要用Python开发语音助手,你需要使用语音识别库、文本到语音转换库、自然语言处理库,并结合逻辑控制来实现。 常用的库包括:SpeechRecognition、pyttsx3、NLTK等。以下是详细描述如何使用SpeechRecognition库进行语音识别。
SpeechRecognition库是一个流行的Python库,它提供了简单的API来处理从麦克风或音频文件中的语音转换为文本。要使用这个库,你首先需要安装它:
pip install SpeechRecognition
下面是一个简单的示例代码,演示如何使用SpeechRecognition库来识别麦克风输入的语音:
import speech_recognition as sr
创建识别器对象
recognizer = sr.Recognizer()
使用麦克风作为音频输入源
with sr.Microphone() as source:
print("请说话:")
audio = recognizer.listen(source)
try:
# 使用Google Web Speech API将音频转换为文本
text = recognizer.recognize_google(audio, language="zh-CN")
print("你说的是: " + text)
except sr.UnknownValueError:
print("无法识别音频")
except sr.RequestError as e:
print("请求错误; {0}".format(e))
这个示例代码展示了如何捕获麦克风输入并将其转换为文本。接下来,我们将详细讨论如何开发一个完整的语音助手。
一、语音识别
SpeechRecognition库
SpeechRecognition库提供了多个语音识别引擎的接口,包括Google Web Speech API、CMU Sphinx、Microsoft Bing Voice Recognition等。通过简单的API调用,可以轻松实现语音识别功能。
安装和基本使用
首先,确保你已经安装了SpeechRecognition库:
pip install SpeechRecognition
接下来,使用以下代码实现语音识别:
import speech_recognition as sr
def recognize_speech_from_mic():
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("请说话:")
audio = recognizer.listen(source)
try:
text = recognizer.recognize_google(audio, language="zh-CN")
print("你说的是: " + text)
except sr.UnknownValueError:
print("无法识别音频")
except sr.RequestError as e:
print("请求错误; {0}".format(e))
return text
recognized_text = recognize_speech_from_mic()
提高语音识别准确性
为了提高语音识别的准确性,可以使用以下几种方法:
- 减少背景噪音:在安静的环境中使用麦克风,减少背景噪音。
- 调整麦克风灵敏度:可以通过调整麦克风的灵敏度来提高识别准确性。
- 训练自定义模型:使用CMU Sphinx等引擎,可以训练自定义语音模型,以提高特定领域的识别准确性。
二、文本到语音转换
pyttsx3库
pyttsx3是一个文本到语音转换库,支持多种语音引擎,包括SAPI5(Windows)、nsss(Mac OS X)和espeak(Linux)。它支持离线工作,并提供了简单的API来将文本转换为语音。
安装和基本使用
首先,安装pyttsx3库:
pip install pyttsx3
使用以下代码将文本转换为语音:
import pyttsx3
def text_to_speech(text):
engine = pyttsx3.init()
engine.say(text)
engine.runAndWait()
text_to_speech("你好,这是一个语音助手示例。")
调整语音属性
pyttsx3库允许调整语音属性,如语速、音量和声音类型。以下是一些示例代码:
def text_to_speech_with_custom_settings(text):
engine = pyttsx3.init()
# 设置语速
engine.setProperty('rate', 150)
# 设置音量
engine.setProperty('volume', 0.9)
# 设置声音类型
voices = engine.getProperty('voices')
engine.setProperty('voice', voices[1].id)
engine.say(text)
engine.runAndWait()
text_to_speech_with_custom_settings("这是一个带有自定义设置的语音助手示例。")
三、自然语言处理
NLTK库
NLTK(Natural Language Toolkit)是一个广泛使用的自然语言处理库,提供了丰富的功能来处理文本数据,包括分词、词性标注、句法分析等。
安装和基本使用
首先,安装NLTK库:
pip install nltk
使用以下代码进行基本的文本处理:
import nltk
from nltk.tokenize import word_tokenize
from nltk.tag import pos_tag
def process_text(text):
tokens = word_tokenize(text)
tagged = pos_tag(tokens)
print(tagged)
nltk.download('punkt')
nltk.download('averaged_perceptron_tagger')
process_text("这是一个自然语言处理的示例。")
意图识别
为了实现语音助手的智能交互,需要对用户的意图进行识别。可以使用NLTK或其他自然语言处理库来实现意图识别。例如,通过预定义的规则或训练分类模型来识别用户意图:
def recognize_intent(text):
if "天气" in text:
return "查询天气"
elif "时间" in text:
return "查询时间"
else:
return "未知意图"
intent = recognize_intent("今天的天气怎么样?")
print("识别的意图是:", intent)
四、逻辑控制
处理用户指令
在识别用户意图后,可以根据不同的意图执行相应的操作。例如,如果用户查询天气,可以调用天气API获取当前天气信息:
import requests
def get_weather():
api_key = "YOUR_API_KEY"
location = "Shanghai"
url = f"http://api.weatherapi.com/v1/current.json?key={api_key}&q={location}&lang=zh"
response = requests.get(url)
weather_data = response.json()
weather = weather_data['current']['condition']['text']
return f"当前天气是:{weather}"
def handle_intent(intent):
if intent == "查询天气":
weather_info = get_weather()
text_to_speech(weather_info)
elif intent == "查询时间":
import datetime
now = datetime.datetime.now()
current_time = now.strftime("%H:%M:%S")
text_to_speech(f"当前时间是:{current_time}")
else:
text_to_speech("对不起,我无法识别你的指令。")
user_intent = recognize_intent("今天的天气怎么样?")
