在Python中,mode函数通常是指用于计算一组数据中出现频率最高的值。Python标准库中没有直接提供mode函数,但可以通过statistics
模块中的mode
函数来实现这一功能,此外也可以使用collections
模块中的Counter
类来实现。statistics.mode
函数简单易用、Counter
类功能强大,下面将详细介绍这两种方法。
statistics.mode
的详细介绍:
statistics
模块是Python内置的统计模块,提供了许多统计相关的函数,包括mean
、median
和mode
等。mode
函数用于计算众数(即数据集中出现次数最多的值),其语法为:
import statistics
mode_value = statistics.mode(data)
其中,data
是一个包含数字或字符串的可迭代对象。
示例代码:
import statistics
data = [1, 2, 2, 3, 3, 3, 4]
mode_value = statistics.mode(data)
print(f"The mode of the data set is: {mode_value}")
collections.Counter
的使用:
Counter
是collections
模块中的一个类,用于计数可哈希对象。它的most_common
方法可以用来找到出现频率最高的值。其语法为:
from collections import Counter
counter = Counter(data)
mode_value = counter.most_common(1)[0][0]
示例代码:
from collections import Counter
data = [1, 2, 2, 3, 3, 3, 4]
counter = Counter(data)
mode_value = counter.most_common(1)[0][0]
print(f"The mode of the data set is: {mode_value}")
接下来,我们将深入探讨这两种方法的具体使用和其他相关内容。
一、STATISTICS模块的使用
1、安装和导入
首先,确保你的Python环境中已经安装了statistics
模块。statistics
是Python标准库的一部分,通常不需要额外安装。导入statistics
模块的方法如下:
import statistics
2、基本用法
mode
函数用于计算众数,适用于数字和字符串数据。以下是一个简单的示例:
import statistics
data = [1, 1, 2, 2, 3, 3, 3, 4]
mode_value = statistics.mode(data)
print(f"The mode of the data set is: {mode_value}")
该代码会输出:
The mode of the data set is: 3
3、处理多个众数
statistics.mode
函数在处理有多个众数的数据时会抛出StatisticsError
。例如:
import statistics
data = [1, 1, 2, 2, 3, 3]
try:
mode_value = statistics.mode(data)
except statistics.StatisticsError as e:
print(f"StatisticsError: {e}")
这段代码会输出:
StatisticsError: no unique mode; found 3 equally common values
4、使用multimode
函数
为了处理有多个众数的情况,statistics
模块提供了multimode
函数。它返回所有众数的列表:
import statistics
data = [1, 1, 2, 2, 3, 3]
modes = statistics.multimode(data)
print(f"The modes of the data set are: {modes}")
该代码会输出:
The modes of the data set are: [1, 2, 3]
二、COLLECTIONS模块的使用
1、安装和导入
collections
模块是Python标准库的一部分,因此通常不需要额外安装。导入Counter
类的方法如下:
from collections import Counter
2、基本用法
Counter
类可以用于计数可哈希对象,以下是一个简单的示例:
from collections import Counter
data = [1, 1, 2, 2, 3, 3, 3, 4]
counter = Counter(data)
mode_value = counter.most_common(1)[0][0]
