如何关闭Python中的Dialog,可以通过调用Dialog的destroy方法、使用Dialog的quit方法、绑定关闭按钮事件等方式来实现。其中一种常用的方法是调用Dialog的destroy
方法来销毁对话框。
一、调用Dialog的destroy方法
在Python中,使用Tkinter库创建和管理GUI应用程序是非常常见的。在Tkinter中,Dialog(对话框)是一种常见的GUI组件,通常用于提示用户信息或获取用户输入。要关闭一个Dialog,可以调用其destroy
方法,这将销毁对话框窗口,并释放与该窗口关联的所有资源。
import tkinter as tk
from tkinter import simpledialog
def show_dialog():
dialog = simpledialog.askstring("Input", "Enter your name:")
if dialog is not None:
print("Your name is:", dialog)
else:
print("Dialog was canceled")
root = tk.Tk()
root.withdraw() # Hide the main window
Show the dialog
show_dialog()
Close the main window when done
root.destroy()
在以上代码示例中,simpledialog.askstring
用于显示一个简单的输入对话框,用户可以输入文本并点击确定或取消按钮。无论用户选择什么,代码都会继续执行,并最终调用root.destroy()
来关闭主窗口。
二、使用Dialog的quit方法
除了destroy
方法,Tkinter中的quit
方法也可以用于关闭对话框。quit
方法会停止Tkinter的主事件循环,从而关闭所有Tkinter窗口。
import tkinter as tk
from tkinter import simpledialog
def show_dialog():
dialog = simpledialog.askstring("Input", "Enter your name:")
if dialog is not None:
print("Your name is:", dialog)
else:
print("Dialog was canceled")
root = tk.Tk()
root.withdraw() # Hide the main window
Show the dialog
show_dialog()
Quit the main loop when done
root.quit()
在以上代码中,调用root.quit()
会停止Tkinter的主事件循环,关闭所有Tkinter窗口。
三、绑定关闭按钮事件
在更复杂的GUI应用程序中,可能需要绑定关闭按钮事件,以便在用户点击关闭按钮时执行特定的操作。可以通过使用protocol
方法来绑定窗口关闭事件。
import tkinter as tk
from tkinter import simpledialog
def on_closing():
print("Dialog was closed")
root.destroy()
def show_dialog():
dialog = simpledialog.askstring("Input", "Enter your name:")
if dialog is not None:
print("Your name is:", dialog)
else:
print("Dialog was canceled")
root = tk.Tk()
root.withdraw() # Hide the main window
Bind the close event
root.protocol("WM_DELETE_WINDOW", on_closing)
Show the dialog
show_dialog()
Main loop
root.mainloop()
在以上代码中,root.protocol("WM_DELETE_WINDOW", on_closing)
用于绑定窗口关闭事件,当用户点击窗口的关闭按钮时,会调用on_closing
函数,并执行其中的代码。
四、使用Toplevel窗口管理Dialog
在Tkinter中,Toplevel
窗口是一个独立的窗口,可以用来创建自定义对话框。通过控制Toplevel
窗口的行为,可以实现对对话框的更细粒度的控制。
import tkinter as tk
def on_closing():
print("Dialog was closed")
dialog.destroy()
def show_dialog():
global dialog
dialog = tk.Toplevel(root)
dialog.title("Input Dialog")
label = tk.Label(dialog, text="Enter your name:")
label.pack(pady=10)
entry = tk.Entry(dialog)
entry.pack(pady=10)
button = tk.Button(dialog, text="OK", command=lambda: print("Your name is:", entry.get()))
button.pack(pady=10)
dialog.protocol("WM_DELETE_WINDOW", on_closing)
root = tk.Tk()
root.withdraw() # Hide the main window
Show the custom dialog
show_dialog()
Main loop
root.mainloop()
在以上代码中,Toplevel
窗口用于创建一个自定义对话框,并通过protocol
方法绑定窗口关闭事件。
五、使用第三方库管理Dialog
除了Tkinter,Python还有许多其他第三方库可以用来创建和管理GUI应用程序,如PyQt、wxPython等。这些库提供了更加丰富的功能和更好的跨平台支持。下面是一个使用PyQt5创建和关闭对话框的示例。
import sys
