) # process is a placeholder for actual processing logic
2、逐块读取
按块读取文件内容,可以指定块的大小:
with open('large_file.txt', 'r') as file:
while True:
chunk = file.read(1024) # 读取1024字节
if not chunk:
break
process(chunk) # process is a placeholder for actual processing logic
六、其他常见操作
1、文件指针操作
文件指针位置可以通过seek
和tell
方法操作:
with open('output.txt', 'r+') as file:
file.seek(0, 2) # 移动到文件末尾
file.write('Appended content.')
file.seek(0) # 移动到文件开头
content = file.read()
print('Updated content:', content)
2、文件检查
在操作文件前,可以检查文件是否存在:
import os
if os.path.exists('output.txt'):
with open('output.txt', 'r') as file:
content = file.read()
print(content)
else:
print("File does not exist.")
3、文件删除
删除文件可以使用os.remove
方法:
import os
if os.path.exists('output.txt'):
os.remove('output.txt')
print("File deleted.")
else:
print("File does not exist.")
七、总结
Python提供了多种方式将数据输出到txt文件,包括使用open
函数、with
语句、write
方法、writelines
方法和print
函数重定向输出。不同的方法适用于不同的场景,如逐行写入、批量写入和格式化输出等。同时,了解文件模式和文件指针操作有助于更灵活地处理文件。
通过本文的介绍,相信读者能够掌握Python输出到txt文件的各种方法,并在实际应用中灵活运用这些技术。
希望本文对您有所帮助。如果有任何问题或建议,欢迎在评论区交流讨论。
相关问答FAQs:
如何在Python中创建一个新的txt文件并写入内容?
在Python中,可以使用内置的open()
函数来创建一个新的txt文件并写入内容。通过设置文件模式为'w'
(写入模式),您可以创建一个新文件(如果文件已存在,则会覆盖它),并使用write()
方法将文本写入文件。例如:
with open('output.txt', 'w') as file:
file.write('Hello, World!')
这段代码将在当前目录下创建一个名为output.txt
的文件,并写入“Hello, World!”这段文本。
如何向已存在的txt文件追加内容?
如果您想在一个已存在的txt文件中添加内容而不覆盖原有内容,可以使用'a'
模式(追加模式)打开文件。这样,您可以在文件末尾添加新的文本。示例如下:
with open('output.txt', 'a') as file:
file.write('\nThis is an additional line.')
以上代码将在output.txt
文件的末尾添加一行新内容。
如何读取txt文件中的内容并在控制台输出?
要读取txt文件中的内容,可以使用open()
函数与'r'
模式(读取模式)。使用read()
方法可以读取整个文件内容,或者使用readlines()
方法逐行读取。下面是读取文件并输出到控制台的示例:
with open('output.txt', 'r') as file:
content = file.read()
print(content)
这段代码将打开output.txt
文件,读取所有内容,并将其打印到控制台。
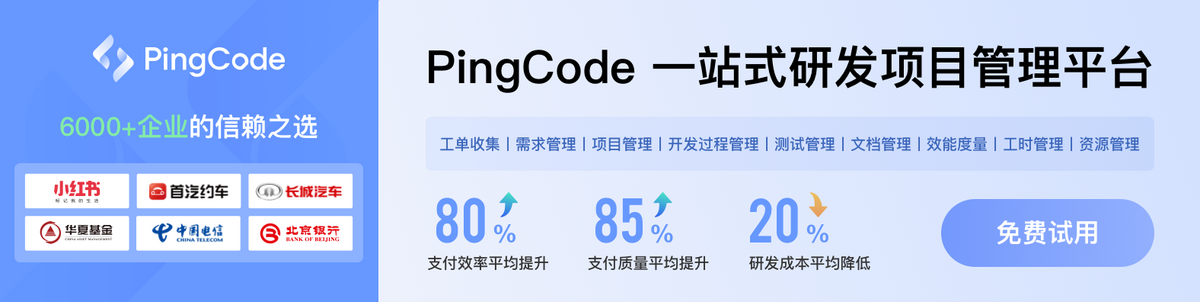