在Python中,函数可以通过使用内置的open()
函数、with
语句和文件对象的方法来访问文件、读取文件内容或写入文件。通过调用open()
函数打开文件,可以选择不同的模式进行文件操作,比如读模式('r')、写模式('w')、追加模式('a')以及二进制模式('b')。使用with
语句可以确保文件在操作完成后自动关闭,避免资源泄露。
使用open()
函数打开文件、使用with
语句来管理文件上下文、使用文件对象的方法进行读取或写入。
下面将详细描述如何在Python中使用函数来访问文件。
一、使用 open()
函数打开文件
1.1 打开文件进行读取
在Python中,可以使用open()
函数打开一个文件,并指定文件路径和模式。读取文件时,常用模式为'r'。
def read_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
在上述代码中,with open(file_path, 'r') as file:
语句打开文件并返回文件对象file
。file.read()
方法读取文件内容,并将其存储在变量content
中。with
语句确保在读取操作完成后,文件自动关闭。
1.2 打开文件进行写入
写入文件时,可以使用模式'w',它会覆盖文件内容。如果希望追加内容,可以使用模式'a'。
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
在上述代码中,with open(file_path, 'w') as file:
语句打开文件并返回文件对象file
。file.write(content)
方法将变量content
的内容写入文件。with
语句确保在写入操作完成后,文件自动关闭。
二、读取文件内容
2.1 逐行读取文件内容
在某些情况下,逐行读取文件内容会更加方便和高效。
def read_file_by_line(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
return lines
在上述代码中,file.readlines()
方法读取文件的所有行,并返回一个包含每行内容的列表。通过这种方式,可以方便地逐行处理文件内容。
2.2 使用迭代器逐行读取文件内容
另一种逐行读取文件内容的方法是使用文件对象的迭代器。
def read_file_line_by_line(file_path):
with open(file_path, 'r') as file:
for line in file:
process_line(line)
在上述代码中,for line in file:
语句逐行迭代文件对象file
,并将每行内容存储在变量line
中。通过这种方式,可以逐行处理文件内容,而无需将所有内容一次性加载到内存中。
三、写入文件内容
3.1 覆盖文件内容
使用模式'w'打开文件,会覆盖文件的原有内容。
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
在上述代码中,file.write(content)
方法将变量content
的内容写入文件。使用模式'w'会覆盖文件的原有内容。
3.2 追加文件内容
使用模式'a'打开文件,可以在文件末尾追加内容,而不覆盖原有内容。
def append_file(file_path, content):
with open(file_path, 'a') as file:
file.write(content)
在上述代码中,file.write(content)
方法将变量content
的内容追加到文件末尾。使用模式'a'不会覆盖文件的原有内容。
四、使用二进制模式访问文件
在某些情况下,需要以二进制模式读取或写入文件。可以通过在模式字符串后添加'b'来指定二进制模式。
4.1 读取二进制文件
def read_binary_file(file_path):
with open(file_path, 'rb') as file:
content = file.read()
return content
在上述代码中,with open(file_path, 'rb') as file:
语句以二进制模式打开文件,并返回文件对象file
。file.read()
方法读取文件内容,并将其存储在变量content
中。
4.2 写入二进制文件
def write_binary_file(file_path, content):
with open(file_path, 'wb') as file:
file.write(content)
在上述代码中,with open(file_path, 'wb') as file:
语句以二进制模式打开文件,并返回文件对象file
。file.write(content)
方法将变量content
的内容写入文件。
五、文件操作中的错误处理
在进行文件操作时,可能会遇到各种错误,例如文件不存在、权限不足等。可以使用try-except
语句进行错误处理。
5.1 捕获文件操作错误
def read_file_with_error_handling(file_path):
try:
with open(file_path, 'r') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"Error: The file '{file_path}' was not found.")
except PermissionError:
print(f"Error: Permission denied to read the file '{file_path}'.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
在上述代码中,try
块中的代码尝试打开并读取文件。如果发生FileNotFoundError
或PermissionError
,相应的except
块将捕获并处理错误。except Exception as e:
语句捕获所有其他类型的异常,并打印错误信息。
5.2 捕获写入文件时的错误
def write_file_with_error_handling(file_path, content):
try:
with open(file_path, 'w') as file:
file.write(content)
except PermissionError:
print(f"Error: Permission denied to write to the file '{file_path}'.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
在上述代码中,try
块中的代码尝试打开并写入文件。如果发生PermissionError
,相应的except
块将捕获并处理错误。except Exception as e:
语句捕获所有其他类型的异常,并打印错误信息。
六、使用 os
和 shutil
模块进行文件操作
除了基本的文件读取和写入操作外,Python的os
和shutil
模块提供了更多高级的文件操作功能。
6.1 使用 os
模块操作文件和目录
os
模块提供了多种文件和目录操作函数,例如创建目录、删除文件、重命名文件等。
import os
创建目录
def create_directory(directory_path):
try:
os.makedirs(directory_path)
except OSError as e:
print(f"Error: {e}")
删除文件
def delete_file(file_path):
try:
os.remove(file_path)
except FileNotFoundError:
print(f"Error: The file '{file_path}' does not exist.")
