在Python中计算奖金发放通常涉及到多个因素,如员工的基本工资、绩效评分、工作年限等。常见的计算奖金发放的方法包括:根据基本工资的百分比、基于绩效评分的额外奖金、按照公司政策的固定奖金。以下将详细描述基于绩效评分计算奖金的方法。
基于绩效评分的奖金计算是一种常见且公平的方式。通常公司会根据员工的绩效评分来决定额外奖金的数额。绩效评分可以根据员工的工作表现、目标完成情况、团队合作等多个维度综合评定。以下是一个简单的Python示例,展示了如何基于绩效评分计算奖金:
def calculate_bonus(base_salary, performance_score):
# 定义绩效评分对应的奖金百分比
bonus_percentage = 0
if performance_score >= 90:
bonus_percentage = 0.20
elif performance_score >= 80:
bonus_percentage = 0.15
elif performance_score >= 70:
bonus_percentage = 0.10
elif performance_score >= 60:
bonus_percentage = 0.05
else:
bonus_percentage = 0.00
# 计算奖金
bonus = base_salary * bonus_percentage
return bonus
示例
base_salary = 50000
performance_score = 85
bonus = calculate_bonus(base_salary, performance_score)
print(f"Calculated bonus: ${bonus}")
在这个示例中,我们定义了一个函数calculate_bonus
,它接受两个参数:基本工资和绩效评分。根据绩效评分,我们确定奖金的百分比并计算出最终的奖金数额。
接下来,我们将更详细地探讨其他几种计算奖金的方法及其具体实现。
一、根据基本工资的百分比
根据基本工资的百分比计算奖金是一种最简单和常见的方法。公司通常会根据员工的基本工资设定一个奖金百分比,然后根据这一百分比计算出具体的奖金数额。
def calculate_bonus_by_percentage(base_salary, percentage):
bonus = base_salary * (percentage / 100)
return bonus
示例
base_salary = 50000
percentage = 10 # 奖金百分比
bonus = calculate_bonus_by_percentage(base_salary, percentage)
print(f"Calculated bonus: ${bonus}")
在这个例子中,calculate_bonus_by_percentage
函数接受基本工资和奖金百分比两个参数,然后返回计算出的奖金数额。这种方法的优点是简单易行,但可能无法充分反映员工的实际绩效和贡献。
二、基于公司政策的固定奖金
有些公司会根据员工的职位、工作年限或公司政策发放固定数额的奖金。这种方法通常用于节假日或特定的项目奖励。
def calculate_fixed_bonus(position, years_of_service):
# 定义不同职位和工龄对应的固定奖金
bonus_table = {
'Junior': 1000,
'Mid': 2000,
'Senior': 3000,
'Manager': 5000
}
# 根据工龄增加额外的奖金
extra_bonus = years_of_service * 100
bonus = bonus_table.get(position, 0) + extra_bonus
return bonus
示例
position = 'Senior'
years_of_service = 5
bonus = calculate_fixed_bonus(position, years_of_service)
print(f"Calculated bonus: ${bonus}")
在这个示例中,calculate_fixed_bonus
函数根据员工的职位和工作年限计算固定奖金。不同职位有不同的基本奖金,同时根据工龄增加额外的奖金。这种方法简单且容易管理,但可能会忽略员工的实际绩效和贡献。
三、综合多因素计算奖金
在实际应用中,奖金计算往往是多个因素的综合结果。我们可以结合基本工资、绩效评分和公司政策来计算奖金。
def calculate_bonus_comprehensive(base_salary, performance_score, position, years_of_service):
# 基于绩效评分的奖金百分比
if performance_score >= 90:
performance_bonus_percentage = 0.20
elif performance_score >= 80:
performance_bonus_percentage = 0.15
elif performance_score >= 70:
performance_bonus_percentage = 0.10
elif performance_score >= 60:
performance_bonus_percentage = 0.05
else:
performance_bonus_percentage = 0.00
# 基于公司政策的固定奖金
bonus_table = {
'Junior': 1000,
'Mid': 2000,
'Senior': 3000,
'Manager': 5000
}
extra_bonus = years_of_service * 100
fixed_bonus = bonus_table.get(position, 0) + extra_bonus
# 计算综合奖金
performance_bonus = base_salary * performance_bonus_percentage
total_bonus = performance_bonus + fixed_bonus
return total_bonus
示例
base_salary = 50000
performance_score = 85
position = 'Senior'
years_of_service = 5
