在Python项目中更改图片的方式有多种,具体取决于你需要进行的操作类型,如图像处理、图像转换、图像增强等。常见方法包括使用PIL(Pillow)、OpenCV、Matplotlib等库、每个库都有其独特的功能和优势。下面我们详细讲解如何使用这些库来进行图片的各种更改:
一、使用Pillow库进行图片更改
Pillow是一个强大的Python图像处理库,原名为PIL,支持打开、操作和保存多种格式的图像文件。
1、安装Pillow库
首先,我们需要安装Pillow库。可以通过pip进行安装:
pip install pillow
2、打开和保存图片
使用Pillow库,我们可以轻松地打开和保存图片。以下是一个简单的例子:
from PIL import Image
打开图片
image = Image.open('example.jpg')
保存图片
image.save('example_copy.jpg')
3、图像大小的调整
我们可以使用resize
方法来调整图像的大小:
from PIL import Image
打开图片
image = Image.open('example.jpg')
调整图像大小
resized_image = image.resize((800, 600))
保存调整后的图像
resized_image.save('resized_example.jpg')
4、图像旋转
Pillow库还支持图像的旋转操作:
from PIL import Image
打开图片
image = Image.open('example.jpg')
旋转图像
rotated_image = image.rotate(45)
保存旋转后的图像
rotated_image.save('rotated_example.jpg')
5、图像裁剪
我们可以使用crop
方法来裁剪图像:
from PIL import Image
打开图片
image = Image.open('example.jpg')
定义裁剪区域
left = 100
top = 100
right = 400
bottom = 400
crop_area = (left, top, right, bottom)
裁剪图像
cropped_image = image.crop(crop_area)
保存裁剪后的图像
cropped_image.save('cropped_example.jpg')
6、图像滤镜
Pillow库还支持为图像应用各种滤镜:
from PIL import Image, ImageFilter
打开图片
image = Image.open('example.jpg')
应用模糊滤镜
blurred_image = image.filter(ImageFilter.BLUR)
保存应用滤镜后的图像
blurred_image.save('blurred_example.jpg')
二、使用OpenCV库进行图片更改
OpenCV是一个开源计算机视觉库,支持多种图像处理操作。
1、安装OpenCV库
首先,我们需要安装OpenCV库。可以通过pip进行安装:
pip install opencv-python
2、打开和保存图片
使用OpenCV库,我们可以打开和保存图片:
import cv2
打开图片
image = cv2.imread('example.jpg')
保存图片
cv2.imwrite('example_copy.jpg', image)
3、图像大小的调整
我们可以使用resize
方法来调整图像的大小:
import cv2
打开图片
image = cv2.imread('example.jpg')
调整图像大小
resized_image = cv2.resize(image, (800, 600))
保存调整后的图像
cv2.imwrite('resized_example.jpg', resized_image)
4、图像旋转
OpenCV库支持图像的旋转操作:
import cv2
打开图片
image = cv2.imread('example.jpg')
获取图像中心
center = (image.shape[1] // 2, image.shape[0] // 2)
定义旋转矩阵
matrix = cv2.getRotationMatrix2D(center, 45, 1.0)
旋转图像
rotated_image = cv2.warpAffine(image, matrix, (image.shape[1], image.shape[0]))
保存旋转后的图像
cv2.imwrite('rotated_example.jpg', rotated_image)
5、图像裁剪
我们可以通过数组切片来裁剪图像:
import cv2
打开图片
image = cv2.imread('example.jpg')
定义裁剪区域
crop_area = image[100:400, 100:400]
保存裁剪后的图像
cv2.imwrite('cropped_example.jpg', crop_area)
6、图像滤镜
OpenCV库也支持为图像应用各种滤镜:
import cv2
打开图片
image = cv2.imread('example.jpg')
应用模糊滤镜
blurred_image = cv2.GaussianBlur(image, (15, 15), 0)
保存应用滤镜后的图像
cv2.imwrite('blurred_example.jpg', blurred_image)
三、使用Matplotlib库进行图片更改
