Python可以通过使用随机库、类、以及字典等多种方法自动随机生成属性,其中最常见的方法包括:使用random
库生成随机数、使用choice
从列表中随机选择、以及结合类和字典结构自动生成随机属性。下面将详细介绍如何通过这些方法来实现自动随机生成属性。
一、使用random
库生成随机数
random
库是Python标准库中的一个模块,提供了生成随机数的功能。通过使用random
库,可以生成随机整数、浮点数以及其他随机值。
1. 随机生成整数
使用random.randint(a, b)
方法可以生成一个范围在a
到b
之间的随机整数。
import random
class RandomAttributes:
def __init__(self):
self.attribute1 = random.randint(1, 100)
self.attribute2 = random.randint(200, 300)
示例
obj = RandomAttributes()
print(obj.attribute1)
print(obj.attribute2)
2. 随机生成浮点数
使用random.uniform(a, b)
方法可以生成一个范围在a
到b
之间的随机浮点数。
import random
class RandomAttributes:
def __init__(self):
self.attribute1 = random.uniform(1.0, 10.0)
self.attribute2 = random.uniform(20.0, 30.0)
示例
obj = RandomAttributes()
print(obj.attribute1)
print(obj.attribute2)
3. 随机生成布尔值
使用random.choice([True, False])
方法可以随机生成布尔值。
import random
class RandomAttributes:
def __init__(self):
self.attribute1 = random.choice([True, False])
self.attribute2 = random.choice([True, False])
示例
obj = RandomAttributes()
print(obj.attribute1)
print(obj.attribute2)
二、使用choice
方法从列表中随机选择
random.choice
方法可以从一个列表中随机选择一个元素,这在需要从一组预定义值中随机选择时非常有用。
1. 随机选择字符串
import random
class RandomAttributes:
def __init__(self):
self.attribute1 = random.choice(['red', 'blue', 'green', 'yellow'])
self.attribute2 = random.choice(['apple', 'banana', 'cherry', 'date'])
示例
obj = RandomAttributes()
print(obj.attribute1)
print(obj.attribute2)
2. 随机选择自定义对象
可以使用random.choice
方法从一个包含自定义对象的列表中随机选择。
import random
class CustomObject:
def __init__(self, name):
self.name = name
class RandomAttributes:
def __init__(self):
self.attribute1 = random.choice([CustomObject('A'), CustomObject('B'), CustomObject('C')])
self.attribute2 = random.choice([CustomObject('X'), CustomObject('Y'), CustomObject('Z')])
示例
obj = RandomAttributes()
print(obj.attribute1.name)
print(obj.attribute2.name)
三、结合类和字典自动生成随机属性
通过结合类和字典,可以更灵活地自动生成随机属性,特别是当属性种类多样且数量较多时。
1. 使用字典存储属性
可以使用字典来存储属性,并通过随机方法为这些属性赋值。
import random
class RandomAttributes:
def __init__(self):
self.attributes = {
'attribute1': random.randint(1, 100),
'attribute2': random.uniform(10.0, 20.0),
'attribute3': random.choice(['alpha', 'beta', 'gamma']),
'attribute4': random.choice([True, False])
}
示例
obj = RandomAttributes()
for attr, value in obj.attributes.items():
print(f"{attr}: {value}")
2. 动态添加属性
使用setattr
方法可以动态地为对象添加属性。
import random
class RandomAttributes:
def __init__(self):
attributes = {
'attribute1': random.randint(1, 100),
'attribute2': random.uniform(10.0, 20.0),
'attribute3': random.choice(['alpha', 'beta', 'gamma']),
'attribute4': random.choice([True, False])
}
for attr, value in attributes.items():
setattr(self, attr, value)
示例
obj = RandomAttributes()
print(obj.attribute1)
print(obj.attribute2)
print(obj.attribute3)
print(obj.attribute4)
四、结合生成器和装饰器生成随机属性
生成器和装饰器是Python中非常强大的工具,通过结合它们,可以更优雅地生成随机属性。
1. 使用生成器生成随机属性
生成器是一种特殊的迭代器,可以使用yield
关键字来逐个生成属性值。
import random
def attribute_generator():
while True:
yield random.randint(1, 100)
yield random.uniform(10.0, 20.0)
yield random.choice(['alpha', 'beta', 'gamma'])
yield random.choice([True, False])
class RandomAttributes:
def __init__(self, generator):
self.attribute1 = next(generator)
self.attribute2 = next(generator)
self.attribute3 = next(generator)
self.attribute4 = next(generator)
示例
gen = attribute_generator()
obj = RandomAttributes(gen)
print(obj.attribute1)
