Python解析网页源码的方法包括:使用requests库获取网页源码、使用BeautifulSoup库解析HTML、使用lxml库解析XML、使用Selenium模拟浏览器操作。其中,使用requests库获取网页源码和使用BeautifulSoup库解析HTML是最常见的方式。下面详细描述如何使用requests和BeautifulSoup库来解析网页源码。
使用requests库获取网页源码
requests库是一个简单易用的HTTP库,用于发送HTTP请求并获取响应数据。使用requests库获取网页源码的步骤如下:
- 安装requests库:可以使用pip命令安装requests库。
pip install requests
- 导入requests库并发送HTTP请求:使用requests.get()方法发送HTTP请求,并获取响应对象。
import requests
url = 'http://example.com'
response = requests.get(url)
- 获取网页源码:通过响应对象的text属性获取网页源码。
html_content = response.text
使用BeautifulSoup库解析HTML
BeautifulSoup库是一个用于解析HTML和XML文档的库,它提供了一些简单的方法来导航、搜索和修改解析树。使用BeautifulSoup库解析HTML的步骤如下:
- 安装BeautifulSoup库:可以使用pip命令安装BeautifulSoup库。
pip install beautifulsoup4
- 导入BeautifulSoup库并解析HTML:使用BeautifulSoup类解析HTML文档。
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
- 查找元素:使用BeautifulSoup提供的各种方法查找HTML文档中的元素。
# 查找所有的链接
links = soup.find_all('a')
查找第一个标题
first_title = soup.find('h1')
通过requests库和BeautifulSoup库的结合,可以轻松地获取并解析网页源码。下面将更详细地介绍Python解析网页源码的其他方法。
一、使用requests库获取网页源码
1. 安装requests库
使用pip命令安装requests库:
pip install requests
2. 发送HTTP请求
导入requests库并使用requests.get()方法发送HTTP请求,获取响应对象:
import requests
url = 'http://example.com'
response = requests.get(url)
3. 获取网页源码
通过响应对象的text属性获取网页源码:
html_content = response.text
print(html_content)
4. 处理响应状态码
在发送HTTP请求后,检查响应状态码以确保请求成功:
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print(f"Failed to retrieve content: {response.status_code}")
二、使用BeautifulSoup库解析HTML
1. 安装BeautifulSoup库
使用pip命令安装BeautifulSoup库:
pip install beautifulsoup4
2. 导入BeautifulSoup库并解析HTML
导入BeautifulSoup库并使用BeautifulSoup类解析HTML文档:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
3. 查找元素
使用BeautifulSoup提供的各种方法查找HTML文档中的元素:
# 查找所有的链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
查找第一个标题
first_title = soup.find('h1')
print(first_title.text)
4. 查找具有特定属性的元素
使用BeautifulSoup查找具有特定属性的元素:
# 查找具有特定类名的元素
special_elements = soup.find_all(class_='special')
for element in special_elements:
print(element.text)
查找具有特定ID的元素
unique_element = soup.find(id='unique')
print(unique_element.text)
三、使用lxml库解析XML
1. 安装lxml库
使用pip命令安装lxml库:
pip install lxml
2. 导入lxml库并解析XML
导入lxml库并使用etree模块解析XML文档:
from lxml import etree
xml_content = '''<root>
<child id="1">Child 1</child>
<child id="2">Child 2</child>
</root>'''
root = etree.fromstring(xml_content)
3. 查找元素
使用lxml库查找XML文档中的元素:
# 查找所有的子元素
children = root.findall('child')
for child in children:
print(child.text)
查找具有特定属性的元素
child_with_id_2 = root.find('child[@id="2"]')
print(child_with_id_2.text)
四、使用Selenium模拟浏览器操作
1. 安装Selenium库
使用pip命令安装Selenium库:
pip install selenium
2. 安装浏览器驱动
