通过使用C语言的库、函数和结构体、指针等功能,可以实现Python的一些功能。 例如,Python的动态内存管理可以通过C中的malloc和free函数实现,Python的高级数据结构如列表、字典等可以通过C语言中的结构体和链表实现。接下来,我们将详细介绍如何在C语言中实现这些功能。
一、动态内存管理
在Python中,内存管理是由解释器处理的,开发者不需要手动管理内存。然而,在C语言中,动态内存管理是通过malloc和free函数来实现的。
1、使用malloc和free进行内存分配和释放
#include <stdio.h>
#include <stdlib.h>
int main() {
int *arr;
int n, i;
printf("Enter number of elements: ");
scanf("%d", &n);
// 动态分配内存
arr = (int*)malloc(n * sizeof(int));
// 检查内存是否分配成功
if (arr == NULL) {
printf("Memory not allocated.\n");
exit(0);
}
// 初始化内存并打印
for (i = 0; i < n; ++i) {
arr[i] = i + 1;
}
printf("The elements of the array are: ");
for (i = 0; i < n; ++i) {
printf("%d ", arr[i]);
}
// 释放内存
free(arr);
return 0;
}
二、数据结构
Python中有许多高级数据结构,如列表、字典、集合等。在C语言中,我们可以通过结构体和链表来实现这些数据结构。
1、实现链表
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点
struct Node {
int data;
struct Node* next;
};
// 在链表末尾添加新节点
void append(struct Node head_ref, int new_data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
struct Node* last = *head_ref;
new_node->data = new_data;
new_node->next = NULL;
if (*head_ref == NULL) {
*head_ref = new_node;
return;
}
while (last->next != NULL) {
last = last->next;
}
last->next = new_node;
return;
}
// 打印链表
void printList(struct Node* node) {
while (node != NULL) {
printf("%d -> ", node->data);
node = node->next;
}
printf("NULL\n");
}
int main() {
struct Node* head = NULL;
append(&head, 1);
append(&head, 2);
append(&head, 3);
append(&head, 4);
printList(head);
return 0;
}
2、实现字典(哈希表)
在C中实现字典通常使用哈希表。下面是一个简单的哈希表实现:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 10
typedef struct Entry {
char* key;
char* value;
struct Entry* next;
} Entry;
typedef struct {
Entry entries;
} HashTable;
// 哈希函数
unsigned int hash(const char* key) {
unsigned long int value = 0;
unsigned int i = 0;
unsigned int key_len = strlen(key);
for (; i < key_len; ++i) {
value = value * 37 + key[i];
}
value = value % TABLE_SIZE;
return value;
}
// 创建哈希表
HashTable* create_table() {
HashTable* table = (HashTable*)malloc(sizeof(HashTable));
table->entries = (Entry)malloc(sizeof(Entry*) * TABLE_SIZE);
for (int i = 0; i < TABLE_SIZE; ++i) {
table->entries[i] = NULL;
}
return table;
}
// 创建条目
Entry* create_entry(const char* key, const char* value) {
Entry* entry = (Entry*)malloc(sizeof(Entry));
entry->key = strdup(key);
entry->value = strdup(value);
entry->next = NULL;
return entry;
}
// 插入条目
void ht_insert(HashTable* table, const char* key, const char* value) {
unsigned int slot = hash(key);
Entry* entry = table->entries[slot];
if (entry == NULL) {
table->entries[slot] = create_entry(key, value);
return;
}
Entry* prev;
while (entry != NULL) {
if (strcmp(entry->key, key) == 0) {
free(entry->value);
entry->value = strdup(value);
return;
}
prev = entry;
entry = entry->next;
}
prev->next = create_entry(key, value);
}
// 查找条目
char* ht_search(HashTable* table, const char* key) {
unsigned int slot = hash(key);
Entry* entry = table->entries[slot];
while (entry != NULL) {
if (strcmp(entry->key, key) == 0) {
return entry->value;
}
entry = entry->next;
}
return NULL;
}
// 删除条目
void ht_delete(HashTable* table, const char* key) {
unsigned int slot = hash(key);
Entry* entry = table->entries[slot];
if (entry == NULL) {
return;
}
Entry* prev;
while (entry != NULL) {
if (strcmp(entry->key, key) == 0) {