Matplotlib是一个广泛使用的绘图库,虽然它主要用于绘制图表,但也可以用于简单的图像处理任务。
1、安装Matplotlib库
首先,我们需要安装Matplotlib库。可以通过pip进行安装:
pip install matplotlib
2、打开和保存图片
使用Matplotlib库,我们可以打开和保存图片:
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
打开图片
image = mpimg.imread('example.jpg')
显示图片
plt.imshow(image)
plt.show()
保存图片
plt.imsave('example_copy.jpg', image)
3、图像大小的调整
我们可以使用resize
函数来调整图像的大小:
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
import numpy as np
from skimage.transform import resize
打开图片
image = mpimg.imread('example.jpg')
调整图像大小
resized_image = resize(image, (600, 800))
保存调整后的图像
plt.imsave('resized_example.jpg', resized_image)
4、图像旋转
Matplotlib库支持图像的旋转操作:
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
from skimage.transform import rotate
打开图片
image = mpimg.imread('example.jpg')
旋转图像
rotated_image = rotate(image, 45)
保存旋转后的图像
plt.imsave('rotated_example.jpg', rotated_image)
5、图像裁剪
我们可以通过数组切片来裁剪图像:
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
打开图片
image = mpimg.imread('example.jpg')
定义裁剪区域
cropped_image = image[100:400, 100:400]
保存裁剪后的图像
plt.imsave('cropped_example.jpg', cropped_image)
6、图像滤镜
虽然Matplotlib本身不提供滤镜功能,但我们可以结合其他库如scipy
来应用滤镜:
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
from scipy.ndimage import gaussian_filter
打开图片
image = mpimg.imread('example.jpg')
应用模糊滤镜
blurred_image = gaussian_filter(image, sigma=2)
保存应用滤镜后的图像
plt.imsave('blurred_example.jpg', blurred_image)
四、其他图像处理库及工具
除了上述的Pillow、OpenCV和Matplotlib库,还有一些其他有用的图像处理库和工具。
1、Scikit-Image库
Scikit-Image是一个用于图像处理的Python库,基于SciPy构建,提供了许多高级图像处理功能。
安装Scikit-Image库
可以通过pip进行安装:
pip install scikit-image
基本图像操作
from skimage import io, transform
打开图片
image = io.imread('example.jpg')
调整图像大小
resized_image = transform.resize(image, (600, 800))
旋转图像
rotated_image = transform.rotate(image, 45)
保存调整后的图像
io.imsave('resized_example.jpg', resized_image)
io.imsave('rotated_example.jpg', rotated_image)
2、Imageio库
Imageio是一个用于读取和写入图像(以及其他媒体数据)的Python库,支持多种图像格式。
安装Imageio库
可以通过pip进行安装:
pip install imageio
基本图像操作
import imageio
打开图片
image = imageio.imread('example.jpg')
保存图片
imageio.imwrite('example_copy.jpg', image)
3、TensorFlow和Keras
TensorFlow和Keras不仅用于深度学习模型的训练和推理,还可以用于图像预处理和增强。
安装TensorFlow和Keras
可以通过pip进行安装:
pip install tensorflow
pip install keras
图像预处理
import tensorflow as tf
from tensorflow.keras.preprocessing import image
加载图片
img = image.load_img('example.jpg', target_size=(224, 224))
转换为数组
x = image.img_to_array(img)