print(obj.attribute2)
print(obj.attribute3)
print(obj.attribute4)
2. 使用装饰器生成随机属性
装饰器是一种高阶函数,可以用来修改或扩展函数的行为。
import random
def random_attribute_decorator(func):
def wrapper(*args, kwargs):
obj = func(*args, kwargs)
obj.attribute1 = random.randint(1, 100)
obj.attribute2 = random.uniform(10.0, 20.0)
obj.attribute3 = random.choice(['alpha', 'beta', 'gamma'])
obj.attribute4 = random.choice([True, False])
return obj
return wrapper
@random_attribute_decorator
class RandomAttributes:
def __init__(self):
pass
示例
obj = RandomAttributes()
print(obj.attribute1)
print(obj.attribute2)
print(obj.attribute3)
print(obj.attribute4)
五、实际应用中的场景
在实际应用中,自动生成随机属性的需求非常广泛,包括测试数据生成、游戏开发、模拟仿真等。
1. 测试数据生成
在测试过程中,可能需要生成大量的随机数据来测试系统的性能和稳定性。
import random
def generate_test_data(num_samples):
test_data = []
for _ in range(num_samples):
sample = {
'id': random.randint(1000, 9999),
'name': random.choice(['Alice', 'Bob', 'Charlie', 'Dave']),
'score': random.uniform(0.0, 100.0)
}
test_data.append(sample)
return test_data
示例
data = generate_test_data(5)
for d in data:
print(d)
2. 游戏开发
在游戏开发中,通常需要生成随机的游戏角色属性。
import random
class GameCharacter:
def __init__(self):
self.health = random.randint(50, 100)
self.strength = random.randint(10, 20)
self.dexterity = random.randint(5, 15)
self.intelligence = random.randint(1, 10)
示例
character = GameCharacter()
print(f"Health: {character.health}")
print(f"Strength: {character.strength}")
print(f"Dexterity: {character.dexterity}")
print(f"Intelligence: {character.intelligence}")
3. 模拟仿真
在模拟仿真中,可以通过自动生成随机属性来模拟真实世界中的随机事件。
import random
class WeatherSimulation:
def __init__(self):
self.temperature = random.uniform(-10.0, 40.0)
self.humidity = random.uniform(0.0, 100.0)
self.wind_speed = random.uniform(0.0, 20.0)
示例
weather = WeatherSimulation()
print(f"Temperature: {weather.temperature}°C")
print(f"Humidity: {weather.humidity}%")
print(f"Wind Speed: {weather.wind_speed} m/s")
六、进一步优化和扩展
在实际应用中,可以根据具体需求进一步优化和扩展自动生成随机属性的方法。
1. 使用配置文件
可以将属性的生成规则存储在配置文件中,便于管理和修改。
import random
import json
def load_config(file_path):
with open(file_path, 'r') as file:
return json.load(file)
class RandomAttributes:
def __init__(self, config):
self.attribute1 = random.randint(config['attribute1']['min'], config['attribute1']['max'])
self.attribute2 = random.uniform(config['attribute2']['min'], config['attribute2']['max'])
self.attribute3 = random.choice(config['attribute3']['choices'])
self.attribute4 = random.choice(config['attribute4']['choices'])
示例
config = load_config('config.json')
obj = RandomAttributes(config)
print(obj.attribute1)
print(obj.attribute2)
print(obj.attribute3)
print(obj.attribute4)
2. 使用工厂模式
通过使用工厂模式,可以更灵活地生成不同类型的对象及其属性。
import random
class RandomAttributesFactory:
@staticmethod
def create(type_):
if type_ == 'type1':
return {
'attribute1': random.randint(1, 50),
'attribute2': random.uniform(5.0, 15.0),
'attribute3': random.choice(['a', 'b', 'c'])
}
elif type_ == 'type2':
return {
'attribute1': random.randint(51, 100),
'attribute2': random.uniform(15.0, 25.0),
'attribute3': random.choice(['x', 'y', 'z'])
}
else:
raise ValueError("Unknown type")
示例
obj1 = RandomAttributesFactory.create('type1')
obj2 = RandomAttributesFactory.create('type2')
print(obj1)
print(obj2)
通过以上方法,可以在Python中灵活地自动随机生成属性,满足不同应用场景的需求。无论是简单的随机数生成,还是复杂的对象属性管理,都可以通过合理的设计和实现,达到自动化和高效化的目标。
相关问答FAQs:
1. 如何使用Python生成随机属性的字典?