bonus = calculate_bonus_comprehensive(base_salary, performance_score, position, years_of_service)
print(f"Calculated total bonus: ${bonus}")
这个示例展示了如何将多个因素综合起来计算奖金。calculate_bonus_comprehensive
函数结合了基于绩效评分的奖金百分比和公司政策的固定奖金,通过多个维度来评估和计算最终的奖金数额。
四、基于团队和项目的奖金分配
在一些公司,特别是科技公司和项目导向的组织中,奖金的分配不仅考虑个人绩效,还会考虑团队和项目的表现。这样可以激励团队合作,共同完成目标。
def calculate_team_bonus(team_performance_score, project_success, base_salary):
# 定义团队绩效评分对应的奖金百分比
if team_performance_score >= 90:
team_bonus_percentage = 0.10
elif team_performance_score >= 80:
team_bonus_percentage = 0.07
elif team_performance_score >= 70:
team_bonus_percentage = 0.05
elif team_performance_score >= 60:
team_bonus_percentage = 0.03
else:
team_bonus_percentage = 0.00
# 定义项目成功的额外奖金
project_bonus = 0
if project_success:
project_bonus = 5000
# 计算团队奖金
team_bonus = base_salary * team_bonus_percentage
total_bonus = team_bonus + project_bonus
return total_bonus
示例
team_performance_score = 85
project_success = True
base_salary = 50000
bonus = calculate_team_bonus(team_performance_score, project_success, base_salary)
print(f"Calculated team and project bonus: ${bonus}")
在这个示例中,calculate_team_bonus
函数不仅考虑了团队绩效评分,还加入了项目成功与否的因素,从而更加全面地评估和计算奖金。这种方法能更好地激励团队成员共同努力,完成团队和项目目标。
五、基于个人和团队绩效的综合评估
对于一些公司而言,单纯的个人绩效或团队绩效评估可能都不够全面。因此,综合个人和团队绩效来计算奖金也是一种常见的做法。
def calculate_combined_bonus(individual_performance_score, team_performance_score, base_salary):
# 定义个人绩效评分对应的奖金百分比
if individual_performance_score >= 90:
individual_bonus_percentage = 0.15
elif individual_performance_score >= 80:
individual_bonus_percentage = 0.10
elif individual_performance_score >= 70:
individual_bonus_percentage = 0.05
elif individual_performance_score >= 60:
individual_bonus_percentage = 0.03
else:
individual_bonus_percentage = 0.00
# 定义团队绩效评分对应的奖金百分比
if team_performance_score >= 90:
team_bonus_percentage = 0.10
elif team_performance_score >= 80:
team_bonus_percentage = 0.07
elif team_performance_score >= 70:
team_bonus_percentage = 0.05
elif team_performance_score >= 60:
team_bonus_percentage = 0.03
else:
team_bonus_percentage = 0.00
# 计算综合奖金
individual_bonus = base_salary * individual_bonus_percentage
team_bonus = base_salary * team_bonus_percentage
total_bonus = individual_bonus + team_bonus
return total_bonus
示例
individual_performance_score = 85
team_performance_score = 80
base_salary = 50000
bonus = calculate_combined_bonus(individual_performance_score, team_performance_score, base_salary)
print(f"Calculated combined bonus: ${bonus}")
在这个示例中,calculate_combined_bonus
函数结合了个人绩效评分和团队绩效评分来计算最终奖金。通过这种方法,可以更加全面、公正地评估员工的贡献,同时鼓励个人和团队的共同进步。
六、基于KPI的奖金发放
关键绩效指标(KPI)是企业常用来评估员工绩效的重要工具。根据KPI来计算奖金,可以确保奖金发放与员工的实际贡献挂钩,从而激励员工朝着公司的目标努力。
def calculate_kpi_bonus(kpi_scores, base_salary):
# 定义KPI对应的奖金权重
kpi_weights = {
'sales': 0.40,
'customer_satisfaction': 0.30,
