Python实现多线程并行的方式有多种,如使用threading
模块、concurrent.futures
模块、以及multiprocessing
模块。 其中,threading
模块是最常用的方式之一,适合I/O密集型任务。concurrent.futures
模块提供了更高级的接口,简化了多线程和多进程的使用。multiprocessing
模块则适用于CPU密集型任务,因为它能够绕过GIL(全局解释器锁)的限制。下面我们将详细展开threading
模块的使用方式。
threading
模块实现多线程并行
threading
模块是Python标准库的一部分,用于创建和管理线程。它允许在程序中创建多个线程并发执行,从而提高程序的执行效率。下面是使用threading
模块实现多线程并行的详细步骤:
一、基础知识
1、线程与进程的区别
线程是进程中的一个执行单元,一个进程可以包含多个线程。线程之间共享进程的资源,如内存空间和文件句柄。线程的创建和销毁比进程更轻量级,因此适用于需要并发执行的任务。
2、全局解释器锁(GIL)
Python中的GIL限制了同一时刻只有一个线程执行Python字节码,尽管这使得多线程在CPU密集型任务中的性能提升有限,但在I/O密集型任务中,多线程仍然能够显著提高程序的执行效率。
二、threading
模块的使用
1、创建线程
可以通过继承threading.Thread
类或者直接创建Thread
对象来创建线程。
import threading
方式一:继承Thread类
class MyThread(threading.Thread):
def __init__(self, name):
super().__init__()
self.name = name
def run(self):
print(f'Thread {self.name} is running')
方式二:直接创建Thread对象
def thread_function(name):
print(f'Thread {name} is running')
创建线程
thread1 = MyThread(name='Thread-1')
thread2 = threading.Thread(target=thread_function, args=('Thread-2',))
启动线程
thread1.start()
thread2.start()
等待线程完成
thread1.join()
thread2.join()
print('All threads are done')
2、线程同步
多线程共享同一进程的资源,可能会导致资源竞争问题。可以使用Lock
、RLock
、Semaphore
、Event
等同步原语来解决这些问题。
import threading
import time
使用Lock进行线程同步
lock = threading.Lock()
counter = 0
def increment_counter():
global counter
for _ in range(100000):
lock.acquire()
counter += 1
lock.release()
创建多个线程
threads = []
for _ in range(10):
thread = threading.Thread(target=increment_counter)
threads.append(thread)
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
print(f'Final counter value: {counter}')
3、线程池
使用concurrent.futures.ThreadPoolExecutor
可以简化线程的管理,特别是当需要创建大量线程时。
from concurrent.futures import ThreadPoolExecutor
def thread_function(name):
print(f'Thread {name} is running')
创建线程池
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(thread_function, f'Thread-{i}') for i in range(10)]
等待所有线程完成
for future in futures:
future.result()
print('All threads are done')
三、应用场景
1、I/O密集型任务
多线程适合I/O密集型任务,如网络请求、文件读写、数据库操作等。在这些场景中,线程可以在等待I/O操作完成时切换到其他任务,从而提高程序的并发性和响应速度。
import threading
import requests
def fetch_url(url):
response = requests.get(url)
print(f'{url}: {response.status_code}')
urls = ['https://www.example.com', 'https://www.python.org', 'https://www.github.com']
创建并启动线程
threads = [threading.Thread(target=fetch_url, args=(url,)) for url in urls]
for thread in threads:
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
print('All URLs fetched')
2、后台任务
可以使用线程来执行后台任务,而不会阻塞主线程的执行。例如,在GUI应用中,可以使用线程来处理耗时的操作,以保持界面的响应性。
import threading
import time
import tkinter as tk
def long_running_task():
time.sleep(5)
print('Task completed')
def start_task():
thread = threading.Thread(target=long_running_task)
thread.start()
创建GUI
root = tk.Tk()
button = tk.Button(root, text='Start Task', command=start_task)