下载并安装适用于所使用浏览器的驱动程序(如ChromeDriver、GeckoDriver等)。
3. 导入Selenium库并模拟浏览器操作
导入Selenium库并使用WebDriver类模拟浏览器操作:
from selenium import webdriver
创建WebDriver对象(以Chrome为例)
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
打开网页
driver.get('http://example.com')
获取网页源码
html_content = driver.page_source
print(html_content)
关闭浏览器
driver.quit()
4. 查找元素
使用Selenium查找网页中的元素:
# 查找所有的链接
links = driver.find_elements_by_tag_name('a')
for link in links:
print(link.get_attribute('href'))
查找第一个标题
first_title = driver.find_element_by_tag_name('h1')
print(first_title.text)
五、结合使用requests和BeautifulSoup进行网页解析
通过结合使用requests库和BeautifulSoup库,可以高效地获取并解析网页源码。以下是一个完整的示例:
import requests
from bs4 import BeautifulSoup
发送HTTP请求并获取网页源码
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
# 解析HTML文档
soup = BeautifulSoup(html_content, 'html.parser')
# 查找所有的链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
# 查找第一个标题
first_title = soup.find('h1')
if first_title:
print(first_title.text)
else:
print(f"Failed to retrieve content: {response.status_code}")
六、处理动态加载的内容
有些网页使用JavaScript动态加载内容,在这种情况下,使用requests库可能无法获取到完整的网页源码。可以使用Selenium模拟浏览器操作来处理动态加载的内容。以下是一个示例:
from selenium import webdriver
from bs4 import BeautifulSoup
创建WebDriver对象(以Chrome为例)
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
打开网页
driver.get('http://example.com')
等待页面加载完成
driver.implicitly_wait(10)
获取网页源码
html_content = driver.page_source
解析HTML文档
soup = BeautifulSoup(html_content, 'html.parser')
查找所有的链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
查找第一个标题
first_title = soup.find('h1')
if first_title:
print(first_title.text)
关闭浏览器
driver.quit()
七、处理反爬虫机制
有些网站会使用各种反爬虫机制来阻止自动化程序访问其内容。以下是一些处理反爬虫机制的常用技巧:
1. 设置请求头
通过设置HTTP请求头,可以伪装成真实的浏览器请求,避免被反爬虫机制检测到:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36'
}
response = requests.get(url, headers=headers)
2. 使用代理
使用代理服务器可以隐藏真实的IP地址,避免被反爬虫机制封锁:
proxies = {
'http': 'http://proxy.example.com:8080',
'https': 'http://proxy.example.com:8080'
}
response = requests.get(url, headers=headers, proxies=proxies)
3. 添加延时
添加延时可以模拟人类的浏览行为,避免被反爬虫机制检测到:
import time
time.sleep(2) # 延时2秒
response = requests.get(url, headers=headers)
八、处理表单提交和登录
有些网站需要提交表单或进行登录操作,才能访问特定内容。可以使用requests库或Selenium来处理表单提交和登录。
1. 使用requests库处理表单提交
以下是一个使用requests库处理表单提交的示例:
import requests
url = 'http://example.com/login'
data = {
'username': 'your_username',
'password': 'your_password'
}
response = requests.post(url, data=data)
if response.status_code == 200:
print("Login successful")
else:
print("Login failed")
2. 使用Selenium处理表单提交和登录
以下是一个使用Selenium处理表单提交和登录的示例:
from selenium import webdriver
创建WebDriver对象(以Chrome为例)
