Python实现单位转换的方法有很多种,主要有:使用内置函数、利用第三方库、编写自定义函数。其中利用第三方库的方法是最为方便和常用的,下面将详细介绍如何使用这些方法来实现单位转换。
一、使用内置函数进行单位转换
Python内置函数可以处理一些简单的单位转换,比如时间和角度的转换。
1、时间单位转换
Python内置的datetime
模块可以非常方便地进行时间单位转换。
import datetime
秒转换为小时、分钟
seconds = 3600
hours = seconds / 3600
minutes = seconds / 60
print(f"{seconds}秒等于{hours}小时或{minutes}分钟")
2、角度单位转换
Python的math
模块可以用于角度单位的转换,比如将度转换为弧度。
import math
度转换为弧度
degrees = 180
radians = math.radians(degrees)
print(f"{degrees}度等于{radians}弧度")
二、利用第三方库进行单位转换
有许多Python第三方库可以用于单位转换,比如pint
和unitconvert
。
1、使用Pint库
Pint
是一个非常强大的物理单位处理库,可以处理各种复杂的单位转换。
import pint
创建一个UnitRegistry对象
ureg = pint.UnitRegistry()
定义单位
distance = 10 * ureg.meter
time = 2 * ureg.second
单位转换
velocity = distance / time
velocity_in_kmph = velocity.to(ureg.kilometer / ureg.hour)
print(f"速度为{velocity_in_kmph}")
2、使用Unitconvert库
unitconvert
是另一个用于单位转换的库,使用起来也非常简单。
from unitconvert import convert, ureg
长度单位转换
meters = 100
feet = convert(meters, 'meters', 'feet')
print(f"{meters}米等于{feet}英尺")
质量单位转换
kilograms = 70
pounds = convert(kilograms, 'kilograms', 'pounds')
print(f"{kilograms}千克等于{pounds}磅")
三、编写自定义函数进行单位转换
如果需要转换的单位比较特殊,或者没有合适的第三方库,可以编写自定义函数来进行单位转换。
1、长度单位转换
def length_conversion(value, from_unit, to_unit):
conversion_factors = {
'meter': 1,
'kilometer': 1000,
'centimeter': 0.01,
'millimeter': 0.001,
'mile': 1609.34,
'yard': 0.9144,
'foot': 0.3048,
'inch': 0.0254
}
if from_unit not in conversion_factors or to_unit not in conversion_factors:
raise ValueError("Unsupported unit")
return value * conversion_factors[from_unit] / conversion_factors[to_unit]
示例
meters = 100
feet = length_conversion(meters, 'meter', 'foot')
print(f"{meters}米等于{feet}英尺")
2、质量单位转换
def weight_conversion(value, from_unit, to_unit):
conversion_factors = {
'kilogram': 1,
'gram': 0.001,
'milligram': 1e-6,
'pound': 0.453592,
'ounce': 0.0283495
}
if from_unit not in conversion_factors or to_unit not in conversion_factors:
raise ValueError("Unsupported unit")
return value * conversion_factors[from_unit] / conversion_factors[to_unit]
示例
kilograms = 70
pounds = weight_conversion(kilograms, 'kilogram', 'pound')
print(f"{kilograms}千克等于{pounds}磅")
3、温度单位转换
def temperature_conversion(value, from_unit, to_unit):
if from_unit == 'celsius':
if to_unit == 'fahrenheit':
return (value * 9/5) + 32
elif to_unit == 'kelvin':
return value + 273.15
elif from_unit == 'fahrenheit':
if to_unit == 'celsius':
return (value - 32) * 5/9
elif to_unit == 'kelvin':
return (value - 32) * 5/9 + 273.15
elif from_unit == 'kelvin':
if to_unit == 'celsius':
return value - 273.15
elif to_unit == 'fahrenheit':
return (value - 273.15) * 9/5 + 32
raise ValueError("Unsupported unit")
示例
celsius = 25
fahrenheit = temperature_conversion(celsius, 'celsius', 'fahrenheit')
print(f"{celsius}摄氏度等于{fahrenheit}华氏度")
四、总结
通过以上介绍,我们可以看到Python在进行单位转换时提供了多种方法,包括使用内置函数、第三方库和自定义函数。利用第三方库进行单位转换是最为方便和灵活的选择,比如Pint
和unitconvert
可以处理各种复杂的单位转换。如果没有合适的第三方库,也可以编写自定义函数来处理特定的单位转换需求。无论采用哪种方法,都可以根据具体需求灵活应用,以实现高效、准确的单位转换。
相关问答FAQs:
如何在Python中进行不同单位之间的转换?
Python提供了丰富的库和工具,可以轻松实现单位转换。例如,使用pint
库可以轻松管理和转换不同的物理单位。您只需定义单位并进行计算,pint
会自动处理转换。例如,如果需要将英里转换为公里,可以这样写:from pint import UnitRegistry; ureg = UnitRegistry(); distance = 5 * ureg.mile; distance.to(ureg.kilometer)
。这种方法使单位转换变得简单而直观。
在Python中,如何处理自定义单位的转换?
如果您需要处理特定的自定义单位,您可以创建一个转换函数。首先,定义每个单位与基本单位之间的关系,然后在函数中进行相应的计算。例如,可以定义一个将摄氏度转换为华氏度的函数。通过简单的数学公式,您可以轻松实现这一目标:def celsius_to_fahrenheit(celsius): return (celsius * 9/5) + 32
。这种方法为处理自定义单位提供了灵活性。
Python中是否有现成的库可以简化单位转换的过程?
确实存在多个库可以帮助简化单位转换过程。其中最受欢迎的包括pint
、quantities
和sympy
等。这些库不仅支持标准单位转换,还允许用户创建和使用自定义单位。通过这些库,您可以实现更复杂的计算和更便捷的单位管理。例如,sympy
提供符号计算功能,使得单位转换不仅限于数值计算,还可以处理符号表达式。选择合适的库可以大大提高工作效率。
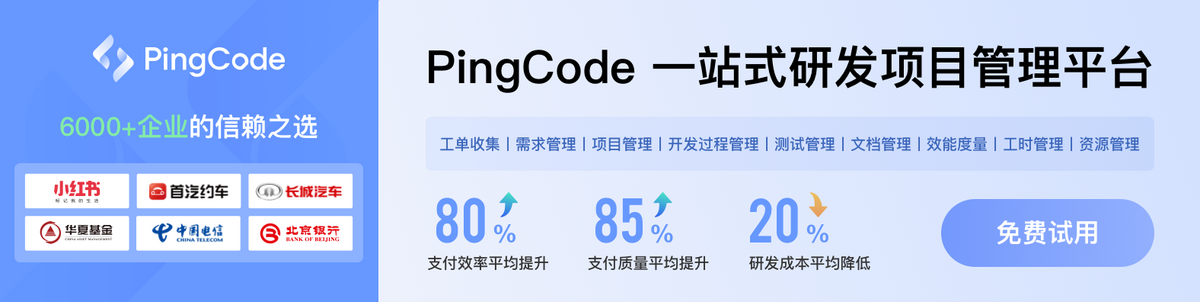