在Python中,使用队列(Queue)和线程(Thread)可以方便地实现多线程编程。队列模块提供了一种线程安全的队列实现,能够在多线程环境中高效地传递数据。通过将线程与队列结合,可以轻松地管理和协调多线程任务,避免线程之间的数据竞争问题。在实际应用中,可以通过以下步骤来实现:
- 导入必要的模块:需要导入
queue
和threading
模块。 - 创建队列对象:使用
queue.Queue
创建一个队列对象。 - 定义线程任务函数:定义一个函数,该函数将作为线程执行的任务。通常,该函数会从队列中获取任务并执行。
- 创建并启动线程:使用
threading.Thread
创建线程,并将线程任务函数作为参数传递给线程对象。 - 将任务添加到队列:将需要处理的任务添加到队列中。
- 等待所有线程完成:使用
join
方法等待所有线程执行完毕。
下面是一个具体的示例,展示了如何使用队列和线程在Python中实现多线程任务处理:
import threading
import queue
import time
定义线程任务函数
def thread_task(q, thread_id):
while True:
task = q.get()
if task is None:
break
print(f"Thread {thread_id} is processing task: {task}")
time.sleep(1) # 模拟任务处理时间
q.task_done()
创建队列对象
task_queue = queue.Queue()
创建并启动线程
num_threads = 5
threads = []
for i in range(num_threads):
thread = threading.Thread(target=thread_task, args=(task_queue, i))
thread.start()
threads.append(thread)
将任务添加到队列
num_tasks = 10
for task in range(num_tasks):
task_queue.put(task)
等待队列中的所有任务完成
task_queue.join()
停止所有线程
for i in range(num_threads):
task_queue.put(None)
for thread in threads:
thread.join()
print("All tasks are completed.")
在上面的示例中,我们使用queue.Queue
创建了一个任务队列,并定义了一个线程任务函数thread_task
。我们创建了多个线程并启动它们,每个线程从队列中获取任务并处理。最后,我们等待所有任务完成,并使用None
标记停止线程。
一、导入必要的模块
在使用队列和线程时,首先需要导入queue
和threading
模块。这两个模块分别提供了队列和线程的实现:
import threading
import queue
threading
模块提供了创建和管理线程的功能,而queue
模块提供了线程安全的队列实现。
二、创建队列对象
创建队列对象是多线程任务管理的基础。可以使用queue.Queue
创建一个FIFO队列:
task_queue = queue.Queue()
队列对象可以存储需要处理的任务,并且能够确保线程之间的数据传递是线程安全的。
三、定义线程任务函数
定义线程任务函数是实现多线程任务处理的重要步骤。通常,该函数会从队列中获取任务并执行:
def thread_task(q, thread_id):
while True:
task = q.get()
if task is None:
break
print(f"Thread {thread_id} is processing task: {task}")
time.sleep(1) # 模拟任务处理时间
q.task_done()
在这个函数中,线程会不断从队列中获取任务并处理,直到队列中没有更多的任务。通过调用q.task_done()
通知队列任务已经完成。
四、创建并启动线程
创建并启动线程是多线程任务管理的关键步骤。可以使用threading.Thread
创建线程,并将线程任务函数作为参数传递给线程对象:
num_threads = 5
threads = []
for i in range(num_threads):
thread = threading.Thread(target=thread_task, args=(task_queue, i))
thread.start()
threads.append(thread)
在这个示例中,我们创建了5个线程,每个线程执行thread_task
函数,并传递队列对象和线程ID作为参数。
五、将任务添加到队列
将任务添加到队列是多线程任务管理的核心步骤。可以使用put
方法将任务添加到队列中:
num_tasks = 10
for task in range(num_tasks):
task_queue.put(task)
在这个示例中,我们添加了10个任务到队列中,每个任务是一个整数。
六、等待所有线程完成
等待所有线程完成是确保所有任务都已经处理完毕的重要步骤。可以使用join
方法等待队列中的所有任务完成,并使用None
标记停止线程:
task_queue.join()
for i in range(num_threads):
task_queue.put(None)
for thread in threads:
thread.join()
在这个示例中,我们首先等待队列中的所有任务完成,然后使用None
标记停止线程,最后等待所有线程停止。
七、扩展应用
以上示例展示了如何使用队列和线程实现基本的多线程任务管理。在实际应用中,可以根据具体需求对示例进行扩展和优化。以下是几个常见的扩展应用:
1. 处理复杂任务
在实际应用中,任务可能会更加复杂。可以通过定义更复杂的任务结构和处理逻辑来适应不同的应用场景。例如,可以将任务定义为字典或对象,并在线程任务函数中处理复杂的数据结构:
