使用Python代码发送邮件,可以通过以下步骤:导入smtplib库、设置邮件服务器、编写邮件内容、登录邮箱并发送邮件。其中,编写邮件内容是一个重要的环节。为了确保邮件能够正确发送并被接收方识别,编写邮件内容时需要设置邮件的主题、发件人、收件人和邮件正文。下面将详细介绍如何编写邮件内容,并演示一个完整的Python代码发送邮件的例子。
一、导入smtplib库
Python内置了一个smtplib库,用于发送邮件。首先需要导入这个库:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.header import Header
二、设置邮件服务器
要发送邮件,首先需要连接到邮件服务器。以Gmail为例:
smtp_server = 'smtp.gmail.com'
smtp_port = 587
username = 'your_email@gmail.com'
password = 'your_password'
三、编写邮件内容
编写邮件内容包括设置邮件的主题、发件人、收件人和邮件正文。可以使用MIMEText和MIMEMultipart来构建邮件内容。
1、设置邮件主题
邮件的主题可以通过Header来设置:
subject = 'Python Email Test'
msg = MIMEMultipart()
msg['From'] = Header(username, 'utf-8')
msg['To'] = Header('recipient@example.com', 'utf-8')
msg['Subject'] = Header(subject, 'utf-8')
2、设置邮件正文
邮件正文可以是纯文本,也可以是HTML格式:
body = 'This is a test email sent from Python.'
msg.attach(MIMEText(body, 'plain', 'utf-8'))
如果需要发送HTML格式的邮件正文,可以这样做:
html = '<html><body><h1>Hello</h1><p>This is a test email sent from Python.</p></body></html>'
msg.attach(MIMEText(html, 'html', 'utf-8'))
四、登录邮箱并发送邮件
使用smtplib登录邮箱并发送邮件:
try:
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.ehlo()
server.starttls()
server.login(username, password)
server.sendmail(username, ['recipient@example.com'], msg.as_string())
print('Email sent successfully!')
except Exception as e:
print(f'Error: {e}')
五、完整代码示例
下面是一个完整的代码示例,展示了如何使用Python发送邮件:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.header import Header
设置邮件服务器
smtp_server = 'smtp.gmail.com'
smtp_port = 587
username = 'your_email@gmail.com'
password = 'your_password'
设置邮件内容
subject = 'Python Email Test'
msg = MIMEMultipart()
msg['From'] = Header(username, 'utf-8')
msg['To'] = Header('recipient@example.com', 'utf-8')
msg['Subject'] = Header(subject, 'utf-8')
设置邮件正文
body = 'This is a test email sent from Python.'
msg.attach(MIMEText(body, 'plain', 'utf-8'))
如果需要发送HTML格式的邮件正文,可以这样做:
html = '<html><body><h1>Hello</h1><p>This is a test email sent from Python.</p></body></html>'
msg.attach(MIMEText(html, 'html', 'utf-8'))
登录邮箱并发送邮件
try:
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.ehlo()
server.starttls()
server.login(username, password)
server.sendmail(username, ['recipient@example.com'], msg.as_string())
print('Email sent successfully!')
except Exception as e:
print(f'Error: {e}')
六、附加功能
1、添加附件
可以通过MIMEBase来添加附件:
from email.mime.base import MIMEBase
from email import encoders
filename = 'example.txt'
attachment = open(filename, 'rb')
part = MIMEBase('application', 'octet-stream')
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', f'attachment; filename= {filename}')
msg.attach(part)
2、发送多封邮件
可以通过循环来发送多封邮件:
recipients = ['recipient1@example.com', 'recipient2@example.com']
for recipient in recipients:
msg['To'] = Header(recipient, 'utf-8')
try:
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.ehlo()
server.starttls()
server.login(username, password)
server.sendmail(username, [recipient], msg.as_string())
print(f'Email sent successfully to {recipient}!')
except Exception as e:
print(f'Error sending email to {recipient}: {e}')
七、使用其他邮件服务器
除了Gmail,还可以使用其他邮件服务器,例如QQ邮箱、Outlook等。只需更改邮件服务器地址和端口号:
1、QQ邮箱
smtp_server = 'smtp.qq.com'
smtp_port = 465
username = 'your_email@qq.com'
password = 'your_password'
2、Outlook
smtp_server = 'smtp-mail.outlook.com'
smtp_port = 587
username = 'your_email@outlook.com'
password = 'your_password'
八、总结
使用Python发送邮件是一个常见的需求,smtplib库提供了便捷的接口来实现这一功能。通过导入smtplib库、设置邮件服务器、编写邮件内容、登录邮箱并发送邮件,可以轻松地发送邮件。此外,还可以通过MIMEBase添加附件,通过循环发送多封邮件,以及使用其他邮件服务器。希望这篇文章能够帮助你更好地理解和使用Python发送邮件。
相关问答FAQs:
如何使用Python发送邮件的基本步骤是什么?
要使用Python发送邮件,首先需要安装smtplib
库,这是Python自带的库。接下来,您需要设置SMTP服务器的信息,包括服务器地址、端口号和您的邮箱账户凭据。创建邮件内容后,使用smtplib
连接到服务器并发送邮件。最后,确保安全性,使用SSL加密连接。
可以使用哪些Python库来发送邮件?
除了smtplib
,您还可以使用其他库,例如email
库来构建邮件内容,支持文本和HTML格式。yagmail
是一个更简单的库,封装了smtplib
,提供了更直观的接口。此外,sendgrid
和mailgun
等第三方服务也提供Python SDK,适合需要发送大量邮件的场景。
发送邮件时需要注意哪些安全问题?
在发送邮件时,确保使用SSL或TLS加密连接,以保护您的账户凭据和邮件内容。此外,避免在代码中硬编码敏感信息,建议使用环境变量存储账户信息。定期更改密码,并确保您的邮箱开启两步验证,以增加安全性。
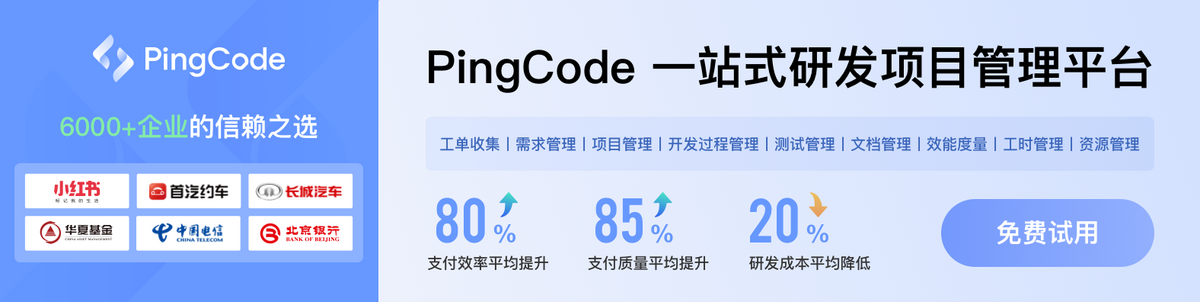