Python爬取整个读者的方法包括:使用requests库进行HTTP请求、使用BeautifulSoup解析HTML、处理翻页、处理反爬机制。 其中,处理反爬机制是爬取过程中最为复杂的一部分,因为网站通常会有多种措施来防止爬虫过度访问,例如IP封禁、验证码等。
处理反爬机制:为了应对网站的反爬机制,我们可以采取以下几种措施:
- 使用代理IP:通过代理IP的轮换,可以避免被服务器检测到频繁的请求来自同一IP,从而防止IP封禁。
- 设置请求头:通过设置合适的User-Agent等请求头信息,可以伪装成浏览器访问,避免被服务器识别为爬虫。
- 模拟登录:有些网站需要登录才能访问全部内容,可以通过模拟登录的方式获取必要的Cookies和Session信息。
- 请求间隔:设置合理的请求间隔,避免频繁请求给服务器带来压力,从而减少被封的概率。
一、使用requests库进行HTTP请求
使用requests库可以方便地发送HTTP请求,并获取响应内容。以下是一个简单的示例代码:
import requests
url = "http://example.com"
response = requests.get(url)
print(response.text)
在实际应用中,我们需要根据网站的具体情况,设置合适的请求头信息。例如:
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36"
}
response = requests.get(url, headers=headers)
二、使用BeautifulSoup解析HTML
获取到网页内容后,可以使用BeautifulSoup库进行HTML解析,从中提取所需的信息。以下是一个示例代码:
from bs4 import BeautifulSoup
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
提取标题
title = soup.find('title').text
print(title)
提取所有的段落文本
paragraphs = soup.find_all('p')
for p in paragraphs:
print(p.text)
三、处理翻页
对于需要翻页的情况,我们需要找到下一页的链接,并循环请求直到没有下一页为止。以下是一个示例代码:
base_url = "http://example.com/page/"
page_number = 1
while True:
url = f"{base_url}{page_number}"
response = requests.get(url, headers=headers)
if response.status_code != 200:
break
soup = BeautifulSoup(response.text, 'html.parser')
# 提取所需信息
# ...
# 检查是否存在下一页
next_page = soup.find('a', text='Next')
if not next_page:
break
page_number += 1
四、处理反爬机制
1、使用代理IP
通过使用代理IP,可以避免频繁请求导致IP被封禁。以下是一个示例代码:
proxies = {
"http": "http://10.10.1.10:3128",
"https": "http://10.10.1.10:1080",
}
response = requests.get(url, headers=headers, proxies=proxies)
我们可以使用代理池来轮换使用多个代理IP,例如:
import random
proxy_pool = [
"http://10.10.1.10:3128",
"http://10.10.1.11:3128",
"http://10.10.1.12:3128",
]
proxy = random.choice(proxy_pool)
proxies = {"http": proxy, "https": proxy}
response = requests.get(url, headers=headers, proxies=proxies)
2、设置请求头
设置合适的请求头信息,可以伪装成浏览器访问。例如:
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36",
"Referer": "http://example.com",
"Accept-Language": "en-US,en;q=0.9",
}
response = requests.get(url, headers=headers)
3、模拟登录
对于需要登录才能访问的内容,可以通过模拟登录的方式获取必要的Cookies和Session信息。以下是一个示例代码:
login_url = "http://example.com/login"
login_data = {
"username": "your_username",
"password": "your_password",
}
session = requests.Session()
response = session.post(login_url, data=login_data, headers=headers)
使用登录后的Session访问其他页面
response = session.get(url, headers=headers)
4、请求间隔
设置合理的请求间隔,避免频繁请求给服务器带来压力。例如:
import time
for page in range(1, 101):
url = f"http://example.com/page/{page}"
response = requests.get(url, headers=headers)
