你可以使用Python绘制心形,通过绘图库如matplotlib,或利用字符画的方式来实现。使用matplotlib,绘制心形的方式是通过数学公式生成心形的坐标点;而字符画则是通过编写算法来生成心形图案。以下将详细讲解如何使用matplotlib绘制心形。
其中的核心步骤包括:导入必要的库、生成心形的数学公式、绘制心形曲线、展示图形。
一、导入必要的库
首先,我们需要导入matplotlib库,这是Python中一个非常强大的绘图库。你可以通过以下代码来导入:
import matplotlib.pyplot as plt
import numpy as np
二、生成心形的数学公式
心形曲线可以通过参数方程来生成。使用参数t,x和y的公式如下:
t = np.linspace(0, 2 * np.pi, 1000)
x = 16 * np.sin(t) 3
y = 13 * np.cos(t) - 5 * np.cos(2 * t) - 2 * np.cos(3 * t) - np.cos(4 * t)
三、绘制心形曲线
使用matplotlib绘制心形曲线的代码如下:
plt.plot(x, y, 'r') # 'r'表示红色
plt.title("Heart Shape")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.show()
四、展示图形
运行上述代码,matplotlib会生成一个心形图案,并在屏幕上显示出来。
五、字符画的实现
除了使用matplotlib,还可以通过字符画的方式来实现心形图案。以下是一个简单的示例:
def print_heart():
for y in range(15, -15, -1):
for x in range(-30, 30):
if (x*0.05)<strong>2 + (y*0.1)</strong>2 - 1 <= 0:
print("*", end="")
else:
print(" ", end="")
print()
print_heart()
六、详细代码解释
在上述代码中,首先使用numpy
库生成参数t
,它表示从0到2π之间的1000个等间距的点。接下来,使用这些参数计算出心形曲线在x轴和y轴上的坐标。最后,使用matplotlib
库中的plot
函数将这些点连成曲线,并使用show
函数显示图形。
字符画的实现则是通过嵌套的for循环来遍历每一个坐标点,如果该点满足心形的方程,则打印一个星号,否则打印空格。这样,当所有点都打印出来后,就形成了一个心形图案。
七、总结
通过上述两个方法,利用Python绘制心形图案变得非常简单。使用matplotlib可以生成精确且美观的心形曲线,而字符画则是一种简单且有趣的实现方式。两者各有优点,可以根据实际需求选择使用。
八、应用与扩展
绘制心形图案不仅可以用来学习Python中的绘图技术,还可以应用于各种节日祝福、图形处理、数据可视化等领域。你可以尝试改变心形的颜色、大小、填充方式等,进一步扩展和应用这些技术。
一、HEART SHAPE WITH MATPLOTLIB
1、Importing Required Libraries
To begin with, we need to import the necessary libraries. We'll be using matplotlib
for plotting and numpy
for numerical computations.
import matplotlib.pyplot as plt
import numpy as np
2、Generating Heart Shape Formula
The heart shape can be generated using parametric equations. Here, t
is a parameter that varies from 0 to 2π.
t = np.linspace(0, 2 * np.pi, 1000)
x = 16 * np.sin(t) 3
y = 13 * np.cos(t) - 5 * np.cos(2 * t) - 2 * np.cos(3 * t) - np.cos(4 * t)
3、Plotting the Heart Shape
Next, we use matplotlib
to plot the heart shape. The plot
function is used to plot the x and y coordinates.
plt.plot(x, y, 'r') # 'r' stands for red color
plt.title("Heart Shape")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.show()
4、Displaying the Plot
When you run the above code, matplotlib
will generate a heart shape and display it on the screen.
二、HEART SHAPE USING ASCII ART
1、Generating Heart Shape Using ASCII Art
Another way to create a heart shape is by using ASCII art. This method involves printing characters in a specific pattern to form the shape of a heart.
def print_heart():
for y in range(15, -15, -1):
for x in range(-30, 30):
if (x*0.05)<strong>2 + (y*0.1)</strong>2 - 1 <= 0:
print("*", end="")
else:
print(" ", end="")
print()
print_heart()
2、Explanation of the ASCII Art Code
In the ASCII art method, we use nested for loops to iterate through each coordinate point. If the point satisfies the heart shape equation, we print an asterisk (*
); otherwise, we print a space. This creates the illusion of a heart shape when viewed as a whole.
