在Python中输出字符换行的方式有很多种。可以使用换行符\n
、使用多行字符串、以及使用print函数的sep
和end
参数。其中,使用换行符\n
是最常见和最直接的方法。
一、使用换行符\n
换行符\n
是一个特殊的字符,用来指示文本换行。在Python中,可以直接在字符串中插入\n
来实现换行。
print("Hello\nWorld")
上面的代码会输出:
Hello
World
二、使用多行字符串
多行字符串是用三重引号('''
或 """
)包围的字符串,可以直接在字符串中换行。
print("""Hello
World""")
这段代码同样会输出:
Hello
World
三、使用print函数的sep
和end
参数
sep
参数是用来设置多个参数之间的分隔符,而end
参数是用来设置输出结束时的字符,默认是换行符'\n'
。
print("Hello", "World", sep="\n")
这段代码会输出:
Hello
World
你也可以通过修改end
参数来控制输出的结束符。
print("Hello", end=" ")
print("World")
这段代码会输出:
Hello World
使用换行符\n
详细描述
使用换行符\n
是最常见和最直接的方法,因为它可以灵活地插入到任意位置的字符串中,不局限于打印函数。换行符在很多编程语言中都有类似的功能,这使得它在跨语言编程中更为通用。比如在日志记录、生成多行文本文件或者处理输出格式时,\n
都非常有用。
text = "First Line\nSecond Line\nThird Line"
print(text)
这段代码会输出:
First Line
Second Line
Third Line
换行符还可以与其他字符串操作结合使用,比如拼接字符串:
line1 = "First Line"
line2 = "Second Line"
line3 = "Third Line"
text = line1 + "\n" + line2 + "\n" + line3
print(text)
这段代码会输出:
First Line
Second Line
Third Line
通过这种方式,你可以在任何需要的地方插入换行符,非常方便。
四、Python中的字符串操作
Python中的字符串操作非常强大,除了换行符,还有很多其他的操作方法,比如拼接、分割、替换等。
字符串拼接
字符串拼接可以使用加号+
或者join
方法。
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result)
这段代码会输出:
Hello World
使用join
方法:
words = ["Hello", "World"]
result = " ".join(words)
print(result)
这段代码会输出:
Hello World
字符串分割
字符串分割可以使用split
方法。
text = "Hello World"
words = text.split()
print(words)
这段代码会输出:
['Hello', 'World']
你可以指定分隔符:
text = "Hello,World"
words = text.split(',')
print(words)
这段代码会输出:
['Hello', 'World']
字符串替换
字符串替换可以使用replace
方法。
text = "Hello World"
new_text = text.replace("World", "Python")
print(new_text)
这段代码会输出:
Hello Python
字符串格式化
Python提供了多种字符串格式化方法,最常用的是format
方法和f字符串。
name = "World"
text = "Hello, {}".format(name)
print(text)
这段代码会输出:
Hello, World
使用f字符串:
name = "World"
text = f"Hello, {name}"
print(text)
这段代码会输出:
Hello, World
字符串索引和切片
字符串可以像列表一样进行索引和切片操作。
text = "Hello World"
first_char = text[0]
print(first_char)
这段代码会输出:
H
切片操作:
text = "Hello World"
first_word = text[:5]
print(first_word)
这段代码会输出:
Hello
字符串的常见方法
Python的字符串对象提供了很多内置方法,比如upper
、lower
、strip
等。
将字符串转换为大写:
text = "Hello World"
upper_text = text.upper()
print(upper_text)
这段代码会输出:
HELLO WORLD
将字符串转换为小写:
text = "Hello World"
lower_text = text.lower()
print(lower_text)
这段代码会输出:
hello world
去掉字符串两端的空白字符:
text = " Hello World "
stripped_text = text.strip()
print(stripped_text)
这段代码会输出:
Hello World
多行字符串的使用场景
多行字符串在编写文档字符串(docstring)、多行注释和生成多行文本时非常有用。文档字符串用于描述模块、类或函数的目的和用法。
def greet(name):
"""
This function greets the person passed as a parameter.
Parameters:
name (str): The name of the person to greet
Returns:
None
"""
print(f"Hello, {name}")
在这个例子中,三重引号内的内容是函数的文档字符串,用于解释函数的用途和参数。
多行字符串还可以用于生成多行文本,比如电子邮件内容、代码生成等。
email_content = """
Dear John,
I hope this email finds you well.
Best regards,
Jane
"""
print(email_content)
这段代码会输出:
Dear John,
I hope this email finds you well.
