在Python中,用代码打开文件可以使用内置的open()
函数、with
语句、以及os
、pathlib
等模块。 其中,open()
函数是最常用的方法。以下将详细介绍如何使用这些方法来打开和处理文件。
使用open()
函数
open()
函数是Python中最常用的打开文件的方法,它可以打开文件进行读、写或追加操作。使用open()
函数时,需要指定文件名和操作模式:
file = open('example.txt', 'r')
在这个例子中,'example.txt'
是文件名,'r'
是操作模式,表示以读模式打开文件。以下是一些常用的操作模式:
'r'
: 以读模式打开文件(默认)。'w'
: 以写模式打开文件,若文件存在则覆盖,不存在则创建。'a'
: 以追加模式打开文件,若文件存在则在末尾追加,不存在则创建。'b'
: 以二进制模式打开文件,例如'rb'
,'wb'
。'+'
: 读/写模式,例如'r+'
,'w+'
,'a+'
。
使用open()
函数打开文件后,需要记得关闭文件,以释放系统资源:
file.close()
使用with
语句
在打开文件进行读写操作时,推荐使用with
语句,这样可以确保文件在操作完成后自动关闭,避免资源泄漏。with
语句的基本用法如下:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
在这个例子中,with
语句会在代码块执行完毕后自动关闭文件,无需显式调用close()
方法。使用with
语句的好处是更加简洁、安全。
使用os
模块
os
模块提供了与操作系统交互的方法,可以用于文件的打开和操作。虽然os
模块不直接提供打开文件的方法,但可以结合os
模块的其他方法进行文件操作。
例如,可以使用os.path
模块检查文件是否存在,然后再使用open()
函数打开文件:
import os
if os.path.exists('example.txt'):
with open('example.txt', 'r') as file:
content = file.read()
print(content)
else:
print('File does not exist.')
使用pathlib
模块
pathlib
模块提供了面向对象的方法来处理文件和路径。Python 3.4及以上版本推荐使用pathlib
模块,它使代码更具可读性和可维护性。
from pathlib import Path
file_path = Path('example.txt')
if file_path.exists():
with file_path.open('r') as file:
content = file.read()
print(content)
else:
print('File does not exist.')
pathlib
模块中的Path
对象可以方便地进行路径操作和文件操作,是现代Python编程中的推荐选择。
一、open()
函数的详细使用
open()
函数是Python中打开文件的基础方法。它的基本语法如下:
open(file, mode='r', buffering=-1, encoding=None, errors=None, newline=None, closefd=True, opener=None)
file
: 文件路径(字符串),必须参数。mode
: 文件打开模式(字符串),默认为'r'
。buffering
: 缓冲策略,默认为 -1 表示使用系统默认策略。encoding
: 文件编码,默认为None
,一般用于文本文件。errors
: 错误处理策略。newline
: 控制换行符的处理。closefd
: 文件描述符,默认为True
。opener
: 自定义打开器。
读文件
以读模式打开文件,可以读取文件内容。常用的读模式有 'r'
(文本模式)和 'rb'
(二进制模式)。
# 读文本文件
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
读二进制文件
with open('example.bin', 'rb') as file:
content = file.read()
print(content)
写文件
以写模式打开文件,可以写入文件内容。常用的写模式有 'w'
(文本模式)和 'wb'
(二进制模式)。
# 写文本文件
with open('example.txt', 'w', encoding='utf-8') as file:
file.write('Hello, World!')
写二进制文件
with open('example.bin', 'wb') as file:
file.write(b'\x00\x01\x02\x03')
追加文件
以追加模式打开文件,可以在文件末尾追加内容。常用的追加模式有 'a'
(文本模式)和 'ab'
(二进制模式)。
# 追加文本文件
with open('example.txt', 'a', encoding='utf-8') as file:
file.write('Hello, again!')