调整图像大小
resized_image = tf.image.resize(x, [600, 800])
旋转图像
rotated_image = tf.image.rot90(x, k=1)
保存图像
image.save_img('resized_example.jpg', resized_image)
image.save_img('rotated_example.jpg', rotated_image)
五、图像批处理
在实际项目中,常常需要对大量图像进行批量处理。我们可以使用Python脚本结合上述库来实现图像的批处理。
1、批量调整图像大小
import os
from PIL import Image
def resize_images(input_folder, output_folder, size):
if not os.path.exists(output_folder):
os.makedirs(output_folder)
for filename in os.listdir(input_folder):
if filename.endswith('.jpg') or filename.endswith('.png'):
img = Image.open(os.path.join(input_folder, filename))
img = img.resize(size)
img.save(os.path.join(output_folder, filename))
resize_images('input_images', 'output_images', (800, 600))
2、批量旋转图像
import os
from PIL import Image
def rotate_images(input_folder, output_folder, angle):
if not os.path.exists(output_folder):
os.makedirs(output_folder)
for filename in os.listdir(input_folder):
if filename.endswith('.jpg') or filename.endswith('.png'):
img = Image.open(os.path.join(input_folder, filename))
img = img.rotate(angle)
img.save(os.path.join(output_folder, filename))
rotate_images('input_images', 'output_images', 45)
3、批量应用滤镜
import os
from PIL import Image, ImageFilter
def apply_filter_to_images(input_folder, output_folder, filter):
if not os.path.exists(output_folder):
os.makedirs(output_folder)
for filename in os.listdir(input_folder):
if filename.endswith('.jpg') or filename.endswith('.png'):
img = Image.open(os.path.join(input_folder, filename))
img = img.filter(filter)
img.save(os.path.join(output_folder, filename))
apply_filter_to_images('input_images', 'output_images', ImageFilter.BLUR)
六、结论
在Python项目中更改图片有多种方式,不同的库提供了不同的功能和使用方法。Pillow、OpenCV、Matplotlib等库各有优势,可以根据具体需求选择合适的库来实现图像的打开、保存、调整大小、旋转、裁剪和应用滤镜等操作。此外,还可以结合其他图像处理库如Scikit-Image、Imageio,以及深度学习框架TensorFlow和Keras来实现更高级的图像处理任务。通过批处理脚本,可以方便地对大量图像进行批量操作,提高工作效率。
相关问答FAQs:
在Python项目中,如何替换特定目录下的图片文件?
要替换特定目录下的图片文件,可以使用Python的os
模块和shutil
模块。首先,使用os
模块遍历目录,找到需要替换的图片文件。接着,使用shutil.copy
或shutil.move
方法将新的图片文件复制或移动到目标位置。确保在替换前备份原始图片,以免数据丢失。
如何在Python项目中动态加载和显示图片?
动态加载和显示图片通常可以通过PIL
库(Pillow)和matplotlib
库实现。使用Pillow
打开图片后,可以通过matplotlib
的imshow
函数展示图片。这种方法适合需要频繁更新或显示不同图片的场景,比如图像处理或机器学习项目中的可视化。
在Python中,如何使用图形用户界面(GUI)来选择和更改图片?
可以使用tkinter
库创建图形用户界面,通过按钮或菜单让用户选择图片文件。使用tkinter.filedialog.askopenfilename
方法可以弹出文件选择对话框,用户选择后可以使用PIL
库加载并显示所选的图片。这种方法提高了用户体验,使得程序更加友好和直观。
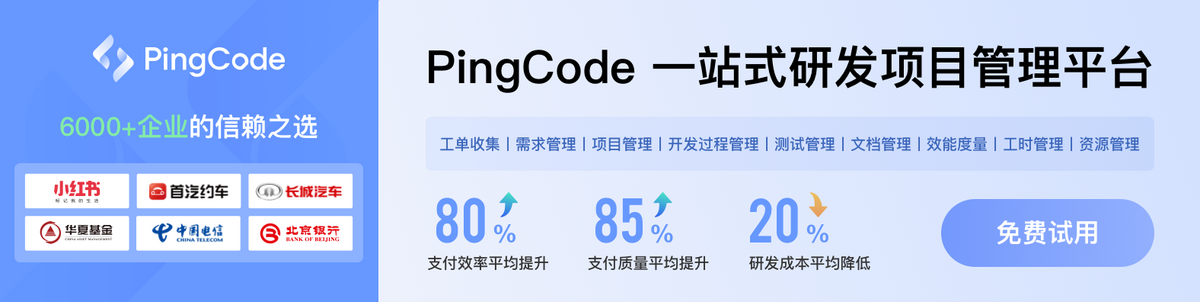