Python调用其它软件程序的方法有多种,包括使用subprocess模块、os.system函数、通过第三方库、与API交互等。这些方法各有优劣,适用于不同的场景。subprocess模块提供了更强大的功能和控制,可以捕获输出、处理错误等,是推荐的方式。下面我们将详细介绍这些方法。
一、SUBPROCESS模块
1、基本使用
subprocess
模块是Python标准库的一部分,提供了更强大的功能来创建和管理子进程。可以使用subprocess.run
来执行外部程序。以下是一个简单的示例:
import subprocess
执行外部命令
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
输出结果
print(result.stdout)
在这个示例中,我们调用了Unix系统的ls -l
命令,并打印出其输出。capture_output=True
选项表示我们希望捕获命令的输出,而text=True
选项表示我们希望以字符串形式处理输出。
2、处理错误
使用subprocess
模块时,可以捕获和处理错误。例如:
import subprocess
try:
result = subprocess.run(['ls', '-l', '/nonexistentpath'], check=True, capture_output=True, text=True)
except subprocess.CalledProcessError as e:
print(f"Error occurred: {e}")
在这个示例中,如果命令执行失败,会引发subprocess.CalledProcessError
异常,我们可以捕获并处理该异常。
3、与标准输入输出交互
有时需要与外部程序进行交互,可以通过subprocess.Popen
实现:
import subprocess
process = subprocess.Popen(['python3', '-i'], stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
向子进程发送命令
stdout, stderr = process.communicate('print("Hello from subprocess")\n')
输出结果
print(stdout)
在这个示例中,我们启动了一个交互式Python解释器,并向其发送了一条命令。
二、OS.SYSTEM函数
1、基本使用
os.system
是调用外部程序的最简单方式,但它的功能有限,无法捕获输出。例如:
import os
执行外部命令
os.system('ls -l')
2、与SUBPROCESS的对比
与subprocess
相比,os.system
的主要缺点是不能捕获输出,也无法处理错误。因此,建议在需要更多控制和功能时使用subprocess
。
三、第三方库
1、SH库
sh
是一个第三方库,提供了一种更简单、更Pythonic的方式来调用外部程序。可以通过pip install sh
进行安装。以下是一个示例:
import sh
执行外部命令
output = sh.ls('-l')
输出结果
print(output)
2、PEXPECT库
pexpect
是另一个有用的库,特别适用于需要与交互式命令行工具进行交互的场景。可以通过pip install pexpect
进行安装。以下是一个示例:
import pexpect
启动交互式命令行工具
child = pexpect.spawn('python3 -i')
发送命令
child.sendline('print("Hello from pexpect")')
捕获输出
child.expect('>>>')
print(child.before.decode())
四、通过API交互
1、调用REST API
许多软件提供REST API,Python可以通过requests
库与这些API进行交互。以下是一个示例:
import requests
发送GET请求
response = requests.get('https://api.github.com/repos/python/cpython')
输出结果
print(response.json())
2、使用SDK
某些软件提供官方SDK,Python可以通过这些SDK调用软件功能。例如,AWS提供了boto3
库。以下是一个示例:
import boto3
创建S3客户端
s3 = boto3.client('s3')
列出存储桶
response = s3.list_buckets()
输出结果
for bucket in response['Buckets']:
print(bucket['Name'])
五、总结
Python提供了多种调用其它软件程序的方法。subprocess模块功能强大,推荐用于需要更多控制和功能的场景;os.system函数简单易用,但功能有限;第三方库如sh
和pexpect
提供了更简洁的接口,适用于特定场景;通过API交互可以调用许多现代软件的功能。根据具体需求选择合适的方法,将极大提高开发效率。
六、具体示例与应用
1、自动化运维脚本
在自动化运维中,常常需要调用各种命令行工具。以下是一个示例脚本,使用subprocess
模块管理服务器:
import subprocess
def execute_command(command):
result = subprocess.run(command, capture_output=True, text=True)
if result.returncode == 0:
print(f"Command succeeded: {result.stdout}")
else:
print(f"Command failed: {result.stderr}")
示例命令
commands = [
['systemctl', 'restart', 'nginx'],
['df', '-h'],
['uptime']
]
for command in commands:
execute_command(command)
2、数据处理与分析
在数据处理与分析中,可能需要调用各种数据处理工具。以下是一个使用subprocess
模块调用ffmpeg
工具处理视频文件的示例:
import subprocess