Best regards,
Jane
使用print函数的高级技巧
Python的print
函数提供了很多高级技巧,可以更灵活地控制输出格式。除了sep
和end
参数,还可以使用file
参数将输出重定向到文件。
with open("output.txt", "w") as file:
print("Hello, World", file=file)
这段代码会将Hello, World
写入output.txt
文件中。
你还可以使用flush
参数立即刷新输出缓冲区:
import time
for i in range(5):
print(i, end=" ", flush=True)
time.sleep(1)
这段代码会每隔一秒输出一个数字,而不是等待循环结束后一起输出。
Python中的字符串与编码
Python中的字符串是Unicode字符序列,这使得它们可以处理各种国际字符集。然而,文件读写和网络通信中,字符串通常需要以字节形式表示。Python提供了encode
和decode
方法来进行字符串与字节之间的转换。
text = "Hello, World"
encoded_text = text.encode("utf-8")
print(encoded_text)
这段代码会输出:
b'Hello, World'
将字节转换回字符串:
decoded_text = encoded_text.decode("utf-8")
print(decoded_text)
这段代码会输出:
Hello, World
字符串与正则表达式
正则表达式是一种强大的字符串处理工具,可以用于匹配、查找、替换字符串中的模式。Python的re
模块提供了正则表达式支持。
匹配字符串中的模式:
import re
text = "The rain in Spain"
match = re.search(r"rain", text)
if match:
print("Match found:", match.group())
这段代码会输出:
Match found: rain
查找所有匹配:
matches = re.findall(r"in", text)
print("Matches found:", matches)
这段代码会输出:
Matches found: ['in', 'in']
替换字符串中的模式:
new_text = re.sub(r"Spain", "Italy", text)
print(new_text)
这段代码会输出:
The rain in Italy
字符串与文件操作
Python提供了方便的文件操作方法,可以读写字符串到文件中。
写入字符串到文件:
text = "Hello, World"
with open("output.txt", "w") as file:
file.write(text)
从文件中读取字符串:
with open("output.txt", "r") as file:
content = file.read()
print(content)
这段代码会输出文件中的内容:
Hello, World
字符串与数据转换
在处理数据时,通常需要在字符串与其他数据类型之间进行转换。Python提供了很多内置函数来进行这些转换。
将字符串转换为整数:
number_str = "123"
number = int(number_str)
print(number)
这段代码会输出:
123
将字符串转换为浮点数:
float_str = "123.45"
number = float(float_str)
print(number)
这段代码会输出:
123.45
将数字转换为字符串:
number = 123
number_str = str(number)
print(number_str)
这段代码会输出:
123
将列表转换为字符串:
list_items = ["apple", "banana", "cherry"]
list_str = ", ".join(list_items)
print(list_str)
这段代码会输出:
apple, banana, cherry
字符串与日期时间
在处理日期和时间时,通常需要将日期时间对象转换为字符串,或者将字符串解析为日期时间对象。Python的datetime
模块提供了这些功能。
将日期时间对象转换为字符串:
from datetime import datetime
now = datetime.now()
now_str = now.strftime("%Y-%m-%d %H:%M:%S")
print(now_str)
这段代码会输出当前日期和时间:
2023-10-12 15:45:30
将字符串解析为日期时间对象:
date_str = "2023-10-12 15:45:30"
date_obj = datetime.strptime(date_str, "%Y-%m-%d %H:%M:%S")
print(date_obj)
这段代码会输出解析后的日期时间对象:
2023-10-12 15:45:30
字符串与JSON
JSON(JavaScript Object Notation)是一种常用的数据交换格式。Python的json
模块提供了方便的函数来处理JSON数据。
将Python对象转换为JSON字符串:
import json
data = {"name": "John", "age": 30}
json_str = json.dumps(data)
print(json_str)
这段代码会输出:
{"name": "John", "age": 30}
将JSON字符串转换为Python对象:
json_str = '{"name": "John", "age": 30}'
data = json.loads(json_str)
print(data)
这段代码会输出:
{'name': 'John', 'age': 30}
字符串与XML
XML(eXtensible Markup Language)是一种常用的数据表示格式。Python的xml