追加二进制文件
with open('example.bin', 'ab') as file:
file.write(b'\x04\x05\x06\x07')
二、with
语句的优势
with
语句用于简化文件操作,确保文件使用完毕后自动关闭,避免资源泄漏。它的基本语法如下:
with open('example.txt', 'r') as file:
# 进行文件操作
pass
读文件
使用with
语句读取文件内容,确保文件会在代码块结束后自动关闭。
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
写文件
使用with
语句写入文件内容,确保文件会在代码块结束后自动关闭。
with open('example.txt', 'w', encoding='utf-8') as file:
file.write('Hello, World!')
追加文件
使用with
语句追加文件内容,确保文件会在代码块结束后自动关闭。
with open('example.txt', 'a', encoding='utf-8') as file:
file.write('Hello, again!')
三、os
模块的使用
os
模块提供了与操作系统交互的方法,可以用于文件和目录操作。虽然os
模块不直接提供打开文件的方法,但可以结合open()
函数进行文件操作。
检查文件是否存在
使用os.path.exists()
方法检查文件是否存在:
import os
if os.path.exists('example.txt'):
print('File exists.')
else:
print('File does not exist.')
创建目录
使用os.makedirs()
方法创建目录:
import os
if not os.path.exists('example_dir'):
os.makedirs('example_dir')
print('Directory created.')
else:
print('Directory already exists.')
删除文件
使用os.remove()
方法删除文件:
import os
if os.path.exists('example.txt'):
os.remove('example.txt')
print('File deleted.')
else:
print('File does not exist.')
重命名文件
使用os.rename()
方法重命名文件:
import os
if os.path.exists('example.txt'):
os.rename('example.txt', 'new_example.txt')
print('File renamed.')
else:
print('File does not exist.')
四、pathlib
模块的使用
pathlib
模块提供了面向对象的方法来处理文件和路径。它使代码更具可读性和可维护性,是现代Python编程中的推荐选择。
创建Path对象
创建Path
对象:
from pathlib import Path
file_path = Path('example.txt')
检查文件是否存在
使用Path.exists()
方法检查文件是否存在:
if file_path.exists():
print('File exists.')
else:
print('File does not exist.')
读取文件内容
使用Path.read_text()
方法读取文件内容:
if file_path.exists():
content = file_path.read_text(encoding='utf-8')
print(content)
else:
print('File does not exist.')
写入文件内容
使用Path.write_text()
方法写入文件内容:
file_path.write_text('Hello, World!', encoding='utf-8')
追加文件内容
使用Path.open()
方法以追加模式打开文件,然后写入内容:
with file_path.open('a', encoding='utf-8') as file:
file.write('Hello, again!')
创建目录
使用Path.mkdir()
方法创建目录:
dir_path = Path('example_dir')
if not dir_path.exists():
dir_path.mkdir()
print('Directory created.')
else:
print('Directory already exists.')
删除文件
使用Path.unlink()
方法删除文件:
if file_path.exists():
file_path.unlink()
print('File deleted.')
else:
print('File does not exist.')
重命名文件
使用Path.rename()
方法重命名文件:
new_file_path = Path('new_example.txt')
if file_path.exists():
file_path.rename(new_file_path)
print('File renamed.')
else:
print('File does not exist.')
五、文件处理的高级技巧
在实际项目中,文件处理经常涉及到更多复杂的操作,比如处理大文件、读取特定格式的数据、处理文件异常等。以下是一些高级技巧和最佳实践。
处理大文件
处理大文件时,建议使用逐行读取的方法,以减少内存占用:
with open('large_file.txt', 'r', encoding='utf-8') as file:
for line in file:
process(line) # 处理每一行内容
读取特定格式的数据
对于特定格式的数据文件,可以使用专门的库进行处理。例如,读取CSV文件可以使用csv
模块:
import csv
with open('data.csv', 'r', encoding='utf-8') as file:
reader = csv.reader(file)
for row in reader:
print(row)
处理文件异常
文件操作时,可能会遇到各种异常情况,如文件不存在、权限不足等。可以使用try-except
语句捕获异常并进行处理:
try:
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
except FileNotFoundError:
print('File not found.')
