实现Python调用API接口的步骤包括:选择合适的HTTP库、构建请求URL、设置请求头和参数、发送请求、处理响应、处理错误。 其中,选择合适的HTTP库是实现API调用的关键步骤之一。Python有多个HTTP库,如requests
、http.client
、urllib
等,其中requests
库因其简单易用和功能强大而被广泛使用。使用requests
库可以轻松地发送各种类型的HTTP请求,如GET、POST、PUT、DELETE等,并处理返回的响应数据。
一、选择合适的HTTP库
Python提供了多个HTTP库供用户选择,其中最常用的是requests
库。它是一个简洁且功能强大的HTTP库,支持发送GET、POST、PUT、DELETE等请求类型,并且可以轻松处理响应数据。相比于其他库,requests
库更易于使用,并且具有良好的文档和社区支持。
安装requests库
在使用requests
库之前,需要先安装它。可以使用以下命令通过pip进行安装:
pip install requests
安装完成后,就可以在Python代码中导入并使用requests
库来发送HTTP请求。
二、构建请求URL
在发送HTTP请求之前,需要构建请求的URL。API接口通常由基础URL和路径参数组成,有时还需要添加查询参数。基础URL是API的根地址,路径参数用于指定资源,查询参数用于传递额外的信息。
示例
假设我们要调用一个天气API接口来获取某个城市的天气信息,基础URL为http://api.weather.com/v3/wx/conditions/current
,我们需要提供城市名称作为查询参数。构建请求URL的示例如下:
import requests
base_url = "http://api.weather.com/v3/wx/conditions/current"
city = "London"
query_params = {
"apiKey": "your_api_key",
"format": "json",
"language": "en-US",
"location": city
}
response = requests.get(base_url, params=query_params)
print(response.json())
三、设置请求头和参数
在构建请求URL时,可能需要设置请求头和参数。请求头用于传递HTTP协议相关的信息,如用户代理、授权信息等。请求参数用于传递请求的具体数据,如查询参数、路径参数等。
设置请求头
请求头可以通过requests
库的headers
参数进行设置。例如,设置用户代理和授权信息的请求头:
headers = {
"User-Agent": "my-app",
"Authorization": "Bearer your_access_token"
}
response = requests.get(base_url, headers=headers, params=query_params)
print(response.json())
四、发送请求
构建好请求URL并设置好请求头和参数后,就可以发送HTTP请求。requests
库支持发送多种类型的HTTP请求,如GET、POST、PUT、DELETE等。
发送GET请求
GET请求用于从服务器获取数据。调用API接口获取数据时,通常使用GET请求。示例代码如下:
response = requests.get(base_url, headers=headers, params=query_params)
print(response.json())
发送POST请求
POST请求用于向服务器提交数据。例如,调用API接口向服务器提交表单数据或JSON数据时,通常使用POST请求。示例代码如下:
data = {
"name": "John",
"age": 30
}
response = requests.post("http://api.example.com/v1/users", headers=headers, json=data)
print(response.json())
五、处理响应
发送HTTP请求后,需要处理服务器返回的响应。响应通常包含状态码、响应头和响应体。状态码用于表示请求的结果,如200表示成功,404表示资源未找到,500表示服务器错误等。响应头包含关于响应的元数据,如内容类型、日期等。响应体包含实际的响应数据,通常是JSON格式。
处理状态码
可以通过response.status_code
获取响应的状态码,并根据状态码进行相应的处理。例如:
if response.status_code == 200:
print("Request was successful")
elif response.status_code == 404:
print("Resource not found")
else:
print(f"Unexpected status code: {response.status_code}")
处理响应体
可以通过response.json()
方法将响应体解析为JSON对象,或者通过response.text
获取响应体的原始文本。例如:
response_data = response.json()
print(response_data)
六、处理错误
在调用API接口时,可能会遇到各种错误,如网络错误、超时错误、服务器错误等。需要使用异常处理机制来捕获并处理这些错误。例如:
try:
response = requests.get(base_url, headers=headers, params=query_params)
response.raise_for_status() # 如果响应状态码不是200,会引发HTTPError异常
response_data = response.json()
print(response_data)
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
七、示例项目
下面通过一个完整的示例项目来演示如何实现Python调用API接口。假设我们要构建一个天气应用,通过调用天气API接口获取指定城市的天气信息。
项目结构
weather_app/
├── main.py
└── requirements.txt
requirements.txt
requests
main.py
import requests
def get_weather(city):
base_url = "http://api.weather.com/v3/wx/conditions/current"
query_params = {
"apiKey": "your_api_key",
"format": "json",
"language": "en-US",
"location": city
}
try:
response = requests.get(base_url, params=query_params)
response.raise_for_status() # 如果响应状态码不是200,会引发HTTPError异常
return response.json()
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return None
def main():
city = input("Enter the city name: ")
weather_data = get_weather(city)
if weather_data:
print(f"Weather data for {city}:")
print(weather_data)
else:
