在Python中,可以通过多种方法从题库中匹配题目。使用正则表达式、利用字符串方法、结合数据库查询等都是常见且有效的方法。本文将详细介绍这些方法,并提供相关代码示例和专业见解。
一、使用正则表达式
正则表达式是一种强大的字符串匹配工具,能够帮助我们在题库中匹配特定的题目。
1.1 基础知识
正则表达式可以通过 re
模块在Python中实现。常见的正则表达式符号有:
.
: 匹配任意字符*
: 匹配前一个字符0次或多次+
: 匹配前一个字符1次或多次?
: 匹配前一个字符0次或1次[]
: 匹配括号内的任意字符^
: 匹配字符串的开始$
: 匹配字符串的结束
1.2 示例代码
以下是一个使用正则表达式匹配题库中题目的示例代码:
import re
题库列表
question_bank = [
"What is the capital of France?",
"How many continents are there in the world?",
"What is the largest ocean on Earth?",
"Who wrote 'To Kill a Mockingbird'?",
"What is the square root of 64?"
]
匹配函数
def match_questions(pattern, questions):
matched_questions = []
for question in questions:
if re.search(pattern, question):
matched_questions.append(question)
return matched_questions
示例匹配
pattern = r'\bWhat\b'
matched = match_questions(pattern, question_bank)
print(matched)
在这个示例中,我们定义了一个函数 match_questions
,它接受一个正则表达式模式和一个问题列表,并返回所有与模式匹配的问题。我们使用 re.search
函数来检查每个问题是否与模式匹配。
二、利用字符串方法
Python 提供了丰富的字符串方法,可以帮助我们从题库中匹配题目。这些方法包括 find
, startswith
, endswith
, in
等。
2.1 基础知识
find(sub)
: 返回子字符串在字符串中的最低索引,如果子字符串不存在则返回 -1startswith(prefix)
: 检查字符串是否以指定前缀开头endswith(suffix)
: 检查字符串是否以指定后缀结尾in
: 检查子字符串是否存在于字符串中
2.2 示例代码
以下是一个利用字符串方法匹配题库中题目的示例代码:
# 题库列表
question_bank = [
"What is the capital of France?",
"How many continents are there in the world?",
"What is the largest ocean on Earth?",
"Who wrote 'To Kill a Mockingbird'?",
"What is the square root of 64?"
]
匹配函数
def match_questions(substring, questions):
matched_questions = []
for question in questions:
if substring in question:
matched_questions.append(question)
return matched_questions
示例匹配
substring = "What"
matched = match_questions(substring, question_bank)
print(matched)
在这个示例中,我们定义了一个函数 match_questions
,它接受一个子字符串和一个问题列表,并返回所有包含该子字符串的问题。
三、结合数据库查询
在实际应用中,题库可能存储在数据库中。通过数据库查询,我们可以高效地匹配题目。
3.1 使用SQLite
SQLite 是一个轻量级的数据库,可以很方便地集成到Python项目中。
3.2 示例代码
以下是一个使用SQLite数据库匹配题库中题目的示例代码:
import sqlite3
创建数据库连接
conn = sqlite3.connect(':memory:')
cursor = conn.cursor()
创建题库表
cursor.execute('''
CREATE TABLE question_bank (
id INTEGER PRIMARY KEY,
question TEXT
)
''')
插入题目数据
questions = [
"What is the capital of France?",
"How many continents are there in the world?",
"What is the largest ocean on Earth?",
"Who wrote 'To Kill a Mockingbird'?",
"What is the square root of 64?"
