如何用Python跑网络脚本:使用requests库、使用BeautifulSoup库、处理HTTP请求、解析HTML内容
在Python中运行网络脚本主要涉及到如何处理HTTP请求和解析网络数据。使用requests库是其中最常见和简便的方法之一。requests库是一个强大而易用的HTTP库,它让你能够轻松地发送HTTP请求并获取响应。下面,我们详细展开这一点。
使用requests库
requests库是一个非常流行的Python库,专门用于发送HTTP请求。它可以处理GET、POST、PUT、DELETE等各种HTTP请求。以下是一个基本的使用示例:
import requests
发送GET请求
response = requests.get('https://api.example.com/data')
检查响应状态码
if response.status_code == 200:
# 获取响应内容
data = response.json()
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们首先导入了requests库,然后使用requests.get()
方法发送一个GET请求到指定的URL。接着,我们检查响应的状态码,如果状态码是200(表示请求成功),我们就通过response.json()
方法获取响应内容并打印出来。
接下来,我们将详细探讨如何用Python跑网络脚本的其他方面。
一、使用requests库
1. 发送GET请求
GET请求是最常用的HTTP请求之一,用于从服务器获取数据。使用requests库发送GET请求非常简单,只需调用requests.get()
方法并传入目标URL即可。
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们首先定义了目标URL,然后使用requests.get()
方法发送GET请求。接着,我们检查响应的状态码,如果请求成功,就通过response.json()
方法获取响应数据并打印出来。
2. 发送POST请求
POST请求用于向服务器提交数据。使用requests库发送POST请求同样很简单,只需调用requests.post()
方法并传入目标URL和要提交的数据即可。
import requests
url = 'https://api.example.com/data'
payload = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, data=payload)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们首先定义了目标URL和要提交的数据,然后使用requests.post()
方法发送POST请求。接着,我们检查响应的状态码,如果请求成功,就通过response.json()
方法获取响应数据并打印出来。
3. 处理HTTP头部
在发送HTTP请求时,有时需要自定义HTTP头部。requests库允许我们通过headers
参数来指定HTTP头部。
import requests
url = 'https://api.example.com/data'
headers = {'User-Agent': 'my-app/0.0.1'}
response = requests.get(url, headers=headers)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们首先定义了目标URL和自定义的HTTP头部,然后使用requests.get()
方法发送GET请求,并通过headers
参数传入自定义的HTTP头部。接着,我们检查响应的状态码,如果请求成功,就通过response.json()
方法获取响应数据并打印出来。
4. 处理Cookies
有些Web应用程序需要处理Cookies。requests库允许我们通过cookies
参数来指定请求的Cookies,并通过response.cookies
属性来获取响应的Cookies。
import requests
url = 'https://api.example.com/data'
cookies = {'session_id': '123456'}
response = requests.get(url, cookies=cookies)
if response.status_code == 200:
data = response.json()
print(data)
print(response.cookies)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们首先定义了目标URL和请求的Cookies,然后使用requests.get()
方法发送GET请求,并通过cookies
参数传入请求的Cookies。接着,我们检查响应的状态码,如果请求成功,就通过response.json()
方法获取响应数据并打印出来,并输出响应的Cookies。
5. 处理HTTPS
requests库默认支持HTTPS请求,但有时我们可能需要忽略SSL证书验证。可以通过verify
参数来实现。
import requests
url = 'https://api.example.com/data'
response = requests.get(url, verify=False)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们通过verify=False
参数来忽略SSL证书验证。
二、使用BeautifulSoup库
1. 安装BeautifulSoup
BeautifulSoup是一个用于解析HTML和XML文档的Python库。要使用BeautifulSoup,首先需要安装它。
pip install beautifulsoup4
2. 解析HTML内容
BeautifulSoup库可以轻松地解析HTML内容,并提取所需的数据。
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
print(soup.prettify())
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们首先发送GET请求获取网页内容,然后使用BeautifulSoup解析HTML内容,并通过prettify()
方法打印格式化后的HTML内容。
3. 查找元素
BeautifulSoup提供了多种方法来查找HTML元素,例如find()
和find_all()
方法。
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
title = soup.find('title').text
print(f"页面标题:{title}")
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们使用find()
