Python实现前后端交互的方式主要有以下几种:使用Flask或Django框架、通过RESTful API、使用WebSocket、结合前端框架如React或Vue进行完整开发。其中,通过RESTful API进行前后端交互是一种常见且高效的方式。
一、使用Flask框架实现前后端交互
Flask是一个轻量级的Python Web框架,非常适合小型应用和快速开发。它可以很方便地创建RESTful API,并与前端进行交互。
1、安装Flask
首先,确保你已经安装了Flask。你可以使用pip来安装:
pip install Flask
2、创建一个简单的Flask应用
下面是一个简单的Flask应用示例:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/api/data', methods=['GET'])
def get_data():
sample_data = {
"name": "John",
"age": 30,
"city": "New York"
}
return jsonify(sample_data)
@app.route('/api/data', methods=['POST'])
def post_data():
data = request.json
response = {
"status": "success",
"data_received": data
}
return jsonify(response)
if __name__ == '__main__':
app.run(debug=True)
在这个示例中,我们创建了两个API端点,一个用于GET请求,另一个用于POST请求。
3、前端调用Flask API
前端可以使用任何现代的JavaScript框架(如React、Vue、Angular)或纯JavaScript来调用这些API端点。以下是使用纯JavaScript的示例:
<!DOCTYPE html>
<html>
<head>
<title>Flask API Example</title>
</head>
<body>
<h1>Flask API Example</h1>
<button onclick="fetchData()">Get Data</button>
<button onclick="sendData()">Send Data</button>
<script>
function fetchData() {
fetch('http://127.0.0.1:5000/api/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
}
function sendData() {
const data = {
name: 'Jane',
age: 25,
city: 'San Francisco'
};
fetch('http://127.0.0.1:5000/api/data', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
}
</script>
</body>
</html>
二、使用Django框架实现前后端交互
Django是一个功能强大的Web框架,适合大型应用开发。它自带了许多实用工具,可以方便地进行前后端交互。
1、安装Django
首先,确保你已经安装了Django。你可以使用pip来安装:
pip install Django
2、创建一个Django项目和应用
创建一个新的Django项目:
django-admin startproject myproject
cd myproject
创建一个新的Django应用:
python manage.py startapp myapp
3、配置Django项目
在myproject/settings.py
中,添加你的应用到INSTALLED_APPS
:
INSTALLED_APPS = [
...
'myapp',
]
4、创建一个简单的Django视图
在myapp/views.py
中,创建两个视图,一个用于GET请求,另一个用于POST请求:
from django.http import JsonResponse
from django.views.decorators.csrf import csrf_exempt
import json
def get_data(request):
sample_data = {
"name": "John",
"age": 30,
"city": "New York"
}
return JsonResponse(sample_data)
@csrf_exempt
def post_data(request):
if request.method == 'POST':
data = json.loads(request.body)
response = {
"status": "success",
"data_received": data
}
return JsonResponse(response)
else:
return JsonResponse({"status": "error"}, status=400)
5、配置Django URLs
在myapp/urls.py
中,配置URL路由:
from django.urls import path
from .views import get_data, post_data
urlpatterns = [
path('api/data', get_data, name='get_data'),
path('api/data', post_data, name='post_data'),
]
在myproject/urls.py
中,包含应用的URL:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('myapp.urls')),
]
6、前端调用Django API
前端调用Django API的方式与调用Flask API类似,可以使用纯JavaScript或其他前端框架。以下是一个使用纯JavaScript的示例(与上面的Flask示例相同):
<!DOCTYPE html>
<html>
<head>
<title>Django API Example</title>
</head>
<body>
<h1>Django API Example</h1>
<button onclick="fetchData()">Get Data</button>
<button onclick="sendData()">Send Data</button>
<script>
function fetchData() {
fetch('http://127.0.0.1:8000/api/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
}
function sendData() {
const data = {
name: 'Jane',
age: 25,
city: 'San Francisco'
};