def process_video(input_file, output_file):
command = ['ffmpeg', '-i', input_file, '-vf', 'scale=640:480', output_file]
result = subprocess.run(command, capture_output=True, text=True)
if result.returncode == 0:
print(f"Video processed successfully: {result.stdout}")
else:
print(f"Video processing failed: {result.stderr}")
示例调用
process_video('input.mp4', 'output.mp4')
3、与Web服务交互
在与Web服务交互时,可能需要调用外部程序或API。以下是一个使用requests
库调用REST API获取天气信息的示例:
import requests
def get_weather(city):
api_key = 'your_api_key'
url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(f"Weather in {city}: {data['weather'][0]['description']}")
else:
print(f"Failed to get weather data: {response.status_code}")
示例调用
get_weather('London')
4、测试与调试
在测试与调试中,可能需要调用外部测试工具或调试器。以下是一个使用subprocess
模块调用pytest
运行测试用例的示例:
import subprocess
def run_tests():
command = ['pytest', 'tests/']
result = subprocess.run(command, capture_output=True, text=True)
if result.returncode == 0:
print(f"Tests passed: {result.stdout}")
else:
print(f"Tests failed: {result.stderr}")
示例调用
run_tests()
5、图形界面应用
在图形界面应用中,可能需要调用外部程序处理图像或生成图表。以下是一个使用subprocess
模块调用ImageMagick
处理图像的示例:
import subprocess
def resize_image(input_file, output_file, width, height):
command = ['convert', input_file, '-resize', f'{width}x{height}', output_file]
result = subprocess.run(command, capture_output=True, text=True)
if result.returncode == 0:
print(f"Image resized successfully: {result.stdout}")
else:
print(f"Image resizing failed: {result.stderr}")
示例调用
resize_image('input.jpg', 'output.jpg', 640, 480)
6、数据库操作
在数据库操作中,可能需要调用数据库管理工具。以下是一个使用subprocess
模块调用psql
管理PostgreSQL数据库的示例:
import subprocess
def execute_sql(database, sql):
command = ['psql', database, '-c', sql]
result = subprocess.run(command, capture_output=True, text=True)
if result.returncode == 0:
print(f"SQL executed successfully: {result.stdout}")
else:
print(f"SQL execution failed: {result.stderr}")
示例调用
execute_sql('mydatabase', 'SELECT * FROM mytable;')
通过这些示例,可以看到Python调用其它软件程序在不同场景中的应用。选择合适的方法和工具,可以大大提高开发效率和程序的可维护性。
相关问答FAQs:
如何使用Python调用本地安装的应用程序?
您可以使用Python的subprocess
模块来调用本地安装的应用程序。通过subprocess.run()
或subprocess.Popen()
函数,您可以执行外部程序并与其交互。例如,若要打开记事本,可以使用以下代码:
import subprocess
subprocess.run(["notepad.exe"])
这种方法不仅可以启动应用程序,还可以传递参数和获取返回值。
Python可以与哪些类型的软件进行交互?
Python能够与多种类型的软件进行交互,包括但不限于命令行工具、图形用户界面应用程序、数据库管理系统和Web应用程序。通过适当的库和模块,如pyodbc
用于数据库,requests
用于Web API调用,您可以轻松实现与这些软件的协作。
如何处理调用外部程序时的错误和异常?
在调用外部程序时,可能会遇到各种错误,例如程序未找到或运行时错误。使用try-except
语句可以有效捕获这些异常,并采取相应的措施。例如:
import subprocess
try:
subprocess.run(["notepad.exe"], check=True)
except subprocess.CalledProcessError as e:
print(f"程序调用失败: {e}")
except FileNotFoundError:
print("指定的程序未找到,请检查路径。")
这种方式确保了您的代码在遇到问题时不会崩溃,并能提供用户友好的错误信息。
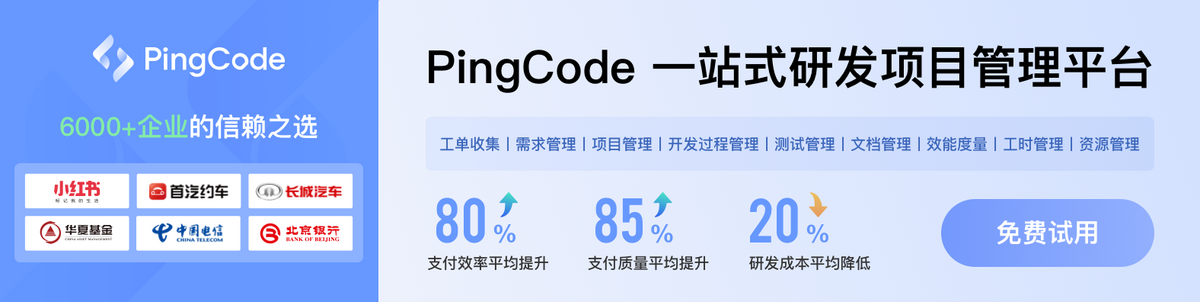