要用Python打开.nc文件,可以使用多个库,如NetCDF4、xarray、h5netcdf等。NetCDF4、xarray、h5netcdf是常用的三种库。接下来,我们详细介绍如何使用NetCDF4库来打开.nc文件。
一、使用 NetCDF4 库
NetCDF4 是一个强大的库,可以方便地读写NetCDF文件。
1、安装 NetCDF4 库
你需要首先安装 NetCDF4 库。可以使用以下命令:
pip install netCDF4
2、打开.nc文件
使用 NetCDF4 库打开.nc文件非常简单。以下是一个示例代码:
import netCDF4 as nc
打开.nc文件
file_path = 'your_file.nc'
dataset = nc.Dataset(file_path, 'r')
print(dataset)
二、使用 xarray 库
xarray 是一个用于处理多维数组的数据分析库,特别适合处理NetCDF文件。
1、安装 xarray 库
首先需要安装 xarray 库及其依赖项:
pip install xarray netCDF4
2、打开.nc文件
使用 xarray 库可以更方便地打开和操作.nc文件。以下是示例代码:
import xarray as xr
打开.nc文件
file_path = 'your_file.nc'
dataset = xr.open_dataset(file_path)
print(dataset)
三、使用 h5netcdf 库
h5netcdf 是一个轻量级库,用于处理NetCDF文件。
1、安装 h5netcdf 库
首先需要安装 h5netcdf 库及其依赖项:
pip install h5netcdf
2、打开.nc文件
使用 h5netcdf 库打开.nc文件的示例代码如下:
import h5netcdf
打开.nc文件
file_path = 'your_file.nc'
with h5netcdf.File(file_path, 'r') as f:
print(f)
四、读取和操作数据
在打开.nc文件后,你可以读取数据集中的变量和属性。
1、使用 NetCDF4 库读取数据
import netCDF4 as nc
打开.nc文件
file_path = 'your_file.nc'
dataset = nc.Dataset(file_path, 'r')
读取变量
temperature = dataset.variables['temperature'][:]
print(temperature)
2、使用 xarray 库读取数据
import xarray as xr
打开.nc文件
file_path = 'your_file.nc'
dataset = xr.open_dataset(file_path)
读取变量
temperature = dataset['temperature']
print(temperature)
3、使用 h5netcdf 库读取数据
import h5netcdf
打开.nc文件
file_path = 'your_file.nc'
with h5netcdf.File(file_path, 'r') as f:
temperature = f.variables['temperature'][:]
print(temperature)
五、写入.nc文件
你还可以使用这些库将数据写入.nc文件。
1、使用 NetCDF4 库写入数据
import netCDF4 as nc
import numpy as np
创建一个新的.nc文件
file_path = 'new_file.nc'
dataset = nc.Dataset(file_path, 'w', format='NETCDF4')
创建维度
time_dim = dataset.createDimension('time', None)
lat_dim = dataset.createDimension('lat', 73)
lon_dim = dataset.createDimension('lon', 144)
创建变量
times = dataset.createVariable('time', np.float64, ('time',))
latitudes = dataset.createVariable('lat', np.float32, ('lat',))
longitudes = dataset.createVariable('lon', np.float32, ('lon',))
temperature = dataset.createVariable('temperature', np.float32, ('time', 'lat', 'lon'))
写入数据
times[:] = np.arange(0, 10)
latitudes[:] = np.linspace(-90, 90, 73)
longitudes[:] = np.linspace(-180, 180, 144)
temperature[0, :, :] = np.random.rand(73, 144)
关闭文件
dataset.close()
2、使用 xarray 库写入数据
import xarray as xr
import numpy as np
创建一个新的数据集
data = xr.Dataset(
{
"temperature": (["time", "lat", "lon"], np.random.rand(10, 73, 144)),
},
coords={
"time": np.arange(0, 10),
"lat": np.linspace(-90, 90, 73),
"lon": np.linspace(-180, 180, 144),
},
)
写入.nc文件
file_path = 'new_file.nc'
data.to_netcdf(file_path)
3、使用 h5netcdf 库写入数据
import h5netcdf
import numpy as np
创建一个新的.nc文件
file_path = 'new_file.nc'
with h5netcdf.File(file_path, 'w') as f:
f.dimensions['time'] = 10
f.dimensions['lat'] = 73
f.dimensions['lon'] = 144
f.create_variable('time', ('time',), dtype='f8')
f.create_variable('lat', ('lat',), dtype='f4')
f.create_variable('lon', ('lon',), dtype='f4')