可以使用Python的random
模块结合字典生成器来创建随机属性。首先,定义属性名称及其可能的值,然后利用循环和随机选择函数为每个属性赋值。示例代码如下:
import random
attributes = {
'color': ['red', 'blue', 'green', 'yellow'],
'size': ['small', 'medium', 'large'],
'shape': ['circle', 'square', 'triangle']
}
random_attributes = {key: random.choice(value) for key, value in attributes.items()}
print(random_attributes)
以上代码将输出一个随机选择的属性字典。
2. 能否使用类和对象来生成随机属性?
当然可以!通过定义一个类并在初始化时生成随机属性,可以实现对象的随机化。可以在类中使用__init__
方法设置默认属性值,并在创建对象时随机生成属性。下面是一个简单的示例:
import random
class RandomObject:
def __init__(self):
self.color = random.choice(['red', 'blue', 'green', 'yellow'])
self.size = random.choice(['small', 'medium', 'large'])
self.shape = random.choice(['circle', 'square', 'triangle'])
random_instance = RandomObject()
print(vars(random_instance))
这个例子展示了如何创建一个包含随机属性的对象。
3. 如何生成特定类型的随机属性,例如随机姓名或地址?
可以通过使用第三方库如Faker
来生成更复杂的随机属性。Faker
库允许用户生成虚拟数据,包括姓名、地址、公司名称等。使用此库非常简单,只需安装并调用即可。以下是一个使用Faker
生成随机姓名和地址的示例:
from faker import Faker
fake = Faker()
random_name = fake.name()
random_address = fake.address()
print(f"Name: {random_name}")
print(f"Address: {random_address}")
通过这种方式,可以快速生成各种类型的随机属性,适合用于测试或模拟数据生成。
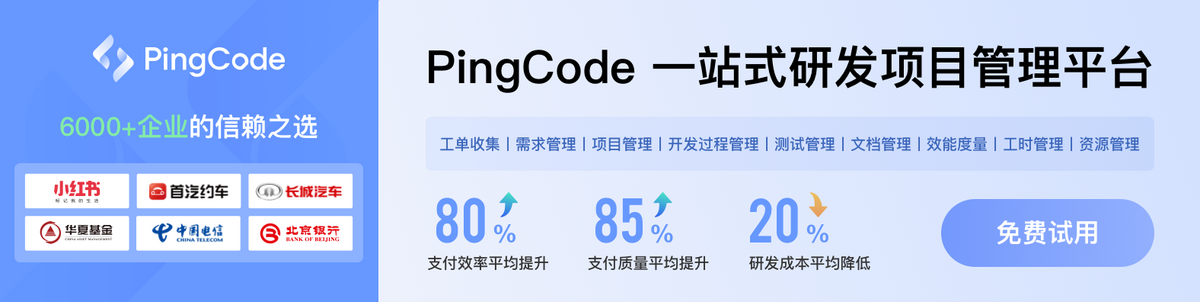