如何用Python编写圆形钟表: 可以通过使用Tkinter库、Pygame库、Matplotlib库、Turtle库中的一个来实现,本文将详细介绍如何使用Tkinter库来编写一个圆形钟表。
一、Tkinter库的概述
Tkinter是Python的标准GUI(图形用户界面)库。它提供了一种简便的方法来创建窗口、按钮、文本框等。利用Tkinter,我们可以很方便地绘制图形、添加各种控件,从而实现一个功能完备的圆形钟表。
二、实现圆形钟表的步骤
1、导入必要的库
首先,我们需要导入必要的库,包括Tkinter和time库。
import tkinter as tk
import time
import math
2、创建主窗口
使用Tkinter创建一个主窗口,并设置窗口的大小和标题。
root = tk.Tk()
root.title("圆形钟表")
root.geometry("400x400")
3、绘制钟表表盘
使用Canvas组件来绘制钟表的表盘。Canvas组件允许我们在窗口中绘制各种图形,如线条、椭圆、矩形等。
canvas = tk.Canvas(root, width=400, height=400, bg='white')
canvas.pack()
canvas.create_oval(50, 50, 350, 350, outline='black')
4、绘制时针、分针和秒针
使用Canvas组件的create_line方法来绘制时针、分针和秒针。我们需要根据当前时间来计算每个指针的角度,从而确定指针的终点坐标。
def draw_hand(length, angle, color):
x_center, y_center = 200, 200
x_end = x_center + length * math.cos(math.radians(angle))
y_end = y_center - length * math.sin(math.radians(angle))
canvas.create_line(x_center, y_center, x_end, y_end, fill=color, width=2)
def update_clock():
canvas.delete("all")
canvas.create_oval(50, 50, 350, 350, outline='black')
current_time = time.localtime()
hours = current_time.tm_hour % 12
minutes = current_time.tm_min
seconds = current_time.tm_sec
hour_angle = 360 * (hours / 12)
minute_angle = 360 * (minutes / 60)
second_angle = 360 * (seconds / 60)
draw_hand(50, hour_angle, 'black')
draw_hand(70, minute_angle, 'blue')
draw_hand(90, second_angle, 'red')
root.after(1000, update_clock)
update_clock()
三、运行程序
最后,我们需要进入Tkinter的主循环,运行程序。
root.mainloop()
四、总结
通过上述步骤,我们可以使用Tkinter库来编写一个简单的圆形钟表。Tkinter库提供了强大的绘图功能,使我们能够轻松地绘制各种图形,并通过定时器来实现动态更新。通过不断学习和实践,我们可以进一步优化和扩展这个钟表程序,如添加刻度、数字显示等功能。
五、附加功能
1、添加刻度
在钟表表盘上添加刻度,可以让钟表看起来更加真实和易读。我们可以使用Canvas组件的create_line方法来绘制刻度。
def draw_ticks():
for i in range(12):
angle = 360 * (i / 12)
x_start = 200 + 140 * math.cos(math.radians(angle))
y_start = 200 - 140 * math.sin(math.radians(angle))
x_end = 200 + 150 * math.cos(math.radians(angle))
y_end = 200 - 150 * math.sin(math.radians(angle))
canvas.create_line(x_start, y_start, x_end, y_end, fill='black', width=2)
draw_ticks()
2、添加数字显示
在钟表表盘上添加数字显示,可以让钟表更加直观。我们可以使用Canvas组件的create_text方法来绘制数字。
def draw_numbers():
for i in range(1, 13):
angle = 360 * (i / 12)
x = 200 + 120 * math.cos(math.radians(angle))
y = 200 - 120 * math.sin(math.radians(angle))
canvas.create_text(x, y, text=str(i), font=("Arial", 12, "bold"))
draw_numbers()
3、优化指针的绘制
为了让指针更加美观和准确,我们可以对指针的绘制进行优化,如调整指针的长度、宽度和颜色。
def draw_hand(length, angle, color, width):
x_center, y_center = 200, 200
x_end = x_center + length * math.cos(math.radians(angle))
y_end = y_center - length * math.sin(math.radians(angle))
canvas.create_line(x_center, y_center, x_end, y_end, fill=color, width=width)
def update_clock():
canvas.delete("all")
canvas.create_oval(50, 50, 350, 350, outline='black')
draw_ticks()
draw_numbers()
current_time = time.localtime()
