在Python中选取某行内容的方法有很多,主要取决于数据的存储格式及具体的需求。常见的方法包括读取文本文件、读取CSV文件、读取Excel文件、读取数据库中的数据。其中,读取CSV和Excel文件较为常见,尤其在数据分析领域。下面我将详细介绍如何使用Python选取某行内容,并重点讲解如何使用Pandas库来处理CSV和Excel文件。
一、读取文本文件
在处理纯文本文件时,可以使用Python的内置函数进行文件操作。以下是一个简单的例子,演示如何读取文本文件并选取指定行的内容。
def read_specific_line(file_path, line_number):
with open(file_path, 'r') as file:
lines = file.readlines()
if line_number <= len(lines):
return lines[line_number - 1].strip()
else:
raise ValueError("Line number exceeds the number of lines in the file.")
file_path = 'example.txt'
line_number = 3
print(read_specific_line(file_path, line_number))
在上述代码中,read_specific_line
函数打开文件并读取所有行,然后通过索引获取指定行的内容。
二、读取CSV文件
CSV文件是数据分析中常用的数据格式之一。Pandas库提供了强大的功能来处理CSV文件,包括读取特定行。以下是一个例子,演示如何使用Pandas读取CSV文件并选取指定行的内容。
import pandas as pd
def read_specific_row_from_csv(file_path, row_number):
df = pd.read_csv(file_path)
if row_number < len(df):
return df.iloc[row_number]
else:
raise ValueError("Row number exceeds the number of rows in the CSV file.")
file_path = 'example.csv'
row_number = 3
print(read_specific_row_from_csv(file_path, row_number))
在上述代码中,read_specific_row_from_csv
函数使用pd.read_csv
读取CSV文件,并通过iloc
方法选取指定行的数据。
三、读取Excel文件
Excel文件在数据处理和分析中也非常常见。Pandas库同样提供了强大的功能来处理Excel文件。以下是一个例子,演示如何使用Pandas读取Excel文件并选取指定行的内容。
import pandas as pd
def read_specific_row_from_excel(file_path, sheet_name, row_number):
df = pd.read_excel(file_path, sheet_name=sheet_name)
if row_number < len(df):
return df.iloc[row_number]
else:
raise ValueError("Row number exceeds the number of rows in the Excel sheet.")
file_path = 'example.xlsx'
sheet_name = 'Sheet1'
row_number = 3
print(read_specific_row_from_excel(file_path, sheet_name, row_number))
在上述代码中,read_specific_row_from_excel
函数使用pd.read_excel
读取Excel文件,并通过iloc
方法选取指定行的数据。
四、读取数据库中的数据
除了文件操作外,读取数据库中的数据也是一个常见的需求。Python提供了多种库来操作数据库,例如sqlite3、SQLAlchemy等。以下是一个例子,演示如何使用sqlite3读取数据库并选取指定行的内容。
import sqlite3
def read_specific_row_from_db(db_path, table_name, row_id):
conn = sqlite3.connect(db_path)
cursor = conn.cursor()
cursor.execute(f"SELECT * FROM {table_name} WHERE id = ?", (row_id,))
row = cursor.fetchone()
conn.close()
return row
db_path = 'example.db'
table_name = 'example_table'
row_id = 3
print(read_specific_row_from_db(db_path, table_name, row_id))
在上述代码中,read_specific_row_from_db
函数连接到数据库,并通过SQL查询选取指定行的数据。
总结
通过以上几个例子,我们可以看到,Python提供了丰富的库和方法来选取某行内容,无论是从文本文件、CSV文件、Excel文件还是数据库中读取数据,都可以轻松实现。关键在于选择合适的工具和方法,根据具体需求进行数据处理。在实际应用中,推荐使用Pandas库进行数据处理,因为它提供了强大的数据操作功能,且语法简洁易用。
接下来,让我们更详细地探讨以上方法,并扩展更多实用技巧和注意事项。
一、读取文本文件:深入解析
在读取文本文件时,除了使用readlines
方法外,我们还可以使用其他方法来提高效率,尤其是处理大文件时。
1. 使用逐行读取
逐行读取文件可以有效减少内存占用,特别适用于大文件。以下是一个示例:
def read_specific_line_efficient(file_path, line_number):
with open(file_path, 'r') as file:
for current_line_number, line in enumerate(file, start=1):
if current_line_number == line_number:
return line.strip()
raise ValueError("Line number exceeds the number of lines in the file.")
