Java调用Python进行人像识别可以通过多种方式实现,主要包括使用Jython、通过ProcessBuilder执行Python脚本、使用Jep库、以及通过RESTful API进行通信。其中,通过ProcessBuilder执行Python脚本是一种较为简单且常用的方式,下面将详细介绍这种方式并涵盖其他几种方法的简要说明。
一、使用ProcessBuilder执行Python脚本
通过Java的ProcessBuilder
类可以执行外部的Python脚本,从而实现调用Python进行人像识别。以下是具体步骤:
1. 准备Python人像识别脚本
假设你已经有一个Python脚本face_recognition.py
,该脚本使用OpenCV和dlib库进行人像识别。脚本内容如下:
import cv2
import dlib
import sys
def detect_faces(image_path):
detector = dlib.get_frontal_face_detector()
img = cv2.imread(image_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
faces = detector(gray)
return len(faces)
if __name__ == "__main__":
image_path = sys.argv[1]
face_count = detect_faces(image_path)
print(f"Number of faces detected: {face_count}")
2. Java代码调用Python脚本
在Java代码中,通过ProcessBuilder
执行上述Python脚本并获取结果:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class FaceRecognition {
public static void main(String[] args) {
String pythonScriptPath = "path/to/face_recognition.py";
String imagePath = "path/to/image.jpg";
try {
ProcessBuilder pb = new ProcessBuilder("python", pythonScriptPath, imagePath);
Process process = pb.start();
BufferedReader in = new BufferedReader(new InputStreamReader(process.getInputStream()));
String result = in.readLine();
System.out.println(result);
} catch (IOException e) {
e.printStackTrace();
}
}
}
二、使用Jython
Jython是一个Python实现的Java平台,可以直接在Java中嵌入Python代码。然而,Jython只支持Python 2.x,并且不支持许多C扩展库(如dlib和部分OpenCV功能),因此使用范围有限。
示例代码
import org.python.util.PythonInterpreter;
public class FaceRecognition {
public static void main(String[] args) {
PythonInterpreter interpreter = new PythonInterpreter();
interpreter.exec("import sys\nsys.path.append('path/to/your/python/scripts')");
interpreter.exec("import face_recognition");
interpreter.exec("print(face_recognition.detect_faces('path/to/image.jpg'))");
}
}
三、使用Jep库
Jep(Java Embedded Python)允许在Java代码中嵌入Python解释器,并且支持调用Python的C扩展库。Jep的性能优于Jython,并且兼容Python 3.x。
安装Jep
首先,需要安装Jep库,可以通过以下命令进行安装:
pip install jep
示例代码
import jep.Jep;
import jep.JepException;
public class FaceRecognition {
public static void main(String[] args) {
try (Jep jep = new Jep()) {
jep.runScript("path/to/face_recognition.py");
int faceCount = (int) jep.invoke("detect_faces", "path/to/image.jpg");
System.out.println("Number of faces detected: " + faceCount);
} catch (JepException e) {
e.printStackTrace();
}
}
}
四、通过RESTful API进行通信
另一种常见的方法是将Python人像识别功能封装成一个RESTful API服务,通过HTTP请求进行调用。这种方式具有语言无关性和较好的扩展性。
1. 创建Python RESTful API服务
可以使用Flask框架创建一个简单的RESTful API服务:
from flask import Flask, request, jsonify
import cv2
import dlib
app = Flask(__name__)
def detect_faces(image_path):
detector = dlib.get_frontal_face_detector()
img = cv2.imread(image_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
faces = detector(gray)
return len(faces)
@app.route('/detect_faces', methods=['POST'])
def detect_faces_api():
image_path = request.json['image_path']
face_count = detect_faces(image_path)
return jsonify({'face_count': face_count})
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
2. Java代码调用RESTful API
在Java代码中,通过HTTP客户端发送请求并获取结果:
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.charset.StandardCharsets;
public class FaceRecognition {
public static void main(String[] args) {
String apiUrl = "http://localhost:5000/detect_faces";
String imagePath = "path/to/image.jpg";
String jsonInputString = "{\"image_path\": \"" + imagePath + "\"}";
try {
URL url = new URL(apiUrl);
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/json; utf-8");
con.setRequestProperty("Accept", "application/json");
con.setDoOutput(true);
try (OutputStream os = con.getOutputStream()) {
byte[] input = jsonInputString.getBytes(StandardCharsets.UTF_8);
os.write(input, 0, input.length);
}
try (BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(), StandardCharsets.UTF_8))) {
StringBuilder response = new StringBuilder();
String responseLine;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
System.out.println(response.toString());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
总结
Java调用Python进行人像识别有多种实现方式,通过ProcessBuilder执行Python脚本是一种简单且常用的方法。Jython和Jep库可以直接在Java中嵌入Python代码,但Jython存在兼容性问题,而Jep性能更优。通过RESTful API进行通信则具有语言无关性和较好的扩展性,适用于分布式系统。
在实际项目中,选择哪种方式取决于具体的需求、开发环境以及团队的技术栈。无论选择哪种方式,都需要确保Python脚本或服务的稳定性和性能,以保证人像识别功能的可靠性。
相关问答FAQs:
如何在Java中与Python进行交互以实现人像识别?
在Java中调用Python脚本可以通过多种方法实现,例如使用ProcessBuilder来执行Python脚本。您可以编写一个Python脚本来处理人像识别,然后在Java中调用这个脚本,将输入图像路径传递给它,并接收输出结果。同时,使用像Jython这样的库也可以在Java中直接运行Python代码。
使用哪些Python库可以进行人像识别?
常用的Python库包括OpenCV和Dlib,这些库提供了强大的图像处理和人脸识别功能。OpenCV支持多种图像处理操作,而Dlib则以其高效的面部识别算法而闻名。此外,TensorFlow和Keras也可用于构建和训练深度学习模型,从而实现更复杂的人像识别任务。
在Java调用Python脚本时,如何处理数据传递和结果获取?
数据传递可以通过命令行参数或标准输入来实现。Java代码可以使用ProcessBuilder创建进程,传递所需的图像路径等参数。在Python脚本中,您可以通过sys.argv获取这些参数。结果获取可以通过标准输出实现,Java可以读取Python脚本的输出,解析结果并在应用程序中使用。
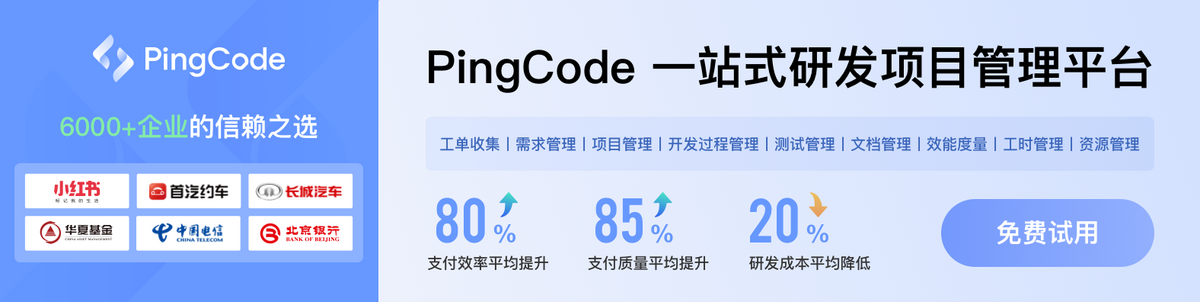