在Python中,发送内容给别人可以通过多种方式来实现,包括电子邮件、即时消息、文件传输等。电子邮件、即时消息、文件传输是常见的几种方式。下面详细描述一下通过电子邮件发送内容的实现方法。
通过电子邮件发送内容是一种常见且有效的方式。Python提供了强大的库smtplib
来处理SMTP(Simple Mail Transfer Protocol)任务,email
库则帮助我们构建邮件内容。以下是一个简单的例子,通过Gmail SMTP服务器发送一封电子邮件:
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
def send_email(subject, body, to_email):
from_email = "your-email@gmail.com"
from_password = "your-email-password"
# Create the container (outer) email message.
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
# Attach the body with the msg instance
msg.attach(MIMEText(body, 'plain'))
# Create server object with SSL option
server = smtplib.SMTP_SSL('smtp.gmail.com', 465)
server.login(from_email, from_password)
server.sendmail(from_email, to_email, msg.as_string())
server.quit()
Usage
send_email("Test Subject", "This is a test email body", "recipient-email@example.com")
在这个例子中,我们使用MIMEMultipart
来创建一个多部分的邮件对象,并使用MIMEText
来附加邮件正文。然后我们通过Gmail的SMTP服务器发送邮件。
一、电子邮件
使用smtplib和email库发送电子邮件
电子邮件是一种非常常见的通信手段,Python内置的smtplib
库和email
库可以很方便地实现邮件发送功能。以下是一个详细的步骤:
-
导入必要的库:
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
-
设置发送者和接收者的信息:
from_email = "your-email@gmail.com"
from_password = "your-email-password"
to_email = "recipient-email@example.com"
-
创建邮件对象:
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = "Test Subject"
-
添加邮件正文:
body = "This is a test email body"
msg.attach(MIMEText(body, 'plain'))
-
发送邮件:
server = smtplib.SMTP_SSL('smtp.gmail.com', 465)
server.login(from_email, from_password)
server.sendmail(from_email, to_email, msg.as_string())
server.quit()
使用第三方库发送邮件
除了标准库,Python还有一些第三方库如yagmail
和emails
,它们提供了更简化和强大的邮件发送功能。
使用yagmail
yagmail
是一个简化邮件发送过程的库,它封装了常用的操作,使用起来非常方便。以下是一个示例:
import yagmail
def send_email_via_yagmail(subject, body, to_email):
yag = yagmail.SMTP("your-email@gmail.com", "your-email-password")
yag.send(to=to_email, subject=subject, contents=body)
Usage
send_email_via_yagmail("Test Subject", "This is a test email body", "recipient-email@example.com")
通过yagmail
,我们可以大大简化邮件发送的代码,使其更加直观和易于维护。
二、即时消息
使用API发送即时消息
即时消息(IM)是一种快速、实时的通信方式。许多即时消息平台提供API接口,允许开发者通过程序发送消息。以下是使用Slack API发送消息的示例:
-
安装slack_sdk库:
pip install slack_sdk
-
使用Slack API发送消息:
from slack_sdk import WebClient
from slack_sdk.errors import SlackApiError
def send_slack_message(token, channel, text):
client = WebClient(token=token)
try:
response = client.chat_postMessage(channel=channel, text=text)
assert response["message"]["text"] == text
except SlackApiError as e:
print(f"Error sending message: {e.response['error']}")
Usage
send_slack_message("your-slack-bot-token", "#general", "This is a test message")
使用第三方库发送即时消息
除了使用官方API,第三方库如python-telegram-bot
也提供了方便的接口来发送即时消息。例如,使用Telegram Bot API发送消息:
-
安装python-telegram-bot库:
pip install python-telegram-bot
-
使用Telegram Bot API发送消息:
from telegram import Bot
def send_telegram_message(token, chat_id, text):
bot = Bot(token=token)
bot.send_message(chat_id=chat_id, text=text)
Usage
send_telegram_message("your-telegram-bot-token", "your-chat-id", "This is a test message")
三、文件传输
使用FTP协议传输文件
