通过使用条件语句、正则表达式、内置的字符串方法等方式,可以确定Python是否有匹配。在这些方法中,正则表达式是一种强大且灵活的工具,可以用于处理复杂的匹配需求。
正则表达式(Regular Expressions,简称regex)是一种用于匹配字符串模式的工具。在Python中,可以通过re
模块来实现正则表达式的功能。使用正则表达式可以轻松查找、替换、验证字符串中的特定模式。下面将详细描述如何使用正则表达式来确定Python中是否有匹配。
一、使用正则表达式确定匹配
1、引入re
模块并定义正则表达式模式
在使用正则表达式之前,需要先引入re
模块,然后定义一个正则表达式模式。正则表达式模式可以是任何符合特定规则的字符串。
import re
pattern = r'\d+' # 匹配一个或多个数字
2、使用re.search()
方法
re.search()
方法用于搜索字符串中是否存在与正则表达式模式匹配的部分。如果找到匹配项,则返回一个匹配对象;如果没有找到匹配项,则返回None
。
text = "The price is 100 dollars."
match = re.search(pattern, text)
if match:
print("Match found:", match.group())
else:
print("No match found.")
3、使用re.match()
方法
re.match()
方法用于从字符串的开头开始匹配正则表达式。如果在字符串的开头找到匹配项,则返回一个匹配对象;如果没有找到匹配项,则返回None
。
text = "100 dollars is the price."
match = re.match(pattern, text)
if match:
print("Match found at the beginning:", match.group())
else:
print("No match found at the beginning.")
4、使用re.findall()
方法
re.findall()
方法用于查找字符串中所有与正则表达式模式匹配的部分,并以列表形式返回所有匹配项。
text = "The price is 100 dollars and the discount is 20 dollars."
matches = re.findall(pattern, text)
if matches:
print("All matches found:", matches)
else:
print("No matches found.")
二、使用内置字符串方法确定匹配
1、使用str.find()
方法
str.find()
方法用于查找子字符串在字符串中的位置。如果找到子字符串,则返回其在字符串中的起始位置索引;如果没有找到子字符串,则返回-1
。
text = "The price is 100 dollars."
substring = "100"
position = text.find(substring)
if position != -1:
print(f"Substring '{substring}' found at position {position}.")
else:
print(f"Substring '{substring}' not found.")
2、使用str.index()
方法
str.index()
方法与str.find()
方法类似,但如果没有找到子字符串,则会引发ValueError
异常。
text = "The price is 100 dollars."
substring = "100"
try:
position = text.index(substring)
print(f"Substring '{substring}' found at position {position}.")
except ValueError:
print(f"Substring '{substring}' not found.")
3、使用str.startswith()
方法
str.startswith()
方法用于检查字符串是否以指定的子字符串开头。如果是,则返回True
;否则返回False
。
text = "100 dollars is the price."
substring = "100"
if text.startswith(substring):
print(f"The string starts with '{substring}'.")
else:
print(f"The string does not start with '{substring}'.")
4、使用str.endswith()
方法
str.endswith()
方法用于检查字符串是否以指定的子字符串结尾。如果是,则返回True
;否则返回False
。
text = "The price is 100 dollars."
substring = "dollars."
if text.endswith(substring):
print(f"The string ends with '{substring}'.")
else:
print(f"The string does not end with '{substring}'.")
三、使用条件语句和循环确定匹配
1、使用in
运算符
in
运算符用于检查子字符串是否存在于字符串中。如果存在,则返回True
;否则返回False
。
text = "The price is 100 dollars."
substring = "100"
if substring in text:
print(f"The substring '{substring}' is found in the string.")
else:
print(f"The substring '{substring}' is not found in the string.")
2、使用循环遍历字符串
可以使用循环遍历字符串中的每个字符,并检查是否存在匹配的子字符串。
text = "The price is 100 dollars."
substring = "100"
length = len(substring)
found = False
for i in range(len(text) - length + 1):
if text[i:i + length] == substring:
found = True
break
if found:
print(f"The substring '{substring}' is found in the string.")
else:
print(f"The substring '{substring}' is not found in the string.")
四、使用第三方库确定匹配
除了Python内置的字符串方法和正则表达式外,还有一些第三方库可以用于处理字符串匹配。例如,regex
库是一个增强版的正则表达式库,提供了更多的功能和更好的性能。
1、安装regex
库
可以使用pip
命令安装regex
库:
pip install regex
2、使用regex
库进行匹配
regex
库的使用方法与re
模块类似,但提供了更多的选项和功能。
import regex
pattern = r'\d+' # 匹配一个或多个数字
text = "The price is 100 dollars."
match = regex.search(pattern, text)
if match:
print("Match found:", match.group())
else:
print("No match found.")