'productivity': 0.20,
'attendance': 0.10
}
# 计算KPI加权评分
weighted_score = 0
for kpi, score in kpi_scores.items():
weighted_score += score * kpi_weights.get(kpi, 0)
# 定义加权评分对应的奖金百分比
if weighted_score >= 90:
bonus_percentage = 0.25
elif weighted_score >= 80:
bonus_percentage = 0.20
elif weighted_score >= 70:
bonus_percentage = 0.15
elif weighted_score >= 60:
bonus_percentage = 0.10
else:
bonus_percentage = 0.00
# 计算奖金
bonus = base_salary * bonus_percentage
return bonus
示例
kpi_scores = {
'sales': 85,
'customer_satisfaction': 90,
'productivity': 80,
'attendance': 95
}
base_salary = 50000
bonus = calculate_kpi_bonus(kpi_scores, base_salary)
print(f"Calculated KPI bonus: ${bonus}")
在这个示例中,calculate_kpi_bonus
函数根据多个KPI的评分和权重计算加权评分,并根据加权评分确定奖金百分比。这种方法能够全面反映员工在不同方面的表现,从而更加公平地分配奖金。
七、基于年度评审的奖金发放
年度评审是企业评估员工年度表现的重要环节。根据年度评审结果发放奖金,可以激励员工在整个年度内保持高水平的工作表现。
def calculate_annual_review_bonus(annual_review_score, base_salary):
# 定义年度评审评分对应的奖金百分比
if annual_review_score >= 90:
bonus_percentage = 0.30
elif annual_review_score >= 80:
bonus_percentage = 0.25
elif annual_review_score >= 70:
bonus_percentage = 0.20
elif annual_review_score >= 60:
bonus_percentage = 0.15
else:
bonus_percentage = 0.00
# 计算奖金
bonus = base_salary * bonus_percentage
return bonus
示例
annual_review_score = 88
base_salary = 50000
bonus = calculate_annual_review_bonus(annual_review_score, base_salary)
print(f"Calculated annual review bonus: ${bonus}")
在这个示例中,calculate_annual_review_bonus
函数根据年度评审评分确定奖金百分比,并计算出最终的奖金数额。这种方法能够激励员工在整个年度内持续努力工作,从而达到公司设定的目标。
八、基于公司业绩的奖金发放
公司业绩也是影响员工奖金的重要因素之一。当公司整体业绩良好时,员工的奖金也会相应增加。这种方法可以激励员工为公司的整体成功而努力。
def calculate_company_performance_bonus(company_performance_score, base_salary):
# 定义公司业绩评分对应的奖金百分比
if company_performance_score >= 90:
bonus_percentage = 0.20
elif company_performance_score >= 80:
bonus_percentage = 0.15
elif company_performance_score >= 70:
bonus_percentage = 0.10
elif company_performance_score >= 60:
bonus_percentage = 0.05
else:
bonus_percentage = 0.00
# 计算奖金
bonus = base_salary * bonus_percentage
return bonus
示例
company_performance_score = 85
base_salary = 50000
bonus = calculate_company_performance_bonus(company_performance_score, base_salary)
print(f"Calculated company performance bonus: ${bonus}")
在这个示例中,calculate_company_performance_bonus
函数根据公司业绩评分确定奖金百分比,并计算出最终的奖金数额。这种方法能够激励员工关注公司的整体业绩,与公司共同成长。
九、基于个人目标达成情况的奖金发放
个人目标设定是员工管理的重要工具。根据个人目标的达成情况发放奖金,可以激励员工积极完成既定目标。
def calculate_goal_achievement_bonus(goal_achievement_score, base_salary):
# 定义目标达成评分对应的奖金百分比
if goal_achievement_score >= 90:
bonus_percentage = 0.25
elif goal_achievement_score >= 80:
bonus_percentage = 0.20
elif goal_achievement_score >= 70:
bonus_percentage = 0.15
elif goal_achievement_score >= 60:
bonus_percentage = 0.10
else:
bonus_percentage = 0.00
# 计算奖金
bonus = base_salary * bonus_percentage
