使用Python进行每日打卡,可以通过编写一个定时任务脚本来实现。我们可以通过使用Python的schedule库来进行任务调度、使用requests库来进行网络请求、结合一些API实现打卡功能。其中,定时任务调度是核心部分。
让我们详细讲解如何使用schedule库来实现定时任务调度。
一、安装所需库
首先,你需要安装所需的Python库。你可以使用pip来安装这些库:
pip install schedule requests
二、创建配置文件
创建一个配置文件来保存打卡信息,例如账号、密码、打卡时间等。可以使用JSON文件来保存这些配置信息。
{
"username": "your_username",
"password": "your_password",
"check_in_time": "08:00"
}
三、编写打卡脚本
1、导入必要的库
import schedule
import time
import requests
import json
2、加载配置文件
def load_config():
with open('config.json', 'r') as f:
config = json.load(f)
return config
3、定义打卡函数
def check_in():
config = load_config()
username = config['username']
password = config['password']
# 这里以一个假设的打卡接口为例
login_url = "https://example.com/api/login"
check_in_url = "https://example.com/api/checkin"
# 登录
login_payload = {
'username': username,
'password': password
}
session = requests.Session()
response = session.post(login_url, data=login_payload)
if response.status_code == 200:
# 打卡
check_in_response = session.post(check_in_url)
if check_in_response.status_code == 200:
print("打卡成功")
else:
print("打卡失败")
else:
print("登录失败")
4、设置定时任务
def schedule_check_in():
config = load_config()
check_in_time = config['check_in_time']
schedule.every().day.at(check_in_time).do(check_in)
while True:
schedule.run_pending()
time.sleep(1)
5、运行脚本
if __name__ == "__main__":
schedule_check_in()
四、完善打卡脚本
1、增加日志记录功能
记录打卡成功或失败的日志信息,以便后续查看。
import logging
logging.basicConfig(filename='check_in.log', level=logging.INFO)
def log_message(message):
logging.info(f"{time.strftime('%Y-%m-%d %H:%M:%S')} - {message}")
在打卡函数中调用日志记录功能:
def check_in():
config = load_config()
username = config['username']
password = config['password']
login_url = "https://example.com/api/login"
check_in_url = "https://example.com/api/checkin"
login_payload = {
'username': username,
'password': password
}
session = requests.Session()
response = session.post(login_url, data=login_payload)
if response.status_code == 200:
check_in_response = session.post(check_in_url)
if check_in_response.status_code == 200:
log_message("打卡成功")
else:
log_message("打卡失败")
else:
log_message("登录失败")
2、增加异常处理
在打卡函数中增加异常处理,确保脚本在发生异常时不会中断。
def check_in():
try:
config = load_config()
username = config['username']
password = config['password']
login_url = "https://example.com/api/login"
check_in_url = "https://example.com/api/checkin"
login_payload = {
'username': username,
'password': password
}
session = requests.Session()
response = session.post(login_url, data=login_payload)
if response.status_code == 200:
check_in_response = session.post(check_in_url)
if check_in_response.status_code == 200:
log_message("打卡成功")
else:
log_message("打卡失败")
else:
log_message("登录失败")
except Exception as e:
log_message(f"打卡时发生异常: {e}")
五、将脚本设为后台运行
在Linux系统中,可以使用nohup命令将脚本设为后台运行:
nohup python3 check_in.py &
在Windows系统中,可以使用任务计划程序来实现脚本的定时运行和后台执行。
六、总结
通过上述步骤,我们实现了一个简单的Python每日打卡脚本。关键步骤包括安装必要的库、创建配置文件、编写打卡函数、设置定时任务、增加日志记录和异常处理。你可以根据自己的需求进一步完善和扩展这个脚本,例如通过邮件或短信通知打卡结果。
七、扩展功能
1、邮件通知
可以通过Python的smtplib库发送邮件通知打卡结果。
import smtplib
from email.mime.text import MIMEText
def send_email(subject, content):
config = load_config()
sender = config['email_sender']
receiver = config['email_receiver']
