使用Python进行网页数据爬取时,常用的方法包括使用requests库进行HTTP请求、使用BeautifulSoup进行HTML解析、使用Selenium进行动态网页处理。 其中,requests库的使用最为广泛,因为它可以方便地发送HTTP请求并获取网页的HTML内容。下面我们将详细介绍如何使用requests和BeautifulSoup进行数据爬取。
一、请求网页数据
-
安装requests库
你可以使用pip来安装requests库,命令如下:
pip install requests
-
发送HTTP请求获取网页内容
使用requests库可以发送GET请求来获取网页的HTML内容:
import requests
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
在使用requests库发送HTTP请求时,需要注意处理可能的错误状态码(如404、500等)。 可以通过检查
response.status_code
来判断请求是否成功。
二、解析网页数据
-
安装BeautifulSoup库
BeautifulSoup是一个用于解析HTML和XML的库,可以轻松地提取网页中的特定内容。使用pip安装BeautifulSoup库和解析器lxml:
pip install beautifulsoup4 lxml
-
使用BeautifulSoup解析HTML内容
解析HTML内容并提取所需数据:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'lxml')
示例:提取网页中的所有链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
BeautifulSoup提供了多种方法来查找和提取HTML元素,如
find
、find_all
、select
等,可以根据标签名、属性、CSS选择器等条件进行查找。
三、处理动态网页
-
安装Selenium库
对于需要处理动态内容(如由JavaScript生成的内容)的网页,可以使用Selenium库。安装Selenium和浏览器驱动(如ChromeDriver):
pip install selenium
下载ChromeDriver,并将其路径添加到系统环境变量中
-
使用Selenium自动化浏览器操作
自动化浏览器操作并获取动态内容:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
options = webdriver.ChromeOptions()
options.add_argument('--headless') # 无头模式
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=options)
url = 'https://example.com'
driver.get(url)
获取动态加载的内容
dynamic_content = driver.page_source
print(dynamic_content)
driver.quit()
Selenium可以自动化浏览器操作,包括点击、填写表单、滚动等,因此特别适合处理需要用户交互或动态加载内容的网页。
四、存储和处理数据
-
存储数据
将提取到的数据存储到文件或数据库中。可以使用Python的内置文件操作函数来存储到文本文件或CSV文件:
import csv
data = [['Name', 'Link'], ['Example', 'https://example.com']]
with open('output.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
对于更复杂的数据,可以使用pandas库将数据存储到Excel文件或数据库中:
import pandas as pd
df = pd.DataFrame(data, columns=['Name', 'Link'])
df.to_excel('output.xlsx', index=False)
-
数据清洗和处理
数据爬取后,可能需要进行清洗和处理。可以使用pandas库进行数据处理:
df = pd.read_csv('output.csv')
示例:删除重复行
df.drop_duplicates(inplace=True)
示例:根据某列的值进行筛选
filtered_df = df[df['Name'].str.contains('Example')]
print(filtered_df)
数据清洗和处理是数据分析的重要步骤,可以根据具体需求对数据进行各种操作,如删除空值、数据格式转换、数据分组等。
五、常见问题和解决方案
-
反爬虫机制
许多网站有反爬虫机制,会检测并阻止频繁的自动请求。常见的反爬虫机制包括IP封禁、验证码、请求速率限制等。以下是一些常见的应对方法:
- 设置请求头:模拟浏览器请求,避免被识别为爬虫:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
- 使用代理IP:通过代理IP轮换请求,避免IP被封禁:
proxies = {
'http': 'http://proxy_ip:proxy_port',
'https': 'http://proxy_ip:proxy_port'
}
response = requests.get(url, headers=headers, proxies=proxies)
- 设置请求间隔:在每次请求之间设置随机间隔,避免频繁请求:
import time
import random
time.sleep(random.uniform(1, 3))
- 设置请求头:模拟浏览器请求,避免被识别为爬虫:
-
处理复杂的HTML结构
有时网页的HTML结构复杂,使用BeautifulSoup查找元素可能会遇到困难。可以使用CSS选择器或XPath进行更精确的查找:
# 使用CSS选择器
elements = soup.select('div.classname > ul > li')
使用XPath
from lxml import etree
tree = etree.HTML(html_content)
elements = tree.xpath('//div[@class="classname"]/ul/li')
-
处理分页数据
有些网页的数据分布在多个分页,需要遍历所有分页进行数据爬取。可以通过循环请求每个分页的URL来获取所有数据:
page_num = 1
while True:
paginated_url = f'https://example.com/page/{page_num}'
response = requests.get(paginated_url)
if response.status_code != 200:
break
# 处理分页数据
html_content = response.text
# ... 解析和提取数据
page_num += 1
六、使用Scrapy框架
Scrapy是一个强大的Python爬虫框架,适用于大规模的数据爬取任务。它提供了丰富的功能,如自动处理请求、数据管道、并发爬取等。
-
安装Scrapy
使用pip安装Scrapy:
pip install scrapy
-
创建Scrapy项目
创建一个新的Scrapy项目:
scrapy startproject myproject
-
定义爬虫
在项目目录中创建一个新的爬虫:
cd myproject