方法查找HTML文档中的<title>
标签,并输出其文本内容。
4. 处理表格数据
BeautifulSoup可以轻松地提取HTML表格中的数据。
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com/table'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
table = soup.find('table')
rows = table.find_all('tr')
for row in rows:
cells = row.find_all('td')
for cell in cells:
print(cell.text)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们首先查找HTML文档中的<table>
标签,然后遍历表格中的每一行和每一个单元格,输出单元格中的文本内容。
三、处理HTTP请求
1. 重定向
requests库会自动处理HTTP重定向,但有时我们需要控制重定向行为。可以通过allow_redirects
参数来控制是否允许重定向。
import requests
url = 'http://example.com/redirect'
response = requests.get(url, allow_redirects=False)
if response.status_code in [301, 302]:
print(f"重定向到:{response.headers['Location']}")
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们通过allow_redirects=False
参数来禁止自动重定向,并检查响应的状态码,如果是301或302(表示重定向),我们输出重定向的目标URL。
2. 处理超时
在发送HTTP请求时,有时需要设置超时时间,以避免请求长时间阻塞。可以通过timeout
参数来设置请求的超时时间。
import requests
url = 'https://api.example.com/data'
try:
response = requests.get(url, timeout=5)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
except requests.exceptions.Timeout:
print("请求超时")
在这个示例中,我们通过timeout=5
参数来设置请求的超时时间为5秒,并使用try-except块来捕获请求超时的异常。
3. 处理代理
有时需要通过代理服务器发送HTTP请求。可以通过proxies
参数来指定代理服务器。
import requests
url = 'https://api.example.com/data'
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'https://10.10.1.10:1080',
}
response = requests.get(url, proxies=proxies)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们通过proxies
参数来指定HTTP和HTTPS代理服务器,并发送GET请求。
四、解析HTML内容
1. 使用lxml库
除了BeautifulSoup,lxml也是一个强大的HTML和XML解析库。要使用lxml,首先需要安装它。
pip install lxml
2. 解析HTML内容
lxml库可以高效地解析HTML内容,并提取所需的数据。
import requests
from lxml import html
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
tree = html.fromstring(response.content)
title = tree.xpath('//title/text()')[0]
print(f"页面标题:{title}")
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们首先发送GET请求获取网页内容,然后使用lxml解析HTML内容,并通过XPath提取页面标题。
3. 查找元素
lxml提供了强大的XPath支持,可以轻松地查找HTML元素。
import requests
from lxml import html
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
tree = html.fromstring(response.content)
links = tree.xpath('//a/@href')
for link in links:
print(link)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们使用XPath查找HTML文档中的所有链接,并输出每一个链接的URL。
4. 处理表格数据
lxml可以高效地提取HTML表格中的数据。
import requests
from lxml import html
url = 'https://www.example.com/table'
response = requests.get(url)
if response.status_code == 200:
tree = html.fromstring(response.content)
rows = tree.xpath('//table//tr')
for row in rows:
cells = row.xpath('.//td/text()')
for cell in cells:
print(cell)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们首先使用XPath查找HTML文档中的所有表格行,然后遍历每一行和每一个单元格,输出单元格中的文本内容。
五、处理JSON数据
1. 解析JSON响应
requests库可以自动解析JSON响应,并将其转换为Python字典或列表。
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们通过response.json()
方法解析JSON响应,并输出解析后的数据。
2. 处理复杂JSON数据
有时,JSON响应可能包含嵌套的数据结构。可以使用标准的Python字典和列表操作来处理复杂的JSON数据。
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
for item in data['items']:
print(f"名称:{item['name']}, 值:{item['value']}")