except PermissionError:
print('Permission denied.')
except Exception as e:
print(f'An error occurred: {e}')
使用临时文件
在某些情况下,可能需要使用临时文件来存储中间结果。可以使用tempfile
模块创建临时文件:
import tempfile
with tempfile.NamedTemporaryFile(delete=False) as temp_file:
temp_file.write(b'Hello, World!')
print(f'Temporary file created: {temp_file.name}')
六、文件处理的最佳实践
在进行文件处理时,遵循一些最佳实践可以提高代码的可靠性和可维护性。
1. 使用with
语句
使用with
语句确保文件在操作完成后自动关闭,避免资源泄漏。
2. 处理异常
捕获并处理文件操作中的异常,提供友好的错误提示。
3. 使用绝对路径
尽量使用绝对路径,避免路径错误导致文件找不到。
4. 检查文件是否存在
在打开文件前,检查文件是否存在,避免文件不存在导致的错误。
5. 避免硬编码路径
使用配置文件或环境变量管理文件路径,避免硬编码路径导致的灵活性不足。
6. 使用现代库
尽量使用pathlib
等现代库,提升代码的可读性和可维护性。
通过以上方法和技巧,您可以在Python中更高效地进行文件处理操作。无论是简单的读写操作,还是复杂的文件管理任务,Python都能提供强大而灵活的解决方案。
相关问答FAQs:
如何在Python中打开文件并读取内容?
在Python中,可以使用内置的open()
函数来打开文件。调用这个函数时,需要提供文件名和模式(如只读、写入等)。例如,使用with open('filename.txt', 'r') as file:
可以打开一个文件并确保在处理完成后自动关闭。读取内容可以使用file.read()
、file.readline()
或file.readlines()
等方法。
Python支持哪些文件打开模式?
Python中的open()
函数支持多种文件打开模式。常见的模式包括:
'r'
:只读模式,文件必须存在。'w'
:写入模式,若文件存在则覆盖,不存在则创建。'a'
:追加模式,数据会添加到文件末尾,若文件不存在则创建。'b'
:二进制模式,配合其他模式使用,比如'rb'
或'wb'
。
在打开文件时如何处理异常?
在Python中,处理文件打开时的异常可以使用try...except
语句。这样可以捕捉到文件不存在、权限不足等问题。例如:
try:
with open('filename.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("文件未找到,请检查文件名。")
except IOError:
print("文件打开失败,请检查权限。")
这种方式能有效提升代码的健壮性和用户体验。
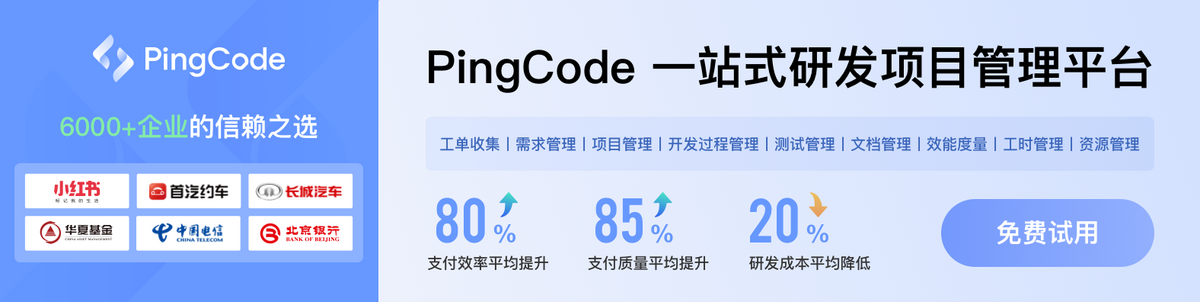