在ROS中使用Python包的方法包括:安装必要的Python包、编写Python节点、配置CMakeLists.txt和package.xml文件、运行Python节点。首先需要确保你已经安装了ROS和相应的Python开发环境。接下来我们详细介绍如何在ROS中使用Python包,并深入探讨其中的一个步骤——编写Python节点。
一、安装必要的Python包
在ROS中使用Python包之前,首先需要安装所需的Python包。可以通过以下步骤进行:
1.1、使用pip
安装Python包
pip
是Python的包管理工具,可以方便地安装和管理Python包。例如,要安装rospy
(ROS的Python客户端库),可以使用以下命令:
pip install rospy
同样地,你可以安装其他需要的Python包,例如numpy
、scipy
等:
pip install numpy scipy
1.2、使用ROS的包管理工具安装
有些ROS包可能需要通过ROS的包管理工具进行安装,例如使用apt-get
命令:
sudo apt-get install ros-noetic-rospy
请注意,ros-noetic-rospy
适用于ROS Noetic版本,根据你的ROS版本,包名可能会有所不同。
二、编写Python节点
编写Python节点是使用Python包的核心步骤。下面是一个简单的Python节点示例,展示了如何在ROS中使用Python包进行节点开发。
2.1、创建一个新的ROS包
首先,在你的工作空间中创建一个新的ROS包:
cd ~/catkin_ws/src
catkin_create_pkg my_python_pkg rospy std_msgs
2.2、编写Python节点脚本
在新创建的包中,编写一个Python节点脚本。例如,创建一个名为talker.py
的文件:
cd ~/catkin_ws/src/my_python_pkg/scripts
touch talker.py
chmod +x talker.py
然后,编辑talker.py
文件,编写节点代码:
#!/usr/bin/env python
import rospy
from std_msgs.msg import String
def talker():
pub = rospy.Publisher('chatter', String, queue_size=10)
rospy.init_node('talker', anonymous=True)
rate = rospy.Rate(10) # 10hz
while not rospy.is_shutdown():
hello_str = "hello world %s" % rospy.get_time()
rospy.loginfo(hello_str)
pub.publish(hello_str)
rate.sleep()
if __name__ == '__main__':
try:
talker()
except rospy.ROSInterruptException:
pass
2.3、配置CMakeLists.txt和package.xml文件
在ROS中使用Python节点时,需要确保在CMakeLists.txt
和package.xml
文件中正确配置。
在package.xml
中,确保包含以下依赖项:
<build_depend>rospy</build_depend>
<exec_depend>rospy</exec_depend>
在CMakeLists.txt
中,确保包含以下配置:
catkin_install_python(PROGRAMS
scripts/talker.py
DESTINATION ${CATKIN_PACKAGE_BIN_DESTINATION}
)
三、运行Python节点
在完成了上述步骤后,可以运行Python节点。首先,确保已经构建了工作空间:
cd ~/catkin_ws
catkin_make
source devel/setup.bash
然后,可以运行Python节点:
rosrun my_python_pkg talker.py
四、实例详解:编写复杂的Python节点
编写Python节点是ROS开发中的核心步骤之一,下面我们详细介绍一个更复杂的Python节点示例,包括订阅和发布多个主题、处理数据并进行简单的控制逻辑。
4.1、创建复杂的Python节点脚本
在my_python_pkg
包的scripts
目录下,创建一个名为complex_node.py
的文件:
cd ~/catkin_ws/src/my_python_pkg/scripts
touch complex_node.py
chmod +x complex_node.py
然后,编辑complex_node.py
文件,编写节点代码:
#!/usr/bin/env python
import rospy
from std_msgs.msg import String, Float32
from sensor_msgs.msg import LaserScan
class ComplexNode:
def __init__(self):
rospy.init_node('complex_node', anonymous=True)
self.laser_sub = rospy.Subscriber('/scan', LaserScan, self.laser_callback)
self.string_pub = rospy.Publisher('/chatter', String, queue_size=10)
self.float_pub = rospy.Publisher('/distance', Float32, queue_size=10)
self.rate = rospy.Rate(10) # 10hz
self.min_distance = float('inf')
def laser_callback(self, data):
self.min_distance = min(data.ranges)
rospy.loginfo("Min distance: %f", self.min_distance)