模块提供了处理XML的函数。
将Python对象转换为XML字符串:
import xml.etree.ElementTree as ET
data = ET.Element("data")
name = ET.SubElement(data, "name")
name.text = "John"
age = ET.SubElement(data, "age")
age.text = "30"
xml_str = ET.tostring(data, encoding="unicode")
print(xml_str)
这段代码会输出:
<data><name>John</name><age>30</age></data>
将XML字符串解析为Python对象:
xml_str = "<data><name>John</name><age>30</age></data>"
data = ET.fromstring(xml_str)
print(data.find("name").text)
print(data.find("age").text)
这段代码会输出:
John
30
字符串与CSV
CSV(Comma-Separated Values)是一种常用的数据存储格式。Python的csv
模块提供了读写CSV文件的函数。
将Python对象写入CSV文件:
import csv
data = [["name", "age"], ["John", 30], ["Jane", 25]]
with open("output.csv", "w", newline="") as file:
writer = csv.writer(file)
writer.writerows(data)
从CSV文件中读取数据:
with open("output.csv", "r") as file:
reader = csv.reader(file)
for row in reader:
print(row)
这段代码会输出CSV文件中的内容:
['name', 'age']
['John', '30']
['Jane', '25']
字符串与YAML
YAML(YAML Ain't Markup Language)是一种常用的数据表示格式,尤其在配置文件中。Python的yaml
模块提供了处理YAML的函数。
将Python对象转换为YAML字符串:
import yaml
data = {"name": "John", "age": 30}
yaml_str = yaml.dump(data)
print(yaml_str)
这段代码会输出:
age: 30
name: John
将YAML字符串解析为Python对象:
yaml_str = "age: 30\nname: John\n"
data = yaml.load(yaml_str, Loader=yaml.FullLoader)
print(data)
这段代码会输出:
{'age': 30, 'name': 'John'}
字符串与HTML
HTML(HyperText Markup Language)是一种常用的网页标记语言。Python的html
模块提供了处理HTML的函数。
将字符串转换为HTML实体:
import html
text = "This is a <b>bold</b> text."
html_str = html.escape(text)
print(html_str)
这段代码会输出:
This is a <b>bold</b> text.
将HTML实体转换回字符串:
html_str = "This is a <b>bold</b> text."
text = html.unescape(html_str)
print(text)
这段代码会输出:
This is a <b>bold</b> text.
字符串与URL编码
URL编码用于在URL中包含特殊字符。Python的urllib.parse
模块提供了处理URL编码的函数。
将字符串进行URL编码:
import urllib.parse
text = "Hello, World!"
encoded_text = urllib.parse.quote(text)
print(encoded_text)
这段代码会输出:
Hello%2C%20World%21
将URL编码的字符串解码:
encoded_text = "Hello%2C%20World%21"
text = urllib.parse.unquote(encoded_text)
print(text)
这段代码会输出:
Hello, World!
通过以上介绍,你可以看到Python中的字符串操作非常丰富和强大。无论是基本的字符串操作,还是与文件、数据格式、编码等的结合使用,Python都提供了很好的支持。希望这些内容能帮助你更好地理解和使用Python中的字符串操作。
相关问答FAQs:
如何在Python中实现字符串的换行?
在Python中,可以使用转义字符\n
来实现字符串的换行。例如:
print("Hello\nWorld")
这段代码会输出:
Hello
World
您还可以在多行字符串中直接使用三重引号('''
或"""
),这将保留换行格式。示例如下:
print("""Hello
World""")
在Python中换行是否会影响字符串的格式?
换行在字符串中是有意义的,它会使输出的内容更加整洁和可读。如果您希望在控制台或文件中以特定格式显示信息,使用换行符可以帮助您实现这一点。然而,换行可能会在某些情况下影响数据的处理,特别是在解析或读取字符串时。
是否可以在字符串中使用其他换行方式?
除了使用\n
换行外,您还可以使用str.join()
方法与列表结合来创建多行字符串。例如:
lines = ["Hello", "World"]
output = "\n".join(lines)
print(output)
这种方式在处理动态生成的多行文本时非常有用,可以更灵活地插入换行符。
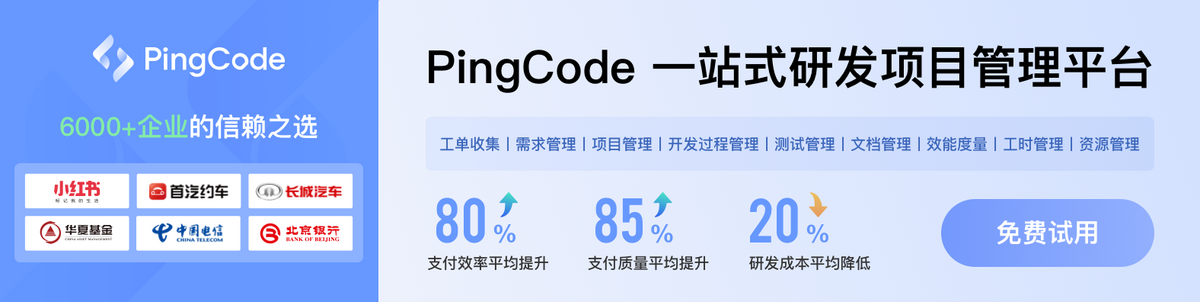