button.pack()
root.mainloop()
四、threading
模块的高级用法
1、线程间通信
可以使用queue.Queue
类在线程之间传递数据,避免直接使用共享变量带来的复杂性。
import threading
import queue
import time
def producer(q):
for i in range(5):
time.sleep(1)
item = f'item-{i}'
q.put(item)
print(f'Produced {item}')
def consumer(q):
while True:
item = q.get()
if item is None:
break
print(f'Consumed {item}')
q.task_done()
q = queue.Queue()
创建生产者和消费者线程
producer_thread = threading.Thread(target=producer, args=(q,))
consumer_thread = threading.Thread(target=consumer, args=(q,))
producer_thread.start()
consumer_thread.start()
等待生产者线程完成
producer_thread.join()
发送停止信号
q.put(None)
等待消费者线程完成
consumer_thread.join()
print('All tasks are done')
2、定时器
threading.Timer
类用于在指定的时间间隔后执行特定的函数,可以用于实现定时任务。
import threading
def delayed_task():
print('Task executed')
创建并启动定时器
timer = threading.Timer(5, delayed_task)
timer.start()
print('Timer started')
五、注意事项
1、GIL的影响
由于GIL的存在,多线程在CPU密集型任务中的性能提升有限。在这种情况下,可以考虑使用multiprocessing
模块来实现并行计算。
2、避免死锁
在使用锁进行线程同步时,需小心避免死锁的发生。可以通过尽量减少锁的使用,或者使用RLock
(可重入锁)来降低死锁的风险。
import threading
lock1 = threading.Lock()
lock2 = threading.Lock()
def task1():
lock1.acquire()
time.sleep(1)
lock2.acquire()
print('Task 1 completed')
lock2.release()
lock1.release()
def task2():
lock2.acquire()
time.sleep(1)
lock1.acquire()
print('Task 2 completed')
lock1.release()
lock2.release()
thread1 = threading.Thread(target=task1)
thread2 = threading.Thread(target=task2)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
print('All tasks are done')
3、线程安全的数据结构
在多线程环境中,可以使用线程安全的数据结构,如queue.Queue
、collections.deque
等,避免因共享数据引发的竞争条件。
import threading
import queue
def producer(q):
for i in range(5):
q.put(i)
print(f'Produced {i}')
def consumer(q):
while True:
item = q.get()
if item is None:
break
print(f'Consumed {item}')
q.task_done()
q = queue.Queue()
producer_thread = threading.Thread(target=producer, args=(q,))
consumer_thread = threading.Thread(target=consumer, args=(q,))
producer_thread.start()
consumer_thread.start()
producer_thread.join()
q.put(None)
consumer_thread.join()
print('All tasks are done')
六、总结
使用threading
模块可以有效地实现多线程并行,适用于I/O密集型任务和需要后台执行的任务。通过合理的线程同步和线程间通信,可以避免资源竞争和死锁问题。此外,高级用法如线程池和定时器,可以进一步简化多线程编程。需要注意的是,由于GIL的存在,多线程在CPU密集型任务中的性能提升有限,此时可以考虑使用multiprocessing
模块。通过掌握这些技术,可以在实际项目中灵活应用多线程并行,提高程序的执行效率和响应速度。
相关问答FAQs:
多线程和多进程的区别是什么?
多线程和多进程都是实现并行处理的技术。多线程是在同一个进程内创建多个线程,这些线程共享进程的资源,如内存和文件句柄,适用于IO密集型任务。多进程则是创建多个独立的进程,每个进程拥有自己的内存空间,适合CPU密集型任务。选择哪种方式取决于具体的应用场景和需求。
在Python中如何创建和管理线程?
在Python中,可以使用threading
模块来创建和管理线程。通过threading.Thread
类,可以定义线程的目标函数,并通过start()
方法启动线程。使用join()
方法可以等待线程完成。为了确保线程安全,可以使用Lock
类来控制对共享资源的访问。
多线程在Python中存在哪些限制?
Python的多线程受到全局解释器锁(GIL)的限制,这意味着同一时刻只有一个线程在执行Python字节码。这会导致在CPU密集型任务中,多线程的性能提升不明显。然而,对于IO密集型任务,如网络请求和文件操作,多线程仍然能够显著提高程序的效率。选择合适的任务类型对于有效利用多线程至关重要。
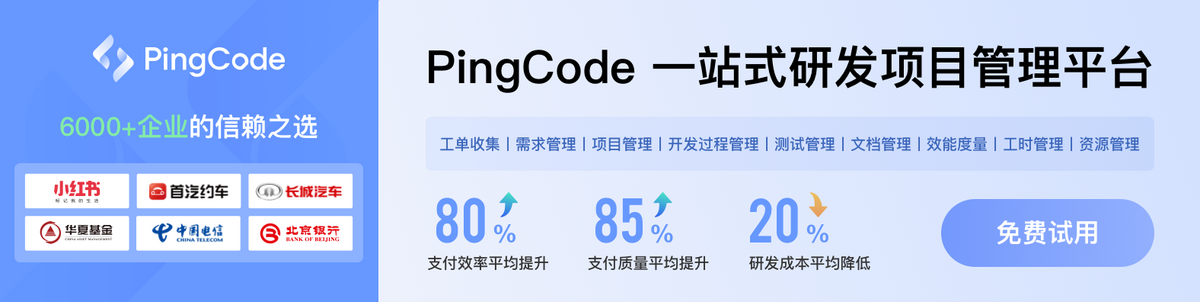