handle_intent(user_intent)
增加更多功能
可以根据需求添加更多功能,例如:
- 设置闹钟:实现设置和提醒闹钟的功能。
- 播放音乐:集成音乐播放功能,支持在线和本地音乐。
- 智能家居控制:与智能家居设备集成,实现语音控制家居设备。
五、综合实现
整合所有模块
将前面介绍的各个模块整合在一起,形成一个完整的语音助手系统:
import speech_recognition as sr
import pyttsx3
import requests
import datetime
def recognize_speech_from_mic():
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("请说话:")
audio = recognizer.listen(source)
try:
text = recognizer.recognize_google(audio, language="zh-CN")
print("你说的是: " + text)
except sr.UnknownValueError:
print("无法识别音频")
except sr.RequestError as e:
print("请求错误; {0}".format(e))
return text
def text_to_speech(text):
engine = pyttsx3.init()
engine.setProperty('rate', 150)
engine.setProperty('volume', 0.9)
voices = engine.getProperty('voices')
engine.setProperty('voice', voices[1].id)
engine.say(text)
engine.runAndWait()
def recognize_intent(text):
if "天气" in text:
return "查询天气"
elif "时间" in text:
return "查询时间"
else:
return "未知意图"
def get_weather():
api_key = "YOUR_API_KEY"
location = "Shanghai"
url = f"http://api.weatherapi.com/v1/current.json?key={api_key}&q={location}&lang=zh"
response = requests.get(url)
weather_data = response.json()
weather = weather_data['current']['condition']['text']
return f"当前天气是:{weather}"
def handle_intent(intent):
if intent == "查询天气":
weather_info = get_weather()
text_to_speech(weather_info)
elif intent == "查询时间":
now = datetime.datetime.now()
current_time = now.strftime("%H:%M:%S")
text_to_speech(f"当前时间是:{current_time}")
else:
text_to_speech("对不起,我无法识别你的指令。")
def main():
while True:
recognized_text = recognize_speech_from_mic()
user_intent = recognize_intent(recognized_text)
handle_intent(user_intent)
if __name__ == "__main__":
main()
进一步优化
- 错误处理:增加更多的错误处理逻辑,提高系统的鲁棒性。
- 用户交互:增加用户确认和反馈机制,提高交互体验。
- 扩展功能:不断扩展语音助手的功能,例如增加日程管理、信息查询、娱乐等功能。
六、总结
通过使用Python开发语音助手,我们可以实现语音识别、文本到语音转换、自然语言处理和逻辑控制等功能。本文详细介绍了如何使用SpeechRecognition、pyttsx3和NLTK等库来实现这些功能,并提供了完整的代码示例。通过不断优化和扩展,可以开发出功能强大、用户体验良好的语音助手系统。
希望本文能够帮助你了解如何用Python开发语音助手,并激发你进一步探索和实现更多有趣的功能。如果你有任何问题或建议,欢迎随时交流。
相关问答FAQs:
如何选择适合的语音识别库来开发Python语音助手?
在开发语音助手时,选择一个合适的语音识别库至关重要。常见的库包括SpeechRecognition、Google Cloud Speech API和PocketSphinx等。SpeechRecognition库易于使用,支持多种语音引擎,适合初学者。而Google Cloud Speech API提供高准确率的识别效果,但需要联网使用并可能产生费用。PocketSphinx则是一个离线语音识别工具,适合对网络连接要求较高的应用场景。选择时可以根据项目需求和资源来决定。
如何实现语音助手的自然语言处理功能?
自然语言处理(NLP)是语音助手的重要组成部分。可以使用NLTK、spaCy或Transformers等库来实现NLP功能。这些库提供了丰富的工具,能帮助解析用户输入,理解意图,并生成自然的响应。通过训练模型或使用预训练模型,可以提升语音助手的理解能力,让其更好地处理复杂的用户请求。
在开发Python语音助手时,如何保证用户数据的安全性?
用户数据的安全性是开发语音助手时必须重视的问题。可以通过加密用户数据,确保传输过程中的安全性。此外,遵循隐私政策和法律法规,确保用户同意数据收集和使用。在设计时,尽量减少对用户敏感信息的存储,使用匿名化处理,降低数据泄露的风险。确保开发的语音助手具有良好的安全性和用户信任度。
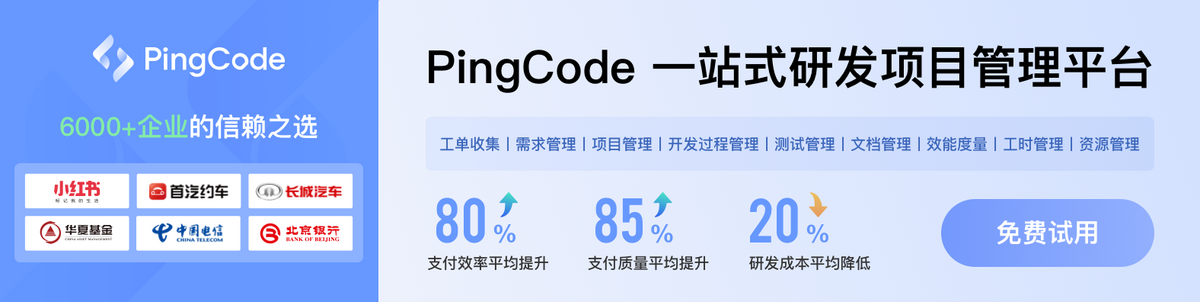