hours = current_time.tm_hour % 12
minutes = current_time.tm_min
seconds = current_time.tm_sec
hour_angle = 360 * (hours / 12) - 90
minute_angle = 360 * (minutes / 60) - 90
second_angle = 360 * (seconds / 60) - 90
draw_hand(50, hour_angle, 'black', 4)
draw_hand(70, minute_angle, 'blue', 2)
draw_hand(90, second_angle, 'red', 1)
root.after(1000, update_clock)
update_clock()
六、其他实现方法
除了使用Tkinter库,我们还可以使用其他库来实现圆形钟表,如Pygame库、Matplotlib库和Turtle库。
1、使用Pygame库
Pygame是一个跨平台的Python模块,专门用于编写视频游戏。它包括计算机图形和声音库。使用Pygame库,我们可以创建一个更具互动性的钟表。
import pygame
import time
import math
pygame.init()
screen = pygame.display.set_mode((400, 400))
pygame.display.set_caption("圆形钟表")
def draw_hand(length, angle, color, width):
x_center, y_center = 200, 200
x_end = x_center + length * math.cos(math.radians(angle))
y_end = y_center - length * math.sin(math.radians(angle))
pygame.draw.line(screen, color, (x_center, y_center), (x_end, y_end), width)
def draw_ticks():
for i in range(12):
angle = 360 * (i / 12)
x_start = 200 + 140 * math.cos(math.radians(angle))
y_start = 200 - 140 * math.sin(math.radians(angle))
x_end = 200 + 150 * math.cos(math.radians(angle))
y_end = 200 - 150 * math.sin(math.radians(angle))
pygame.draw.line(screen, (0, 0, 0), (x_start, y_start), (x_end, y_end), 2)
def draw_numbers():
font = pygame.font.SysFont("Arial", 24, True)
for i in range(1, 13):
angle = 360 * (i / 12)
x = 200 + 120 * math.cos(math.radians(angle))
y = 200 - 120 * math.sin(math.radians(angle))
text = font.render(str(i), True, (0, 0, 0))
screen.blit(text, (x - text.get_width() // 2, y - text.get_height() // 2))
def update_clock():
screen.fill((255, 255, 255))
pygame.draw.circle(screen, (0, 0, 0), (200, 200), 150, 2)
draw_ticks()
draw_numbers()
current_time = time.localtime()
hours = current_time.tm_hour % 12
minutes = current_time.tm_min
seconds = current_time.tm_sec
hour_angle = 360 * (hours / 12) - 90
minute_angle = 360 * (minutes / 60) - 90
second_angle = 360 * (seconds / 60) - 90
draw_hand(50, hour_angle, (0, 0, 0), 4)
draw_hand(70, minute_angle, (0, 0, 255), 2)
draw_hand(90, second_angle, (255, 0, 0), 1)
pygame.display.flip()
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
update_clock()
pygame.time.delay(1000)
pygame.quit()
2、使用Matplotlib库
Matplotlib是一个Python的2D绘图库,能够生成各种图表和图形。使用Matplotlib库,我们可以绘制一个静态的钟表图形。
import matplotlib.pyplot as plt
import math
import time
fig, ax = plt.subplots()
ax.set_xlim(-1.5, 1.5)
ax.set_ylim(-1.5, 1.5)
def draw_hand(length, angle, color, width):
x_end = length * math.cos(math.radians(angle))
y_end = length * math.sin(math.radians(angle))
ax.plot([0, x_end], [0, y_end], color=color, linewidth=width)
def draw_ticks():
for i in range(12):
angle = 360 * (i / 12)
x_start = 1.3 * math.cos(math.radians(angle))
y_start = 1.3 * math.sin(math.radians(angle))
