在Python中,你可以使用不同的方法来指定文件的下载位置。一种常见的方法是使用requests
库进行HTTP请求、结合文件操作来保存文件,另一种方法是使用urllib
库。
下面我将详细描述如何使用requests
库指定下载位置。
使用requests库下载文件并指定保存位置:
import requests
def download_file(url, save_path):
response = requests.get(url, stream=True)
with open(save_path, 'wb') as file:
for chunk in response.iter_content(chunk_size=8192):
file.write(chunk)
print(f"File downloaded and saved to {save_path}")
示例用法
url = "https://example.com/file.zip"
save_path = "/path/to/your/directory/file.zip"
download_file(url, save_path)
在这个例子中,我们使用requests.get()
来发送HTTP GET请求,并将响应内容以流的方式迭代写入到指定路径的文件中。save_path
参数就是指定下载保存的文件路径。
接下来我们将详细探讨其他相关的内容,包括如何在不同操作系统上指定路径、使用urllib
库下载文件、处理下载错误,以及如何创建目录结构。
一、指定路径的基本方法
1.1 使用绝对路径和相对路径
在Python中,可以使用绝对路径或相对路径来指定文件保存位置。
绝对路径:
绝对路径是指从根目录开始的完整路径。例如:
save_path = "/Users/username/Downloads/file.zip"
相对路径:
相对路径是相对于当前工作目录的路径。例如:
save_path = "downloads/file.zip"
在使用相对路径时,需要确保当前工作目录存在指定的相对路径。如果路径不存在,可以使用os.makedirs()
来创建目录。
1.2 使用os.path和pathlib模块
Python的os.path
模块和pathlib
模块提供了处理文件路径的便捷方法。
使用os.path模块:
import os
directory = "downloads"
filename = "file.zip"
save_path = os.path.join(directory, filename)
确保目录存在
if not os.path.exists(directory):
os.makedirs(directory)
print(f"File will be saved to: {save_path}")
使用pathlib模块:
from pathlib import Path
directory = Path("downloads")
filename = "file.zip"
save_path = directory / filename
确保目录存在
directory.mkdir(parents=True, exist_ok=True)
print(f"File will be saved to: {save_path}")
二、使用urllib库下载文件
除了requests
库外,Python的urllib
库也可以用于下载文件。以下是一个示例:
import urllib.request
def download_file(url, save_path):
urllib.request.urlretrieve(url, save_path)
print(f"File downloaded and saved to {save_path}")
示例用法
url = "https://example.com/file.zip"
save_path = "/path/to/your/directory/file.zip"
download_file(url, save_path)
在这个例子中,urllib.request.urlretrieve()
函数将URL内容下载并保存到指定路径。
三、处理下载错误
在下载文件的过程中,可能会遇到各种错误,例如网络问题、文件不存在等。我们可以使用try-except
块来处理这些错误。
import requests
def download_file(url, save_path):
try:
response = requests.get(url, stream=True)
response.raise_for_status() # 检查请求是否成功
with open(save_path, 'wb') as file:
for chunk in response.iter_content(chunk_size=8192):
file.write(chunk)
print(f"File downloaded and saved to {save_path}")
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"An error occurred: {err}")
示例用法
url = "https://example.com/file.zip"
save_path = "/path/to/your/directory/file.zip"
download_file(url, save_path)
在这个例子中,我们使用response.raise_for_status()
来检查HTTP请求是否成功,并使用try-except
块捕获并处理可能的错误。
四、创建目录结构
在下载文件时,有时需要确保目标目录存在。如果目录不存在,可以使用os.makedirs()
或Path.mkdir()
创建目录。
4.1 使用os.makedirs()创建目录
import os
directory = "/path/to/your/directory"
if not os.path.exists(directory):
os.makedirs(directory)
print(f"Directory created: {directory}")
4.2 使用Path.mkdir()创建目录
from pathlib import Path
directory = Path("/path/to/your/directory")
directory.mkdir(parents=True, exist_ok=True)
print(f"Directory created: {directory}")
五、下载进度显示
在下载大文件时,显示下载进度可以提高用户体验。我们可以使用tqdm
库来显示下载进度。
首先安装tqdm
库:
pip install tqdm
然后修改下载代码以显示进度:
import requests
from tqdm import tqdm
def download_file(url, save_path):
response = requests.get(url, stream=True)
total_size = int(response.headers.get('content-length', 0))
with open(save_path, 'wb') as file, tqdm(
desc=save_path,
total=total_size,
unit='B',
unit_scale=True,
unit_divisor=1024,
) as bar:
for chunk in response.iter_content(chunk_size=8192):
file.write(chunk)
bar.update(len(chunk))
print(f"File downloaded and saved to {save_path}")
示例用法
url = "https://example.com/file.zip"
save_path = "/path/to/your/directory/file.zip"
download_file(url, save_path)
在这个例子中,我们使用tqdm
来显示下载进度条。
六、总结
通过上述方法,您可以在Python中指定文件的下载位置,并处理下载过程中的各种问题。核心步骤包括使用requests
或urllib
库下载文件、指定保存路径、处理下载错误、创建必要的目录结构以及显示下载进度。这些技巧可以帮助您在开发中更高效地处理文件下载任务。
继续深入了解相关库和方法,可以帮助您在实际项目中更灵活地应用这些知识。希望本文对您有所帮助!
相关问答FAQs:
如何在Python中设置文件下载路径?
在Python中,您可以通过指定文件路径来设置下载位置。使用requests
库下载文件时,可以在保存文件时指定完整的路径。例如,with open('/path/to/directory/filename', 'wb') as f:
可以将文件保存到特定目录下。
使用Python下载文件时,如何确保文件名不冲突?
为避免文件名冲突,可以在保存文件前检查文件是否已存在。使用os.path.exists()
方法可以判断文件是否存在,如果存在,可以为文件名添加时间戳或序号,确保每次下载的文件具有唯一性。
在Python中,如何处理下载大文件的情况?
下载大文件时,建议使用流式下载的方式,以避免占用过多内存。可以使用requests.get(url, stream=True)
来实现流式下载,并逐块读取文件内容并写入磁盘,这样可以有效管理内存并提高下载效率。
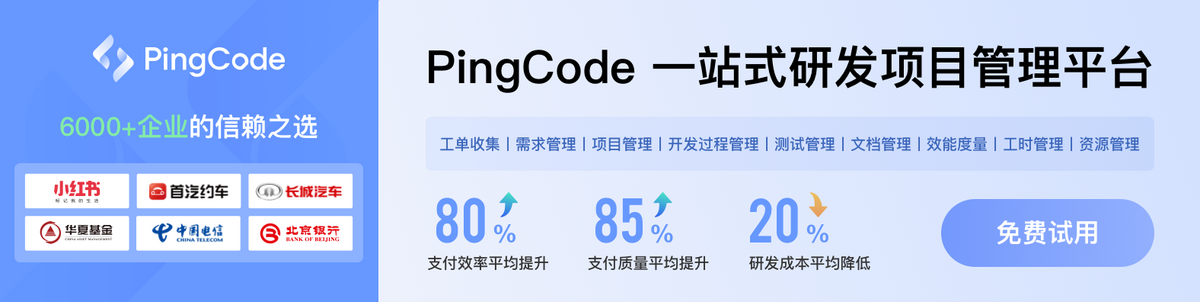