Python可以通过多种方式来修改文件内容,包括使用内置的文件操作函数、第三方库和一些特定的文件格式处理库。 常见的方法包括使用内置的open()
函数进行读写操作、使用shutil
库进行文件复制和移动、使用os
库进行文件重命名和删除、以及使用第三方库如pandas
处理特定类型的文件。下面将详细介绍如何通过这些方法来修改文件内容。
一、使用内置的open()
函数进行文件读写
Python内置的open()
函数是操作文件的基础工具,支持多种模式如读取、写入和追加。下面是一些常见的文件操作方法。
1. 读取文件内容
读取文件内容是修改文件的第一步,了解文件的当前内容有助于确定需要修改的部分。可以使用open()
函数以读取模式('r'
)打开文件,然后使用read()
方法读取整个文件内容,或使用readlines()
方法逐行读取文件内容。
with open('example.txt', 'r') as file:
content = file.read()
print(content)
2. 写入文件内容
写入文件内容可以分为覆盖写入和追加写入。覆盖写入会清空文件的原有内容,而追加写入则会在文件末尾添加新内容。
覆盖写入:
with open('example.txt', 'w') as file:
file.write('This is new content.')
追加写入:
with open('example.txt', 'a') as file:
file.write('\nThis is appended content.')
3. 修改特定内容
修改文件中的特定内容需要先读取文件内容,然后进行相应的修改,再将修改后的内容写回文件。
# 读取文件内容
with open('example.txt', 'r') as file:
lines = file.readlines()
修改特定内容
lines = [line.replace('old_string', 'new_string') for line in lines]
写回文件
with open('example.txt', 'w') as file:
file.writelines(lines)
二、使用shutil
库进行文件操作
shutil
库提供了高级的文件操作功能,如复制、移动和删除文件。它可以与os
库结合使用,实现更多文件操作。
1. 复制文件
使用shutil.copyfile()
函数可以复制文件内容到新文件中。
import shutil
shutil.copyfile('source.txt', 'destination.txt')
2. 移动文件
使用shutil.move()
函数可以移动文件到新位置。
import shutil
shutil.move('source.txt', 'destination.txt')
3. 删除文件
使用os.remove()
函数可以删除文件。
import os
os.remove('example.txt')
三、使用pandas
库处理特定类型的文件
对于处理特定类型的文件,如CSV文件,pandas
库提供了强大的数据处理功能。可以使用pandas
库读取、修改和写入CSV文件。
1. 读取CSV文件
使用pandas.read_csv()
函数可以读取CSV文件内容。
import pandas as pd
df = pd.read_csv('example.csv')
print(df)
2. 修改CSV文件内容
可以直接修改DataFrame
对象,然后将修改后的内容写回CSV文件。
# 修改特定列的内容
df['column_name'] = df['column_name'].replace('old_value', 'new_value')
写回CSV文件
df.to_csv('example.csv', index=False)
四、使用其他第三方库处理特定文件格式
除了pandas
,还有许多其他第三方库可以处理特定的文件格式,如openpyxl
处理Excel文件、json
处理JSON文件、xml.etree.ElementTree
处理XML文件等。
1. 处理Excel文件
使用openpyxl
库可以读取和修改Excel文件。
import openpyxl
读取Excel文件
wb = openpyxl.load_workbook('example.xlsx')
sheet = wb.active
修改单元格内容
sheet['A1'] = 'New Value'
保存修改
wb.save('example.xlsx')
2. 处理JSON文件
使用json
库可以读取和修改JSON文件。
import json
读取JSON文件
with open('example.json', 'r') as file:
data = json.load(file)
修改内容
data['key'] = 'new_value'
写回JSON文件
with open('example.json', 'w') as file:
json.dump(data, file, indent=4)
3. 处理XML文件
使用xml.etree.ElementTree
库可以读取和修改XML文件。
import xml.etree.ElementTree as ET
读取XML文件
tree = ET.parse('example.xml')
root = tree.getroot()
修改内容
for elem in root.iter('tag_name'):
elem.text = 'new_value'
保存修改
tree.write('example.xml')
五、处理二进制文件
二进制文件的操作与文本文件不同,需要以二进制模式打开文件进行读写。这通常用于处理图片、音频和其他非文本文件。
1. 读取二进制文件
with open('example.bin', 'rb') as file:
content = file.read()
print(content)
2. 写入二进制文件
with open('example.bin', 'wb') as file:
file.write(b'This is binary content.')
