在Python中,定义类的函数(通常称为方法)时,需要在类的定义内使用def
关键字。类中的函数称为方法,方法的第一个参数通常是self
,它代表实例本身。在类中定义方法时,需要注意方法的作用域、参数传递、以及如何调用这些方法。以下是详细的解释和例子。
一、类的定义与方法
在Python中,使用class
关键字来定义类。类是面向对象编程的核心概念,它是对象的蓝图或模板。类中的方法是定义在类内部的函数,通常用于操作类的实例数据。
class MyClass:
def __init__(self, name):
self.name = name
def greet(self):
print(f"Hello, {self.name}!")
在上面的例子中,MyClass
是一个类,__init__
是一个特殊的方法,用于类的初始化,而greet
是一个普通方法,用于打印问候语。
二、__init__
方法
__init__
方法是一个特殊的初始化方法,当创建类的实例时会自动调用。__init__
方法用于初始化对象的属性。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def display_info(self):
print(f"Name: {self.name}, Age: {self.age}")
在上面的例子中,__init__
方法接受两个参数name
和age
,并将它们赋值给实例变量self.name
和self.age
。
三、实例方法
实例方法是类中最常见的方法,它们总是接受一个参数self
,用于访问实例的属性和其他方法。
class Animal:
def __init__(self, species):
self.species = species
def make_sound(self, sound):
print(f"The {self.species} says {sound}")
在这个例子中,make_sound
方法接受一个参数sound
,并输出该动物的叫声。
四、类方法和静态方法
除了实例方法,Python还支持类方法和静态方法。类方法使用@classmethod
装饰器定义,接受一个参数cls
,表示类本身。静态方法使用@staticmethod
装饰器定义,不接受特殊的第一个参数。
class MathOperations:
@classmethod
def add(cls, a, b):
return a + b
@staticmethod
def multiply(a, b):
return a * b
在上面的例子中,add
是一个类方法,multiply
是一个静态方法。
五、方法的调用
定义类和方法后,可以通过创建类的实例来调用这些方法。
person = Person("Alice", 30)
person.display_info()
animal = Animal("dog")
animal.make_sound("woof")
print(MathOperations.add(5, 3))
print(MathOperations.multiply(4, 2))
在这个例子中,创建了Person
和Animal
类的实例,并调用了它们的方法。同时,直接调用了MathOperations
的类方法和静态方法。
六、方法重载和多态
在面向对象编程中,方法重载和多态是两个重要概念。方法重载指的是在同一个类中定义多个方法,它们具有相同的名称但参数不同。多态则是指不同类的对象可以通过相同的接口访问。
方法重载
在Python中,不支持传统的函数重载,但可以通过默认参数来实现类似的效果。
class Shape:
def area(self, radius=None, length=None, breadth=None):
if radius is not None:
return 3.14 * radius * radius
elif length is not None and breadth is not None:
return length * breadth
else:
return "Invalid parameters"
在上面的例子中,area
方法可以接受不同的参数来计算不同形状的面积。
多态
多态允许你在不考虑对象具体类型的情况下调用方法。
class Dog:
def speak(self):
return "Woof!"
class Cat:
def speak(self):
return "Meow!"