def thread_task(q, thread_id):
while True:
task = q.get()
if task is None:
break
print(f"Thread {thread_id} is processing task: {task['task_id']}")
# 处理复杂任务逻辑
q.task_done()
将复杂任务添加到队列
tasks = [{'task_id': i, 'data': f"data_{i}"} for i in range(num_tasks)]
for task in tasks:
task_queue.put(task)
2. 错误处理与日志记录
在多线程任务处理中,错误处理和日志记录是非常重要的。可以在线程任务函数中添加错误处理逻辑和日志记录代码,以确保任务处理的稳定性和可追溯性:
import logging
配置日志记录
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def thread_task(q, thread_id):
while True:
task = q.get()
if task is None:
break
try:
print(f"Thread {thread_id} is processing task: {task}")
# 处理任务逻辑
except Exception as e:
logging.error(f"Error processing task {task}: {e}")
finally:
q.task_done()
3. 动态调整线程池大小
在某些情况下,可能需要根据实际任务负载动态调整线程池大小。可以通过创建一个线程管理器来动态添加或移除线程,以适应不同的任务负载:
class ThreadPoolManager:
def __init__(self, num_threads):
self.num_threads = num_threads
self.threads = []
self.queue = queue.Queue()
self._create_threads()
def _create_threads(self):
for i in range(self.num_threads):
thread = threading.Thread(target=self._thread_task, args=(i,))
thread.start()
self.threads.append(thread)
def _thread_task(self, thread_id):
while True:
task = self.queue.get()
if task is None:
break
print(f"Thread {thread_id} is processing task: {task}")
self.queue.task_done()
def add_task(self, task):
self.queue.put(task)
def wait_for_completion(self):
self.queue.join()
for i in range(self.num_threads):
self.queue.put(None)
for thread in self.threads:
thread.join()
def adjust_thread_pool_size(self, new_size):
if new_size > self.num_threads:
for i in range(self.num_threads, new_size):
thread = threading.Thread(target=self._thread_task, args=(i,))
thread.start()
self.threads.append(thread)
elif new_size < self.num_threads:
for i in range(new_size, self.num_threads):
self.queue.put(None)
self.num_threads = new_size
使用线程池管理器
thread_pool = ThreadPoolManager(num_threads=5)
for task in range(num_tasks):
thread_pool.add_task(task)
thread_pool.wait_for_completion()
八、实际应用案例
为了更好地理解队列和线程在Python中的实际应用,下面是几个实际应用案例:
1. 数据爬取
在数据爬取场景中,可以使用队列和线程实现多线程爬取,提高数据爬取效率:
import requests
def fetch_data(url):
response = requests.get(url)
return response.text
def thread_task(q, thread_id):
while True:
url = q.get()
if url is None:
break
try:
data = fetch_data(url)
print(f"Thread {thread_id} fetched data from {url}")
# 处理爬取的数据
except Exception as e:
logging.error(f"Error fetching data from {url}: {e}")
finally:
q.task_done()
创建并启动线程
thread_pool = ThreadPoolManager(num_threads=5)
urls = ["http://example.com/page1", "http://example.com/page2", "http://example.com/page3"]
for url in urls:
thread_pool.add_task(url)
thread_pool.wait_for_completion()
