要用Python爬取题库,可以使用requests库、BeautifulSoup库、Scrapy框架、Selenium库。其中,使用requests库和BeautifulSoup库是最常见的方法。具体实现时,可以通过发送HTTP请求获取网页内容,然后使用BeautifulSoup解析HTML结构,提取题库信息。详细步骤包括:确定目标网站、发送HTTP请求、解析HTML、提取数据、存储数据。以下我们将详细介绍如何使用requests和BeautifulSoup爬取题库。
一、确定目标网站
在开始爬取之前,首先要确定目标网站及其页面结构。可以通过浏览器的开发者工具查看网页的HTML结构,找到题目所在的标签和属性。
二、发送HTTP请求
使用requests库发送HTTP请求获取网页内容。requests库是一个简单但功能强大的HTTP库,可以帮助我们轻松地发送GET和POST请求。
import requests
url = 'http://example.com/questions'
response = requests.get(url)
html_content = response.text
三、解析HTML
使用BeautifulSoup库解析HTML内容。BeautifulSoup是一个可以从HTML或XML文件中提取数据的Python库,它能以一种自然的方式导航、搜索和修改解析树。
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
四、提取数据
根据页面结构,使用BeautifulSoup的方法找到题目所在的标签,并提取题目内容。
questions = soup.find_all('div', class_='question')
for question in questions:
question_text = question.get_text()
print(question_text)
五、存储数据
可以将提取到的题目存储到文件或数据库中。这里我们将题目存储到一个文本文件中。
with open('questions.txt', 'w') as file:
for question in questions:
question_text = question.get_text()
file.write(question_text + '\n')
六、实例代码
以下是一个完整的实例代码,用于爬取某个题库网站的题目,并将其存储到文本文件中。
import requests
from bs4 import BeautifulSoup
def fetch_questions(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
questions = soup.find_all('div', class_='question')
return [question.get_text() for question in questions]
def save_questions_to_file(questions, filename):
with open(filename, 'w') as file:
for question in questions:
file.write(question + '\n')
def main():
url = 'http://example.com/questions'
questions = fetch_questions(url)
save_questions_to_file(questions, 'questions.txt')
if __name__ == '__main__':
main()
七、使用Scrapy框架
Scrapy是一个用于爬取网站并提取结构化数据的强大框架。相比requests和BeautifulSoup,Scrapy提供了更高级的功能和更高的性能。
1、安装Scrapy
pip install scrapy
2、创建Scrapy项目
scrapy startproject question_spider
cd question_spider
3、定义Spider
在spiders
目录下创建一个新的Spider,用于定义爬取逻辑。
import scrapy
class QuestionSpider(scrapy.Spider):
name = 'question'
start_urls = ['http://example.com/questions']
def parse(self, response):
questions = response.css('div.question')
for question in questions:
yield {
'question': question.get_text()
}
4、运行Spider
scrapy crawl question -o questions.json
以上命令会运行Spider并将爬取到的题目存储到questions.json
文件中。
八、使用Selenium
Selenium是一个用于自动化浏览器操作的工具,适用于需要处理动态内容的网站。
1、安装Selenium
pip install selenium
2、安装浏览器驱动
Selenium需要一个浏览器驱动来控制浏览器。以Chrome为例,可以从ChromeDriver下载页面下载并安装ChromeDriver。
3、使用Selenium爬取题库
from selenium import webdriver
from bs4 import BeautifulSoup
def fetch_questions_with_selenium(url):
driver = webdriver.Chrome()
driver.get(url)
html_content = driver.page_source
driver.quit()
soup = BeautifulSoup(html_content, 'html.parser')
questions = soup.find_all('div', class_='question')
return [question.get_text() for question in questions]
def save_questions_to_file(questions, filename):
with open(filename, 'w') as file:
for question in questions:
file.write(question + '\n')
def main():
url = 'http://example.com/questions'
questions = fetch_questions_with_selenium(url)
save_questions_to_file(questions, 'questions.txt')
if __name__ == '__main__':
main()
九、处理反爬虫措施
在实际爬取过程中,可能会遇到反爬虫措施。可以通过以下方法绕过反爬虫:
1、设置请求头
通过设置User-Agent等请求头来模拟浏览器请求。
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
2、使用代理
通过使用代理IP来避免被封禁。
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get(url, headers=headers, proxies=proxies)
3、随机延时
通过随机延时来避免频繁请求导致的封禁。
import time
import random
time.sleep(random.uniform(1, 3))
十、总结
使用Python爬取题库需要了解网页结构、发送HTTP请求、解析HTML、提取数据、存储数据等步骤。requests库和BeautifulSoup库是最常见的方法,也可以使用Scrapy框架和Selenium库来处理更复杂的情况。在实际操作中,可能会遇到反爬虫措施,需要通过设置请求头、使用代理、随机延时等方法绕过反爬虫。通过以上方法,您可以轻松地使用Python爬取题库信息,并将其存储到文件或数据库中。
相关问答FAQs:
用Python爬取题库需要哪些基础知识?
在开始爬取题库之前,建议您熟悉Python的基础语法、数据结构以及常用的库,如requests和BeautifulSoup。了解HTML和CSS的基本知识也非常重要,因为这将帮助您解析网页的结构。此外,掌握一些网络协议和网页请求的概念,可以使您更有效地进行数据抓取。
爬取题库时需要注意哪些法律和道德问题?
在进行爬虫操作时,尊重网站的robots.txt文件是非常重要的。该文件指明了网站允许和禁止爬取的部分。此外,尽量避免对网站造成过多的负载,比如设置合理的请求间隔,避免频繁请求同一页面。对于一些需要登录或提供API的题库,确保您遵循相关的使用条款。
如何处理爬取过程中遇到的反爬虫机制?
网站通常会使用多种反爬虫机制来防止自动化爬取,比如IP封禁、验证码、和请求频率限制等。如果遇到这些问题,可以尝试使用代理IP、设置随机的请求头或使用延时请求来规避。同时,使用selenium等工具模拟人类的浏览行为,也能有效应对一些反爬虫措施。
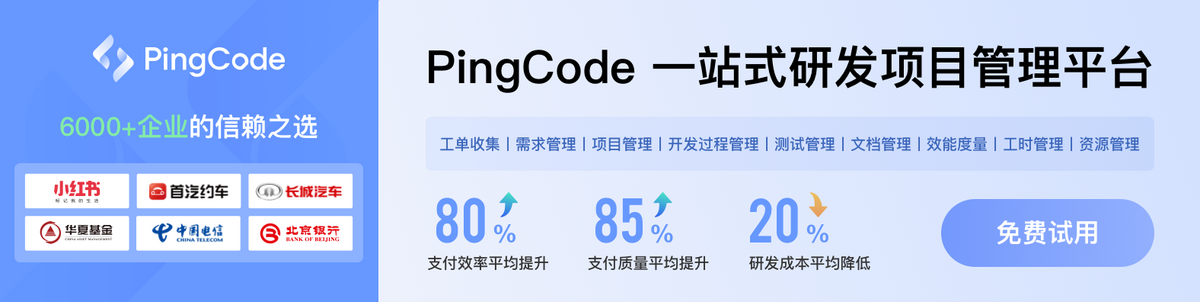