五、总结
通过以上方法,可以灵活地确定Python中是否存在匹配。使用正则表达式是处理复杂匹配需求的强大工具,内置的字符串方法适用于简单的匹配场景,条件语句和循环提供了基本的匹配功能,而第三方库则提供了更多的选项和功能。根据具体需求选择合适的方法,可以有效地解决字符串匹配问题。
六、案例分析
为了更好地理解如何确定Python是否有匹配,下面通过几个具体的案例来分析和演示不同方法的应用。
案例1:验证电子邮件地址格式
电子邮件地址的格式通常包含用户名、@符号和域名。可以使用正则表达式来验证字符串是否符合电子邮件地址的格式。
import re
def is_valid_email(email):
pattern = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
return re.match(pattern, email) is not None
emails = ["test@example.com", "invalid-email", "user@domain", "user@domain.com"]
for email in emails:
if is_valid_email(email):
print(f"'{email}' is a valid email address.")
else:
print(f"'{email}' is not a valid email address.")
案例2:提取文本中的电话号码
电话号码通常由数字、空格、连字符或括号组成。可以使用正则表达式从文本中提取所有的电话号码。
import re
def extract_phone_numbers(text):
pattern = r'\(?\d{3}\)?[-.\s]?\d{3}[-.\s]?\d{4}'
return re.findall(pattern, text)
text = "Contact us at (123) 456-7890 or 987-654-3210."
phone_numbers = extract_phone_numbers(text)
print("Extracted phone numbers:", phone_numbers)
案例3:检查密码强度
强密码通常包含大写字母、小写字母、数字和特殊字符。可以使用正则表达式来检查字符串是否符合强密码的要求。
import re
def is_strong_password(password):
pattern = r'^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$'
return re.match(pattern, password) is not None
passwords = ["Password123!", "weakpassword", "Strong1!", "StrongerPassword1!"]
for password in passwords:
if is_strong_password(password):
print(f"'{password}' is a strong password.")
else:
print(f"'{password}' is not a strong password.")
七、进阶技巧
1、使用命名捕获组
在正则表达式中,可以使用命名捕获组来给匹配的子字符串命名,从而更方便地提取和处理匹配项。
import re
def extract_named_groups(text):
pattern = r'(?P<area_code>\d{3})[-.\s]?(?P<exchange_code>\d{3})[-.\s]?(?P<number>\d{4})'
match = re.search(pattern, text)
if match:
return match.groupdict()
return None
text = "Contact us at 123-456-7890."
named_groups = extract_named_groups(text)
print("Named groups:", named_groups)
2、使用非贪婪匹配
默认情况下,正则表达式采用贪婪匹配,即尽可能多地匹配字符。可以使用非贪婪匹配来尽可能少地匹配字符,从而避免过度匹配。
import re
def extract_non_greedy(text):
pattern = r'<.*?>'
return re.findall(pattern, text)
text = "<p>This is a paragraph.</p><p>This is another paragraph.</p>"
non_greedy_matches = extract_non_greedy(text)
print("Non-greedy matches:", non_greedy_matches)
3、使用断言
断言是一种特殊的正则表达式,用于指定匹配项的上下文条件。常见的断言包括正向先行断言、负向先行断言、正向后行断言和负向后行断言。
import re
def extract_words_followed_by_exclamation(text):
pattern = r'\b\w+(?=!)'
return re.findall(pattern, text)
text = "Hello! How are you? Wow! Amazing!"
words_followed_by_exclamation = extract_words_followed_by_exclamation(text)
print("Words followed by exclamation:", words_followed_by_exclamation)
八、常见问题及解决方案
1、匹配多行文本
在处理多行文本时,可以使用re.MULTILINE
标志来使正则表达式的锚点(如^
和$
)匹配每一行的开头和结尾。
import re
def match_multiline_text(text):
pattern = r'^\w+'
return re.findall(pattern, text, re.MULTILINE)
text = """First line
Second line
Third line"""
multiline_matches = match_multiline_text(text)
print("Multiline matches:", multiline_matches)
2、忽略大小写匹配
在进行匹配时,可以使用re.IGNORECASE
标志来忽略字符的大小写,从而实现不区分大小写的匹配。
import re
def match_ignore_case(text):
pattern = r'hello'
return re.findall(pattern, text, re.IGNORECASE)
text = "Hello, hello, HELLO!"
ignore_case_matches = match_ignore_case(text)
print("Ignore case matches:", ignore_case_matches)
3、处理特殊字符
在正则表达式中,一些字符具有特殊含义(如.