3. 修改二进制文件
修改二进制文件内容需要谨慎,通常是读取文件内容到字节数组中进行操作,然后写回文件。
# 读取二进制文件内容
with open('example.bin', 'rb') as file:
content = file.read()
修改内容
modified_content = content.replace(b'old_value', b'new_value')
写回二进制文件
with open('example.bin', 'wb') as file:
file.write(modified_content)
六、使用临时文件进行安全修改
在修改文件内容时,为了避免数据丢失和文件损坏,可以使用临时文件进行操作。tempfile
库提供了创建临时文件的功能,可以在修改完成后替换原文件。
import tempfile
import shutil
创建临时文件
with tempfile.NamedTemporaryFile(delete=False) as temp_file:
temp_file.write(b'New content')
替换原文件
shutil.move(temp_file.name, 'example.txt')
七、处理大文件
处理大文件时,直接读取整个文件内容到内存可能导致内存不足。可以采用逐行读取和写入的方法。
1. 逐行读取和写入
with open('large_example.txt', 'r') as read_file, open('modified_example.txt', 'w') as write_file:
for line in read_file:
modified_line = line.replace('old_value', 'new_value')
write_file.write(modified_line)
八、使用多线程和多进程处理文件
对于需要并行处理的文件操作,可以使用多线程和多进程技术来提高效率。
1. 多线程处理文件
import threading
def modify_file(file_path):
with open(file_path, 'r+') as file:
content = file.read()
modified_content = content.replace('old_value', 'new_value')
file.seek(0)
file.write(modified_content)
file.truncate()
threads = []
for i in range(5):
thread = threading.Thread(target=modify_file, args=(f'example_{i}.txt',))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
2. 多进程处理文件
import multiprocessing
def modify_file(file_path):
with open(file_path, 'r+') as file:
content = file.read()
modified_content = content.replace('old_value', 'new_value')
file.seek(0)
file.write(modified_content)
file.truncate()
processes = []
for i in range(5):
process = multiprocessing.Process(target=modify_file, args=(f'example_{i}.txt',))
processes.append(process)
process.start()
for process in processes:
process.join()
九、文件权限和错误处理
在修改文件时,可能会遇到文件权限不足和其他错误,需要进行错误处理和权限管理。
1. 文件权限检查
可以使用os.access()
函数检查文件的读写权限。
import os
if os.access('example.txt', os.W_OK):
print('File is writable')
else:
print('File is not writable')
2. 错误处理
使用try-except
块进行错误处理,确保程序在遇到错误时不会崩溃。
try:
with open('example.txt', 'r+') as file:
content = file.read()
file.seek(0)
file.write('New content')
file.truncate()
except PermissionError:
print('Permission denied')
except FileNotFoundError:
print('File not found')
except Exception as e:
print(f'An error occurred: {e}')
十、总结
通过上述方法,Python可以灵活地实现文件内容的修改。无论是使用内置函数、标准库还是第三方库,都需要根据具体需求选择合适的方法,并注意文件操作的安全性和效率。希望这些方法能够帮助你在实际项目中更好地处理文件内容。
相关问答FAQs:
如何在Python中读取文件并修改其内容?
在Python中,可以使用内置的open()
函数以读写模式打开文件。通过读取文件内容到内存中,进行所需的修改后,再将修改后的内容写回文件。使用with
语句可以确保文件在操作后自动关闭,避免资源泄露。例如:
with open('example.txt', 'r') as file:
content = file.read()
modified_content = content.replace('旧内容', '新内容')
with open('example.txt', 'w') as file:
file.write(modified_content)
这种方式可以有效地读取和修改文件内容。
在Python中如何处理文件的逐行修改?
如果文件较大,不适合一次性读取到内存中,可以逐行读取并修改。使用open()
函数以读取模式打开文件,结合循环和条件判断,逐行处理。以下是一个示例:
with open('example.txt', 'r') as file:
lines = file.readlines()
with open('example.txt', 'w') as file:
for line in lines:
modified_line = line.replace('旧内容', '新内容')
file.write(modified_line)
这种方法可以高效地逐行修改文件内容。
Python如何在不覆盖原文件的情况下进行修改?
如果希望保留原文件并在修改后创建一个新文件,可以在写入时指定一个不同的文件名。这样可以避免数据丢失,并可以随时恢复原始文件。例如:
with open('example.txt', 'r') as file:
content = file.read()
modified_content = content.replace('旧内容', '新内容')
with open('new_example.txt', 'w') as new_file:
new_file.write(modified_content)
采用这种方式,可以在不影响原文件的情况下完成修改。
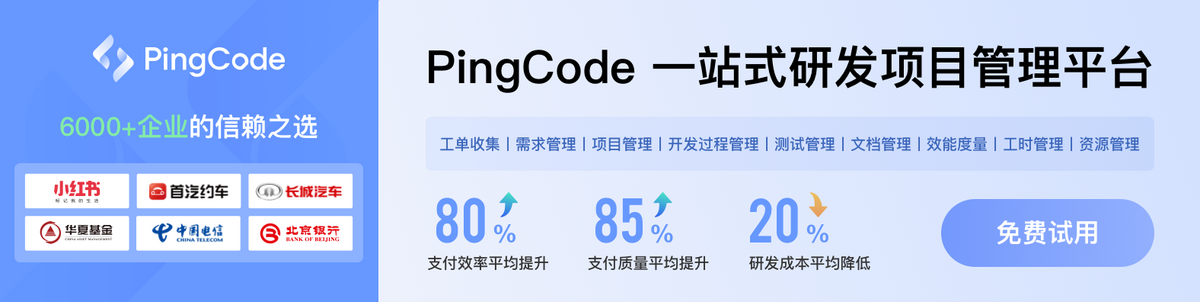