return bonus
示例
goal_achievement_score = 92
base_salary = 50000
bonus = calculate_goal_achievement_bonus(goal_achievement_score, base_salary)
print(f"Calculated goal achievement bonus: ${bonus}")
在这个示例中,calculate_goal_achievement_bonus
函数根据个人目标达成情况的评分确定奖金百分比,并计算出最终的奖金数额。这种方法能够激励员工积极完成个人目标,从而提高整体绩效。
十、基于员工满意度的奖金发放
员工满意度是影响员工积极性和工作效率的重要因素。根据员工满意度发放奖金,可以提升员工的归属感和满意度。
def calculate_employee_satisfaction_bonus(satisfaction_score, base_salary):
# 定义满意度评分对应的奖金百分比
if satisfaction_score >= 90:
bonus_percentage = 0.15
elif satisfaction_score >= 80:
bonus_percentage = 0.10
elif satisfaction_score >= 70:
bonus_percentage = 0.05
elif satisfaction_score >= 60:
bonus_percentage = 0.03
else:
bonus_percentage = 0.00
# 计算奖金
bonus = base_salary * bonus_percentage
return bonus
示例
satisfaction_score = 87
base_salary = 50000
bonus = calculate_employee_satisfaction_bonus(satisfaction_score, base_salary)
print(f"Calculated employee satisfaction bonus: ${bonus}")
在这个示例中,calculate_employee_satisfaction_bonus
函数根据员工满意度评分确定奖金百分比,并计算出最终的奖金数额。这种方法能够提升员工的满意度和归属感,从而提高工作效率和绩效。
十一、基于客户反馈的奖金发放
客户反馈是衡量员工表现的重要指标。根据客户反馈发放奖金,可以激励员工提供更好的服务和产品。
def calculate_customer_feedback_bonus(feedback_score, base_salary):
# 定义客户反馈评分对应的奖金百分比
if feedback_score >= 90:
bonus_percentage = 0.20
elif feedback_score >= 80:
bonus_percentage = 0.15
elif feedback_score >= 70:
bonus_percentage = 0.10
elif feedback_score >= 60:
bonus_percentage = 0.05
else:
bonus_percentage = 0.00
# 计算奖金
bonus = base_salary * bonus_percentage
return bonus
示例
feedback_score = 91
base_salary = 50000
bonus = calculate_customer_feedback_bonus(feedback_score, base_salary)
print(f"Calculated customer feedback bonus: ${bonus}")
在这个示例中,calculate_customer_feedback_bonus
函数根据客户反馈评分确定奖金百分比,并计算出最终的奖金数额。这种方法能够激励员工提供更好的客户
相关问答FAQs:
如何在Python中计算员工奖金的基本公式是什么?
在Python中计算奖金通常涉及到员工的基本工资、业绩指标和奖金比例。一个常见的公式是:奖金 = 基本工资 × 业绩评分 × 奖金比例。可以通过定义函数来实现这一计算,例如:
def calculate_bonus(base_salary, performance_score, bonus_percentage):
return base_salary * performance_score * bonus_percentage
使用Python计算奖金时,如何处理不同的奖金比例?
不同员工可能会有不同的奖金比例,这通常取决于他们的职位、业绩等因素。在这种情况下,可以使用条件语句来设定不同的比例。例如,可以根据业绩评分来动态调整奖金比例:
def get_bonus_percentage(performance_score):
if performance_score >= 90:
return 0.2
elif performance_score >= 75:
return 0.15
else:
return 0.1
在Python中如何处理多级奖金计算?
多级奖金计算涉及到多个条件和复杂的计算。在这种情况下,可以使用循环和列表来处理多个员工的奖金。例如,假设有一个员工列表和各自的业绩评分,可以用以下方法计算所有员工的奖金:
employees = [{'name': 'Alice', 'salary': 5000, 'performance_score': 95},
{'name': 'Bob', 'salary': 4000, 'performance_score': 80}]
for employee in employees:
bonus_percentage = get_bonus_percentage(employee['performance_score'])
bonus = calculate_bonus(employee['salary'], employee['performance_score'] / 100, bonus_percentage)
print(f"{employee['name']} 的奖金是: {bonus}")
这种方式可以有效处理复杂的奖金计算需求。
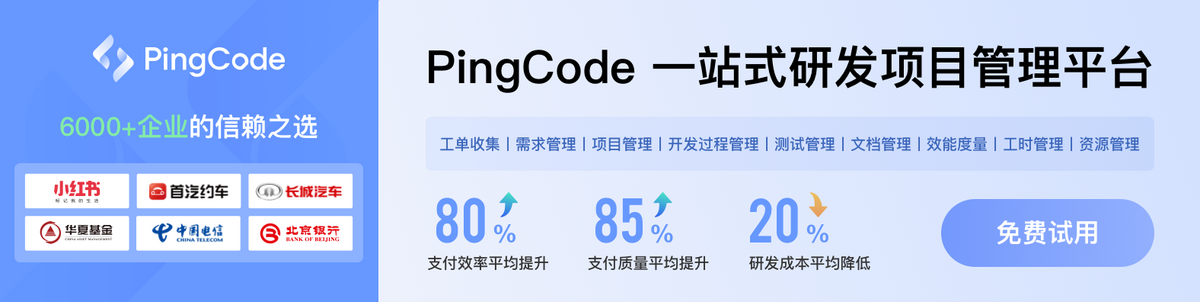