要在Python中设置自动连接WiFi,可以使用一些专门的库和工具。例如,Windows平台可以使用pywifi
库,Linux平台可以使用nmcli
命令行工具。通过使用pywifi库、通过使用nmcli命令、通过编写脚本实现自动连接WiFi。下面我们将详细介绍如何在Windows和Linux系统上使用这些方法来实现自动连接WiFi。
一、在Windows上使用pywifi库
安装pywifi库
首先,需要安装pywifi
库,可以使用pip进行安装:
pip install pywifi
使用pywifi连接WiFi
以下是一个示例代码,展示如何使用pywifi库来连接WiFi:
import time
from pywifi import PyWiFi, const, Profile
def connect_to_wifi(ssid, password):
wifi = PyWiFi()
iface = wifi.interfaces()[0]
iface.disconnect()
time.sleep(1)
while iface.status() == const.IFACE_DISCONNECTED:
pass
profile = Profile()
profile.ssid = ssid
profile.auth = const.AUTH_ALG_OPEN
profile.akm.append(const.AKM_TYPE_WPA2PSK)
profile.cipher = const.CIPHER_TYPE_CCMP
profile.key = password
iface.remove_all_network_profiles()
tmp_profile = iface.add_network_profile(profile)
iface.connect(tmp_profile)
time.sleep(10)
if iface.status() == const.IFACE_CONNECTED:
print("Connected to ", ssid)
else:
print("Failed to connect to ", ssid)
Replace 'your_ssid' and 'your_password' with your WiFi SSID and password
connect_to_wifi('your_ssid', 'your_password')
这段代码首先断开当前的WiFi连接,然后创建一个新的WiFi配置文件,设置SSID和密码,并尝试连接到指定的WiFi网络。如果连接成功,会打印"Connected to "消息;否则,会打印"Failed to connect to "消息。
二、在Linux上使用nmcli命令
使用nmcli命令连接WiFi
在Linux系统上,可以使用nmcli
命令行工具来管理网络连接。以下是一个示例代码,展示如何在Python中使用nmcli
命令来连接WiFi:
import os
def connect_to_wifi(ssid, password):
# Create a new connection
os.system(f"nmcli dev wifi connect '{ssid}' password '{password}'")
# Check the connection status
status = os.popen("nmcli -t -f ACTIVE,SSID dev wifi").read().strip()
if f"yes:{ssid}" in status:
print(f"Connected to {ssid}")
else:
print(f"Failed to connect to {ssid}")
Replace 'your_ssid' and 'your_password' with your WiFi SSID and password
connect_to_wifi('your_ssid', 'your_password')
这段代码使用os.system
来执行nmcli
命令连接WiFi,并使用os.popen
来检查连接状态。如果连接成功,会打印"Connected to "消息;否则,会打印"Failed to connect to "消息。
三、编写脚本实现自动连接WiFi
在实际应用中,我们可能需要编写一个更复杂的脚本来实现自动连接WiFi。例如,可以在启动时自动连接到已知的WiFi网络,并在连接失败时重试。
示例脚本
以下是一个示例脚本,展示如何实现上述功能:
import os
import time
from pywifi import PyWiFi, const, Profile
def connect_to_wifi_windows(ssid, password):
wifi = PyWiFi()
iface = wifi.interfaces()[0]
iface.disconnect()
time.sleep(1)
while iface.status() == const.IFACE_DISCONNECTED:
pass
profile = Profile()
profile.ssid = ssid
profile.auth = const.AUTH_ALG_OPEN
profile.akm.append(const.AKM_TYPE_WPA2PSK)
profile.cipher = const.CIPHER_TYPE_CCMP
profile.key = password
iface.remove_all_network_profiles()
tmp_profile = iface.add_network_profile(profile)
iface.connect(tmp_profile)
time.sleep(10)
if iface.status() == const.IFACE_CONNECTED:
print("Connected to ", ssid)
else:
print("Failed to connect to ", ssid)
def connect_to_wifi_linux(ssid, password):
os.system(f"nmcli dev wifi connect '{ssid}' password '{password}'")
status = os.popen("nmcli -t -f ACTIVE,SSID dev wifi").read().strip()
if f"yes:{ssid}" in status:
print(f"Connected to {ssid}")
else:
print(f"Failed to connect to {ssid}")
def main():
ssid = 'your_ssid'
password = 'your_password'
if os.name == 'nt':
connect_to_wifi_windows(ssid, password)
else:
connect_to_wifi_linux(ssid, password)
if __name__ == "__main__":
main()
这个脚本首先判断操作系统类型,然后选择适当的方法来连接WiFi。在Windows系统上使用pywifi
库,在Linux系统上使用nmcli
命令。
四、错误处理和重试机制
在实际应用中,WiFi连接可能会失败,因此需要添加错误处理和重试机制。
添加重试机制
以下是一个添加重试机制的示例脚本:
import os
import time
from pywifi import PyWiFi, const, Profile