FTP(File Transfer Protocol)是一种常见的文件传输协议,Python的ftplib
库可以用于实现FTP文件传输。以下是一个示例:
-
导入ftplib库:
from ftplib import FTP
-
连接FTP服务器并上传文件:
def upload_file(ftp_server, ftp_user, ftp_password, file_path, remote_path):
ftp = FTP(ftp_server)
ftp.login(user=ftp_user, passwd=ftp_password)
with open(file_path, 'rb') as file:
ftp.storbinary(f'STOR {remote_path}', file)
ftp.quit()
Usage
upload_file("ftp.example.com", "ftp_user", "ftp_password", "local_file.txt", "remote_file.txt")
使用SFTP协议传输文件
SFTP(SSH File Transfer Protocol)是一种安全的文件传输协议,paramiko
库可以用于实现SFTP文件传输。以下是一个示例:
-
安装paramiko库:
pip install paramiko
-
使用SFTP上传文件:
import paramiko
def upload_file_sftp(host, port, username, password, local_path, remote_path):
transport = paramiko.Transport((host, port))
transport.connect(username=username, password=password)
sftp = paramiko.SFTPClient.from_transport(transport)
sftp.put(local_path, remote_path)
sftp.close()
transport.close()
Usage
upload_file_sftp("sftp.example.com", 22, "sftp_user", "sftp_password", "local_file.txt", "remote_file.txt")
四、消息队列
使用RabbitMQ发送消息
消息队列是一种高效的消息传递方式,RabbitMQ是一个常用的消息队列系统。Python的pika
库可以用于与RabbitMQ交互。以下是一个示例:
-
安装pika库:
pip install pika
-
使用RabbitMQ发送消息:
import pika
def send_rabbitmq_message(host, queue, message):
connection = pika.BlockingConnection(pika.ConnectionParameters(host=host))
channel = connection.channel()
channel.queue_declare(queue=queue)
channel.basic_publish(exchange='', routing_key=queue, body=message)
connection.close()
Usage
send_rabbitmq_message("localhost", "test_queue", "This is a test message")
使用Redis发送消息
Redis是一种高性能的内存数据库,也可以用作消息队列。Python的redis
库可以用于与Redis交互。以下是一个示例:
-
安装redis库:
pip install redis
-
使用Redis发送消息:
import redis
def send_redis_message(host, port, channel, message):
r = redis.StrictRedis(host=host, port=port, db=0)
r.publish(channel, message)
Usage
send_redis_message("localhost", 6379, "test_channel", "This is a test message")
五、WebSocket
使用WebSocket发送消息
WebSocket是一种在单个TCP连接上进行全双工通信的协议。Python的websockets
库可以用于实现WebSocket通信。以下是一个示例:
-
安装websockets库:
pip install websockets
-
使用WebSocket发送消息:
import asyncio
import websockets
async def send_websocket_message(uri, message):
async with websockets.connect(uri) as websocket:
await websocket.send(message)
Usage
asyncio.get_event_loop().run_until_complete(send_websocket_message('ws://localhost:8765', 'This is a test message'))
使用Socket.IO发送消息
Socket.IO是一个用于实时双向事件通信的库,Python的sockio
库可以用于实现Socket.IO通信。以下是一个示例:
-
安装sockio库:
pip install python-socketio
-
使用Socket.IO发送消息:
import socketio
def send_socketio_message(url, message):
sio = socketio.Client()
sio.connect(url)
sio.emit('message', message)
sio.disconnect()
Usage
send_socketio_message('http://localhost:5000', 'This is a test message')
六、短信
使用Twilio API发送短信
Twilio是一个著名的通信服务提供商,可以通过其API发送短信。以下是一个示例:
-
安装twilio库:
pip install twilio
-
使用Twilio API发送短信:
from twilio.rest import Client
def send_sms_twilio(account_sid, auth_token, from_number, to_number, body):
client = Client(account_sid, auth_token)
message = client.messages.create(
body=body,
from_=from_number,
to=to_number
)
return message.sid
Usage