smtp_server = config['smtp_server']
smtp_port = config['smtp_port']
smtp_user = config['smtp_user']
smtp_password = config['smtp_password']
msg = MIMEText(content)
msg['Subject'] = subject
msg['From'] = sender
msg['To'] = receiver
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.login(smtp_user, smtp_password)
server.sendmail(sender, receiver, msg.as_string())
在打卡函数中调用发送邮件通知功能:
def check_in():
try:
config = load_config()
username = config['username']
password = config['password']
login_url = "https://example.com/api/login"
check_in_url = "https://example.com/api/checkin"
login_payload = {
'username': username,
'password': password
}
session = requests.Session()
response = session.post(login_url, data=login_payload)
if response.status_code == 200:
check_in_response = session.post(check_in_url)
if check_in_response.status_code == 200:
log_message("打卡成功")
send_email("打卡通知", "打卡成功")
else:
log_message("打卡失败")
send_email("打卡通知", "打卡失败")
else:
log_message("登录失败")
send_email("打卡通知", "登录失败")
except Exception as e:
log_message(f"打卡时发生异常: {e}")
send_email("打卡通知", f"打卡时发生异常: {e}")
2、短信通知
可以通过第三方短信服务API发送短信通知打卡结果。例如,可以使用Twilio的API发送短信。
首先,安装Twilio的Python库:
pip install twilio
然后,编写发送短信通知的函数:
from twilio.rest import Client
def send_sms(content):
config = load_config()
account_sid = config['twilio_account_sid']
auth_token = config['twilio_auth_token']
from_number = config['twilio_from_number']
to_number = config['twilio_to_number']
client = Client(account_sid, auth_token)
message = client.messages.create(
body=content,
from_=from_number,
to=to_number
)
在打卡函数中调用发送短信通知功能:
def check_in():
try:
config = load_config()
username = config['username']
password = config['password']
login_url = "https://example.com/api/login"
check_in_url = "https://example.com/api/checkin"
login_payload = {
'username': username,
'password': password
}
session = requests.Session()
response = session.post(login_url, data=login_payload)
if response.status_code == 200:
check_in_response = session.post(check_in_url)
if check_in_response.status_code == 200:
log_message("打卡成功")
send_email("打卡通知", "打卡成功")
send_sms("打卡成功")
else:
log_message("打卡失败")
send_email("打卡通知", "打卡失败")
send_sms("打卡失败")
else:
log_message("登录失败")
send_email("打卡通知", "登录失败")
send_sms("登录失败")
except Exception as e:
log_message(f"打卡时发生异常: {e}")
send_email("打卡通知", f"打卡时发生异常: {e}")
send_sms(f"打卡时发生异常: {e}")
八、测试与部署
在实际使用前,应该对脚本进行充分的测试,确保打卡功能正常运行,并且能够正确发送通知。
1、测试脚本
可以修改配置文件中的打卡时间为当前时间的几分钟后,观察脚本是否能正常执行打卡操作,并发送通知。
2、部署脚本
可以将脚本部署到云服务器上,确保脚本能够每天按时运行。可以使用nohup命令或其他方式将脚本设为后台运行。
3、监控运行状态
可以通过查看日志文件、接收邮件或短信通知来监控脚本的运行状态,确保打卡功能正常。
九、总结
通过上述步骤,我们实现了一个功能完善的Python每日打卡脚本。关键步骤包括安装必要的库、创建配置文件、编写打卡函数、设置定时任务、增加日志记录和异常处理、实现邮件和短信通知功能。你可以根据自己的需求进一步完善和扩展这个脚本,确保每日打卡顺利进行。
相关问答FAQs:
如何用Python创建一个每日打卡程序?
要创建一个每日打卡程序,可以使用Python的内置模块如datetime来记录打卡时间,并结合文件操作将打卡记录保存到本地文件。你可以使用一个简单的命令行界面,让用户输入打卡信息,并将这些信息写入一个文本文件。还可以使用定时任务(如cron或Windows任务计划程序)来自动运行该脚本。
我可以使用哪些Python库来增强打卡程序的功能?
为了提高打卡程序的功能,推荐使用像pandas库来处理和分析打卡数据,或者使用sqlite3库来创建一个简单的数据库存储打卡记录。此外,使用Flask或Django等框架可以将程序转变为一个Web应用,使用户能够通过网页进行打卡。
如何确保我的打卡程序不会重复记录同一天的打卡?
可以在打卡程序中添加逻辑,以检查当天是否已经存在打卡记录。通过读取存储打卡记录的文件或数据库,获取当前日期并与最新记录进行比较,如果存在当天的记录,则可以提示用户已打卡,无需重复打卡。
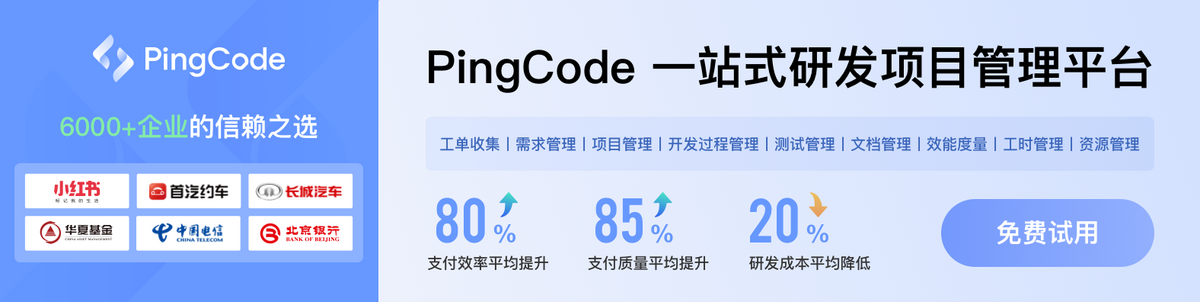