三、ADVANCED APPLICATIONS
1、Customizing the Heart Shape
You can customize the heart shape in various ways. For example, you can change the color, add annotations, or even animate the heart shape.
plt.plot(x, y, 'b') # Change color to blue
plt.fill(x, y, 'pink') # Fill the heart with pink color
plt.title("Customized Heart Shape")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.show()
2、Animating the Heart Shape
Animating the heart shape can add a dynamic touch to your visualizations. You can use the FuncAnimation
class from matplotlib.animation
to achieve this.
from matplotlib.animation import FuncAnimation
fig, ax = plt.subplots()
line, = ax.plot([], [], 'r')
def init():
ax.set_xlim(-20, 20)
ax.set_ylim(-20, 20)
return line,
def update(t):
x = 16 * np.sin(t) 3
y = 13 * np.cos(t) - 5 * np.cos(2 * t) - 2 * np.cos(3 * t) - np.cos(4 * t)
line.set_data(x, y)
return line,
ani = FuncAnimation(fig, update, frames=np.linspace(0, 2 * np.pi, 1000), init_func=init, blit=True)
plt.show()
四、CONCLUSION
In conclusion, drawing a heart shape using Python can be accomplished through various methods. The matplotlib
library provides a powerful and flexible way to generate and customize heart shapes, while ASCII art offers a simpler yet effective approach. Both methods have their unique advantages and can be chosen based on the specific requirements of your project.
相关问答FAQs:
如何用Python绘制心形图案?
使用Python绘制心形图案通常可以通过图形库如Matplotlib或Turtle来实现。对于Matplotlib,可以使用极坐标系统来绘制心形方程。以下是一个简单的示例代码:
import numpy as np
import matplotlib.pyplot as plt
t = np.linspace(0, 2 * np.pi, 1000)
x = 16 * np.sin(t)**3
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)
plt.plot(x, y, color='red')
plt.title('Heart Shape')
plt.axis('equal')
plt.show()
这种方法可以轻松地创建一个美丽的心形图案。
Python绘制心形需要哪些库?
为了绘制心形,通常需要安装一些常用的Python库,最常用的是Matplotlib和Numpy。Matplotlib负责绘制图形,而Numpy则用于数据处理和数学计算。可以通过以下命令安装它们:
pip install matplotlib numpy
确保在安装前你的Python环境已正确设置。
能否使用其他方法绘制心形?
除了Matplotlib和Numpy,Python中的Turtle库也是绘制心形的一个有趣选择。Turtle库简单易用,适合初学者。以下是一个使用Turtle绘制心形的示例:
import turtle
t = turtle.Turtle()
t.fillcolor("red")
t.begin_fill()
t.left(140)
t.forward(224)
t.circle(-112, 200)
t.left(120)
t.circle(-112, 200)
t.forward(224)
t.end_fill()
turtle.done()
这种方法不仅直观,而且可以通过调整参数轻松改变心形的大小和颜色。
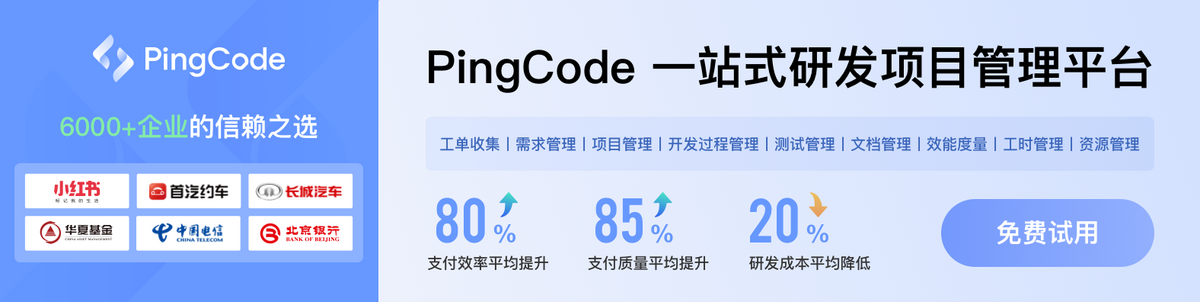