send_sms_twilio("your_account_sid", "your_auth_token", "+1234567890", "+0987654321", "This is a test message")
使用Nexmo API发送短信
Nexmo也是一个著名的通信服务提供商,可以通过其API发送短信。以下是一个示例:
-
安装nexmo库:
pip install nexmo
-
使用Nexmo API发送短信:
import nexmo
def send_sms_nexmo(api_key, api_secret, from_number, to_number, body):
client = nexmo.Client(key=api_key, secret=api_secret)
response = client.send_message({
'from': from_number,
'to': to_number,
'text': body,
})
return response
Usage
send_sms_nexmo("your_api_key", "your_api_secret", "Nexmo", "+0987654321", "This is a test message")
七、社交媒体
使用Twitter API发送推文
Twitter API允许开发者通过程序发送推文。Python的tweepy
库可以用于与Twitter API交互。以下是一个示例:
-
安装tweepy库:
pip install tweepy
-
使用Twitter API发送推文:
import tweepy
def send_tweet(api_key, api_secret_key, access_token, access_token_secret, status):
auth = tweepy.OAuth1UserHandler(api_key, api_secret_key, access_token, access_token_secret)
api = tweepy.API(auth)
api.update_status(status=status)
Usage
send_tweet("your_api_key", "your_api_secret_key", "your_access_token", "your_access_token_secret", "This is a test tweet")
使用Facebook API发送消息
Facebook API允许开发者通过程序发送消息。Python的facebook-sdk
库可以用于与Facebook API交互。以下是一个示例:
-
安装facebook-sdk库:
pip install facebook-sdk
-
使用Facebook API发送消息:
import facebook
def send_facebook_message(access_token, recipient_id, message):
graph = facebook.GraphAPI(access_token)
graph.put_object(parent_object=recipient_id, connection_name='feed', message=message)
Usage
send_facebook_message("your_access_token", "recipient_id", "This is a test message")
八、通知服务
使用Pushbullet API发送通知
Pushbullet是一个允许设备间发送通知的服务。Python的pushbullet
库可以用于与Pushbullet API交互。以下是一个示例:
-
安装pushbullet库:
pip install pushbullet.py
-
使用Pushbullet API发送通知:
from pushbullet import Pushbullet
def send_pushbullet_notification(api_key, title, body):
pb = Pushbullet(api_key)
pb.push_note(title, body)
Usage
send_pushbullet_notification("your_api_key", "Test Notification", "This is a test notification")
使用Pushover API发送通知
Pushover是一个允许设备间发送通知的服务。Python的requests
库可以用于与Pushover API交互。以下是一个示例:
-
安装requests库:
pip install requests
-
使用Pushover API发送通知:
import requests
def send_pushover_notification(token, user, message):
url = "https://api.pushover.net/1/messages.json"
data = {
"token": token,
"user": user,
"message": message
}
response = requests.post(url, data=data)
return response
Usage
send_pushover_notification("your_app_token", "your_user_key", "This is a test notification")
九、总结
通过以上多种方式,我们可以在Python中实现向别人发送信息的功能。无论是通过电子邮件、即时消息、文件传输还是消息队列等方式,都可以利用Python的丰富库资源来实现。选择合适的方式取决于具体的需求和应用场景。无论是哪种方式,合理的错误处理和安全性考虑都是必不可少的,以确保信息传递的可靠性和安全性。
相关问答FAQs:
如何将Python代码分享给他人?
可以通过多种方式分享Python代码。最常见的方法是将代码保存为.py文件,并通过电子邮件、云存储(如Google Drive、Dropbox)或版本控制平台(如GitHub)进行分享。您也可以将代码粘贴到代码分享网站上,例如Pastebin或Gist,以便于他人查看和下载。
在发送Python代码时,应该注意哪些事项?
发送代码时,确保代码的可读性是至关重要的。您可以添加注释以解释复杂的部分,并确保代码遵循良好的编码规范。此外,检查代码中是否包含敏感信息(如密码或API密钥),并在分享之前将其移除或替换为占位符。
是否有工具可以帮助我在发送代码时进行格式化?
有很多工具可以帮助您格式化Python代码,使其更加易读。例如,您可以使用在线代码格式化工具,如Black或YAPF,这些工具会自动调整代码的格式。此外,许多集成开发环境(IDE)也提供了代码格式化的功能,您可以在保存文件前进行格式化处理。
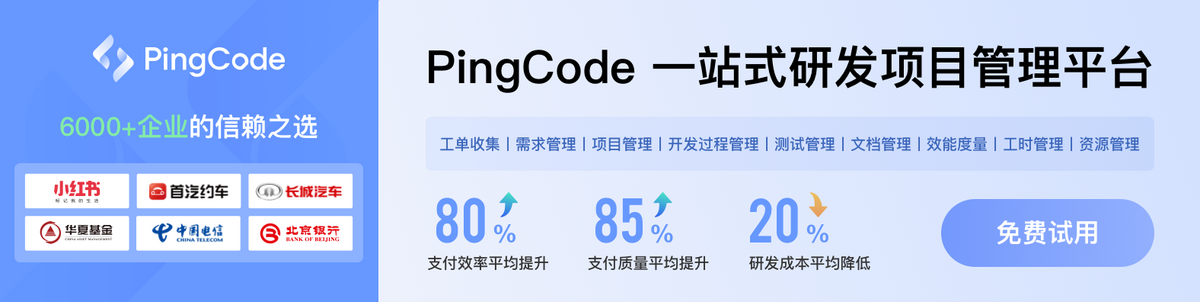