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
打开登录页面
driver.get('http://example.com/login')
输入用户名和密码
username_input = driver.find_element_by_name('username')
password_input = driver.find_element_by_name('password')
username_input.send_keys('your_username')
password_input.send_keys('your_password')
提交表单
login_button = driver.find_element_by_name('login')
login_button.click()
等待页面加载完成
driver.implicitly_wait(10)
获取登录后的网页源码
html_content = driver.page_source
print(html_content)
关闭浏览器
driver.quit()
九、处理复杂的网页结构
有些网页的结构比较复杂,可能包含嵌套的元素或多层级的导航。在这种情况下,可以使用BeautifulSoup库提供的各种方法来处理复杂的网页结构。
1. 处理嵌套元素
以下是一个处理嵌套元素的示例:
from bs4 import BeautifulSoup
html_content = '''
<div class="container">
<div class="header">
<h1>Title</h1>
</div>
<div class="content">
<p>Paragraph 1</p>
<p>Paragraph 2</p>
</div>
</div>
'''
soup = BeautifulSoup(html_content, 'html.parser')
查找容器元素
container = soup.find(class_='container')
查找嵌套的标题
title = container.find(class_='header').find('h1')
print(title.text)
查找嵌套的段落
paragraphs = container.find(class_='content').find_all('p')
for paragraph in paragraphs:
print(paragraph.text)
2. 处理多层级导航
以下是一个处理多层级导航的示例:
from bs4 import BeautifulSoup
html_content = '''
<ul class="nav">
<li><a href="/home">Home</a></li>
<li>
<a href="/services">Services</a>
<ul class="sub-nav">
<li><a href="/services/consulting">Consulting</a></li>
<li><a href="/services/support">Support</a></li>
</ul>
</li>
<li><a href="/contact">Contact</a></li>
</ul>
'''
soup = BeautifulSoup(html_content, 'html.parser')
查找导航元素
nav = soup.find(class_='nav')
查找一级菜单项
main_items = nav.find_all('li', recursive=False)
for item in main_items:
link = item.find('a')
print(link.text, link.get('href'))
# 查找二级菜单项
sub_nav = item.find(class_='sub-nav')
if sub_nav:
sub_items = sub_nav.find_all('li')
for sub_item in sub_items:
sub_link = sub_item.find('a')
print(' ', sub_link.text, sub_link.get('href'))
十、总结
通过使用Python的requests库、BeautifulSoup库、lxml库和Selenium库,可以高效地解析网页源码。根据具体需求选择合适的库和方法,处理静态和动态加载的内容、表单提交和登录、复杂的网页结构以及反爬虫机制。掌握这些技巧,可以帮助你在网页数据抓取和解析方面更加得心应手。
相关问答FAQs:
如何使用Python解析网页源码?
Python提供了多种库来解析网页源码,其中最常用的包括Beautiful Soup和lxml。通过这些库,可以轻松地提取网页中的特定内容,例如标题、文本或链接。首先,需要安装相应的库,例如使用pip命令:pip install beautifulsoup4
和pip install lxml
。然后,可以使用requests库获取网页源码,并利用Beautiful Soup进行解析。
在解析网页时,如何处理动态加载的内容?
很多网站使用JavaScript动态加载内容,这使得直接获取网页源码时,无法看到所有数据。为了解决这个问题,可以使用Selenium库,它模拟浏览器行为,从而能够加载所有内容并提取所需信息。安装Selenium后,需要下载对应的浏览器驱动,并通过脚本控制浏览器进行数据抓取。
解析网页源码时,如何避免被网站封禁?
在进行网页解析时,频繁的请求可能会导致IP被封禁。为了降低被封禁的风险,可以采取一些措施,例如设置请求间隔时间、随机化请求头或使用代理服务器。使用Python的time库可以轻松设置请求间隔,而通过requests库可以设置自定义的请求头,从而模拟真实用户的行为,减少被封禁的可能性。
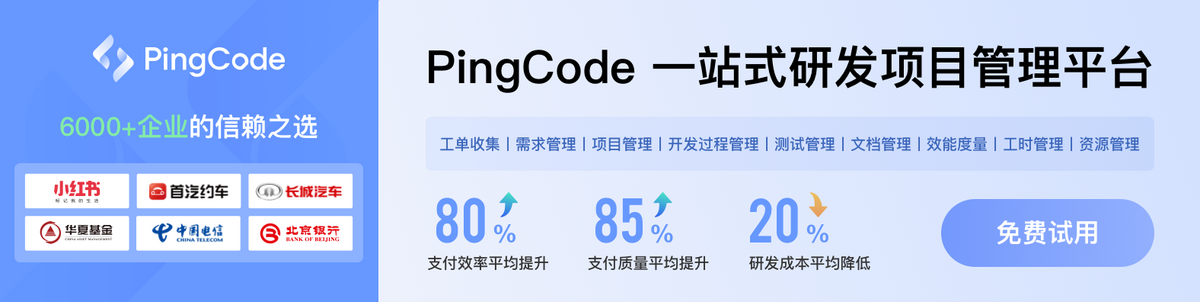