def make_sound(animal):
print(animal.speak())
dog = Dog()
cat = Cat()
make_sound(dog)
make_sound(cat)
在上面的例子中,make_sound
函数可以接受Dog
和Cat
的实例,并调用它们的speak
方法。
七、访问控制
Python类中的属性和方法可以通过名称前加下划线或双下划线来设置访问控制。
- 单下划线
_
:表示属性或方法是受保护的,不建议外部访问。 - 双下划线
__
:表示属性或方法是私有的,不能在类外部直接访问。
class BankAccount:
def __init__(self, balance):
self.__balance = balance
def deposit(self, amount):
self.__balance += amount
def withdraw(self, amount):
if amount <= self.__balance:
self.__balance -= amount
else:
print("Insufficient funds")
def get_balance(self):
return self.__balance
在上面的例子中,__balance
是一个私有属性,不能在类外部直接访问,只能通过类的方法来修改和访问。
八、继承和方法重写
继承允许一个类(子类)继承另一个类(父类)的属性和方法。子类可以重写父类的方法。
class Vehicle:
def start(self):
print("Starting the vehicle")
class Car(Vehicle):
def start(self):
print("Starting the car")
car = Car()
car.start()
在上面的例子中,Car
类继承了Vehicle
类,并重写了start
方法。
九、抽象类和方法
抽象类和方法用于定义接口,不能直接实例化,需要子类实现抽象方法。
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius * self.radius
circle = Circle(5)
print(circle.area())
在上面的例子中,Shape
是一个抽象类,不能直接实例化,Circle
类继承了Shape
并实现了area
方法。
十、特殊方法与运算符重载
Python类还可以定义一些特殊方法来实现运算符重载,例如__add__
、__sub__
、__str__
等。
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __str__(self):
return f"Vector({self.x}, {self.y})"
v1 = Vector(2, 3)
v2 = Vector(4, 5)
print(v1 + v2)
在上面的例子中,Vector
类重载了__add__
方法来实现向量的加法,并重载了__str__
方法来定义对象的字符串表示。
十一、装饰器在类中的应用
装饰器是一种修改函数或方法行为的方式,可以应用于类的方法。常用的装饰器有@property
、@classmethod
和@staticmethod
。
class Temperature:
def __init__(self, celsius):
self._celsius = celsius
@property
def fahrenheit(self):
return self._celsius * 9 / 5 + 32
@fahrenheit.setter
def fahrenheit(self, value):
self._celsius = (value - 32) * 5 / 9
temp = Temperature(25)
print(temp.fahrenheit) # Output: 77.0
temp.fahrenheit = 86
print(temp._celsius) # Output: 30.0
在上面的例子中,@property
装饰器将fahrenheit
方法变成了属性,可以像访问属性一样访问它。
十二、类的组合和聚合
类的组合和聚合是将不同的类结合在一起使用的两种方式。组合表示类包含另一个类的实例,聚合表示类包含另一个类的引用。
class Engine:
def start(self):
print("Engine started")
class Car:
def __init__(self, engine):
self.engine = engine
def start(self):
self.engine.start()
print("Car started")
engine = Engine()
car = Car(engine)
car.start()
在上面的例子中,Car
类包含了Engine
类的实例,通过组合的方式实现了对Engine
类的使用。
十三、类的元编程
元编程是指在运行时操作类和对象。在Python中,元类可以用于控制类的创建和行为。
class Meta(type):
def __new__(cls, name, bases, dct):
print(f"Creating class {name}")
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=Meta):
pass
在上面的例子中,Meta
是一个元类,MyClass
使用了Meta
作为元类,在创建MyClass
时会输出创建信息。
十四、类的反射
反射是指在运行时动态地获取类的信息。Python提供了一些内置函数用于反射,例如getattr
、setattr
、hasattr
和delattr
。
class Person:
def __init__(self, name):
self.name = name
person = Person("Alice")
Get attribute
print(getattr(person, 'name')) # Output: Alice
Set attribute
setattr(person, 'name', 'Bob')
print(person.name) # Output: Bob
Check attribute
print(hasattr(person, 'name')) # Output: True
Delete attribute
delattr(person, 'name')
print(hasattr(person, 'name')) # Output: False
在上面的例子中,使用了反射函数来动态地获取、设置、检查和删除对象的属性。
十五、类的深拷贝和浅拷贝
拷贝是创建对象副本的过程。浅拷贝复制对象的引用,而深拷贝复制对象及其引用的对象。
import copy
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
point1 = Point(1, 2)
point2 = copy.copy(point1) # Shallow copy
point3 = copy.deepcopy(point1) # Deep copy
print(point1 is point2) # Output: False
print(point1 is point3) # Output: False
print(point1.x is point2.x) # Output: True
print(point1.x is point3.x) # Output: True
在上面的例子中,copy.copy
创建了对象的浅拷贝,而copy.deepcopy
创建了对象的深拷贝。
十六、类的序列化和反序列化
序列化是将对象转换为字节流的过程,反序列化是将字节流转换为对象的过程。Python提供了pickle
模块用于对象的序列化和反序列化。
import pickle
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person = Person("Alice", 30)
Serialize
with open('person.pkl', 'wb') as file:
pickle.dump(person, file)
Deserialize
with open('person.pkl', 'rb') as file:
loaded_person = pickle.load(file)
print(loaded_person.name) # Output: Alice
print(loaded_person.age) # Output: 30
在上面的例子中,使用pickle.dump
将对象序列化到文件中,使用pickle.load
从文件中反序列化对象。
十七、类的单例模式
单例模式是一种创建类的实例的设计模式,确保一个类只有一个实例。可以通过重写__new__
方法来实现单例模式。
class Singleton:
_instance = None
def __new__(cls, *args, kwargs):
if cls._instance is None:
cls._instance = super().__new__(cls, *args, kwargs)
return cls._instance
singleton1 = Singleton()
singleton2 = Singleton()
print(singleton1 is singleton2) # Output: True
在上面的例子中,Singleton
类通过重写__new__
方法实现了单例模式,确保只有一个实例。
十八、类的上下文管理器
上下文管理器用于管理资源的获取和释放。可以通过实现__enter__
和__exit__
方法来定义上下文管理器。
class FileManager:
def __init__(self, filename, mode):
self.filename = filename
self.mode = mode
def __enter__(self):
self.file = open(self.filename, self.mode)
return self.file
def __exit__(self, exc_type, exc_val, exc_tb):
self.file.close()
with FileManager('test.txt', 'w') as file:
file.write('Hello, world!')