]
cursor.executemany('INSERT INTO question_bank (question) VALUES (?)', [(q,) for q in questions])
匹配函数
def match_questions(pattern, cursor):
cursor.execute('SELECT question FROM question_bank WHERE question LIKE ?', ('%' + pattern + '%',))
return cursor.fetchall()
示例匹配
pattern = "What"
matched = match_questions(pattern, cursor)
print(matched)
关闭数据库连接
conn.close()
在这个示例中,我们创建了一个SQLite数据库并插入了一些问题数据。然后,通过SQL查询语句,我们可以匹配包含特定模式的问题。
四、结合自然语言处理(NLP)
自然语言处理技术可以帮助我们更智能地匹配题目,尤其是当问题的表达方式多样化时。
4.1 使用NLTK
NLTK 是一个广泛使用的自然语言处理库,提供了丰富的工具和资源。
4.2 示例代码
以下是一个使用NLTK匹配题库中题目的示例代码:
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
下载必要的NLTK数据
nltk.download('punkt')
nltk.download('stopwords')
题库列表
question_bank = [
"What is the capital of France?",
"How many continents are there in the world?",
"What is the largest ocean on Earth?",
"Who wrote 'To Kill a Mockingbird'?",
"What is the square root of 64?"
]
预处理函数
def preprocess(text):
tokens = word_tokenize(text)
tokens = [word.lower() for word in tokens if word.isalpha()]
stop_words = set(stopwords.words('english'))
tokens = [word for word in tokens if word not in stop_words]
return tokens
匹配函数
def match_questions(pattern, questions):
pattern_tokens = preprocess(pattern)
matched_questions = []
for question in questions:
question_tokens = preprocess(question)
if all(token in question_tokens for token in pattern_tokens):
matched_questions.append(question)
return matched_questions
示例匹配
pattern = "capital of France"
matched = match_questions(pattern, question_bank)
print(matched)
在这个示例中,我们使用NLTK对问题进行预处理,包括分词、转小写和去除停用词。然后,通过检查每个问题是否包含模式中的所有词,我们可以匹配相关问题。
五、使用机器学习
机器学习算法可以帮助我们从题库中匹配题目,尤其是当问题的表达方式复杂多样时。
5.1 使用TF-IDF和KNN
TF-IDF(词频-逆文档频率)是一种常用的文本表示方法,可以衡量词语的重要性。KNN(K最近邻算法)是一种常用的分类算法。
5.2 示例代码
以下是一个使用TF-IDF和KNN匹配题库中题目的示例代码:
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.neighbors import NearestNeighbors
题库列表
question_bank = [
"What is the capital of France?",
"How many continents are there in the world?",
"What is the largest ocean on Earth?",
"Who wrote 'To Kill a Mockingbird'?",
"What is the square root of 64?"
]
匹配函数
def match_questions(pattern, questions):
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(questions)
pattern_vec = vectorizer.transform([pattern])
knn = NearestNeighbors(n_neighbors=3, metric='cosine')
knn.fit(X)
distances, indices = knn.kneighbors(pattern_vec)
matched_questions = [questions[i] for i in indices.flatten()]
return matched_questions
示例匹配
pattern = "capital of France"
matched = match_questions(pattern, question_bank)
print(matched)
在这个示例中,我们使用TF-IDF对问题进行向量化表示,然后使用KNN算法找到与模式最相似的几个问题。
六、使用深度学习
深度学习模型,尤其是基于Transformer的模型,如BERT,可以帮助我们从题库中匹配复杂的题目。
6.1 使用BERT
BERT(Bidirectional Encoder Representations from Transformers)是一个预训练的语言模型,可以用于各种自然语言处理任务。
6.2 示例代码
以下是一个使用BERT匹配题库中题目的示例代码:
from transformers import BertTokenizer, BertModel
import torch
import numpy as np
初始化BERT模型和分词器
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
model = BertModel.from_pretrained('bert-base-uncased')
题库列表
question_bank = [
"What is the capital of France?",
"How many continents are there in the world?",
"What is the largest ocean on Earth?",
"Who wrote 'To Kill a Mockingbird'?",
"What is the square root of 64?"