from PyQt5.QtWidgets import QApplication, QDialog, QVBoxLayout, QLabel, QLineEdit, QPushButton
class InputDialog(QDialog):
def __init__(self):
super().__init__()
self.setWindowTitle("Input Dialog")
layout = QVBoxLayout()
self.label = QLabel("Enter your name:")
layout.addWidget(self.label)
self.entry = QLineEdit()
layout.addWidget(self.entry)
self.button = QPushButton("OK")
self.button.clicked.connect(self.on_ok)
layout.addWidget(self.button)
self.setLayout(layout)
def on_ok(self):
print("Your name is:", self.entry.text())
self.accept()
def show_dialog():
dialog = InputDialog()
dialog.exec_()
app = QApplication(sys.argv)
Show the custom dialog
show_dialog()
Exit the application
sys.exit(app.exec_())
在以上代码中,使用PyQt5创建一个自定义对话框,并通过QDialog
的accept
方法关闭对话框。
六、处理多线程中的Dialog
在某些情况下,可能需要在多线程环境中处理对话框。使用多线程可以提高程序的响应速度,但需要小心处理线程之间的交互。在Tkinter中,可以使用threading
模块创建新线程,并通过queue
模块与主线程进行通信。
import tkinter as tk
import threading
import queue
def worker(q):
# Simulate a long-running task
import time
time.sleep(5)
# Send a message to the main thread
q.put("Task completed")
def on_closing():
print("Dialog was closed")
root.destroy()
def show_dialog():
global dialog
dialog = tk.Toplevel(root)
dialog.title("Input Dialog")
label = tk.Label(dialog, text="Enter your name:")
label.pack(pady=10)
entry = tk.Entry(dialog)
entry.pack(pady=10)
button = tk.Button(dialog, text="OK", command=lambda: print("Your name is:", entry.get()))
button.pack(pady=10)
dialog.protocol("WM_DELETE_WINDOW", on_closing)
# Create a new thread to run the worker function
q = queue.Queue()
t = threading.Thread(target=worker, args=(q,))
t.start()
# Check the queue for messages from the worker thread
def check_queue():
try:
msg = q.get_nowait()
print(msg)
dialog.destroy()
except queue.Empty:
root.after(100, check_queue)
root.after(100, check_queue)
root = tk.Tk()
root.withdraw() # Hide the main window
Show the custom dialog
show_dialog()
Main loop
root.mainloop()
在以上代码中,使用threading
模块创建了一个新线程来执行长时间运行的任务,并通过queue
模块与主线程进行通信。当任务完成时,向队列中放入一条消息,并在主线程中检查队列中的消息,从而关闭对话框。
七、使用Asyncio处理异步Dialog
在Python中,asyncio
是一个用于编写异步程序的标准库。通过使用asyncio
,可以更方便地处理异步任务和事件循环。下面是一个使用asyncio
处理异步对话框的示例。
import tkinter as tk
import asyncio
def on_closing():
print("Dialog was closed")
root.destroy()
def show_dialog():
global dialog
dialog = tk.Toplevel(root)
dialog.title("Input Dialog")
label = tk.Label(dialog, text="Enter your name:")
label.pack(pady=10)
entry = tk.Entry(dialog)
entry.pack(pady=10)
button = tk.Button(dialog, text="OK", command=lambda: print("Your name is:", entry.get()))
button.pack(pady=10)
dialog.protocol("WM_DELETE_WINDOW", on_closing)
# Start the asyncio event loop
loop = asyncio.get_event_loop()
loop.run_until_complete(asyncio_task())
async def asyncio_task():