file_path = 'example.txt'
line_number = 3
print(read_specific_line_efficient(file_path, line_number))
在上述代码中,read_specific_line_efficient
函数逐行读取文件,直到找到指定行。这种方法在处理大文件时更加高效。
2. 使用seek
方法定位文件位置
如果知道行的位置(字节数),可以使用seek
方法快速定位文件位置:
def read_line_with_seek(file_path, byte_position):
with open(file_path, 'r') as file:
file.seek(byte_position)
return file.readline().strip()
file_path = 'example.txt'
byte_position = 50 # 假设知道第3行从第50个字节开始
print(read_line_with_seek(file_path, byte_position))
这种方法需要预先知道行的位置,但在某些情况下可以极大提高读取速度。
二、读取CSV文件:深入解析
在读取CSV文件时,Pandas库提供了多种方法来处理数据,包括过滤、筛选、排序等。以下是一些常见的操作:
1. 使用条件筛选行
除了通过索引选取行,还可以使用条件筛选行。例如,筛选某列值大于特定值的行:
import pandas as pd
def filter_rows_from_csv(file_path, column_name, threshold):
df = pd.read_csv(file_path)
filtered_df = df[df[column_name] > threshold]
return filtered_df
file_path = 'example.csv'
column_name = 'age'
threshold = 30
print(filter_rows_from_csv(file_path, column_name, threshold))
在上述代码中,filter_rows_from_csv
函数通过条件筛选出符合条件的行。
2. 处理大文件
在处理大CSV文件时,可以使用chunksize
参数分批读取数据,避免内存占用过高:
import pandas as pd
def read_large_csv_in_chunks(file_path, chunksize):
for chunk in pd.read_csv(file_path, chunksize=chunksize):
print(chunk.head()) # 处理每个块的数据
file_path = 'large_example.csv'
chunksize = 1000
read_large_csv_in_chunks(file_path, chunksize)
在上述代码中,read_large_csv_in_chunks
函数分批读取大文件,每次读取1000行数据。
三、读取Excel文件:深入解析
在读取Excel文件时,Pandas库还提供了许多高级功能,例如读取特定工作表、多表合并等。
1. 读取多个工作表
可以同时读取多个工作表,并进行合并处理:
import pandas as pd
def read_multiple_sheets(file_path, sheet_names):
dfs = pd.read_excel(file_path, sheet_name=sheet_names)
combined_df = pd.concat(dfs.values(), ignore_index=True)
return combined_df
file_path = 'example.xlsx'
sheet_names = ['Sheet1', 'Sheet2']
print(read_multiple_sheets(file_path, sheet_names))
在上述代码中,read_multiple_sheets
函数读取多个工作表,并将其合并为一个DataFrame。
2. 处理大文件
类似CSV文件,Excel文件也可以分批读取:
import pandas as pd
def read_large_excel_in_chunks(file_path, sheet_name, chunksize):
for chunk in pd.read_excel(file_path, sheet_name=sheet_name, chunksize=chunksize):
print(chunk.head()) # 处理每个块的数据
file_path = 'large_example.xlsx'
sheet_name = 'Sheet1'
chunksize = 1000
read_large_excel_in_chunks(file_path, sheet_name, chunksize)
在上述代码中,read_large_excel_in_chunks
函数分批读取大Excel文件,每次读取1000行数据。