def connect_to_wifi_windows(ssid, password, retries=3):
wifi = PyWiFi()
iface = wifi.interfaces()[0]
for _ in range(retries):
iface.disconnect()
time.sleep(1)
while iface.status() == const.IFACE_DISCONNECTED:
pass
profile = Profile()
profile.ssid = ssid
profile.auth = const.AUTH_ALG_OPEN
profile.akm.append(const.AKM_TYPE_WPA2PSK)
profile.cipher = const.CIPHER_TYPE_CCMP
profile.key = password
iface.remove_all_network_profiles()
tmp_profile = iface.add_network_profile(profile)
iface.connect(tmp_profile)
time.sleep(10)
if iface.status() == const.IFACE_CONNECTED:
print("Connected to ", ssid)
return True
else:
print("Failed to connect to ", ssid, "Retrying...")
return False
def connect_to_wifi_linux(ssid, password, retries=3):
for _ in range(retries):
os.system(f"nmcli dev wifi connect '{ssid}' password '{password}'")
status = os.popen("nmcli -t -f ACTIVE,SSID dev wifi").read().strip()
if f"yes:{ssid}" in status:
print(f"Connected to {ssid}")
return True
else:
print(f"Failed to connect to {ssid}, Retrying...")
return False
def main():
ssid = 'your_ssid'
password = 'your_password'
retries = 3
if os.name == 'nt':
if connect_to_wifi_windows(ssid, password, retries):
print("Successfully connected to WiFi")
else:
print("Failed to connect to WiFi after multiple attempts")
else:
if connect_to_wifi_linux(ssid, password, retries):
print("Successfully connected to WiFi")
else:
print("Failed to connect to WiFi after multiple attempts")
if __name__ == "__main__":
main()
这个脚本在连接失败时会尝试重新连接最多三次。如果连接成功,会打印"Successfully connected to WiFi"消息;如果多次尝试后仍然失败,会打印"Failed to connect to WiFi after multiple attempts"消息。
五、保存和读取WiFi配置
在实际应用中,我们可能需要保存WiFi配置到文件,并在下次启动时读取配置。
保存WiFi配置到文件
以下是一个示例代码,展示如何将WiFi配置保存到文件:
import json
def save_wifi_config(ssid, password, filename='wifi_config.json'):
config = {
'ssid': ssid,
'password': password
}
with open(filename, 'w') as f:
json.dump(config, f)
def load_wifi_config(filename='wifi_config.json'):
with open(filename, 'r') as f:
config = json.load(f)
return config['ssid'], config['password']
Replace 'your_ssid' and 'your_password' with your WiFi SSID and password
save_wifi_config('your_ssid', 'your_password')
ssid, password = load_wifi_config()
print(f"SSID: {ssid}, Password: {password}")
这个代码展示了如何将WiFi配置保存到JSON文件,并在需要时读取配置。
使用保存的WiFi配置
可以将读取的配置应用到前面的连接脚本中:
import os
import time
import json
from pywifi import PyWiFi, const, Profile
def save_wifi_config(ssid, password, filename='wifi_config.json'):
config = {
'ssid': ssid,
'password': password
}
with open(filename, 'w') as f:
json.dump(config, f)
def load_wifi_config(filename='wifi_config.json'):
with open(filename, 'r') as f:
config = json.load(f)
return config['ssid'], config['password']
def connect_to_wifi_windows(ssid, password, retries=3):
wifi = PyWiFi()
iface = wifi.interfaces()[0]
for _ in range(retries):
iface.disconnect()
time.sleep(1)
while iface.status() == const.IFACE_DISCONNECTED:
pass
profile = Profile()
profile.ssid = ssid
profile.auth = const.AUTH_ALG_OPEN
profile.akm.append(const.AKM_TYPE_WPA2PSK)
profile.cipher = const.CIPHER_TYPE_CCMP
profile.key = password
iface.remove_all_network_profiles()
tmp_profile = iface.add_network_profile(profile)
iface.connect(tmp_profile)
time.sleep(10)
if iface.status() == const.IFACE_CONNECTED:
print("Connected to ", ssid)
return True
else:
print("Failed to connect to ", ssid, "Retrying...")