fetch('http://127.0.0.1:8000/api/data', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
}
</script>
</body>
</html>
三、通过RESTful API实现前后端交互
RESTful API是一种常见的Web服务架构,适合用于前后端分离的项目。它使用HTTP方法(如GET、POST、PUT、DELETE)进行资源的操作。
1、定义RESTful API
首先,定义你的RESTful API端点和资源。例如,假设你有一个用户资源,你可以定义以下端点:
- GET /users – 获取所有用户
- GET /users/{id} – 获取特定用户
- POST /users – 创建一个新用户
- PUT /users/{id} – 更新特定用户
- DELETE /users/{id} – 删除特定用户
2、创建API端点
你可以使用Flask或Django来创建这些API端点。以下是一个使用Flask的示例:
from flask import Flask, request, jsonify
app = Flask(__name__)
users = [
{"id": 1, "name": "John", "age": 30},
{"id": 2, "name": "Jane", "age": 25}
]
@app.route('/users', methods=['GET'])
def get_users():
return jsonify(users)
@app.route('/users/<int:user_id>', methods=['GET'])
def get_user(user_id):
user = next((user for user in users if user["id"] == user_id), None)
if user:
return jsonify(user)
else:
return jsonify({"message": "User not found"}), 404
@app.route('/users', methods=['POST'])
def create_user():
new_user = request.json
new_user["id"] = len(users) + 1
users.append(new_user)
return jsonify(new_user), 201
@app.route('/users/<int:user_id>', methods=['PUT'])
def update_user(user_id):
user = next((user for user in users if user["id"] == user_id), None)
if user:
updated_data = request.json
user.update(updated_data)
return jsonify(user)
else:
return jsonify({"message": "User not found"}), 404
@app.route('/users/<int:user_id>', methods=['DELETE'])
def delete_user(user_id):
global users
users = [user for user in users if user["id"] != user_id]
return jsonify({"message": "User deleted"})
if __name__ == '__main__':
app.run(debug=True)
3、前端调用RESTful API
前端可以使用类似前面的方式调用这些API端点。以下是使用纯JavaScript的示例:
<!DOCTYPE html>
<html>
<head>
<title>RESTful API Example</title>
</head>
<body>
<h1>RESTful API Example</h1>
<button onclick="fetchUsers()">Get Users</button>
<button onclick="createUser()">Create User</button>
<script>
function fetchUsers() {
fetch('http://127.0.0.1:5000/users')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
}
function createUser() {
const data = {
name: 'Alice',
age: 28
};
fetch('http://127.0.0.1:5000/users', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
}
</script>
</body>
</html>
四、使用WebSocket实现前后端交互
WebSocket是一种在单个TCP连接上进行全双工通信的协议,适用于需要实时数据更新的应用,如聊天应用、在线游戏等。
1、安装WebSocket库
你可以使用websockets
库来实现WebSocket服务器。使用pip安装:
pip install websockets
2、创建WebSocket服务器
下面是一个简单的WebSocket服务器示例:
import asyncio
import websockets
async def echo(websocket, path):
async for message in websocket:
await websocket.send(f"Echo: {message}")
start_server = websockets.serve(echo, "localhost", 8765)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
3、前端连接WebSocket服务器
前端可以使用JavaScript的WebSocket API来连接和通信。以下是一个简单的示例:
<!DOCTYPE html>
<html>
<head>
<title>WebSocket Example</title>
</head>
<body>
<h1>WebSocket Example</h1>
<button onclick="connectWebSocket()">Connect WebSocket</button>
<button onclick="sendMessage()">Send Message</button>
<script>
let socket;
function connectWebSocket() {
socket = new WebSocket('ws://localhost:8765');
socket.onopen = function(event) {
console.log('WebSocket is open now.');