print("Failed to retrieve weather data")
if __name__ == "__main__":
main()
运行项目
在终端中运行以下命令启动项目:
python main.py
输入城市名称后,程序会调用天气API接口获取该城市的天气信息,并输出到控制台。
八、扩展功能
在实际项目中,可能需要实现更多的功能,如缓存请求结果、异步请求、批量请求等。下面介绍一些常见的扩展功能。
缓存请求结果
为了减少API调用次数和提高响应速度,可以缓存请求结果。可以使用requests_cache
库来实现缓存功能。
安装requests_cache库
pip install requests_cache
使用requests_cache库
import requests
import requests_cache
requests_cache.install_cache('weather_cache', expire_after=3600) # 缓存1小时
def get_weather(city):
base_url = "http://api.weather.com/v3/wx/conditions/current"
query_params = {
"apiKey": "your_api_key",
"format": "json",
"language": "en-US",
"location": city
}
try:
response = requests.get(base_url, params=query_params)
response.raise_for_status() # 如果响应状态码不是200,会引发HTTPError异常
return response.json()
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return None
异步请求
为了提高请求的并发性能,可以使用异步请求。可以使用aiohttp
库来实现异步请求。
安装aiohttp库
pip install aiohttp
使用aiohttp库
import aiohttp
import asyncio
async def get_weather(city):
base_url = "http://api.weather.com/v3/wx/conditions/current"
query_params = {
"apiKey": "your_api_key",
"format": "json",
"language": "en-US",
"location": city
}
async with aiohttp.ClientSession() as session:
try:
async with session.get(base_url, params=query_params) as response:
response.raise_for_status() # 如果响应状态码不是200,会引发HTTPError异常
return await response.json()
except aiohttp.ClientError as e:
print(f"An error occurred: {e}")
return None
async def main():
city = input("Enter the city name: ")
weather_data = await get_weather(city)
if weather_data:
print(f"Weather data for {city}:")
print(weather_data)
else:
print("Failed to retrieve weather data")
if __name__ == "__main__":
asyncio.run(main())
批量请求
为了提高批量请求的效率,可以使用多线程或多进程来并发发送请求。可以使用concurrent.futures
库来实现批量请求。
使用concurrent.futures库
import requests
from concurrent.futures import ThreadPoolExecutor, as_completed
def get_weather(city):
base_url = "http://api.weather.com/v3/wx/conditions/current"
query_params = {
"apiKey": "your_api_key",
"format": "json",
"language": "en-US",
"location": city
}
try:
response = requests.get(base_url, params=query_params)
response.raise_for_status() # 如果响应状态码不是200,会引发HTTPError异常
return response.json()
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
return None
def main():
cities = ["London", "New York", "Paris", "Tokyo", "Beijing"]
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(get_weather, city) for city in cities]
for future in as_completed(futures):
weather_data = future.result()
if weather_data:
print(weather_data)
else:
print("Failed to retrieve weather data")
if __name__ == "__main__":
main()
总结
通过本文的介绍,我们详细讨论了如何实现Python调用API接口,包括选择合适的HTTP库、构建请求URL、设置请求头和参数、发送请求、处理响应、处理错误等步骤。我们还通过一个完整的示例项目演示了如何调用天气API接口获取指定城市的天气信息,并介绍了一些常见的扩展功能,如缓存请求结果、异步请求、批量请求等。希望本文能够帮助大家更好地理解和掌握Python调用API接口的方法和技巧。
相关问答FAQs:
如何在Python中找到适合的API接口文档?
在使用Python调用API接口时,了解API的功能和使用方法至关重要。通常,API提供者会在其官方网站上提供详细的API文档,文档中会包含如何进行身份验证、可用的端点、请求和响应示例等信息。选择合适的API时,可以根据功能、易用性和社区支持等因素进行评估。
使用Python调用API接口时需要注意哪些事项?
在调用API接口时,需要考虑几个重要因素。首先,确保处理好API请求的速率限制,以免因过于频繁的请求导致被封禁。其次,关注数据格式,通常API会返回JSON或XML格式的数据,确保能够正确解析这些数据。此外,处理异常情况也是必不可少的,例如网络问题或API返回错误信息。
如何调试Python中调用API接口的代码?
调试时,可以使用Python的内置库如requests
,结合打印调试信息来观察请求和响应的内容。如果遇到问题,可以使用print()
函数输出请求的URL、参数以及响应状态码和内容。此外,使用工具如Postman可以在编写代码之前先测试API接口,确保接口正常工作,再将其整合进Python代码中,从而减少调试的难度。
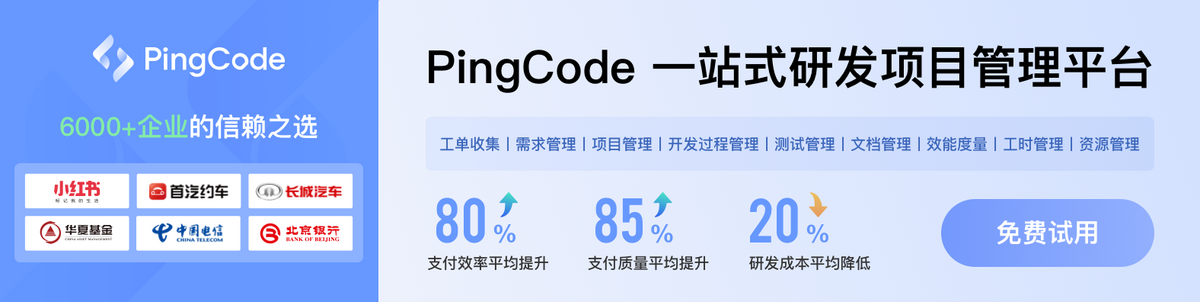