2. 文件处理
在文件处理场景中,可以使用队列和线程实现多线程文件处理,提高处理效率:
def process_file(file_path):
with open(file_path, 'r') as file:
data = file.read()
# 处理文件数据
return data
def thread_task(q, thread_id):
while True:
file_path = q.get()
if file_path is None:
break
try:
data = process_file(file_path)
print(f"Thread {thread_id} processed file: {file_path}")
# 处理文件数据
except Exception as e:
logging.error(f"Error processing file {file_path}: {e}")
finally:
q.task_done()
创建并启动线程
thread_pool = ThreadPoolManager(num_threads=5)
file_paths = ["file1.txt", "file2.txt", "file3.txt"]
for file_path in file_paths:
thread_pool.add_task(file_path)
thread_pool.wait_for_completion()
3. 数据处理与分析
在数据处理与分析场景中,可以使用队列和线程实现多线程数据处理,提高处理效率:
import numpy as np
def process_data(data):
result = np.mean(data)
return result
def thread_task(q, thread_id):
while True:
data = q.get()
if data is None:
break
try:
result = process_data(data)
print(f"Thread {thread_id} processed data: {result}")
# 处理分析结果
except Exception as e:
logging.error(f"Error processing data: {e}")
finally:
q.task_done()
创建并启动线程
thread_pool = ThreadPoolManager(num_threads=5)
data_batches = [np.random.rand(100) for _ in range(10)]
for data in data_batches:
thread_pool.add_task(data)
thread_pool.wait_for_completion()
九、优化与注意事项
在使用队列和线程实现多线程任务管理时,有几个优化和注意事项需要考虑:
1. 避免死锁
在多线程环境中,容易发生死锁问题。确保在使用queue.Queue
时,正确使用q.task_done()
方法,以避免死锁。
2. 控制线程数量
在实际应用中,线程数量过多可能导致系统资源耗尽。根据具体应用场景,合理控制线程数量,以保证系统稳定性。
3. 锁机制
在多线程环境中,访问共享资源时需要使用锁机制,以确保线程安全。例如,可以使用threading.Lock
来保护共享资源:
lock = threading.Lock()
def thread_task(q, thread_id):
while True:
task = q.get()
if task is None:
break
with lock:
# 访问共享资源
print(f"Thread {thread_id} is processing task: {task}")
q.task_done()
4. 使用线程池
在某些情况下,使用线程池可以简化多线程任务管理。可以使用concurrent.futures.ThreadPoolExecutor
来创建线程池,并提交任务:
from concurrent.futures import ThreadPoolExecutor
def thread_task(task):
print(f"Processing task: {task}")
with ThreadPoolExecutor(max_workers=5) as executor:
tasks = [executor.submit(thread_task, task) for task in range(num_tasks)]
十、总结
通过以上内容,我们深入探讨了如何在Python中使用队列和线程实现多线程任务管理。通过合理使用队列和线程,可以有效地提高多线程任务处理的效率和稳定性。在实际应用中,可以根据具体需求对示例进行扩展和优化,以适应不同的应用场景。希望通过本文的介绍,能够帮助读者更好地理解和应用Python中的队列和线程,实现高效的多线程任务管理。
相关问答FAQs:
如何在Python中使用队列来管理线程?
在Python中,可以使用queue
模块来创建线程安全的队列。通过Queue
类,可以轻松地将任务放入队列,并由多个线程同时取出并处理。创建队列后,可以使用put()
方法添加任务,使用get()
方法取出任务,确保多线程环境中的数据安全。
使用线程池和队列有什么优势?
使用线程池结合队列能够提高程序的效率,避免频繁创建和销毁线程的开销。通过concurrent.futures.ThreadPoolExecutor
,您可以创建一个线程池,将队列与线程池结合,可以实现更好的资源管理和任务调度,提升程序的运行效率。
在队列中添加线程时需要注意哪些问题?
在队列中添加线程时,需确保线程安全。使用Queue
类能够自动处理线程之间的竞争问题。此外,合理设置队列的大小可以避免内存过载的问题,确保程序在高负载情况下依然能够稳定运行。还应注意线程的生命周期管理,以防止出现线程泄漏。
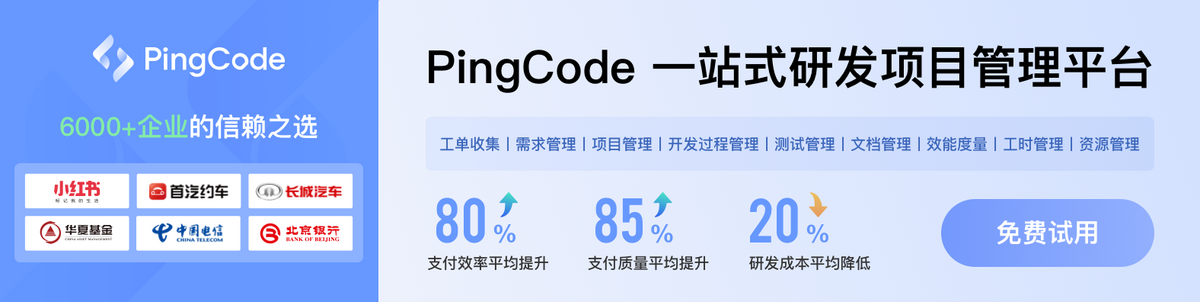