return False
def connect_to_wifi_linux(ssid, password, retries=3):
for _ in range(retries):
os.system(f"nmcli dev wifi connect '{ssid}' password '{password}'")
status = os.popen("nmcli -t -f ACTIVE,SSID dev wifi").read().strip()
if f"yes:{ssid}" in status:
print(f"Connected to {ssid}")
return True
else:
print(f"Failed to connect to {ssid}, Retrying...")
return False
def main():
ssid, password = load_wifi_config()
retries = 3
if os.name == 'nt':
if connect_to_wifi_windows(ssid, password, retries):
print("Successfully connected to WiFi")
else:
print("Failed to connect to WiFi after multiple attempts")
else:
if connect_to_wifi_linux(ssid, password, retries):
print("Successfully connected to WiFi")
else:
print("Failed to connect to WiFi after multiple attempts")
if __name__ == "__main__":
main()
这个脚本首先从文件中读取WiFi配置,然后使用读取的配置尝试连接WiFi。
六、总结
通过本文的介绍,我们了解了如何在Python中设置自动连接WiFi的方法,包括通过使用pywifi库、通过使用nmcli命令、通过编写脚本实现自动连接WiFi。此外,我们还探讨了如何处理连接错误、添加重试机制,以及如何保存和读取WiFi配置。希望这些内容对您有所帮助。如果您有任何疑问或需要进一步的帮助,请随时联系我。
相关问答FAQs:
如何使用Python自动连接WiFi?
要使用Python自动连接WiFi,您可以利用系统命令和一些库。比如在Windows上,可以使用subprocess
库来调用netsh
命令,或者在Linux上利用nmcli
命令。确保您已经安装了必要的库,并获得适当的管理员权限。
我需要哪些Python库来实现WiFi自动连接?
一般来说,您可能需要安装subprocess
(Python自带),以及os
库来执行系统命令。对于更复杂的操作,您可以考虑使用pywifi
库,它可以帮助您更方便地管理无线网络连接。
Python连接WiFi时,如何处理连接失败的情况?
在处理WiFi连接时,您可以通过捕获异常来应对连接失败的情况。可以设置重试机制,尝试多次连接,或者在连接失败后记录错误信息,便于后续排查。务必确保您的网络凭证正确无误,以减少连接问题的发生。
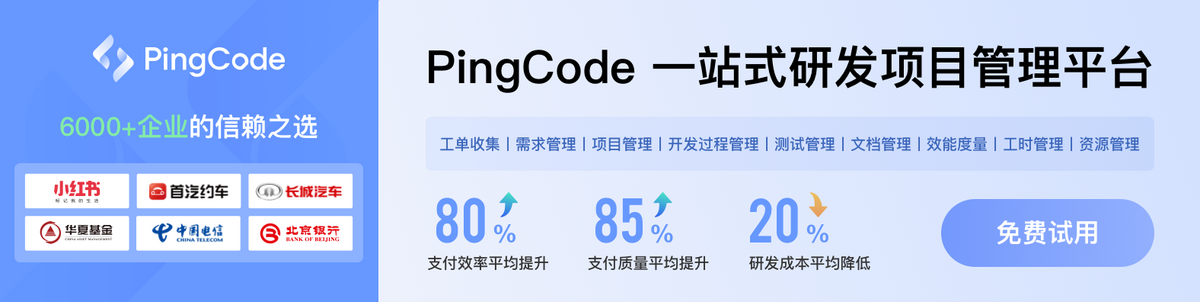