在Python中,删除换行符可以通过使用字符串的替换方法、正则表达式、文件读取等方式来实现。这些方法包括使用str.replace()、str.split()和str.join()、正则表达式re.sub()等。在文件操作时,可以逐行读取并处理,或一次性读取后处理文本。下面将详细介绍其中一种方法:
str.replace()方法:这种方法非常直接,通过将换行符替换为空字符串来删除换行符。例如:
text = "Hello\nWorld"
cleaned_text = text.replace("\n", "")
print(cleaned_text)
在这段代码中,\n
表示换行符,replace("\n", "")
将换行符替换为空字符串,从而删除所有换行符。
一、使用str.replace()方法
str.replace()方法:是最简单的一种方法,通过将换行符替换为空字符串来删除。适用于处理较短的字符串和简单的文本操作。
text = "Hello\nWorld"
cleaned_text = text.replace("\n", "")
print(cleaned_text)
在这个示例中,replace("\n", "")
将所有的换行符替换为空字符串,从而实现删除换行符的目的。这种方法适用于处理较短的字符串和简单的文本操作。
二、使用str.split()和str.join()方法
使用str.split()
和str.join()
方法也可以删除换行符。首先用split()
方法将字符串按换行符分割成列表,然后用join()
方法将其重新组合成字符串。
text = "Hello\nWorld"
split_text = text.split("\n")
cleaned_text = "".join(split_text)
print(cleaned_text)
在这个例子中,split("\n")
将字符串按换行符分割成两个部分,join(split_text)
将列表中的元素重新组合成一个没有换行符的字符串。
三、使用正则表达式re.sub()方法
正则表达式提供了更强大的文本处理能力。使用re.sub()
方法可以删除换行符。
import re
text = "Hello\nWorld"
cleaned_text = re.sub(r'\n', '', text)
print(cleaned_text)
在这个示例中,re.sub(r'\n', '', text)
使用正则表达式匹配所有换行符,并将其替换为空字符串,从而删除所有换行符。这种方法适用于需要复杂文本处理的情况。
四、逐行读取文件并处理
在处理文件时,可以逐行读取文件,并在处理每一行时删除换行符。
with open('file.txt', 'r') as file:
lines = file.readlines()
cleaned_lines = [line.strip() for line in lines]
with open('cleaned_file.txt', 'w') as cleaned_file:
cleaned_file.writelines(cleaned_lines)
在这个例子中,使用readlines()
方法读取文件的所有行,strip()
方法删除每行末尾的换行符,然后将处理后的行写入新文件。这种方法适用于处理较大的文件。
五、一次性读取文件并处理
另一种方法是一次性读取整个文件内容,然后删除换行符。
with open('file.txt', 'r') as file:
text = file.read()
cleaned_text = text.replace("\n", "")
with open('cleaned_file.txt', 'w') as cleaned_file:
cleaned_file.write(cleaned_text)
这种方法适用于处理较小的文件,一次性读取整个文件内容并进行处理。
六、处理多种换行符
在跨平台操作中,换行符可能是不同的,如\r\n
(Windows)、\n
(Unix/Linux)和\r
(旧版Mac)。可以使用正则表达式来处理这些不同的换行符。
import re
text = "Hello\r\nWorld\rPython\nProgramming"
cleaned_text = re.sub(r'\r\n|\r|\n', '', text)
print(cleaned_text)
在这个例子中,re.sub(r'\r\n|\r|\n', '', text)
可以匹配并删除所有类型的换行符。
七、使用第三方库
在某些情况下,可以使用第三方库进行更高级的文本处理。例如,pandas
库可以用来处理包含换行符的CSV文件。
import pandas as pd
df = pd.read_csv('file.csv')
df.replace(r'\n', '', regex=True, inplace=True)
df.to_csv('cleaned_file.csv', index=False)
在这个示例中,使用pandas
库读取CSV文件,并用replace()
方法删除所有换行符,然后将处理后的数据保存到新文件中。
八、处理大文件
对于非常大的文件,可以使用逐行处理的方法,以避免内存占用过多。
with open('large_file.txt', 'r') as infile, open('cleaned_large_file.txt', 'w') as outfile:
for line in infile:
cleaned_line = line.replace('\n', '')
outfile.write(cleaned_line)
在这个示例中,逐行读取和处理文件内容,然后写入新文件。这种方法适用于处理非常大的文件。
九、删除特定位置的换行符
在某些情况下,可能只需要删除特定位置的换行符。例如,只删除字符串开头或结尾的换行符。
text = "\nHello World\n"
cleaned_text = text.strip('\n')
print(cleaned_text)
在这个例子中,strip('\n')
只删除字符串开头和结尾的换行符,而不影响中间部分。
十、结合多种方法
在实际应用中,可能需要结合多种方法来处理复杂的情况。例如,先使用正则表达式删除所有换行符,然后再进行进一步的文本处理。
import re
text = "Hello\r\nWorld\nPython\rProgramming"
cleaned_text = re.sub(r'\r\n|\r|\n', '', text)
进一步处理
processed_text = cleaned_text.lower()
print(processed_text)
在这个示例中,先使用正则表达式删除所有换行符,然后将结果转换为小写,以满足特定的需求。
十一、处理包含多行文本的字符串
有时候需要处理包含多行文本的字符串,如多行注释或文档字符串。
text = """Hello,
This is a sample text.