scrapy genspider myspider example.com
-
编写爬虫代码
编辑生成的爬虫文件
myspider.py
,编写爬虫逻辑:import scrapy
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['https://example.com']
def parse(self, response):
# 示例:提取网页中的所有链接
for link in response.css('a::attr(href)').getall():
yield {'link': link}
-
运行爬虫
在项目目录中运行爬虫:
scrapy crawl myspider -o output.json
Scrapy提供了强大的数据管道,可以将数据存储到多种格式(如JSON、CSV、数据库)中,还可以对数据进行清洗和处理。
七、处理JavaScript加载的内容
对于需要执行JavaScript才能获取到数据的网页,可以使用Selenium或Splash。前文已经介绍了Selenium的使用,下面简要介绍使用Splash的方法。
-
安装Splash
Splash是一个JavaScript渲染服务,可以通过Docker进行安装:
docker run -p 8050:8050 scrapinghub/splash
-
使用Scrapy-Splash进行爬取
安装Scrapy-Splash扩展:
pip install scrapy-splash
-
配置Scrapy-Splash
在Scrapy项目的
settings.py
中添加Scrapy-Splash的配置:SPLASH_URL = 'http://localhost:8050'
DOWNLOADER_MIDDLEWARES = {
'scrapy_splash.SplashCookiesMiddleware': 723,
'scrapy_splash.SplashMiddleware': 725,
'scrapy.downloadermiddlewares.httpcompression.HttpCompressionMiddleware': 810,
}
SPIDER_MIDDLEWARES = {
'scrapy_splash.SplashDeduplicateArgsMiddleware': 100,
}
DUPEFILTER_CLASS = 'scrapy_splash.SplashAwareDupeFilter'
-
使用SplashRequest进行请求
在爬虫代码中使用SplashRequest来处理JavaScript加载的内容:
import scrapy
from scrapy_splash import SplashRequest
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['https://example.com']
def start_requests(self):
for url in self.start_urls:
yield SplashRequest(url, self.parse, args={'wait': 2})
def parse(self, response):
# 示例:提取网页中的所有链接
for link in response.css('a::attr(href)').getall():
yield {'link': link}
八、实际应用案例
-
爬取新闻网站的文章
以爬取某新闻网站的文章标题和链接为例,完整的代码如下:
import requests
from bs4 import BeautifulSoup
import csv
url = 'https://news.ycombinator.com/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
articles = []
for item in soup.find_all('a', class_='storylink'):
title = item.get_text()
link = item.get('href')
articles.append([title, link])
with open('articles.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Title', 'Link'])
writer.writerows(articles)
-
爬取电商网站的产品信息
以爬取某电商网站的产品名称和价格为例,完整的代码如下:
import requests
from bs4 import BeautifulSoup
import csv
url = 'https://example-ecommerce.com/products'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
products = []
for item in soup.find_all('div', class_='product-item'):
name = item.find('h2').get_text()
price = item.find('span', class_='price').get_text()
products.append([name, price])
with open('products.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Price'])
writer.writerows(products)
九、总结
使用Python进行网页数据爬取是一项非常有用的技能,requests库和BeautifulSoup是处理静态网页数据爬取的常用工具,而Selenium和Scrapy-Splash则适用于处理动态网页内容。在实际应用中,可能会遇到反爬虫机制、复杂HTML结构、分页数据等问题,需要根据具体情况采用相应的解决方法。通过以上方法和案例的介绍,相信你已经掌握了如何使用Python进行网页数据爬取的基本技能。
相关问答FAQs:
如何选择合适的Python库进行网页爬虫?
在进行网页数据爬取时,选择合适的Python库至关重要。常用的库包括Requests和BeautifulSoup,它们分别用于发送HTTP请求和解析HTML文档。Requests库使得网络请求变得简单,而BeautifulSoup则提供了便捷的工具来查找和提取网页中的数据。此外,Scrapy是一个功能强大的框架,适合进行复杂的爬虫项目。根据你的需求,选择相应的工具可以提高开发效率。
在爬取数据时,如何处理反爬虫机制?
许多网站会使用反爬虫机制来阻止自动化数据抓取。为了有效应对这些机制,可以采取多种策略,如使用随机的User-Agent字符串,模拟真实用户的行为,避免过于频繁的请求。此外,使用代理服务器可以隐藏真实IP地址,降低被封禁的风险。设置请求间隔时间和引入随机延迟也是有效的防止被识别为爬虫的手段。
爬取数据后,如何进行数据存储和处理?
在成功爬取数据后,存储和处理数据是接下来的重要步骤。可以将数据存储在CSV文件、JSON格式或数据库中,如SQLite或MongoDB,具体选择取决于数据的规模和后续使用需求。对于数据处理,可以使用Pandas库进行清洗和分析,帮助你从原始数据中提取有价值的信息。此外,数据可视化工具如Matplotlib和Seaborn可以帮助你直观展示数据分析结果。
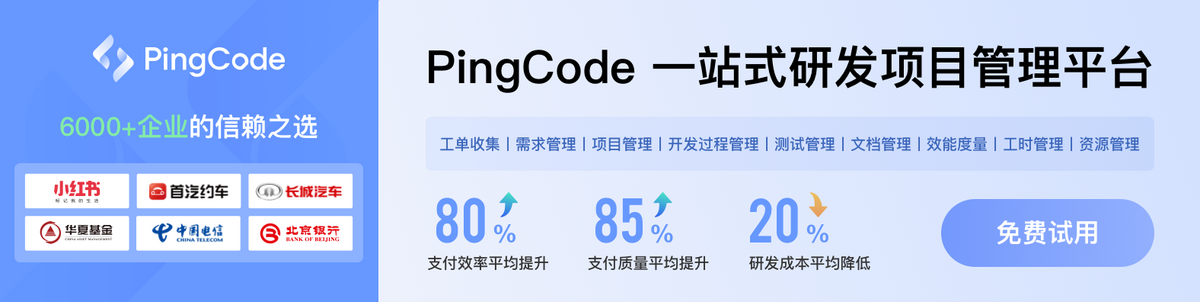