# 处理响应内容
# ...
time.sleep(2) # 等待2秒
五、完整示例代码
下面是一个完整的示例代码,演示如何爬取一个需要翻页的网站,并处理反爬机制:
import requests
from bs4 import BeautifulSoup
import random
import time
def get_proxies():
# 返回代理IP列表
return [
"http://10.10.1.10:3128",
"http://10.10.1.11:3128",
"http://10.10.1.12:3128",
]
def fetch_page(url, headers, proxies):
proxy = random.choice(proxies)
response = requests.get(url, headers=headers, proxies={"http": proxy, "https": proxy})
return response
def parse_page(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
# 提取所需信息
titles = soup.find_all('h2')
for title in titles:
print(title.text)
def main():
base_url = "http://example.com/page/"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36",
"Referer": "http://example.com",
"Accept-Language": "en-US,en;q=0.9",
}
proxies = get_proxies()
page_number = 1
while True:
url = f"{base_url}{page_number}"
response = fetch_page(url, headers, proxies)
if response.status_code != 200:
break
parse_page(response.text)
# 检查是否存在下一页
soup = BeautifulSoup(response.text, 'html.parser')
next_page = soup.find('a', text='Next')
if not next_page:
break
page_number += 1
time.sleep(2) # 等待2秒
if __name__ == "__main__":
main()
通过以上步骤和代码示例,我们可以使用Python爬取整个读者网站,并处理翻页和反爬机制。在实际应用中,我们需要根据目标网站的具体情况进行调整和优化,以确保爬虫的稳定性和效率。
相关问答FAQs:
如何使用Python爬取整个网站的内容?
要使用Python爬取整个网站的内容,通常可以使用库如BeautifulSoup和Requests来解析和提取HTML数据。首先,使用Requests获取页面的HTML代码,然后利用BeautifulSoup解析这些代码,提取所需的信息。为了爬取整个网站,您需要编写一个递归函数,以便访问网站内的所有链接。请注意遵守网站的robots.txt文件和相关法律法规。
爬取数据时需要注意哪些法律和道德问题?
在爬取网站数据时,了解和遵守相关法律法规非常重要。许多网站在其使用条款中禁止自动化爬取。同时,尊重网站的robots.txt文件中列出的规则,确保不对服务器造成过大负担。此外,使用爬取的数据时要考虑版权问题,避免侵犯他人的知识产权。
如何处理爬取过程中遇到的反爬虫机制?
许多网站使用反爬虫机制来阻止自动化访问。如果遇到这些机制,可以尝试更改User-Agent头信息,模拟浏览器的请求;使用代理IP来隐藏真实IP地址;或者通过设置请求间隔来模拟人工访问的行为。此外,使用selenium等工具,可以模拟用户在浏览器中的操作,从而绕过一些简单的反爬虫措施。
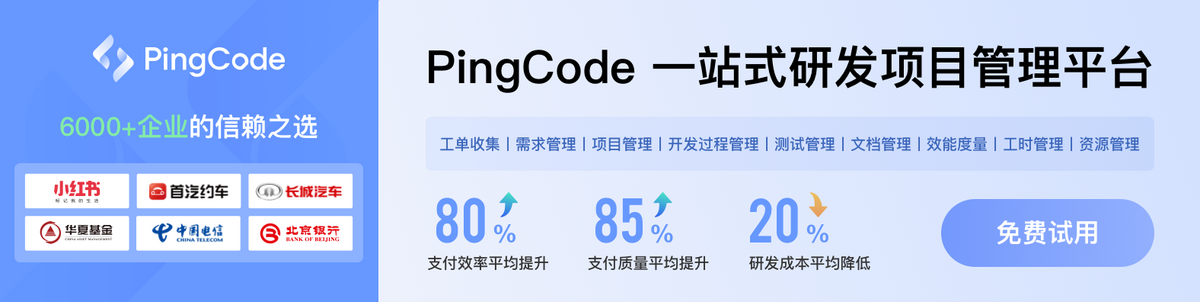