四、读取数据库中的数据:深入解析
在读取数据库中的数据时,除了sqlite3,还可以使用更高级的库如SQLAlchemy,它支持多种数据库类型,并提供ORM功能。
1. 使用SQLAlchemy读取数据
SQLAlchemy可以更方便地操作数据库,并支持复杂查询:
from sqlalchemy import create_engine
import pandas as pd
def read_data_from_db_with_sqlalchemy(db_url, table_name):
engine = create_engine(db_url)
with engine.connect() as connection:
df = pd.read_sql_table(table_name, connection)
return df
db_url = 'sqlite:///example.db'
table_name = 'example_table'
print(read_data_from_db_with_sqlalchemy(db_url, table_name))
在上述代码中,read_data_from_db_with_sqlalchemy
函数使用SQLAlchemy连接数据库,并读取指定表的数据。
2. 使用ORM操作数据库
SQLAlchemy还支持ORM操作,使得数据库操作更加面向对象:
from sqlalchemy.orm import sessionmaker
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy import Column, Integer, String, create_engine
Base = declarative_base()
class ExampleTable(Base):
__tablename__ = 'example_table'
id = Column(Integer, primary_key=True)
name = Column(String)
age = Column(Integer)
def read_data_with_orm(db_url):
engine = create_engine(db_url)
Session = sessionmaker(bind=engine)
session = Session()
data = session.query(ExampleTable).all()
session.close()
return data
db_url = 'sqlite:///example.db'
print(read_data_with_orm(db_url))
在上述代码中,read_data_with_orm
函数使用SQLAlchemy的ORM功能读取数据库数据。
总结
通过上述方法,我们可以灵活地读取和处理各种格式的数据文件和数据库。选择合适的工具和方法,是高效处理数据的关键。在实际应用中,推荐使用Pandas库进行数据处理,因为它提供了丰富的功能和简洁的语法,极大地提高了数据处理的效率和便利性。
无论是读取文本文件、CSV文件、Excel文件还是数据库数据,都需要根据具体需求选择最优的方法,并注意处理大文件时的性能问题。希望通过本文的详细介绍,能够帮助你在实际工作中更高效地处理数据。
相关问答FAQs:
如何在Python中读取特定行的内容?
在Python中,您可以使用文件操作来读取特定行的内容。可以通过循环遍历每一行,或使用enumerate()
函数来实现。以下是一个简单的示例:
with open('yourfile.txt', 'r') as file:
for index, line in enumerate(file):
if index == desired_line_number: # 替换为您想要的行号
print(line)
这种方法可以灵活地读取您指定的行。
在Pandas中如何选择特定行的数据?
如果您正在使用Pandas库处理数据,可以通过.iloc[]
或.loc[]
方法来选取特定行。.iloc[]
用于按位置选择行,而.loc[]
则根据标签选择。例如:
import pandas as pd
df = pd.read_csv('yourdata.csv')
specific_row = df.iloc[desired_row_index] # 替换为您想要的行索引
print(specific_row)
这种方式使得数据处理更为便捷和高效。
如何处理大型文本文件以选取某一行?
处理大型文本文件时,直接读取整个文件可能会占用大量内存。可以考虑使用file.readline()
方法逐行读取,直到达到您想要的行:
with open('largefile.txt', 'r') as file:
for current_line_number in range(desired_line_number + 1):
line = file.readline()
print(line)
这种方法可以有效地节省内存,同时快速定位所需行。
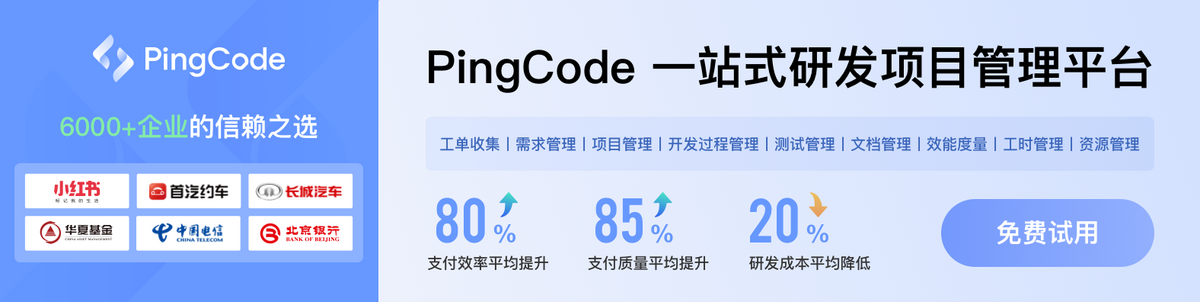