、*
、+
等)。如果需要匹配这些字符本身,可以使用反斜杠进行转义。
import re
def match_special_characters(text):
pattern = r'\.'
return re.findall(pattern, text)
text = "Match the period character."
special_char_matches = match_special_characters(text)
print("Special character matches:", special_char_matches)
九、优化正则表达式性能
正则表达式的性能可能会受到模式复杂度和输入字符串长度的影响。以下是一些优化正则表达式性能的建议:
1、避免过度回溯
在设计正则表达式模式时,应避免使用可能导致过度回溯的结构,如嵌套的量词和不必要的捕获组。
import re
def optimized_pattern(text):
pattern = r'(?:\d+|[a-zA-Z]+)'
return re.findall(pattern, text)
text = "123abc456def"
optimized_matches = optimized_pattern(text)
print("Optimized matches:", optimized_matches)
2、使用预编译模式
对于需要多次使用的正则表达式,可以使用re.compile()
方法预编译模式,从而提高匹配的性能。
import re
def precompiled_pattern(text, pattern):
compiled_pattern = re.compile(pattern)
return compiled_pattern.findall(text)
text = "The price is 100 dollars and the discount is 20 dollars."
pattern = r'\d+'
precompiled_matches = precompiled_pattern(text, pattern)
print("Precompiled matches:", precompiled_matches)
3、分割大文本
对于非常大的文本,可以将其分割为较小的部分进行匹配,从而减少单次匹配的负担。
import re
def split_and_match(text, pattern, chunk_size=1000):
matches = []
for i in range(0, len(text), chunk_size):
chunk = text[i:i + chunk_size]
matches.extend(re.findall(pattern, chunk))
return matches
text = "a" * 5000 + "100" + "b" * 5000 + "200"
pattern = r'\d+'
split_matches = split_and_match(text, pattern)
print("Split matches:", split_matches)
十、总结
确定Python是否有匹配可以通过多种方式实现,包括正则表达式、内置字符串方法、条件语句和循环等。正则表达式是一种强大且灵活的工具,适用于处理复杂的匹配需求;内置字符串方法提供了简单高效的解决方案;条件语句和循环提供了基本的匹配功能。通过结合使用这些方法,可以有效地解决各种字符串匹配问题。同时,优化正则表达式性能和处理常见问题也是提高匹配效率和准确性的关键。
在实际应用中,根据具体需求选择合适的方法,并灵活运用进阶技巧和优化策略,可以更好地解决字符串匹配问题。希望本文提供的内容能够帮助您深入理解和应用Python中的匹配技术。
相关问答FAQs:
如何检查Python中是否存在特定的匹配项?
在Python中,您可以使用re
模块来检查字符串中是否有匹配项。通过re.search()
函数,您可以搜索整个字符串以找到匹配的模式。如果找到了匹配项,它将返回一个匹配对象;如果没有找到,则返回None
。示例代码如下:
import re
pattern = r"your_pattern"
string = "your_string"
match = re.search(pattern, string)
if match:
print("匹配项存在")
else:
print("没有匹配项")
这段代码可以帮助您轻松确定字符串中是否有您想要的匹配。
在使用正则表达式时,如何优化匹配效率?
为了提高正则表达式的匹配效率,可以考虑使用原生的字符串方法,如in
关键字进行简单的查找。对于复杂的匹配,尽量避免使用过于复杂的模式,并使用非捕获组来优化性能。此外,使用re.compile()
将正则表达式编译为可重用的模式,可以提高多次匹配的效率。
如果没有找到匹配,如何处理?
如果在Python中没有找到匹配项,您可以选择多种处理方式。例如,可以通过返回一个默认值、抛出异常或记录日志来处理这种情况。根据具体需求,您可以设计合适的错误处理逻辑,以确保程序的健壮性和用户体验。使用try-except
块也可以捕获潜在的错误,确保代码的正常运行。
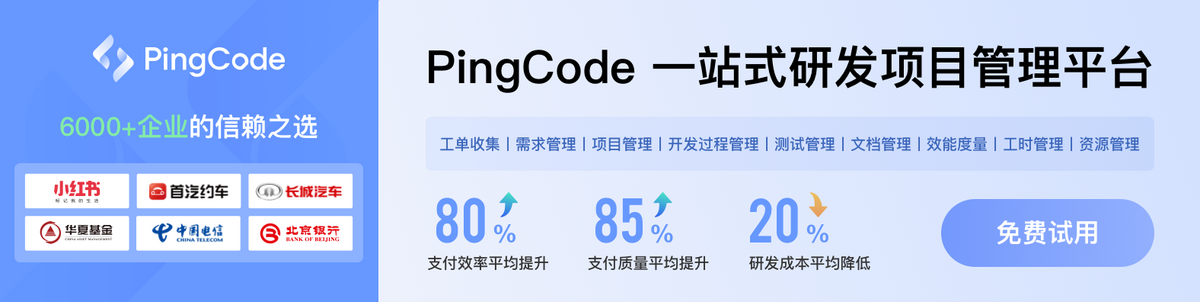