if (prev == NULL) {
table->entries[slot] = entry->next;
} else {
prev->next = entry->next;
}
free(entry->key);
free(entry->value);
free(entry);
return;
}
prev = entry;
entry = entry->next;
}
}
int main() {
HashTable* ht = create_table();
ht_insert(ht, "name", "Alice");
ht_insert(ht, "age", "25");
ht_insert(ht, "city", "New York");
printf("name: %s\n", ht_search(ht, "name"));
printf("age: %s\n", ht_search(ht, "age"));
printf("city: %s\n", ht_search(ht, "city"));
ht_delete(ht, "age");
printf("age after deletion: %s\n", ht_search(ht, "age"));
return 0;
}
三、字符串操作
Python中的字符串操作非常方便,而在C中需要使用标准库函数来进行字符串操作。
1、字符串复制和连接
#include <stdio.h>
#include <string.h>
int main() {
char str1[100] = "Hello";
char str2[100] = "World";
char str3[100];
// 字符串复制
strcpy(str3, str1);
printf("str3: %s\n", str3);
// 字符串连接
strcat(str1, str2);
printf("str1: %s\n", str1);
return 0;
}
2、字符串长度和比较
#include <stdio.h>
#include <string.h>
int main() {
char str1[100] = "Hello";
char str2[100] = "World";
// 字符串长度
printf("Length of str1: %lu\n", strlen(str1));
printf("Length of str2: %lu\n", strlen(str2));
// 字符串比较
if (strcmp(str1, str2) == 0) {
printf("str1 and str2 are equal\n");
} else {
printf("str1 and str2 are not equal\n");
}
return 0;
}
四、文件操作
Python提供了非常方便的文件操作接口,在C中,文件操作是通过标准库函数来实现的。
1、文件读取和写入
#include <stdio.h>
int main() {
FILE *fp;
char buffer[255];
// 写入文件
fp = fopen("test.txt", "w");
fprintf(fp, "Hello, World!\n");
fclose(fp);
// 读取文件
fp = fopen("test.txt", "r");
while (fgets(buffer, 255, fp) != NULL) {
printf("%s", buffer);
}
fclose(fp);
return 0;
}
五、异常处理
Python提供了异常处理机制,通过try-except块来捕获和处理异常。在C中,可以使用返回值和全局错误变量来实现类似的功能。
1、使用返回值进行错误处理
#include <stdio.h>
#include <stdlib.h>
int divide(int a, int b, int *result) {
if (b == 0) {
return -1; // 错误代码
}
*result = a / b;
return 0; // 成功代码
}
int main() {
int a = 10, b = 0;
int result;
if (divide(a, b, &result) != 0) {
printf("Error: Division by zero\n");
} else {
printf("Result: %d\n", result);
}
return 0;
}
六、面向对象编程
Python是一种面向对象的语言,可以非常方便地定义类和对象。在C中,可以通过结构体和函数指针来模拟面向对象编程。
1、定义类和对象
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义类
typedef struct {
char name[50];
int age;
void (*print)(struct Person*);
} Person;
// 定义成员函数
void print_person(Person* p) {
printf("Name: %s, Age: %d\n", p->name, p->age);
}
// 创建对象
Person* create_person(const char* name, int age) {
Person* p = (Person*)malloc(sizeof(Person));
strcpy(p->name, name);
p->age = age;
p->print = print_person;
return p;
}
int main() {
// 创建对象并调用成员函数
Person* p = create_person("Alice", 25);
p->print(p);
free(p);
return 0;
}
七、多线程编程
Python的多线程编程可以通过threading模块实现。在C中,可以使用pthread库来实现多线程编程。
1、创建和管理线程
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void* thread_func(void* arg) {
int* num = (int*)arg;
printf("Thread %d is running\n", *num);
return NULL;
}
int main() {
pthread_t threads[5];
int thread_args[5];
for (int i = 0; i < 5; ++i) {
thread_args[i] = i;
pthread_create(&threads[i], NULL, thread_func, (void*)&thread_args[i]);
}
for (int i = 0; i < 5; ++i) {
pthread_join(threads[i], NULL);
}
return 0;
}
八、网络编程
Python的网络编程可以通过socket模块实现。在C中,可以使用socket库来进行网络编程。
1、实现简单的TCP服务器和客户端
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <arpa/inet.h>
#define PORT 8080
void error(const char *msg) {
perror(msg);
exit(1);
}
int main() {
int sockfd, newsockfd;
