Python找出高频词的步骤包括:数据预处理、分词、词频统计、排序。其中,分词是一个非常重要的步骤,因为它直接影响到后续的词频统计结果。本文将详细介绍如何使用Python找出文本中的高频词。
一、数据预处理
数据预处理是文本分析的第一步,主要包括去除标点符号、转换为小写、去除停用词等。以下是一个简单的数据预处理示例:
import re
import string
def preprocess_text(text):
# 去除标点符号
text = re.sub(f'[{re.escape(string.punctuation)}]', '', text)
# 转换为小写
text = text.lower()
return text
二、分词
分词是将文本切分成一个个单词的过程。Python有很多分词工具,如NLTK、spaCy等。以下是使用NLTK进行分词的示例:
import nltk
nltk.download('punkt')
def tokenize_text(text):
return nltk.word_tokenize(text)
三、词频统计
词频统计是找出高频词的关键步骤。可以使用Python的collections.Counter
类来方便地统计词频。以下是一个示例:
from collections import Counter
def count_word_frequency(tokens):
return Counter(tokens)
四、排序
为了找出高频词,需要对词频统计结果进行排序。可以使用Counter
类的most_common
方法来实现:
def get_most_common_words(word_freq, n=10):
return word_freq.most_common(n)
五、完整示例
以下是一个完整的示例,展示了如何使用上述步骤找出高频词:
import re
import string
import nltk
from collections import Counter
nltk.download('punkt')
def preprocess_text(text):
text = re.sub(f'[{re.escape(string.punctuation)}]', '', text)
text = text.lower()
return text
def tokenize_text(text):
return nltk.word_tokenize(text)
def count_word_frequency(tokens):
return Counter(tokens)
def get_most_common_words(word_freq, n=10):
return word_freq.most_common(n)
if __name__ == "__main__":
text = "Python is an interpreted, high-level and general-purpose programming language. Python's design philosophy emphasizes code readability with its notable use of significant whitespace."
preprocessed_text = preprocess_text(text)
tokens = tokenize_text(preprocessed_text)
word_freq = count_word_frequency(tokens)
most_common_words = get_most_common_words(word_freq)
print(most_common_words)
六、去除停用词
为了提高高频词的准确性,通常需要去除停用词。停用词是指在文本中频繁出现但对文本分析意义不大的词,如“the”、“is”等。可以使用NLTK的停用词列表来去除停用词:
from nltk.corpus import stopwords
nltk.download('stopwords')
def remove_stopwords(tokens):
stop_words = set(stopwords.words('english'))
return [word for word in tokens if word not in stop_words]
将去除停用词的步骤添加到完整示例中:
import re
import string
import nltk
from collections import Counter
nltk.download('punkt')
nltk.download('stopwords')
def preprocess_text(text):
text = re.sub(f'[{re.escape(string.punctuation)}]', '', text)
text = text.lower()
return text
def tokenize_text(text):
return nltk.word_tokenize(text)
def remove_stopwords(tokens):
stop_words = set(stopwords.words('english'))
return [word for word in tokens if word not in stop_words]
def count_word_frequency(tokens):
return Counter(tokens)
def get_most_common_words(word_freq, n=10):
return word_freq.most_common(n)
if __name__ == "__main__":
text = "Python is an interpreted, high-level and general-purpose programming language. Python's design philosophy emphasizes code readability with its notable use of significant whitespace."
preprocessed_text = preprocess_text(text)
tokens = tokenize_text(preprocessed_text)
tokens = remove_stopwords(tokens)
word_freq = count_word_frequency(tokens)
most_common_words = get_most_common_words(word_freq)
print(most_common_words)
七、使用spaCy进行分词和停用词去除
除了NLTK,spaCy也是一个非常强大的自然语言处理库。以下是使用spaCy进行分词和停用词去除的示例:
import spacy
nlp = spacy.load('en_core_web_sm')
def spacy_tokenize_and_remove_stopwords(text):
doc = nlp(text)
tokens = [token.text for token in doc if not token.is_stop and not token.is_punct]
return tokens
if __name__ == "__main__":
text = "Python is an interpreted, high-level and general-purpose programming language. Python's design philosophy emphasizes code readability with its notable use of significant whitespace."
