在Python中,print语句默认会在输出内容后自动添加一个换行符。可以通过指定end
参数来控制是否在输出后添加特定的字符、使用end
参数指定为空字符串来避免换行、或者使用多行字符串来输出多行内容。 例如:
- 默认换行
- 使用
end
参数控制换行 - 使用多行字符串
以下是详细描述的内容:
默认换行:在Python中,print()
函数默认会在输出内容后添加一个换行符。换句话说,每次调用print()
函数时,输出内容会自动换行。例如:
print("Hello, World!")
print("This is a new line.")
上述代码将输出:
Hello, World!
This is a new line.
使用end
参数控制换行:print()
函数的end
参数允许您指定在输出内容后附加的字符。默认情况下,end
参数的值是换行符(\n
)。如果想要避免换行,可以将end
参数设为空字符串(''
)或其他字符串。例如:
print("Hello, World!", end='')
print("This is on the same line.")
上述代码将输出:
Hello, World!This is on the same line.
通过这种方式,您可以灵活地控制输出内容是否换行以及如何换行。
使用多行字符串:在Python中,可以使用三重引号('''
或 """
)来定义多行字符串。这种方法允许您在字符串中包含多个换行符。例如:
print("""Hello, World!
This is a new line.
And this is another line.""")
上述代码将输出:
Hello, World!
This is a new line.
And this is another line.
通过这种方式,您可以轻松地在一个print()
语句中输出多行内容。
接下来,我们将详细探讨每种方法的应用和特点。
一、默认换行
Python的print()
函数默认会在输出内容后添加一个换行符。这使得每次调用print()
函数时,输出内容会自动换行。这种默认行为非常适合大多数情况,因为它让输出内容更易于阅读和理解。
例如,以下代码段展示了默认换行的效果:
print("First line")
print("Second line")
print("Third line")
上述代码将输出三行内容,每行内容会自动换行:
First line
Second line
Third line
这种默认换行的行为非常适合逐行输出内容,但有时您可能希望控制换行行为,这时可以使用end
参数。
二、使用end
参数控制换行
在Python中,print()
函数的end
参数允许您指定在输出内容后附加的字符。默认情况下,end
参数的值是换行符(\n
)。通过设置end
参数,您可以灵活地控制输出内容是否换行以及如何换行。
避免换行
如果希望避免输出内容换行,可以将end
参数设为空字符串(''
)。例如:
print("Hello, World!", end='')
print("This is on the same line.")
上述代码将输出:
Hello, World!This is on the same line.
自定义换行符
除了避免换行,您还可以使用end
参数自定义换行符。例如,您可以使用逗号和空格作为换行符:
print("Hello, World!", end=', ')
print("This is a new line.")
上述代码将输出:
Hello, World!, This is a new line.
通过这种方式,您可以根据需要灵活地控制输出内容的格式。
三、使用多行字符串
在Python中,可以使用三重引号('''
或 """
)来定义多行字符串。这种方法允许您在一个字符串中包含多个换行符,从而在一个print()
语句中输出多行内容。
多行字符串示例
以下代码展示了如何使用多行字符串输出多行内容:
print("""Hello, World!
This is a new line.
And this is another line.""")
上述代码将输出:
Hello, World!
This is a new line.
And this is another line.
自动换行
使用多行字符串时,您可以在字符串中直接包含换行符,从而实现自动换行。例如:
multi_line_string = """This is the first line.
This is the second line.
This is the third line."""
print(multi_line_string)
上述代码将输出:
This is the first line.
This is the second line.
This is the third line.
这种方法非常适合需要在一个字符串中包含多个换行符的情况。
四、结合使用end
参数和多行字符串
在某些情况下,您可能希望结合使用end
参数和多行字符串,以实现更复杂的输出格式。例如,以下代码展示了如何结合使用这两种方法:
print("First part of the line", end='')
print(" - Second part of the line")
print("""This is a new line.
This is another new line.""")
上述代码将输出:
First part of the line - Second part of the line
This is a new line.
This is another new line.
通过这种方式,您可以灵活地控制输出内容的格式和换行行为。
五、其他控制输出的方法
除了上述方法之外,Python还提供了一些其他控制输出内容的方式,例如使用sys.stdout.write()
函数和字符串格式化方法。
使用sys.stdout.write()
sys.stdout.write()
函数可以用于精细控制输出内容,而不会自动添加换行符。例如:
import sys
sys.stdout.write("Hello, World!")
sys.stdout.write("This is on the same line.")
上述代码将输出:
Hello, World!This is on the same line.