};
socket.onmessage = function(event) {
console.log('Received: ' + event.data);
};
socket.onclose = function(event) {
console.log('WebSocket is closed now.');
};
socket.onerror = function(error) {
console.log('WebSocket Error: ' + error);
};
}
function sendMessage() {
if (socket && socket.readyState === WebSocket.OPEN) {
socket.send('Hello, WebSocket!');
} else {
console.log('WebSocket is not open.');
}
}
</script>
</body>
</html>
五、结合前端框架如React或Vue进行完整开发
在现代Web开发中,前后端分离的做法越来越普遍。你可以使用前端框架如React或Vue来创建用户界面,并通过API与后端进行交互。
1、使用React框架
首先,确保你已经安装了Node.js和npm。然后,使用Create React App创建一个新的React项目:
npx create-react-app my-react-app
cd my-react-app
2、创建React组件
在你的React项目中,创建一个新的组件来调用API:
import React, { useState, useEffect } from 'react';
function App() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('http://127.0.0.1:5000/api/data')
.then(response => response.json())
.then(data => setData(data))
.catch(error => console.error('Error:', error));
}, []);
const sendData = () => {
const dataToSend = {
name: 'Alice',
age: 28,
city: 'San Francisco'
};
fetch('http://127.0.0.1:5000/api/data', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(dataToSend)
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
};
return (
<div>
<h1>React API Example</h1>
<button onClick={sendData}>Send Data</button>
{data && (
<div>
<h2>Data from API:</h2>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
)}
</div>
);
}
export default App;
3、运行React应用
在项目目录中,运行以下命令启动React开发服务器:
npm start
六、使用Vue框架
Vue是另一个流行的前端框架,适合用于创建动态和响应式的用户界面。
1、创建Vue项目
首先,确保你已经安装了Vue CLI。然后,使用Vue CLI创建一个新的Vue项目:
npm install -g @vue/cli
vue create my-vue-app
cd my-vue-app
2、创建Vue组件
在你的Vue项目中,创建一个新的组件来调用API。编辑src/components/HelloWorld.vue
:
<template>
<div>
<h1>Vue API Example</h1>
<button @click="sendData">Send Data</button>
<div v-if="data">
<h2>Data from API:</h2>
<pre>{{ data }}</pre>
</div>
</div>
</template>
<script>
export default {
data() {
return {
data: null,
};
},
mounted() {
fetch('http://127.0.0.1:5000/api/data')
.then(response => response.json())
.then(data => {
this.data = data;
})
.catch(error => console.error('Error:', error));
},
methods: {
sendData() {
const dataToSend = {
name: 'Alice',
age: 28,
city: 'San Francisco',
};
fetch('http://127.0.0.1:5000/api/data', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(dataToSend),
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
},
},
};
</script>
3、运行Vue应用
在项目目录中,运行以下命令启动Vue开发服务器:
npm run serve
通过以上步骤,你可以使用Python和现代前端框架(如React或Vue)创建一个完整的前后端交互应用。无论选择哪种方式,关键在于理解和掌握API的创建和调用,以及前端与后端如何通过API进行数据交换。这些技巧和方法将极大地提升你的Web开发能力。
相关问答FAQs:
如何使用Python实现与前端的有效数据交互?
在Python中,前后端交互通常通过API实现。可以使用Flask或Django等框架搭建RESTful API,前端通过HTTP请求(如GET、POST)与后端进行数据交换。使用JSON格式传输数据是最常见的方式,确保前端能够轻松解析和使用这些数据。
在Python中,如何处理前端发送的请求数据?
当前端发送请求到后端时,后端可以通过Flask的request
对象或Django的request
模块获取数据。对于POST请求,常用request.form
或request.json
来提取表单数据或JSON数据。同时,确保在后端进行数据验证和安全性检查,防止恶意输入。
如何在Python项目中实现WebSocket以实现实时交互?
使用WebSocket可以实现前后端的实时双向通信,适合需要即时更新的应用场景。Python的channels
(Django)或Flask-SocketIO
库可以帮助你轻松实现WebSocket功能。通过设置相应的路由和事件处理程序,前端可以在不刷新页面的情况下接收来自后端的实时数据更新。
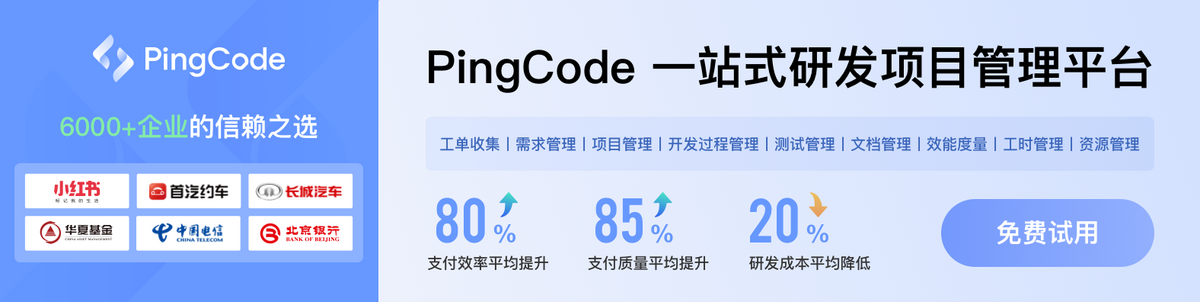