def run(self):
while not rospy.is_shutdown():
hello_str = "hello world %s" % rospy.get_time()
rospy.loginfo(hello_str)
self.string_pub.publish(hello_str)
self.float_pub.publish(self.min_distance)
self.rate.sleep()
if __name__ == '__main__':
try:
node = ComplexNode()
node.run()
except rospy.ROSInterruptException:
pass
4.2、添加依赖项和配置文件
在package.xml
中,确保包含以下依赖项:
<build_depend>rospy</build_depend>
<exec_depend>rospy</exec_depend>
<build_depend>std_msgs</build_depend>
<exec_depend>std_msgs</exec_depend>
<build_depend>sensor_msgs</build_depend>
<exec_depend>sensor_msgs</exec_depend>
在CMakeLists.txt
中,确保包含以下配置:
catkin_install_python(PROGRAMS
scripts/talker.py
scripts/complex_node.py
DESTINATION ${CATKIN_PACKAGE_BIN_DESTINATION}
)
4.3、运行复杂的Python节点
首先,确保已经构建了工作空间:
cd ~/catkin_ws
catkin_make
source devel/setup.bash
然后,可以运行复杂的Python节点:
rosrun my_python_pkg complex_node.py
五、调试与测试
在开发过程中,调试和测试是必不可少的步骤。下面是一些调试和测试Python节点的方法。
5.1、使用rospy.loginfo
进行日志记录
在节点代码中,可以使用rospy.loginfo
、rospy.logwarn
和rospy.logerr
等日志记录函数来输出调试信息。例如:
rospy.loginfo("This is an info message")
rospy.logwarn("This is a warning message")
rospy.logerr("This is an error message")
5.2、使用roslaunch
进行集成测试
可以使用roslaunch
工具来启动多个节点并进行集成测试。创建一个名为test.launch
的文件:
cd ~/catkin_ws/src/my_python_pkg/launch
touch test.launch
编辑test.launch
文件:
<launch>
<node name="talker" pkg="my_python_pkg" type="talker.py" output="screen"/>
<node name="listener" pkg="my_python_pkg" type="listener.py" output="screen"/>
</launch>
然后,运行roslaunch
:
roslaunch my_python_pkg test.launch
5.3、使用rostest
进行单元测试
rostest
是ROS的测试工具,可以用于编写和运行单元测试。创建一个名为test_talker.test
的文件:
cd ~/catkin_ws/src/my_python_pkg/test
touch test_talker.test
编辑test_talker.test
文件:
<launch>
<test test-name="test_talker" pkg="my_python_pkg" type="test_talker.py" />
</launch>
编写测试脚本test_talker.py
:
#!/usr/bin/env python
import rospy
import unittest
from std_msgs.msg import String
class TestTalker(unittest.TestCase):
def callback(self, data):
self.received_msg = data.data
def test_talker(self):
rospy.init_node('test_talker', anonymous=True)
rospy.Subscriber('/chatter', String, self.callback)
self.received_msg = None
timeout = rospy.Time.now() + rospy.Duration(5)
while not rospy.is_shutdown() and rospy.Time.now() < timeout and self.received_msg is None:
rospy.sleep(0.1)
self.assertIsNotNone(self.received_msg, "Did not receive any messages within timeout period")
self.assertIn("hello world", self.received_msg)
if __name__ == '__main__':
import rostest
rostest.rosrun('my_python_pkg', 'test_talker', TestTalker)
运行单元测试:
rostest my_python_pkg test_talker.test
六、优化Python节点性能
在ROS中使用Python节点时,可能需要优化性能以确保系统的实时性和响应速度。以下是一些优化Python节点性能的方法。
6.1、使用NumPy进行高效计算