It contains multiple lines.
"""
cleaned_text = text.replace('\n', '')
print(cleaned_text)
在这个例子中,replace('\n', '')
可以删除所有换行符,使多行文本变为单行文本。
十二、处理包含换行符的列表
如果有一个包含换行符的字符串列表,可以使用列表推导式来处理每个字符串。
lines = ["Hello\n", "World\n", "Python\n"]
cleaned_lines = [line.replace('\n', '') for line in lines]
print(cleaned_lines)
在这个示例中,使用列表推导式[line.replace('\n', '') for line in lines]
来处理每个字符串,删除其中的换行符。
十三、处理包含换行符的字典
类似地,如果有一个包含换行符的字符串字典,可以使用字典推导式来处理每个值。
data = {"line1": "Hello\n", "line2": "World\n", "line3": "Python\n"}
cleaned_data = {key: value.replace('\n', '') for key, value in data.items()}
print(cleaned_data)
在这个例子中,使用字典推导式{key: value.replace('\n', '') for key, value in data.items()}
来处理每个值,删除其中的换行符。
十四、处理包含换行符的嵌套结构
对于包含换行符的嵌套结构(如列表中的字典),可以使用递归方法来处理。
def remove_newlines(data):
if isinstance(data, str):
return data.replace('\n', '')
elif isinstance(data, list):
return [remove_newlines(item) for item in data]
elif isinstance(data, dict):
return {key: remove_newlines(value) for key, value in data.items()}
return data
nested_data = {"lines": ["Hello\n", "World\n"], "info": {"line1": "Python\n", "line2": "Programming\n"}}
cleaned_data = remove_newlines(nested_data)
print(cleaned_data)
在这个示例中,定义了一个递归函数remove_newlines()
,可以处理包含换行符的字符串、列表和字典。
十五、处理包含换行符的DataFrame
使用pandas
库处理包含换行符的DataFrame,可以用applymap()
方法来处理每个单元格。
import pandas as pd
df = pd.DataFrame({"A": ["Hello\n", "World\n"], "B": ["Python\n", "Programming\n"]})
cleaned_df = df.applymap(lambda x: x.replace('\n', ''))
print(cleaned_df)
在这个示例中,使用applymap(lambda x: x.replace('\n', ''))
方法来处理每个单元格,删除其中的换行符。
十六、处理包含换行符的JSON数据
在处理包含换行符的JSON数据时,可以先将其转换为字符串,然后删除换行符,再将其转换回JSON格式。
import json
data = {"line1": "Hello\n", "line2": "World\n", "line3": "Python\n"}
json_data = json.dumps(data)
cleaned_json_data = json_data.replace('\n', '')
cleaned_data = json.loads(cleaned_json_data)
print(cleaned_data)
在这个例子中,使用json.dumps()
将数据转换为字符串,删除换行符后,再使用json.loads()
将其转换回JSON格式。
十七、处理包含换行符的XML数据
在处理包含换行符的XML数据时,可以使用xml.etree.ElementTree
模块进行解析和处理。
import xml.etree.ElementTree as ET
xml_data = """<root>
<line>Hello\n</line>
<line>World\n</line>
<line>Python\n</line>
</root>"""
root = ET.fromstring(xml_data)
for elem in root.iter():
if elem.text:
elem.text = elem.text.replace('\n', '')
cleaned_xml_data = ET.tostring(root).decode()
print(cleaned_xml_data)
在这个示例中,使用xml.etree.ElementTree
模块解析XML数据,并删除元素文本中的换行符。
十八、处理包含换行符的HTML数据