]
获取句子嵌入
def get_sentence_embedding(sentence):
inputs = tokenizer(sentence, return_tensors='pt')
outputs = model(inputs)
return outputs.last_hidden_state.mean(dim=1).detach().numpy()
匹配函数
def match_questions(pattern, questions):
pattern_embedding = get_sentence_embedding(pattern)
question_embeddings = [get_sentence_embedding(question) for question in questions]
similarities = [np.dot(pattern_embedding, q_emb.T) / (np.linalg.norm(pattern_embedding) * np.linalg.norm(q_emb)) for q_emb in question_embeddings]
matched_indices = np.argsort(similarities)[::-1][:3]
matched_questions = [questions[i] for i in matched_indices]
return matched_questions
示例匹配
pattern = "capital of France"
matched = match_questions(pattern, question_bank)
print(matched)
在这个示例中,我们使用BERT对问题进行嵌入表示,然后通过计算余弦相似度来匹配最相似的问题。
七、总结
在Python中从题库中匹配题目可以通过多种方法实现,包括使用正则表达式、利用字符串方法、结合数据库查询、结合自然语言处理、使用机器学习和使用深度学习。每种方法都有其优缺点,选择合适的方法取决于具体的应用场景和需求。
- 正则表达式适合匹配简单的模式,但对于复杂的语言结构可能会力不从心。
- 字符串方法简单易用,但匹配能力有限。
- 数据库查询适合处理大规模数据,但需要数据库支持。
- 自然语言处理可以处理复杂的语言结构,但需要一定的预处理步骤。
- 机器学习能够处理多样化的表达方式,但需要训练和模型选择。
- 深度学习在处理复杂语言任务方面表现出色,但计算资源需求较高。
根据实际需求,选择合适的方法可以高效地从题库中匹配到所需的题目。
相关问答FAQs:
如何使用Python从题库中提取特定类型的问题?
要从题库中提取特定类型的问题,可以使用Python的字符串匹配功能和正则表达式。通过使用列表解析和条件语句,您可以过滤出符合特定条件的问题,例如根据关键词、难度等级或主题分类。此外,使用Pandas库可以方便地处理CSV格式的题库数据,轻松实现筛选和提取。
在Python中如何实现题库的自动更新?
实现题库的自动更新可以通过编写脚本来定期从指定的源(如数据库或在线API)获取最新的问题数据。使用Python的定时任务库,例如APScheduler,可以设定任务的运行时间,确保题库中的内容保持最新。结合数据存储(如SQLite或MongoDB),可以将新获取的问题自动添加到题库中。
如何在Python中优化题库的查询效率?
优化题库的查询效率可以通过选择合适的数据结构和算法来实现。例如,使用字典或集合来存储题目,以实现O(1)的查找时间。此外,可以考虑使用数据库的索引功能,或者对常用查询进行缓存。对于大规模题库,使用SQLite或SQLAlchemy等库,可以有效管理数据,提高查询速度。
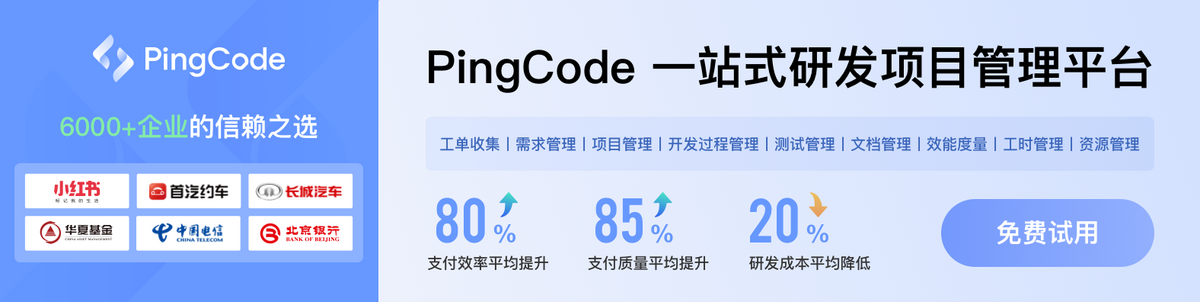