NumPy是一个强大的科学计算库,可以用于高效地处理大规模数据。可以在节点代码中使用NumPy替代原生Python的计算函数。例如:
import numpy as np
def process_data(data):
array = np.array(data)
result = np.sum(array)
return result
6.2、避免全局变量
尽量避免使用全局变量,以减少线程间的竞争和数据不一致的问题。可以将变量封装在类或函数中。例如:
class MyNode:
def __init__(self):
self.data = None
def callback(self, msg):
self.data = msg.data
node = MyNode()
6.3、使用多线程或多进程
在某些情况下,可以使用多线程或多进程来提高节点的性能。Python的threading
和multiprocessing
模块可以方便地实现多线程和多进程。例如:
import threading
class MyNode:
def __init__(self):
self.lock = threading.Lock()
def callback(self, msg):
with self.lock:
# 处理数据
pass
七、使用Python工具和库扩展ROS功能
在ROS中使用Python包时,可以利用Python的丰富生态系统来扩展ROS的功能。以下是一些常用的Python工具和库。
7.1、使用Matplotlib进行数据可视化
Matplotlib是一个强大的数据可视化库,可以用于绘制各种类型的图表。在ROS节点中,可以使用Matplotlib实时绘制数据。例如:
import matplotlib.pyplot as plt
class PlotNode:
def __init__(self):
self.fig, self.ax = plt.subplots()
self.x_data = []
self.y_data = []
def update_plot(self, x, y):
self.x_data.append(x)
self.y_data.append(y)
self.ax.clear()
self.ax.plot(self.x_data, self.y_data)
plt.pause(0.01)
plot_node = PlotNode()
7.2、使用Scipy进行科学计算
Scipy是一个科学计算库,提供了许多高级算法和函数。在ROS节点中,可以使用Scipy进行复杂的计算。例如:
from scipy.optimize import minimize
def objective_function(x):
return x2 + 2*x + 1
result = minimize(objective_function, 0)
print(result.x)
7.3、使用OpenCV进行图像处理
OpenCV是一个开源的计算机视觉库,可以用于图像处理和计算机视觉任务。在ROS节点中,可以使用OpenCV处理图像数据。例如:
import cv2
from sensor_msgs.msg import Image
from cv_bridge import CvBridge
class ImageNode:
def __init__(self):
self.bridge = CvBridge()
self.image_sub = rospy.Subscriber('/camera/image', Image, self.image_callback)
def image_callback(self, data):
cv_image = self.bridge.imgmsg_to_cv2(data, "bgr8")
gray_image = cv2.cvtColor(cv_image, cv2.COLOR_BGR2GRAY)
cv2.imshow("Image Window", gray_image)
cv2.waitKey(3)
image_node = ImageNode()
八、总结
在本文中,我们详细介绍了在ROS中使用Python包的方法,包括安装必要的Python包、编写Python节点、配置CMakeLists.txt和package.xml文件、运行Python节点、调试与测试、优化Python节点性能以及使用Python工具和库扩展ROS功能。通过这些步骤和方法,你可以在ROS中高效地开发和使用Python节点。在实际开发过程中,合理利用Python的丰富生态系统和ROS的强大功能,可以大大提高开发效率和系统性能。
相关问答FAQs:
如何在ROS中安装Python包?
在ROS中安装Python包通常可以通过使用pip
命令来实现。您可以在终端中运行pip install package_name
来安装所需的Python库。如果您希望在特定的ROS工作空间中使用这些包,建议在您的工作空间目录下创建一个虚拟环境,这样可以避免与系统包发生冲突。此外,确保在安装包之前激活ROS环境,以便正确配置依赖关系。
在ROS中,如何使用Python脚本进行节点编写?
编写Python脚本作为ROS节点非常简单。您只需创建一个Python文件,并在文件开头添加#!/usr/bin/env python
来指定Python解释器。接下来,您需要导入rospy
库,并使用rospy.init_node()
函数初始化节点。通过定义回调函数和使用rospy.Subscriber()
或rospy.Publisher()
,您可以实现消息的发送和接收。完成后,确保将脚本设置为可执行文件,以便ROS可以运行它。
如何调试在ROS中使用的Python包?
调试Python包时,您可以利用Python的内置调试工具,如pdb
,它可以让您逐步执行代码并检查变量值。此外,使用rospy.loginfo()
、rospy.logwarn()
和rospy.logerr()
等日志记录功能,可以帮助您更好地了解程序的运行状态。借助这些工具,您可以有效地识别和解决在ROS环境中可能出现的各种问题。
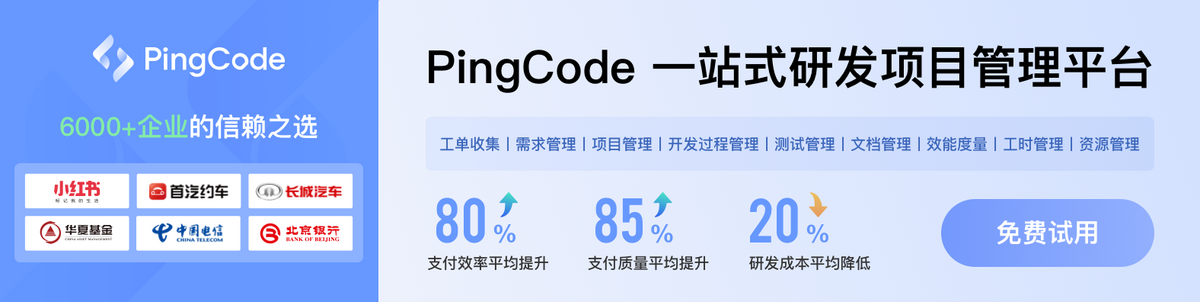