要用Python写一个京东监控系统,我们可以使用requests库进行网络请求、BeautifulSoup库进行网页解析、以及selenium库进行自动化浏览器操作。这些工具结合在一起,可以帮助我们实现对京东商品价格的监控、库存情况的监控以及自动下单等功能。下面我将详细描述如何实现这些功能。
一、使用requests库进行网络请求
requests库是Python中非常强大的HTTP库,可以用于发送HTTP请求,包括GET和POST请求。通过requests库,我们可以轻松地获取网页的HTML内容。
import requests
def fetch_product_page(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
return response.text
示例用法
url = 'https://item.jd.com/100012043978.html'
html_content = fetch_product_page(url)
print(html_content)
二、使用BeautifulSoup进行网页解析
BeautifulSoup是一个用于解析HTML和XML文档的Python库。我们可以使用它来提取网页中的特定信息,例如商品名称、价格和库存情况。
from bs4 import BeautifulSoup
def parse_product_page(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
product_name = soup.find('div', {'class': 'sku-name'}).text.strip()
price = soup.find('span', {'class': 'price'}).text.strip()
return product_name, price
示例用法
product_name, price = parse_product_page(html_content)
print(f'Product Name: {product_name}')
print(f'Price: {price}')
三、使用Selenium进行自动化浏览器操作
Selenium是一个用于Web应用程序测试的自动化工具。我们可以使用它来实现复杂的浏览器操作,例如登录、添加到购物车和下单。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
def automate_login_jd(username, password):
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.get('https://passport.jd.com/new/login.aspx')
user_input = driver.find_element(By.NAME, 'loginname')
pass_input = driver.find_element(By.NAME, 'nloginpwd')
user_input.send_keys(username)
pass_input.send_keys(password)
pass_input.send_keys(Keys.RETURN)
return driver
示例用法
username = 'your_username'
password = 'your_password'
driver = automate_login_jd(username, password)
四、监控价格和库存
我们可以定期发送请求并解析网页内容,以监控商品的价格和库存情况。
import time
def monitor_product(url, target_price):
while True:
html_content = fetch_product_page(url)
product_name, price = parse_product_page(html_content)
current_price = float(price.replace('¥', ''))
if current_price <= target_price:
print(f'{product_name} is now available at {current_price}!')
break
print(f'Current price of {product_name} is {current_price}. Waiting for price drop...')
time.sleep(60) # 每分钟检查一次
示例用法
target_price = 2000.0
monitor_product(url, target_price)
五、自动下单
在价格达到目标时,我们可以使用Selenium自动完成下单操作。
def place_order(driver):
driver.get('https://cart.jd.com/cart.action')
checkout_button = driver.find_element(By.CLASS_NAME, 'checkout-submit')
checkout_button.click()
submit_order_button = driver.find_element(By.ID, 'order-submit')
submit_order_button.click()
示例用法
place_order(driver)
六、综合实现
将以上功能综合到一个完整的京东监控系统中:
import requests
from bs4 import BeautifulSoup
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
import time
def fetch_product_page(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
return response.text
def parse_product_page(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
product_name = soup.find('div', {'class': 'sku-name'}).text.strip()
price = soup.find('span', {'class': 'price'}).text.strip()
return product_name, price
def automate_login_jd(username, password):
driver = webdriver.Chrome(service=ChromeService(ChromeDriverManager().install()))
driver.get('https://passport.jd.com/new/login.aspx')
user_input = driver.find_element(By.NAME, 'loginname')
pass_input = driver.find_element(By.NAME, 'nloginpwd')
user_input.send_keys(username)
pass_input.send_keys(password)
pass_input.send_keys(Keys.RETURN)
return driver
def monitor_product(url, target_price):
while True:
html_content = fetch_product_page(url)
product_name, price = parse_product_page(html_content)
current_price = float(price.replace('¥', ''))
if current_price <= target_price:
print(f'{product_name} is now available at {current_price}!')
break
print(f'Current price of {product_name} is {current_price}. Waiting for price drop...')
time.sleep(60) # 每分钟检查一次
def place_order(driver):
driver.get('https://cart.jd.com/cart.action')
checkout_button = driver.find_element(By.CLASS_NAME, 'checkout-submit')
checkout_button.click()
submit_order_button = driver.find_element(By.ID, 'order-submit')
submit_order_button.click()
if __name__ == '__main__':
url = 'https://item.jd.com/100012043978.html'
target_price = 2000.0
username = 'your_username'
password = 'your_password'
driver = automate_login_jd(username, password)
monitor_product(url, target_price)
place_order(driver)
通过以上代码,我们实现了一个简单的京东商品监控系统。这个系统可以定期检查商品价格,并在价格达到目标时自动下单。当然,这只是一个基础实现,实际应用中可能需要处理更多细节和错误情况,例如网络异常、页面元素变动等。希望这篇文章对你有所帮助!
相关问答FAQs:
如何用Python实现京东商品价格监控?
为了实现京东商品的价格监控,您可以使用Python的爬虫库,如Requests和BeautifulSoup,来抓取商品页面的数据。首先,您需要确定要监控的商品链接,然后用Requests库获取页面内容,接着用BeautifulSoup解析HTML,提取价格信息。可以定时运行这个脚本,比如使用定时任务,来监控价格变化,并在价格变动时发送通知。
监控京东商品价格时需要注意哪些法律和道德问题?
在进行京东商品监控时,应遵循网站的使用条款,避免发送过多请求而对服务器造成负担。许多网站有反爬虫机制,频繁请求可能导致IP被封禁。建议在请求之间设置适当的间隔,并尽量模拟人工浏览行为。此外,使用API(如京东开放平台提供的API)进行数据获取也是一个合规的选择。
如何将监控到的价格变化发送到我的手机或邮箱?
您可以使用Python的邮件库(如smtplib)发送电子邮件,或使用推送通知服务(如Pushover或Pushbullet)将价格变化实时通知到手机。在监控脚本中添加逻辑,当检测到价格变化时,触发邮件或推送通知的发送。这种方式可以让您及时获知商品的价格变动,方便您做出购买决策。
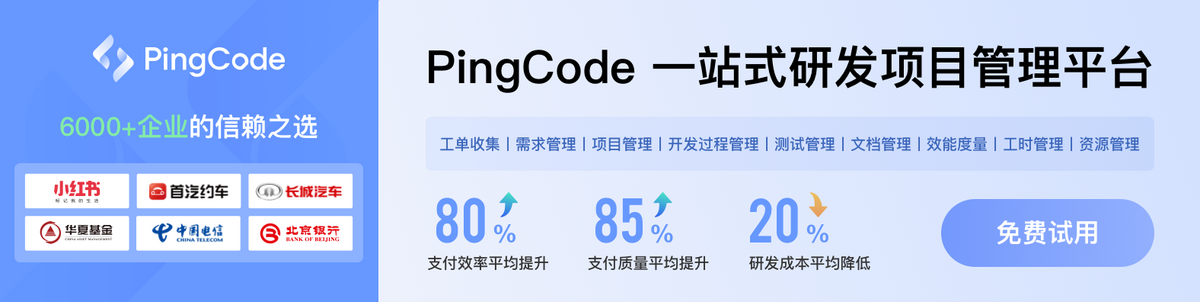