用Python做爬虫软件的方法有很多,主要通过requests库进行网页请求、BeautifulSoup库解析网页、Scrapy框架进行更复杂的爬取任务。其中,Scrapy框架是一个功能强大且灵活的爬虫框架,适合大型项目。以下是一个详细的介绍和示例,帮助你理解如何用Python做爬虫软件。
一、请求网页内容
在编写爬虫程序时,首先需要获取网页的内容。Python的requests
库是一个简单易用的HTTP库,适用于发送HTTP请求。
1、安装requests库
pip install requests
2、发送HTTP请求
import requests
url = 'http://example.com'
response = requests.get(url)
print(response.text)
通过以上代码,我们成功发送了一个HTTP GET请求,并打印了网页的HTML内容。
二、解析网页内容
获取到网页内容后,需要解析HTML以提取我们需要的数据。BeautifulSoup
库是一个用于解析HTML和XML的库。
1、安装BeautifulSoup库
pip install beautifulsoup4
2、解析HTML内容
from bs4 import BeautifulSoup
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
提取标题
title = soup.title.string
print(title)
提取所有链接
for link in soup.find_all('a'):
print(link.get('href'))
通过以上代码,我们使用BeautifulSoup解析了HTML内容,并提取了网页标题和所有链接。
三、Scrapy框架
对于更复杂的爬取任务,建议使用Scrapy框架。Scrapy是一个强大且灵活的爬虫框架,适用于抓取网站并提取结构化数据。
1、安装Scrapy
pip install scrapy
2、创建Scrapy项目
scrapy startproject myproject
3、定义Item
在Scrapy项目中,首先需要定义要抓取的数据结构。可以在items.py
文件中定义Item。
import scrapy
class MyprojectItem(scrapy.Item):
title = scrapy.Field()
link = scrapy.Field()
4、编写爬虫
在spiders
目录下创建一个新的爬虫文件,例如example_spider.py
。
import scrapy
from myproject.items import MyprojectItem
class ExampleSpider(scrapy.Spider):
name = "example"
start_urls = ['http://example.com']
def parse(self, response):
for href in response.css('a::attr(href)').extract():
yield response.follow(href, self.parse_link)
def parse_link(self, response):
item = MyprojectItem()
item['title'] = response.css('title::text').get()
item['link'] = response.url
yield item
5、运行爬虫
scrapy crawl example
通过以上步骤,我们创建了一个简单的Scrapy爬虫,抓取网页标题和链接。
四、处理反爬机制
许多网站会有反爬虫机制,防止频繁的请求。这时候我们可以使用一些策略来绕过这些机制。
1、使用User-Agent
改变请求头中的User-Agent,可以模仿不同的浏览器。
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
2、设置代理IP
使用代理IP,可以避免被目标网站封禁。
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get(url, proxies=proxies)
3、随机等待
在请求之间加入随机等待时间,模拟正常用户的访问行为。
import time
import random
time.sleep(random.uniform(1, 3)) # 随机等待1到3秒
五、存储抓取的数据
抓取的数据可以存储到本地文件、数据库等。这里以存储到CSV文件为例。
1、使用CSV模块
import csv
data = [
{'title': 'Example Title 1', 'link': 'http://example.com/1'},
{'title': 'Example Title 2', 'link': 'http://example.com/2'}
]
with open('output.csv', 'w', newline='', encoding='utf-8') as csvfile:
fieldnames = ['title', 'link']
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for row in data:
writer.writerow(row)
2、使用Pandas库
Pandas库提供了更为方便的方式来处理和存储数据。
import pandas as pd
data = [
{'title': 'Example Title 1', 'link': 'http://example.com/1'},
{'title': 'Example Title 2', 'link': 'http://example.com/2'}
]
df = pd.DataFrame(data)
df.to_csv('output.csv', index=False, encoding='utf-8')
六、常见问题和解决方法
1、处理JavaScript生成的内容
有些网站的内容是通过JavaScript生成的,requests库无法获取。此时可以使用Selenium库。
pip install selenium
from selenium import webdriver
url = 'http://example.com'
driver = webdriver.Chrome() # 需要安装ChromeDriver
driver.get(url)
html_content = driver.page_source
driver.quit()
解析HTML内容