except PermissionError:
print(f"Error: Permission denied to delete the file '{file_path}'.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
重命名文件
def rename_file(old_file_path, new_file_path):
try:
os.rename(old_file_path, new_file_path)
except FileNotFoundError:
print(f"Error: The file '{old_file_path}' does not exist.")
except PermissionError:
print(f"Error: Permission denied to rename the file '{old_file_path}'.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
在上述代码中,分别定义了创建目录、删除文件和重命名文件的函数,并在操作过程中进行错误处理。
6.2 使用 shutil
模块复制和移动文件
shutil
模块提供了复制和移动文件的功能。
import shutil
复制文件
def copy_file(src_file_path, dst_file_path):
try:
shutil.copy(src_file_path, dst_file_path)
except FileNotFoundError:
print(f"Error: The file '{src_file_path}' does not exist.")
except PermissionError:
print(f"Error: Permission denied to copy the file '{src_file_path}'.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
移动文件
def move_file(src_file_path, dst_file_path):
try:
shutil.move(src_file_path, dst_file_path)
except FileNotFoundError:
print(f"Error: The file '{src_file_path}' does not exist.")
except PermissionError:
print(f"Error: Permission denied to move the file '{src_file_path}'.")
except Exception as e:
print(f"An unexpected error occurred: {e}")
在上述代码中,分别定义了复制文件和移动文件的函数,并在操作过程中进行错误处理。
七、总结
在Python中,函数可以通过使用open()
函数、with
语句和文件对象的方法来访问文件、读取文件内容或写入文件。通过调用open()
函数打开文件,可以选择不同的模式进行文件操作,比如读模式('r')、写模式('w')、追加模式('a')以及二进制模式('b')。使用with
语句可以确保文件在操作完成后自动关闭,避免资源泄露。
此外,可以使用try-except
语句进行错误处理,捕获文件操作过程中可能出现的各种错误。os
和shutil
模块提供了更多高级的文件操作功能,例如创建目录、删除文件、重命名文件、复制文件和移动文件。
通过掌握这些文件操作方法,可以在Python中编写更加健壮和灵活的文件处理代码。
相关问答FAQs:
在Python中,如何打开和读取文件?
在Python中,打开和读取文件通常使用内置的open()
函数。您可以通过指定文件名和模式(如'r'
表示读取,'w'
表示写入等)来打开文件。例如,使用with open('file.txt', 'r') as file:
可以安全地打开文件并在代码块结束后自动关闭它。读取内容可以使用file.read()
、file.readline()
或file.readlines()
等方法。
如何在Python函数中处理文件异常?
在处理文件时,可能会遇到各种异常情况,例如文件不存在或权限不足。为了确保代码的健壮性,可以使用try
和except
语句来捕获这些异常。例如,您可以编写如下代码:
try:
with open('file.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("文件未找到,请检查路径。")
except PermissionError:
print("权限错误,请检查文件权限。")
这种方式可以有效地处理文件操作中的常见错误。
是否可以在函数中同时读取和写入文件?
当然可以!在Python中,您可以使用open()
函数的不同模式来同时读取和写入文件。例如,使用模式'r+'
可以实现读取和写入文件。示例代码如下:
with open('file.txt', 'r+') as file:
content = file.read()
file.write("新内容")
请注意,在使用此模式时,文件的指针位置可能会影响读取和写入的结果,因此在进行写入之前,确保您知道当前文件指针的位置。
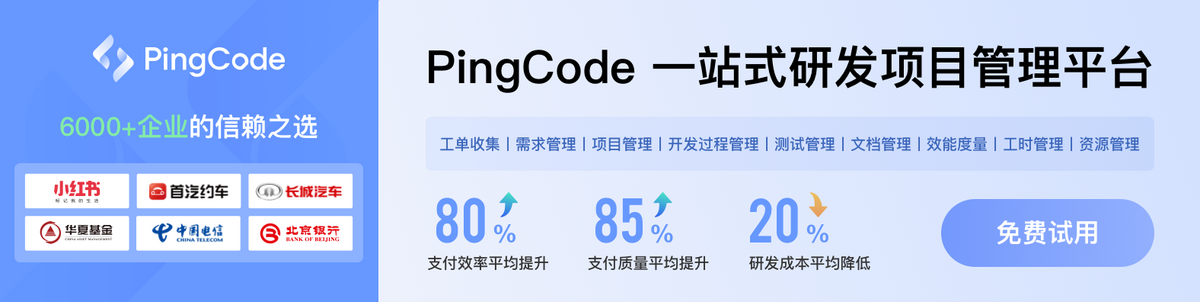