Python解决优化问题的核心方法有:使用优化库、编写自定义优化算法、利用机器学习进行优化。 在这些方法中,使用优化库是最常见且高效的方式。Python提供了多种强大的优化库,如SciPy、PuLP、CVXPY等,这些库包含了丰富的优化算法和功能,可以帮助我们快速解决各种复杂的优化问题。例如,SciPy库中的optimize模块提供了多种优化算法,包括线性规划、非线性规划、最小二乘法等,适用于不同类型的优化问题。
一、使用优化库
1、SciPy库
SciPy是Python中常用的科学计算库,其中的optimize模块提供了多种优化算法,可以解决线性规划、非线性规划、最小二乘法等问题。通过SciPy库,我们可以快速实现优化问题的求解。
线性规划
线性规划是一种常见的优化问题,可以通过SciPy库中的linprog函数来解决。下面是一个简单的例子,展示如何使用linprog函数求解线性规划问题。
from scipy.optimize import linprog
定义目标函数的系数
c = [-1, 4]
定义不等式约束条件的系数矩阵和右侧常数向量
A = [[-3, 1], [1, 2]]
b = [6, 4]
定义变量的上下界
x0_bounds = (None, None)
x1_bounds = (-3, None)
调用linprog函数进行求解
res = linprog(c, A_ub=A, b_ub=b, bounds=[x0_bounds, x1_bounds])
print(res)
非线性规划
对于非线性规划问题,SciPy库提供了minimize函数。下面是一个简单的例子,展示如何使用minimize函数求解非线性规划问题。
from scipy.optimize import minimize
定义目标函数
def objective(x):
return x[0]<strong>2 + x[1]</strong>2
定义约束条件
def constraint1(x):
return x[0] + x[1] - 1
定义初始猜测值
x0 = [0.5, 0.5]
定义约束字典
con1 = {'type': 'eq', 'fun': constraint1}
cons = [con1]
调用minimize函数进行求解
res = minimize(objective, x0, constraints=cons)
print(res)
2、PuLP库
PuLP是一个专门用于线性规划的库,可以帮助我们快速建模和求解线性规划问题。下面是一个简单的例子,展示如何使用PuLP库求解线性规划问题。
import pulp
定义问题
prob = pulp.LpProblem("Simple LP Problem", pulp.LpMinimize)
定义决策变量
x = pulp.LpVariable("x", lowBound=0)
y = pulp.LpVariable("y", lowBound=0)
定义目标函数
prob += x + y
定义约束条件
prob += 2 * x + y >= 4
prob += x + 2 * y >= 5
求解问题
prob.solve()
输出结果
print(f"Optimal value of x: {x.varValue}")
print(f"Optimal value of y: {y.varValue}")
3、CVXPY库
CVXPY是一个专门用于凸优化问题的库,支持多种凸优化问题的求解。下面是一个简单的例子,展示如何使用CVXPY库求解凸优化问题。
import cvxpy as cp
定义变量
x = cp.Variable()
y = cp.Variable()
定义目标函数
objective = cp.Minimize(x<strong>2 + y</strong>2)
定义约束条件
constraints = [x + y == 1]
定义问题
prob = cp.Problem(objective, constraints)
求解问题
prob.solve()
输出结果
print(f"Optimal value of x: {x.value}")
print(f"Optimal value of y: {y.value}")
二、编写自定义优化算法
除了使用现有的优化库,我们还可以根据具体问题编写自定义优化算法。以下是一些常见的优化算法及其实现。
1、梯度下降法
梯度下降法是一种迭代优化算法,通过不断沿着目标函数的负梯度方向移动来找到函数的最小值。下面是一个简单的梯度下降法的实现。
import numpy as np
def gradient_descent(objective, gradient, x0, learning_rate=0.01, max_iter=100):
x = x0
for i in range(max_iter):
grad = gradient(x)
x = x - learning_rate * grad
if np.linalg.norm(grad) < 1e-6:
break
return x
定义目标函数及其梯度
def objective(x):
return x[0]<strong>2 + x[1]</strong>2
def gradient(x):
return np.array([2 * x[0], 2 * x[1]])
初始猜测值
x0 = np.array([1.0, 1.0])
调用梯度下降法
result = gradient_descent(objective, gradient, x0)
print(result)
2、牛顿法