print(f"The mode of the data set is: {mode_value}")
该代码会输出:
The mode of the data set is: 3
3、处理多个众数
Counter
类的most_common
方法返回一个列表,其中包含所有元素及其出现的次数,按出现频率从高到低排序。你可以根据需要提取多个众数:
from collections import Counter
data = [1, 1, 2, 2, 3, 3]
counter = Counter(data)
most_common_elements = counter.most_common()
max_count = most_common_elements[0][1]
modes = [item for item, count in most_common_elements if count == max_count]
print(f"The modes of the data set are: {modes}")
该代码会输出:
The modes of the data set are: [1, 2, 3]
三、其他相关内容
1、处理数据中的异常值
在计算众数之前,可能需要处理数据中的异常值。可以使用filter
函数或列表推导式来过滤掉异常值。例如:
data = [1, 1, 2, 2, 3, 3, 3, 100]
Filter out outliers
filtered_data = [x for x in data if x < 50]
import statistics
mode_value = statistics.mode(filtered_data)
print(f"The mode of the filtered data set is: {mode_value}")
该代码会输出:
The mode of the filtered data set is: 3
2、处理缺失值
在实际数据处理中,可能会遇到缺失值。可以使用filter
函数或列表推导式来移除缺失值。例如:
data = [1, 1, 2, 2, None, 3, 3, 3, None]
Remove None values
filtered_data = [x for x in data if x is not None]
import statistics
mode_value = statistics.mode(filtered_data)
print(f"The mode of the filtered data set is: {mode_value}")
该代码会输出:
The mode of the filtered data set is: 3
3、应用场景
众数计算在许多领域都有应用,包括统计学、数据分析和机器学习。例如,在市场调研中,众数可以用于确定最受欢迎的产品或服务;在数据分析中,众数可以用于识别数据集中的常见模式。
4、总结
statistics
模块和collections
模块都提供了方便的工具来计算众数。statistics.mode
函数简单易用,适用于基本的众数计算;collections.Counter
类功能强大,适用于更复杂的众数计算。在实际应用中,可以根据具体需求选择合适的方法。
无论使用哪种方法,都需要注意数据中的异常值和缺失值,并在计算众数之前进行适当的预处理。通过合理使用这些工具,可以轻松计算数据集中的众数,并从中提取有价值的信息。
相关问答FAQs:
在Python中,mode函数的主要用途是什么?
mode函数用于找出一组数据中出现频率最高的数值,也被称为众数。在数据分析和统计学中,众数是一个非常重要的指标,可以帮助我们理解数据的分布和特征。尤其是在处理分类数据时,使用mode函数能够快速识别出最常见的类别。
如何在Python中使用mode函数来处理数据?
在Python中,可以使用statistics
模块中的mode
函数,或者使用pandas
库中的mode
方法。使用statistics.mode()
时,需要传入一个可迭代对象(如列表),而pandas.DataFrame.mode()
则适用于DataFrame或Series,能够返回一个包含众数的对象。具体用法可以参考相关文档或示例代码,以便更好地理解其应用。
mode函数在处理多众数时会有什么表现?
如果数据集中存在多个众数,Python中的statistics.mode()
会引发StatisticsError
,因为它只能返回一个众数。而在pandas
中,mode
方法会返回一个包含所有众数的Series。这种差异使得在选择使用哪个库时,需要根据具体需求来决定,以确保能够正确处理多众数的情况。
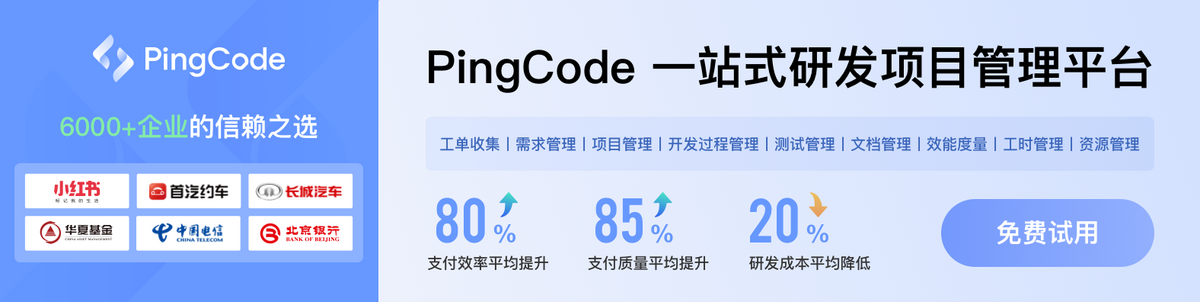