f.create_variable('temperature', ('time', 'lat', 'lon'), dtype='f4')
f.variables['time'][:] = np.arange(0, 10)
f.variables['lat'][:] = np.linspace(-90, 90, 73)
f.variables['lon'][:] = np.linspace(-180, 180, 144)
f.variables['temperature'][0, :, :] = np.random.rand(73, 144)
六、处理复杂数据
NetCDF文件可以包含复杂的多维数据,使用以上库可以轻松处理这些数据。
1、处理多维数据
import netCDF4 as nc
import numpy as np
打开.nc文件
file_path = 'your_file.nc'
dataset = nc.Dataset(file_path, 'r')
读取多维变量
temperature = dataset.variables['temperature'][:, :, :]
print(temperature.shape)
2、使用 xarray 处理多维数据
import xarray as xr
打开.nc文件
file_path = 'your_file.nc'
dataset = xr.open_dataset(file_path)
读取多维变量
temperature = dataset['temperature']
print(temperature.shape)
七、处理时间序列数据
NetCDF文件常用于存储时间序列数据,使用这些库可以方便地处理这些数据。
1、读取时间序列数据
import netCDF4 as nc
打开.nc文件
file_path = 'your_file.nc'
dataset = nc.Dataset(file_path, 'r')
读取时间变量
times = dataset.variables['time'][:]
print(times)
2、使用 xarray 读取时间序列数据
import xarray as xr
打开.nc文件
file_path = 'your_file.nc'
dataset = xr.open_dataset(file_path)
读取时间变量
times = dataset['time']
print(times)
八、绘制数据
你可以使用 Matplotlib 或其他绘图库来可视化数据。
1、使用 Matplotlib 绘制数据
import netCDF4 as nc
import matplotlib.pyplot as plt
打开.nc文件
file_path = 'your_file.nc'
dataset = nc.Dataset(file_path, 'r')
读取变量
temperature = dataset.variables['temperature'][0, :, :]
绘制数据
plt.imshow(temperature, cmap='hot')
plt.colorbar()
plt.show()
2、使用 xarray 和 Matplotlib 绘制数据
import xarray as xr
import matplotlib.pyplot as plt
打开.nc文件
file_path = 'your_file.nc'
dataset = xr.open_dataset(file_path)
读取变量
temperature = dataset['temperature'][0, :, :]
绘制数据
temperature.plot()
plt.show()
九、并行处理和大数据
对于非常大的数据集,可以使用 dask 和 xarray 结合进行并行处理。
1、使用 dask 并行处理
import xarray as xr
import dask.array as da
打开.nc文件并使用 dask 进行并行处理
file_path = 'your_file.nc'
dataset = xr.open_dataset(file_path, chunks={'time': 10})
读取变量
temperature = dataset['temperature'].data
print(temperature)
十、总结
通过以上步骤,你可以使用NetCDF4、xarray、h5netcdf库打开和处理.nc文件。每个库都有其优点和使用场景,NetCDF4库适用于低级操作,xarray库适用于高级数据分析,而h5netcdf库是一个轻量级的选择。根据具体需求选择合适的库,可以更高效地处理和分析NetCDF文件。
相关问答FAQs:
Python中有哪些库可以用于打开nc文件?
在Python中,处理nc(NetCDF)文件的最常用库是netCDF4
和xarray
。netCDF4
库提供了对NetCDF文件的读写功能,而xarray
则在netCDF4
的基础上,提供了更为强大的数据分析能力,特别适合处理多维数组数据。您可以使用pip install netCDF4
或pip install xarray
命令安装这些库。
如何使用Python读取nc文件中的数据?
使用netCDF4
库读取nc文件数据的基本步骤包括:导入库、打开文件、访问数据变量。以下是一个简单的示例代码:
import netCDF4 as nc
# 打开nc文件
dataset = nc.Dataset('your_file.nc', mode='r')
# 读取变量
data_variable = dataset.variables['variable_name'][:]
# 关闭文件
dataset.close()
确保将your_file.nc
替换为您的文件名,并将variable_name
替换为您想要访问的变量名称。
如何处理和分析nc文件中的多维数据?
如果您的nc文件包含多维数据,xarray
库会非常有用。它能够轻松地处理多维数组,并提供丰富的数据分析功能。以下是使用xarray
读取nc文件并进行简单分析的代码示例:
import xarray as xr
# 打开nc文件
ds = xr.open_dataset('your_file.nc')
# 查看数据集信息
print(ds)
# 提取某个变量的数据
data = ds['variable_name']
# 进行一些简单的计算,比如求平均
mean_data = data.mean(dim='time')
这种方式不仅使数据处理变得更简单,也能提高分析效率。
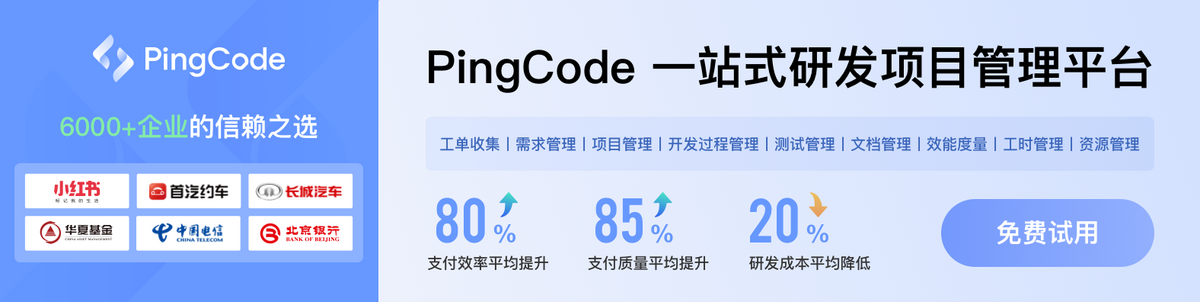