牛顿法是一种利用目标函数二阶导数信息的优化算法,收敛速度较快。下面是一个简单的牛顿法的实现。
import numpy as np
def newton_method(objective, gradient, hessian, x0, max_iter=100):
x = x0
for i in range(max_iter):
grad = gradient(x)
hess = hessian(x)
x = x - np.linalg.inv(hess) @ grad
if np.linalg.norm(grad) < 1e-6:
break
return x
定义目标函数及其梯度和Hessian矩阵
def objective(x):
return x[0]<strong>2 + x[1]</strong>2
def gradient(x):
return np.array([2 * x[0], 2 * x[1]])
def hessian(x):
return np.array([[2, 0], [0, 2]])
初始猜测值
x0 = np.array([1.0, 1.0])
调用牛顿法
result = newton_method(objective, gradient, hessian, x0)
print(result)
3、遗传算法
遗传算法是一种模拟自然选择过程的优化算法,适用于求解全局优化问题。下面是一个简单的遗传算法的实现。
import numpy as np
def genetic_algorithm(objective, bounds, population_size=20, generations=100, mutation_rate=0.01):
def create_individual():
return np.random.uniform(bounds[:, 0], bounds[:, 1])
def crossover(parent1, parent2):
return (parent1 + parent2) / 2
def mutate(individual):
if np.random.rand() < mutation_rate:
idx = np.random.randint(len(individual))
individual[idx] = np.random.uniform(bounds[idx, 0], bounds[idx, 1])
return individual
population = [create_individual() for _ in range(population_size)]
best_individual = min(population, key=objective)
for _ in range(generations):
new_population = []
for _ in range(population_size // 2):
parent1, parent2 = np.random.choice(population, 2)
child1, child2 = crossover(parent1, parent2), crossover(parent2, parent1)
new_population.extend([mutate(child1), mutate(child2)])
population = new_population
best_individual = min(population, key=objective)
return best_individual
定义目标函数
def objective(x):
return x[0]<strong>2 + x[1]</strong>2
定义变量的上下界
bounds = np.array([[-5, 5], [-5, 5]])
调用遗传算法
result = genetic_algorithm(objective, bounds)
print(result)
三、利用机器学习进行优化
机器学习方法在解决复杂优化问题时也具有很大的潜力。通过训练模型,我们可以利用机器学习算法来预测最优解或近似最优解。
1、强化学习
强化学习是一种通过与环境交互来学习最优策略的方法,适用于动态优化问题。以下是一个简单的Q-learning算法的实现。
import numpy as np
class QLearning:
def __init__(self, state_space, action_space, learning_rate=0.1, discount_factor=0.99, exploration_rate=1.0, exploration_decay=0.99):
self.state_space = state_space
self.action_space = action_space
self.q_table = np.zeros((state_space, action_space))
self.learning_rate = learning_rate
self.discount_factor = discount_factor
self.exploration_rate = exploration_rate
self.exploration_decay = exploration_decay
def choose_action(self, state):
if np.random.rand() < self.exploration_rate:
return np.random.choice(self.action_space)
else:
return np.argmax(self.q_table[state, :])
def update_q_table(self, state, action, reward, next_state):
best_next_action = np.argmax(self.q_table[next_state, :])
td_target = reward + self.discount_factor * self.q_table[next_state, best_next_action]
td_error = td_target - self.q_table[state, action]
self.q_table[state, action] += self.learning_rate * td_error
def decay_exploration(self):
self.exploration_rate *= self.exploration_decay
定义环境
class Environment:
def __init__(self):
self.state_space = 5
self.action_space = 2
def reset(self):
return np.random.randint(self.state_space)
def step(self, state, action):
next_state = (state + action) % self.state_space
reward = 1 if next_state == 0 else 0
return next_state, reward
训练Q-learning算法
env = Environment()
agent = QLearning(env.state_space, env.action_space)
for episode in range(1000):
state = env.reset()
done = False
while not done:
action = agent.choose_action(state)
next_state, reward = env.step(state, action)
agent.update_q_table(state, action, reward, next_state)
state = next_state
if next_state == 0:
done = True
agent.decay_exploration()
输出Q-table
print(agent.q_table)
2、神经网络优化
神经网络可以用来构建复杂的优化模型,通过训练网络,我们可以近似最优解或直接预测最优解。以下是一个简单的神经网络优化的实现。
import numpy as np
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
生成训练数据
def generate_data(num_samples):
X = np.random.uniform(-5, 5, (num_samples, 2))
y = np.sum(X2, axis=1)
return X, y
定义神经网络模型
model = Sequential([
Dense(64, activation='relu', input_shape=(2,)),
Dense(64, activation='relu'),
Dense(1)
])
编译模型
model.compile(optimizer='adam', loss='mse')
生成训练数据
X_train, y_train = generate_data(1000)
训练模型
model.fit(X_train, y_train, epochs=100, batch_size=32)
测试模型
X_test = np.array([[1.0, 1.0], [-1.0, -1.0], [0.5, -0.5]])
y_pred = model.predict(X_test)
print(y_pred)
通过上述方法,我们可以利用Python解决各种优化问题。根据具体需求选择合适的方法和工具,可以提高优化问题的求解效率和效果。
相关问答FAQs:
Python在优化问题中可以如何应用?
Python在优化问题中主要通过各种库和工具进行处理。常用的库包括SciPy、NumPy和Pandas等,SciPy提供了多种优化算法,可以用于线性和非线性优化、约束问题等。用户可以利用这些库实现复杂的数学模型,进行数据分析和优化计算。此外,机器学习库如scikit-learn也可以用于优化模型参数,提高模型预测的准确性。
在Python中使用哪些库可以解决线性规划问题?
解决线性规划问题时,用户可以使用PuLP、SciPy中的linprog函数或CVXPY等库。PuLP是一个非常直观的线性规划库,允许用户以Python对象的形式定义变量、目标函数和约束条件。SciPy的linprog函数则可以通过简单的接口快速求解线性规划问题,而CVXPY则提供了更灵活的建模能力,适合处理更复杂的优化问题。
如何评估Python优化算法的性能?
评估Python优化算法性能的方法包括计算收敛速度、解决问题的准确性和计算时间等。用户可以通过比较不同算法在相同问题上的表现来选择最合适的算法。此外,交叉验证技术可以帮助评估模型在不同数据集上的稳定性和泛化能力。针对特定问题,用户还可以使用可视化工具,如Matplotlib,来展示优化过程和结果,进一步分析算法的有效性。
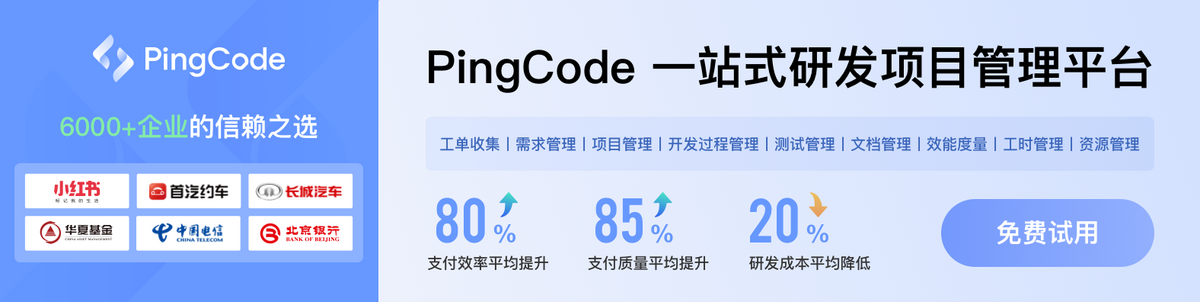