soup = BeautifulSoup(html_content, 'html.parser')
2、处理验证码
遇到验证码时,可以使用第三方打码平台,也可以尝试手动识别或绕过。
七、优化和扩展
1、提高爬取效率
通过多线程或异步方式可以显著提高爬取效率。例如,使用concurrent.futures
库。
import concurrent.futures
def fetch(url):
response = requests.get(url)
return response.text
urls = ['http://example.com/page1', 'http://example.com/page2']
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor:
results = executor.map(fetch, urls)
for result in results:
print(result)
2、错误处理和重试机制
在爬取过程中可能会遇到各种错误,可以使用重试机制来提高爬取的稳定性。
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.util.retry import Retry
session = requests.Session()
retry = Retry(total=5, backoff_factor=1, status_forcelist=[500, 502, 503, 504])
adapter = HTTPAdapter(max_retries=retry)
session.mount('http://', adapter)
session.mount('https://', adapter)
response = session.get(url)
八、使用Scrapy进行高级爬取
Scrapy不仅能处理简单的爬取任务,还能进行高级爬取,如处理登录、表单提交等。
1、处理登录
有些网站需要登录才能访问。可以通过模拟登录来获取登录后的内容。
import scrapy
class LoginSpider(scrapy.Spider):
name = 'login'
start_urls = ['http://example.com/login']
def parse(self, response):
return scrapy.FormRequest.from_response(
response,
formdata={'username': 'user', 'password': 'pass'},
callback=self.after_login
)
def after_login(self, response):
# 检查登录是否成功
if "Welcome" in response.text:
self.log("Login successful")
else:
self.log("Login failed")
2、处理表单提交
通过模拟表单提交,可以获取表单提交后的内容。
class FormSpider(scrapy.Spider):
name = 'form'
start_urls = ['http://example.com/form']
def parse(self, response):
return scrapy.FormRequest.from_response(
response,
formdata={'field1': 'value1', 'field2': 'value2'},
callback=self.after_submit
)
def after_submit(self, response):
self.log("Form submitted")
self.log(response.text)
九、Scrapy中间件
Scrapy中间件是处理请求和响应的组件,可以用于修改请求头、处理代理等。
1、编写中间件
在middlewares.py
文件中编写自定义中间件。
class CustomMiddleware:
def process_request(self, request, spider):
request.headers['User-Agent'] = 'Custom User-Agent'
return None
2、启用中间件
在settings.py
文件中启用自定义中间件。
DOWNLOADER_MIDDLEWARES = {
'myproject.middlewares.CustomMiddleware': 543,
}
十、部署爬虫
在开发完成后,可以将爬虫部署到服务器上运行。Scrapy提供了一个名为Scrapyd的部署工具。
1、安装Scrapyd
pip install scrapyd
2、启动Scrapyd
scrapyd
3、使用Scrapyd-client部署爬虫
pip install scrapyd-client
scrapyd-deploy
通过以上步骤,我们成功将爬虫部署到服务器上运行。
总结
通过以上内容,我们详细介绍了如何用Python做爬虫软件,从请求网页内容、解析网页内容,到使用Scrapy框架进行高级爬取,以及处理反爬机制、存储抓取数据、常见问题解决方法、优化扩展等多个方面。希望这些内容能帮助你更好地理解和应用Python爬虫技术。
相关问答FAQs:
如何选择合适的Python库来开发爬虫软件?
在Python中,有多个库可以帮助你开发爬虫软件。最常用的包括Requests和BeautifulSoup,它们可以帮助你发送HTTP请求和解析HTML文档。对于需要处理JavaScript动态加载内容的网站,可以考虑使用Selenium或Scrapy,这些工具不仅能够抓取数据,还能模拟浏览器行为。根据你的需求选择合适的库会使爬虫开发更加高效。
在进行网络爬虫时,如何避免被网站封禁?
为了降低被网站封禁的风险,可以采取一些措施。首先,设置合理的请求间隔时间,避免短时间内发送大量请求。使用代理IP可以帮助你伪装真实IP地址,减少被检测的可能性。此外,合理地使用User-Agent头信息,模拟不同的浏览器请求,也是一个有效的策略。遵循网站的robots.txt协议,确保遵循网站的抓取规则也是非常重要的。
如何处理爬虫过程中遇到的数据存储问题?
在爬虫过程中,数据存储是一个关键环节。可以选择将抓取的数据存储在本地文件中,如CSV或JSON格式,便于后续处理。也可以使用数据库系统,如SQLite、MySQL或MongoDB,来存储和管理数据。这不仅有助于数据的持久化,还能方便后续的查询和分析。根据数据的规模和使用场景,选择合适的存储方式将提升爬虫的性能和效率。
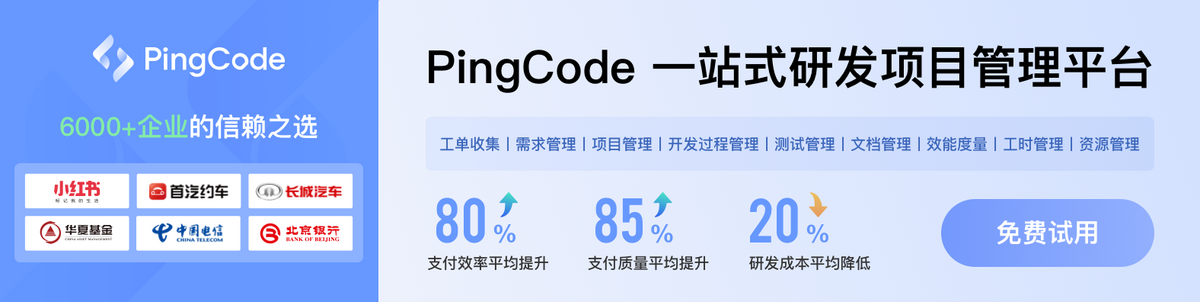