在上面的例子中,FileManager
类实现了上下文管理器,确保文件在操作完成后自动关闭。
十九、类的接口实现
接口定义了类必须实现的一组方法。Python没有显式的接口关键字,可以通过抽象类来定义接口。
from abc import ABC, abstractmethod
class Drawable(ABC):
@abstractmethod
def draw(self):
pass
class Circle(Drawable):
def draw(self):
print("Drawing a circle")
class Square(Drawable):
def draw(self):
print("Drawing a square")
shapes = [Circle(), Square()]
for shape in shapes:
shape.draw()
在上面的例子中,Drawable
是一个接口,定义了一个抽象方法draw
,Circle
和Square
类实现了该接口。
二十、类的文档字符串
文档字符串用于为类和方法编写文档。可以使用三重引号"""
来定义文档字符串。
class Calculator:
"""
A simple calculator class.
"""
def add(self, a, b):
"""
Add two numbers.
:param a: First number
:param b: Second number
:return: Sum of a and b
"""
return a + b
calculator = Calculator()
print(calculator.add(2, 3)) # Output: 5
在上面的例子中,使用文档字符串为Calculator
类和add
方法编写了文档。
通过以上各个小标题的详细解释和示例代码,相信你已经对如何在Python类中定义和使用函数有了深入的了解。定义类中的函数时,首先要明确其用途,然后根据具体需求选择合适的函数类型(实例方法、类方法、静态方法等),并注意参数传递、访问控制、继承、多态等面向对象编程的原则。此外,合理使用装饰器、反射、序列化、单例模式、上下文管理器、接口等高级特性,可以使你的代码更加简洁、优雅和高效。
相关问答FAQs:
在Python类中定义函数时需要注意哪些关键点?
在Python中定义类时,函数通常称为方法。要在类中定义方法,首先需要使用def
关键字,接着定义方法名和参数列表。方法的第一个参数通常是self
,它指向类的实例,允许方法访问类的属性和其他方法。确保合理命名方法,以便于阅读和理解。
如何在Python类中调用其他方法?
在一个类中调用另一个方法时,可以直接使用self
来引用当前实例的方法。例如,在一个方法内部调用另一个方法时,可以使用self.method_name()
的形式。这样做可以增强代码的组织性和可读性,避免重复代码。
类中的方法可以有默认参数吗?
当然可以。在定义方法时,可以为参数指定默认值,这样在调用方法时可以选择性地传递参数。例如,def method_name(self, param1=default_value)
,在调用时如果不传递param1
,将使用指定的默认值。这种做法提升了方法的灵活性,允许用户在不同场景下使用相同的方法。
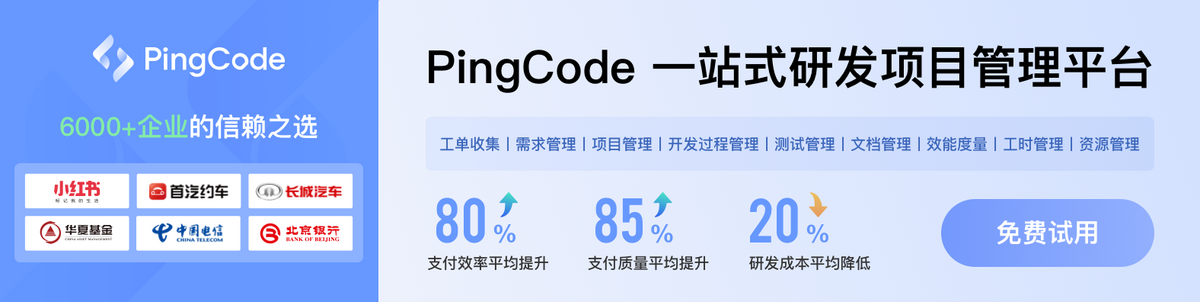