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们首先解析JSON响应,然后遍历JSON数据中的每一个项目,并输出项目的名称和值。
六、处理XML数据
1. 解析XML响应
requests库可以获取XML响应,但需要使用第三方库来解析XML数据,例如lxml。
import requests
from lxml import etree
url = 'https://api.example.com/data.xml'
response = requests.get(url)
if response.status_code == 200:
tree = etree.fromstring(response.content)
print(etree.tostring(tree, pretty_print=True).decode())
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们首先发送GET请求获取XML响应,然后使用lxml解析XML数据,并格式化输出。
2. 查找元素
lxml提供了强大的XPath支持,可以轻松地查找XML元素。
import requests
from lxml import etree
url = 'https://api.example.com/data.xml'
response = requests.get(url)
if response.status_code == 200:
tree = etree.fromstring(response.content)
items = tree.xpath('//item')
for item in items:
name = item.find('name').text
value = item.find('value').text
print(f"名称:{name}, 值:{value}")
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们使用XPath查找XML文档中的所有<item>
元素,并输出每一个元素的名称和值。
七、处理文件下载
1. 下载文件
requests库可以轻松地下载文件,并保存到本地。
import requests
url = 'https://www.example.com/file.zip'
response = requests.get(url)
if response.status_code == 200:
with open('file.zip', 'wb') as f:
f.write(response.content)
print("文件下载成功")
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们发送GET请求下载文件,并将文件内容保存到本地。
2. 大文件下载
对于大文件下载,可以使用流式下载,以避免占用过多内存。
import requests
url = 'https://www.example.com/largefile.zip'
response = requests.get(url, stream=True)
if response.status_code == 200:
with open('largefile.zip', 'wb') as f:
for chunk in response.iter_content(chunk_size=8192):
f.write(chunk)
print("大文件下载成功")
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们通过stream=True
参数启用流式下载,并逐块读取文件内容,写入本地文件。
八、处理API认证
1. 基本认证
requests库支持HTTP基本认证,可以通过auth
参数来指定用户名和密码。
import requests
from requests.auth import HTTPBasicAuth
url = 'https://api.example.com/secure-data'
response = requests.get(url, auth=HTTPBasicAuth('username', 'password'))
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们通过auth
参数指定HTTP基本认证的用户名和密码,并发送GET请求。
2. Bearer Token认证
对于Bearer Token认证,可以通过自定义HTTP头部来实现。
import requests
url = 'https://api.example.com/secure-data'
headers = {'Authorization': 'Bearer your_token'}
response = requests.get(url, headers=headers)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
在这个示例中,我们通过headers
参数指定包含Bearer Token的HTTP头部,并发送GET请求。
3. OAuth认证
requests库可以与第三方OAuth库集成,以实现OAuth认证。
import requests
from requests_oauthlib import OAuth1
url = 'https://api.example.com/secure-data'
auth = OAuth1('your_client_key', 'your_client_secret', 'your_resource_owner_key', '
相关问答FAQs:
如何在Python中执行网络脚本的基本步骤是什么?
要在Python中运行网络脚本,首先需要安装Python环境,并确保相关的库(如requests
、beautifulsoup4
或selenium
等)已被正确安装。可以使用pip install requests beautifulsoup4 selenium
等命令进行安装。编写完脚本后,在命令行中输入python script_name.py
即可运行该脚本,确保在运行前已连接到网络。
使用Python进行网络爬虫需要注意哪些法律和道德问题?
在使用Python进行网络爬虫时,务必要遵守网站的robots.txt
文件中的规则,确保不违反网站的使用条款。同时,尊重网站的服务器负载,避免发送过多请求导致网站崩溃或被封禁。建议在爬取数据时采取适当的延时,并只获取必要的信息。
如何调试Python网络脚本以解决潜在的问题?
调试Python网络脚本时,可以使用print
语句输出关键变量的值,帮助了解程序的运行状态。利用Python内置的pdb
调试器也可以逐行执行代码,查看每一步的执行情况。此外,查看错误日志和使用异常处理机制(如try-except
)能有效捕捉并处理运行时错误,使调试过程更加高效。
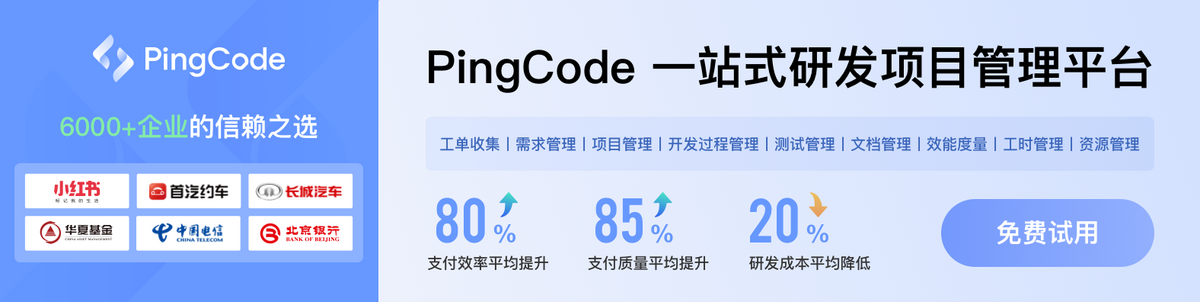