tokens = spacy_tokenize_and_remove_stopwords(text)
word_freq = count_word_frequency(tokens)
most_common_words = get_most_common_words(word_freq)
print(most_common_words)
八、处理大规模文本数据
当处理大规模文本数据时,内存可能成为一个瓶颈。此时,可以使用生成器或分批次处理数据来减小内存占用。以下是一个示例,展示了如何分批次处理大规模文本数据:
def process_large_text_file(file_path, batch_size=1000):
with open(file_path, 'r', encoding='utf-8') as file:
text_batch = []
for line in file:
text_batch.append(line.strip())
if len(text_batch) >= batch_size:
yield ' '.join(text_batch)
text_batch = []
if text_batch:
yield ' '.join(text_batch)
if __name__ == "__main__":
file_path = 'large_text_file.txt'
word_freq = Counter()
for text_batch in process_large_text_file(file_path):
preprocessed_text = preprocess_text(text_batch)
tokens = tokenize_text(preprocessed_text)
tokens = remove_stopwords(tokens)
word_freq.update(tokens)
most_common_words = get_most_common_words(word_freq)
print(most_common_words)
九、可视化高频词
为了更直观地展示高频词,可以使用词云图。以下是使用wordcloud
库生成词云图的示例:
from wordcloud import WordCloud
import matplotlib.pyplot as plt
def generate_word_cloud(word_freq):
wordcloud = WordCloud(width=800, height=400, background_color='white').generate_from_frequencies(word_freq)
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
if __name__ == "__main__":
text = "Python is an interpreted, high-level and general-purpose programming language. Python's design philosophy emphasizes code readability with its notable use of significant whitespace."
preprocessed_text = preprocess_text(text)
tokens = tokenize_text(preprocessed_text)
tokens = remove_stopwords(tokens)
word_freq = count_word_frequency(tokens)
generate_word_cloud(word_freq)
十、总结
通过以上步骤,我们可以使用Python高效地找出文本中的高频词。主要步骤包括数据预处理、分词、去除停用词、词频统计和排序。在处理大规模文本数据时,可以使用生成器或分批次处理数据来减小内存占用。最后,通过词云图可以更直观地展示高频词。希望本文能够帮助你更好地理解和实现文本分析中的高频词统计。
相关问答FAQs:
如何使用Python找出文本中的高频词?
要找出文本中的高频词,首先需要导入相应的库,例如collections
和nltk
。可以通过读取文本文件或直接使用字符串数据,接着使用Counter
类来统计词频,最后根据出现频率排序,筛选出高频词。
在处理中文文本时,有哪些特定的工具或库可以使用?
对于中文文本处理,建议使用jieba
库进行分词。使用jieba
可以将连续的汉字字符串分割成词语,从而有效统计词频。结合使用collections.Counter
,能够快速找出高频词,并且提供中文支持。
如何对高频词进行可视化展示?
可以使用matplotlib
或wordcloud
库对高频词进行可视化。通过绘制条形图或生成词云,可以直观地展示高频词的分布情况。只需将高频词和对应的频率传递给可视化工具,即可生成图形展示。
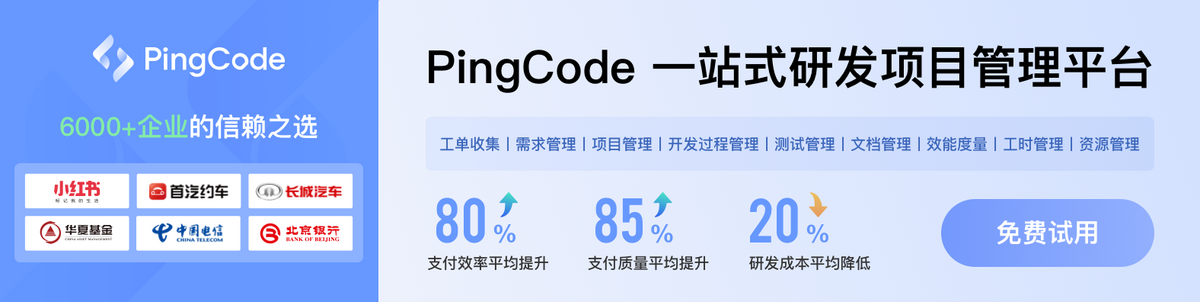