在Python中使用requests
库处理音频文件涉及多个步骤,包括发送、接收和处理音频数据。requests库不能直接处理音频数据,但它可以用于发送和接收音频文件。你可以使用requests
库上传音频文件到服务器或从服务器下载音频文件。下面将详细介绍如何使用requests
库处理音频文件。
一、上传音频文件
1.1 发送音频文件
上传音频文件到服务器的一个常见任务是发送文件作为POST请求的一部分。你可以使用requests
库的post
方法,并将音频文件作为文件对象传递给files
参数。
import requests
url = 'http://example.com/upload'
file_path = 'path_to_your_audio_file.wav'
with open(file_path, 'rb') as f:
files = {'file': f}
response = requests.post(url, files=files)
print(response.status_code)
print(response.text)
在这个例子中,我们打开音频文件并将其作为二进制文件对象传递给files
参数,然后发送POST请求到服务器。服务器的响应可以通过response
对象进行处理。
1.2 附加数据
有时,除了音频文件之外,你还需要发送额外的数据,例如元数据。你可以将这些数据放入POST请求的data
参数中。
import requests
url = 'http://example.com/upload'
file_path = 'path_to_your_audio_file.wav'
with open(file_path, 'rb') as f:
files = {'file': f}
data = {'description': 'This is an audio file', 'author': 'John Doe'}
response = requests.post(url, files=files, data=data)
print(response.status_code)
print(response.text)
在这个例子中,我们在POST请求中同时发送了音频文件和附加数据。
二、下载音频文件
2.1 接收音频文件
从服务器下载音频文件是另一个常见任务。你可以使用requests
库的get
方法来获取音频文件,然后将其保存到本地文件系统。
import requests
url = 'http://example.com/audio_file.wav'
response = requests.get(url)
file_path = 'downloaded_audio_file.wav'
with open(file_path, 'wb') as f:
f.write(response.content)
print('File downloaded successfully')
在这个例子中,我们发送GET请求到服务器以获取音频文件,并将响应内容保存到本地文件中。
2.2 检查文件类型
在处理下载的音频文件时,检查文件类型是个好习惯。你可以通过检查响应头中的Content-Type
字段来确保下载的是音频文件。
import requests
url = 'http://example.com/audio_file.wav'
response = requests.get(url)
if response.headers['Content-Type'] == 'audio/wav':
file_path = 'downloaded_audio_file.wav'
with open(file_path, 'wb') as f:
f.write(response.content)
print('File downloaded successfully')
else:
print('The downloaded file is not an audio file')
在这个例子中,我们检查响应头中的Content-Type
字段,确保下载的文件是WAV音频文件。
三、处理音频数据
3.1 使用pydub进行处理
requests
库只能用于上传和下载音频文件,要处理音频数据,你需要使用第三方库,例如pydub
。pydub
可以用于音频文件的转换、分割、合并等操作。
首先,安装pydub
库:
pip install pydub
然后,使用pydub
处理音频文件:
from pydub import AudioSegment
file_path = 'path_to_your_audio_file.wav'
audio = AudioSegment.from_wav(file_path)
切割音频文件
start_time = 10000 # 单位为毫秒
end_time = 20000
segment = audio[start_time:end_time]
保存切割后的音频文件
segment.export('segment.wav', format='wav')
print('Audio segment saved successfully')
在这个例子中,我们使用pydub
加载音频文件,并提取指定时间段的音频片段,然后将其保存为新的音频文件。
3.2 转换音频格式
你还可以使用pydub
将音频文件转换为其他格式:
from pydub import AudioSegment
file_path = 'path_to_your_audio_file.wav'
audio = AudioSegment.from_wav(file_path)
转换为MP3格式
audio.export('audio_file.mp3', format='mp3')
print('Audio file converted to MP3 format successfully')
在这个例子中,我们将WAV格式的音频文件转换为MP3格式。
四、与API交互
有时,你需要与提供音频处理功能的API服务进行交互。例如,使用Google Cloud Speech-to-Text API进行语音识别。
4.1 Google Cloud Speech-to-Text API
首先,安装Google Cloud客户端库:
pip install google-cloud-speech
然后,使用以下代码与Google Cloud Speech-to-Text API进行交互:
import os
from google.cloud import speech_v1p1beta1 as speech
from google.oauth2 import service_account
credentials = service_account.Credentials.from_service_account_file('path_to_your_service_account_key.json')
client = speech.SpeechClient(credentials=credentials)
file_path = 'path_to_your_audio_file.wav'
with open(file_path, 'rb') as f:
audio_content = f.read()
audio = speech.RecognitionAudio(content=audio_content)
config = speech.RecognitionConfig(
encoding=speech.RecognitionConfig.AudioEncoding.LINEAR16,
sample_rate_hertz=16000,
language_code='en-US'
)
response = client.recognize(config=config, audio=audio)
for result in response.results:
print('Transcript:', result.alternatives[0].transcript)
在这个例子中,我们使用Google Cloud Speech-to-Text API进行语音识别,并输出识别结果。
4.2 处理长音频文件
对于长时间的音频文件,可以使用Google Cloud Speech-to-Text API的异步模式:
import os
from google.cloud import speech_v1p1beta1 as speech
from google.oauth2 import service_account
credentials = service_account.Credentials.from_service_account_file('path_to_your_service_account_key.json')
client = speech.SpeechClient(credentials=credentials)
file_path = 'path_to_your_audio_file.wav'
with open(file_path, 'rb') as f:
audio_content = f.read()
audio = speech.RecognitionAudio(content=audio_content)
config = speech.RecognitionConfig(
encoding=speech.RecognitionConfig.AudioEncoding.LINEAR16,
sample_rate_hertz=16000,
language_code='en-US'
)
operation = client.long_running_recognize(config=config, audio=audio)
print('Waiting for operation to complete...')