x_end = 1.5 * math.cos(math.radians(angle))
y_end = 1.5 * math.sin(math.radians(angle))
ax.plot([x_start, x_end], [y_start, y_end], color='black', linewidth=2)
def draw_numbers():
for i in range(1, 13):
angle = 360 * (i / 12)
x = 1.1 * math.cos(math.radians(angle))
y = 1.1 * math.sin(math.radians(angle))
ax.text(x, y, str(i), fontsize=12, ha='center', va='center')
draw_ticks()
draw_numbers()
current_time = time.localtime()
hours = current_time.tm_hour % 12
minutes = current_time.tm_min
seconds = current_time.tm_sec
hour_angle = 360 * (hours / 12) - 90
minute_angle = 360 * (minutes / 60) - 90
second_angle = 360 * (seconds / 60) - 90
draw_hand(0.5, hour_angle, 'black', 4)
draw_hand(0.7, minute_angle, 'blue', 2)
draw_hand(0.9, second_angle, 'red', 1)
plt.show()
3、使用Turtle库
Turtle是Python内置的一个简单的绘图模块,常用于教学目的。使用Turtle库,我们可以创建一个简单的动画钟表。
import turtle
import time
import math
screen = turtle.Screen()
screen.title("圆形钟表")
screen.setup(width=400, height=400)
screen.tracer(0)
clock = turtle.Turtle()
clock.hideturtle()
clock.speed(0)
def draw_hand(length, angle, color, width):
clock.penup()
clock.goto(0, 0)
clock.setheading(90 - angle)
clock.pendown()
clock.pensize(width)
clock.pencolor(color)
clock.forward(length)
def draw_ticks():
for i in range(12):
angle = 360 * (i / 12)
clock.penup()
clock.goto(0, 0)
clock.setheading(90 - angle)
clock.forward(140)
clock.pendown()
clock.forward(10)
def draw_numbers():
for i in range(1, 13):
angle = 360 * (i / 12)
x = 120 * math.cos(math.radians(angle - 90))
y = 120 * math.sin(math.radians(angle - 90))
clock.penup()
clock.goto(x, y)
clock.write(str(i), align="center", font=("Arial", 12, "bold"))
draw_ticks()
draw_numbers()
def update_clock():
clock.clear()
draw_ticks()
draw_numbers()
current_time = time.localtime()
hours = current_time.tm_hour % 12
minutes = current_time.tm_min
seconds = current_time.tm_sec
hour_angle = 360 * (hours / 12)
minute_angle = 360 * (minutes / 60)
second_angle = 360 * (seconds / 60)
draw_hand(50, hour_angle, 'black', 4)
draw_hand(70, minute_angle, 'blue', 2)
draw_hand(90, second_angle, 'red', 1)
screen.update()
screen.ontimer(update_clock, 1000)
update_clock()
turtle.mainloop()
七、总结
通过本文,我们详细介绍了如何使用Python的Tkinter库来编写一个圆形钟表,并且还介绍了使用Pygame、Matplotlib和Turtle库来实现相同功能的方法。每种方法都有其独特的优点和适用场景,读者可以根据自己的需求选择合适的方法。希望本文对你有所帮助,让你在Python图形编程方面有所收获。
相关问答FAQs:
如何在Python中绘制一个圆形钟表的基本结构?
要绘制一个圆形钟表的基本结构,可以使用Python的图形库,比如Tkinter或Pygame。首先,设置一个圆形的背景,并在适当的位置绘制时针、分针和秒针。可以通过计算当前时间并相应地旋转这些指针来实现动态效果。
有哪些Python库可以帮助我创建图形界面和动画效果?
在Python中,有几个流行的库可以帮助创建图形界面和动画效果。例如,Tkinter是Python自带的库,适合简单的图形界面设计。Pygame则更适合需要复杂动画和游戏开发的项目。Matplotlib也是一个不错的选择,可以绘制静态和动态的图形。
如何使钟表的指针根据系统时间动态更新?
要使钟表的指针动态更新,您可以使用Python的时间模块来获取当前时间。通过定时器或循环,定期更新指针的角度,并重绘钟表。这种方式可以确保钟表显示的时间始终与系统时间同步,从而实现一个实用的动态时钟。
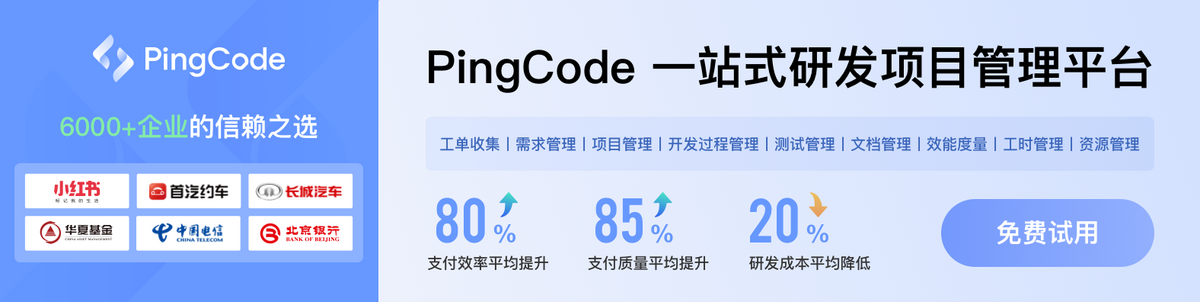