await asyncio.sleep(5)
print("Task completed")
dialog.destroy()
root = tk.Tk()
root.withdraw() # Hide the main window
Show the custom dialog
show_dialog()
Main loop
root.mainloop()
在以上代码中,使用asyncio
模块创建了一个异步任务,并通过await
关键字等待任务完成。当任务完成时,关闭对话框。
八、使用自定义协议处理Dialog
在某些情况下,可能需要实现自定义的协议来处理对话框的关闭操作。通过继承Tkinter的Toplevel
类,可以创建自定义的对话框,并实现自定义的关闭协议。
import tkinter as tk
class CustomDialog(tk.Toplevel):
def __init__(self, master=None, kwargs):
super().__init__(master, kwargs)
self.title("Input Dialog")
label = tk.Label(self, text="Enter your name:")
label.pack(pady=10)
self.entry = tk.Entry(self)
self.entry.pack(pady=10)
button = tk.Button(self, text="OK", command=self.on_ok)
button.pack(pady=10)
self.protocol("WM_DELETE_WINDOW", self.on_closing)
def on_ok(self):
print("Your name is:", self.entry.get())
self.destroy()
def on_closing(self):
print("Dialog was closed")
self.destroy()
def show_dialog():
dialog = CustomDialog(root)
dialog.grab_set() # Make the dialog modal
root = tk.Tk()
root.withdraw() # Hide the main window
Show the custom dialog
show_dialog()
Main loop
root.mainloop()
在以上代码中,通过继承Toplevel
类创建了一个自定义的对话框,并实现了自定义的关闭协议。当用户点击关闭按钮时,会调用on_closing
方法,并执行其中的代码。
九、使用回调函数处理Dialog
在某些情况下,可能需要使用回调函数来处理对话框的关闭操作。通过将回调函数作为参数传递给对话框,可以在对话框关闭时执行特定的操作。
import tkinter as tk
def on_closing(callback):
print("Dialog was closed")
callback()
root.destroy()
def show_dialog(callback):
global dialog
dialog = tk.Toplevel(root)
dialog.title("Input Dialog")
label = tk.Label(dialog, text="Enter your name:")
label.pack(pady=10)
entry = tk.Entry(dialog)
entry.pack(pady=10)
button = tk.Button(dialog, text="OK", command=lambda: callback(entry.get()))
button.pack(pady=10)
dialog.protocol("WM_DELETE_WINDOW", lambda: on_closing(callback))
def on_ok(name):
print("Your name is:", name)
root = tk.Tk()
root.withdraw() # Hide the main window
Show the custom dialog
show_dialog(on_ok)
Main loop
root.mainloop()
在以上代码中,通过将回调函数on_ok
作为参数传递给show_dialog
函数,可以在对话框关闭时执行回调函数,并传递用户输入的名称。
十、总结
在Python中,关闭对话框的方法有很多,可以根据具体需求选择合适的方法。常用的方法包括调用Dialog的destroy
方法、使用Dialog的quit
方法、绑定关闭按钮事件、使用Toplevel
窗口管理Dialog、使用第三方库管理Dialog、处理多线程中的Dialog、使用asyncio
处理异步Dialog、使用自定义协议处理Dialog以及使用回调函数处理Dialog等。每种方法都有其适用的场景和优缺点,选择合适的方法可以提高程序的稳定性和用户体验。
相关问答FAQs:
如何在Python中创建自定义的对话框?
在Python中,可以使用Tkinter或PyQt等库来创建自定义的对话框。Tkinter是Python自带的GUI库,使用简单,适合基础应用。通过调用Toplevel()
函数,可以创建新的对话框,并在其中添加各种控件,如按钮、标签和输入框。PyQt则提供了更加丰富和灵活的对话框选项,适合复杂的应用程序开发。
关闭对话框时,如何处理用户输入的内容?
当用户关闭对话框时,可以通过设置回调函数来处理用户输入的内容。对于Tkinter,可以在对话框的关闭事件中添加逻辑,以获取并保存输入的值。在PyQt中,可以重写对话框的accept()
和reject()
方法来处理用户的选择并执行相应的操作。
在Python中关闭对话框时,有哪些常见的错误需要注意?
关闭对话框时,常见的错误包括未正确绑定关闭事件、未处理输入数据的情况下直接关闭对话框等。确保在关闭之前检查用户的输入是否有效,并在关闭对话框时处理任何未捕获的异常,可以避免这些问题。此外,使用上下文管理器或try-except结构可以帮助更好地管理对话框的关闭过程。
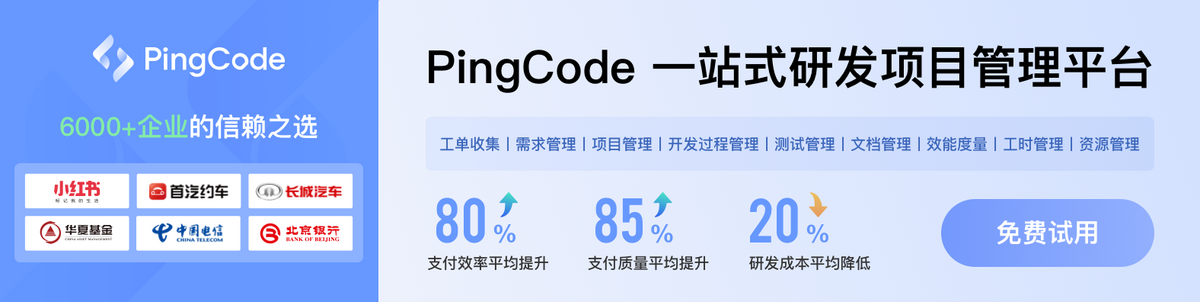