response = operation.result(timeout=90)
for result in response.results:
print('Transcript:', result.alternatives[0].transcript)
在这个例子中,我们使用Google Cloud Speech-to-Text API的异步模式处理长时间的音频文件。
五、错误处理与调试
5.1 捕获异常
在处理音频文件时,可能会遇到各种错误,例如文件不存在、网络问题等。你可以使用try-except块捕获这些异常并进行处理。
import requests
url = 'http://example.com/upload'
file_path = 'path_to_your_audio_file.wav'
try:
with open(file_path, 'rb') as f:
files = {'file': f}
response = requests.post(url, files=files)
response.raise_for_status()
print('File uploaded successfully')
except requests.exceptions.RequestException as e:
print(f'An error occurred: {e}')
except FileNotFoundError:
print('The specified file was not found')
在这个例子中,我们捕获了请求异常和文件未找到异常,并输出错误信息。
5.2 调试信息
在调试代码时,输出调试信息是非常有用的。你可以使用Python的logging
模块来记录调试信息。
import logging
logging.basicConfig(level=logging.DEBUG)
url = 'http://example.com/upload'
file_path = 'path_to_your_audio_file.wav'
try:
with open(file_path, 'rb') as f:
files = {'file': f}
response = requests.post(url, files=files)
response.raise_for_status()
logging.debug('File uploaded successfully')
except requests.exceptions.RequestException as e:
logging.error(f'An error occurred: {e}')
except FileNotFoundError:
logging.error('The specified file was not found')
在这个例子中,我们使用logging
模块记录调试信息和错误信息。
六、总结
使用requests
库处理音频文件主要涉及上传和下载音频文件。requests库不能直接处理音频数据,但它可以用于发送和接收音频文件。在上传音频文件时,你可以将音频文件作为文件对象传递给files
参数,并通过data
参数附加额外数据。在下载音频文件时,你可以使用get
方法获取音频文件,并将其保存到本地文件系统。
要处理音频数据,可以使用pydub
库进行音频文件的转换、分割和合并等操作。此外,你还可以与提供音频处理功能的API服务进行交互,例如Google Cloud Speech-to-Text API进行语音识别。在处理音频文件时,捕获异常和输出调试信息是非常重要的,可以帮助你发现和解决问题。
通过掌握这些技巧和方法,你可以有效地使用Python处理音频文件,满足各种实际需求。
相关问答FAQs:
如何使用Python 3中的requests库下载音频文件?
使用requests库下载音频文件非常简单。你只需使用GET请求获取音频文件的URL,并将其内容写入本地文件。以下是一个示例代码:
import requests
url = '音频文件的URL'
response = requests.get(url)
with open('downloaded_audio.mp3', 'wb') as audio_file:
audio_file.write(response.content)
确保替换URL为实际音频文件的链接,运行代码后,音频文件将保存在当前目录下。
在请求音频文件时,如何处理请求失败的情况?
在下载音频文件时,可能会遇到请求失败的情况(如404或503错误)。为了处理这些错误,可以检查响应的状态码,并在失败时进行相应的处理。示例代码如下:
response = requests.get(url)
if response.status_code == 200:
with open('downloaded_audio.mp3', 'wb') as audio_file:
audio_file.write(response.content)
else:
print(f"下载失败,状态码:{response.status_code}")
这样可以确保你的程序能够优雅地处理错误情况。
使用requests库下载音频文件时,是否需要考虑文件大小和下载速度?
在下载大文件时,建议使用流模式来避免占用过多内存。使用stream=True
参数可以逐块下载文件。以下是一个示例:
response = requests.get(url, stream=True)
with open('downloaded_audio.mp3', 'wb') as audio_file:
for chunk in response.iter_content(chunk_size=1024):
if chunk: # 过滤掉保持活动的块
audio_file.write(chunk)
这种方法可以有效降低内存使用,特别是在下载较大的音频文件时。
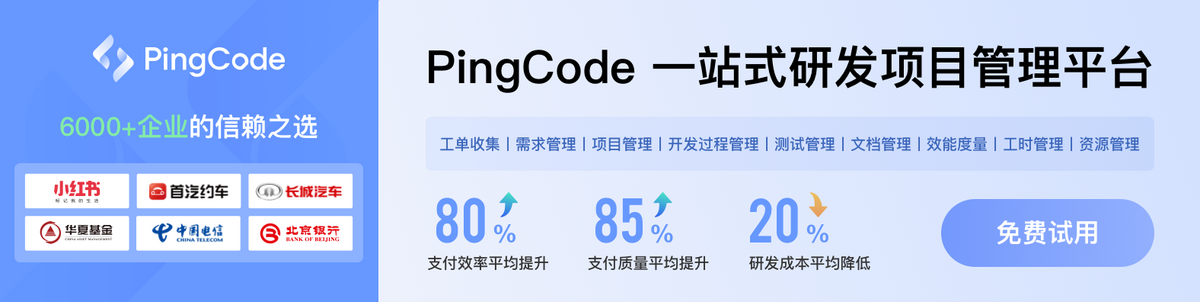