使用字符串格式化
Python提供了多种字符串格式化方法,例如%
操作符、str.format()
方法和f字符串(f-strings)。这些方法可以帮助您更灵活地控制输出内容的格式。
使用%
操作符
name = "Alice"
age = 30
print("Name: %s, Age: %d" % (name, age))
上述代码将输出:
Name: Alice, Age: 30
使用str.format()
方法
name = "Bob"
age = 25
print("Name: {}, Age: {}".format(name, age))
上述代码将输出:
Name: Bob, Age: 25
使用f字符串(f-strings)
name = "Charlie"
age = 35
print(f"Name: {name}, Age: {age}")
上述代码将输出:
Name: Charlie, Age: 35
通过使用字符串格式化方法,您可以更加灵活地控制输出内容的格式和布局。
六、实际应用场景
在实际应用中,控制输出内容的换行行为和格式是非常常见的需求。以下是几个实际应用场景的示例:
日志记录
在日志记录中,控制输出内容的格式和换行行为非常重要。例如:
import logging
logging.basicConfig(level=logging.INFO)
logging.info("This is an info message.")
logging.warning("This is a warning message.")
logging.error("This is an error message.")
上述代码将输出:
INFO:root:This is an info message.
WARNING:root:This is a warning message.
ERROR:root:This is an error message.
表格输出
在输出表格数据时,控制换行行为和格式同样非常重要。例如:
data = [
["Name", "Age", "City"],
["Alice", 30, "New York"],
["Bob", 25, "Los Angeles"],
["Charlie", 35, "Chicago"]
]
for row in data:
print("{:<10} {:<10} {:<10}".format(*row))
上述代码将输出:
Name Age City
Alice 30 New York
Bob 25 Los Angeles
Charlie 35 Chicago
动态进度条
在显示动态进度条时,需要避免输出内容换行。例如:
import time
for i in range(101):
print("\rProgress: [{}{}] {}%".format("#" * i, " " * (100 - i), i), end='')
time.sleep(0.1)
print("\nDone!")
上述代码将输出一个动态进度条,显示进度百分比,并在完成时输出“Done!”。
通过这些实际应用场景的示例,可以看出控制输出内容的换行行为和格式在许多情况下都是非常重要的。掌握这些方法可以帮助您在编写Python代码时更加灵活地控制输出内容的呈现方式。
七、总结
在Python中,控制print()
语句的换行行为和输出格式是非常重要的。通过使用默认换行、end
参数、多行字符串以及其他控制输出的方法,您可以灵活地控制输出内容的格式和布局。这些方法在实际应用中有广泛的应用,例如日志记录、表格输出和动态进度条等。
掌握这些方法可以帮助您在编写Python代码时更加高效地控制输出内容的呈现方式,从而提高代码的可读性和可维护性。希望本文对您理解和应用Python中print()
语句的换行和输出控制有所帮助。
相关问答FAQs:
如何在Python中使用print语句换行?
在Python中,print语句默认在输出后会自动添加一个换行符。如果希望在输出的内容中进行换行,可以使用换行符\n
。例如:print("Hello\nWorld")
将会输出“Hello”和“World”在不同的行中。
可以在print语句中添加额外的空行吗?
是的,可以通过多次使用\n
来添加额外的空行。例如,print("Line 1\n\nLine 2")
将会在“Line 1”和“Line 2”之间插入一个空行。还可以通过在print语句中重复\n
,例如print("Hello\n\n\nWorld")
,来创建多个空行。
如何在print语句中自定义结尾字符?
在Python的print函数中,可以使用end
参数来自定义输出的结尾字符。默认情况下,end
的值是换行符\n
。如果希望在输出后不换行,可以将其设置为空字符串,例如:print("Hello", end="")
。如果想要在输出后添加其他字符,例如空格或其他符号,可以这样设置:print("Hello", end=" ")
。这样将会在“Hello”后添加一个空格而不是换行。
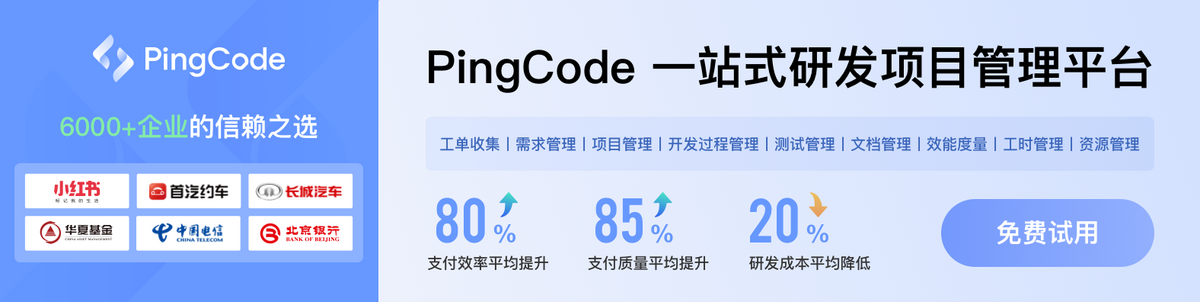