struct sockaddr_in serv_addr, cli_addr;
socklen_t clilen;
char buffer[256];
// 创建套接字
sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
error("ERROR opening socket");
}
// 初始化服务器地址结构
memset((char*)&serv_addr, 0, sizeof(serv_addr));
serv_addr.sin_family = AF_INET;
serv_addr.sin_addr.s_addr = INADDR_ANY;
serv_addr.sin_port = htons(PORT);
// 绑定套接字
if (bind(sockfd, (struct sockaddr*)&serv_addr, sizeof(serv_addr)) < 0) {
error("ERROR on binding");
}
// 监听连接
listen(sockfd, 5);
clilen = sizeof(cli_addr);
// 接受连接
newsockfd = accept(sockfd, (struct sockaddr*)&cli_addr, &clilen);
if (newsockfd < 0) {
error("ERROR on accept");
}
// 读取和发送数据
memset(buffer, 0, 256);
read(newsockfd, buffer, 255);
printf("Here is the message: %s\n", buffer);
write(newsockfd, "I got your message", 18);
close(newsockfd);
close(sockfd);
return 0;
}
九、图形用户界面(GUI)编程
Python的GUI编程可以通过Tkinter、PyQt等库实现。在C中,可以使用GTK、Qt等库进行GUI编程。
1、使用GTK创建简单的窗口
#include <gtk/gtk.h>
void hello(GtkWidget *widget, gpointer data) {
g_print("Hello, World!\n");
}
int main(int argc, char *argv[]) {
GtkWidget *window;
GtkWidget *button;
gtk_init(&argc, &argv);
window = gtk_window_new(GTK_WINDOW_TOPLEVEL);
g_signal_connect(window, "destroy", G_CALLBACK(gtk_main_quit), NULL);
gtk_container_set_border_width(GTK_CONTAINER(window), 10);
button = gtk_button_new_with_label("Hello, World!");
g_signal_connect(button, "clicked", G_CALLBACK(hello), NULL);
gtk_container_add(GTK_CONTAINER(window), button);
gtk_widget_show_all(window);
gtk_main();
return 0;
}
十、正则表达式
Python的正则表达式可以通过re模块实现。在C中,可以使用POSIX正则表达式库进行正则表达式操作。
1、使用POSIX正则表达式库
#include <stdio.h>
#include <stdlib.h>
#include <regex.h>
int main() {
regex_t regex;
int ret;
char msgbuf[100];
// 编译正则表达式
ret = regcomp(®ex, "^[a-zA-Z0-9_]+$", 0);
if (ret) {
fprintf(stderr, "Could not compile regex\n");
exit(1);
}
// 执行正则表达式
ret = regexec(®ex, "example_123", 0, NULL, 0);
if (!ret) {
printf("Match\n");
} else if (ret == REG_NOMATCH) {
printf("No match\n");
} else {
regerror(ret, ®ex, msgbuf, sizeof(msgbuf));
fprintf(stderr, "Regex match failed: %s\n", msgbuf);
exit(1);
}
// 释放正则表达式
regfree(®ex);
return 0;
}
十一、单元测试
Python的单元测试可以通过unittest模块实现。在C中,可以使用CUnit等库进行单元测试。
1、使用CUnit进行单元测试
#include <CUnit/CUnit.h>
#include <CUnit/Basic.h>
void test_example() {
CU_ASSERT(2 + 2 == 4);
CU_ASSERT(2 * 2 == 4);
}
int main() {
CU_initialize_registry();
CU_pSuite suite = CU_add_suite("Example Suite", 0, 0);
CU_add_test(suite, "test_example", test_example);
CU_basic_set_mode(CU_BRM_VERBOSE);
CU_basic_run_tests();
CU_cleanup_registry();
return 0;
}
总结
通过上述内容,我们可以看到,虽然Python提供了丰富的内置功能和库,但这些功能在C语言中同样可以实现。通过使用C语言的库、函数和结构体等特性,我们可以实现Python中的动态内存管理、高级数据结构、字符串操作、文件操作、异常处理、面向对象编程、多线程编程、网络编程、GUI编程、正则表达式和单元测试等功能。
在实际开发中,选择何种语言取决于具体的需求和场景。C语言由于其高效性和对底层硬件的控制能力,适用于系统编程、嵌入式系统等领域。而Python由于其简洁易用和丰富的库支持,更适合快速开发、数据分析和人工智能等领域。
相关问答FAQs:
如何在C语言中实现Python中的数据结构功能?
在C语言中,可以通过定义结构体来模拟Python中的数据结构。比如,使用结构体来实现列表、字典等。对于列表,可以使用动态数组来管理元素;对于字典,则可以使用哈希表。这样可以在C中实现类似于Python的灵活性和功能。
C语言与Python的性能比较如何?
C语言通常具有更高的性能,因为它是编译语言,而Python是解释语言。这意味着C代码在运行时更快,尤其是在计算密集型任务中。然而,Python的开发速度更快,适合原型开发和快速迭代。选择哪种语言取决于具体的项目需求和性能考量。
在C中实现Python的异常处理机制是否可行?
虽然C语言没有内置的异常处理机制,但可以通过使用错误码和条件语句来模拟异常处理。开发者可以通过定义特定的错误码,检查函数返回值,并在发生错误时采取相应的处理措施。这种方式虽然不如Python的try-except机制直观,但仍然能有效管理错误。
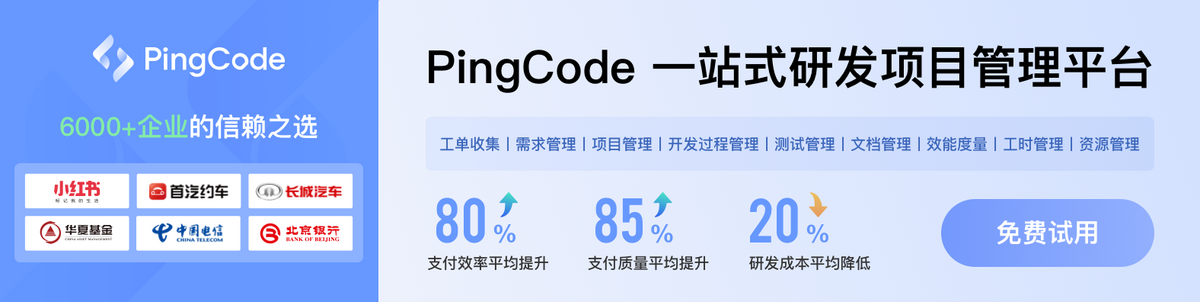