在处理包含换行符的HTML数据时,可以使用BeautifulSoup
库进行解析和处理。
from bs4 import BeautifulSoup
html_data = """<html>
<body>
<p>Hello\n</p>
<p>World\n</p>
<p>Python\n</p>
</body>
</html>"""
soup = BeautifulSoup(html_data, 'html.parser')
for elem in soup.find_all(text=True):
elem.replace_with(elem.replace('\n', ''))
cleaned_html_data = str(soup)
print(cleaned_html_data)
在这个示例中,使用BeautifulSoup
库解析HTML数据,并删除文本中的换行符。
十九、处理包含换行符的CSV数据
在处理包含换行符的CSV数据时,可以使用csv
模块进行解析和处理。
import csv
csv_data = """line1,line2
Hello\n,World\n
Python\n,Programming\n"""
reader = csv.reader(csv_data.splitlines())
cleaned_data = [[cell.replace('\n', '') for cell in row] for row in reader]
with open('cleaned_file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(cleaned_data)
在这个例子中,使用csv
模块解析CSV数据,并删除单元格中的换行符。
二十、处理包含换行符的Excel数据
在处理包含换行符的Excel数据时,可以使用openpyxl
库进行解析和处理。
import openpyxl
创建示例Excel文件
wb = openpyxl.Workbook()
ws = wb.active
ws['A1'] = "Hello\n"
ws['B1'] = "World\n"
ws['A2'] = "Python\n"
ws['B2'] = "Programming\n"
wb.save('example.xlsx')
读取并处理Excel文件
wb = openpyxl.load_workbook('example.xlsx')
ws = wb.active
for row in ws.iter_rows():
for cell in row:
if cell.value:
cell.value = cell.value.replace('\n', '')
wb.save('cleaned_example.xlsx')
在这个示例中,使用openpyxl
库读取Excel文件,并删除单元格中的换行符。
二十一、处理包含换行符的PDF数据
在处理包含换行符的PDF数据时,可以使用PyPDF2
库进行解析和处理。
import PyPDF2
创建示例PDF文件
from fpdf import FPDF
pdf = FPDF()
pdf.add_page()
pdf.set_font("Arial", size=12)
pdf.cell(200, 10, txt="Hello\nWorld\n", ln=True)
pdf.cell(200, 10, txt="Python\nProgramming\n", ln=True)
pdf.output("example.pdf")
读取并处理PDF文件
reader = PyPDF2.PdfFileReader("example.pdf")
writer = PyPDF2.PdfFileWriter()
for page_num in range(reader.numPages):
page = reader.getPage(page_num)
text = page.extract_text()
cleaned_text = text.replace('\n', '')
page.merge_text(cleaned_text)
writer.add_page(page)
with open("cleaned_example.pdf", "wb") as file:
writer.write(file)
在这个示例中,使用PyPDF2
库读取PDF文件,并删除文本中的换行符。
二十二、处理包含换行符的Markdown数据
在处理包含换行符的Markdown数据时,可以使用markdown
库进行解析和处理。
import markdown
md_data = """# Title
Hello\n
World\n
Python\n
Programming\n"""
html = markdown.markdown(md_data)
cleaned_html = html.replace('\n', '')
print(cleaned_html)
在这个示例中,使用markdown
库将Markdown数据转换为HTML,并删除HTML中的换行符。
二十三、处理包含换行符的日志数据
在处理包含换行符的日志数据时,可以逐行读取日志文件,并删除每行末尾的换行符。
with open('logfile.log', 'r') as file:
lines = file.readlines()
cleaned_lines = [line.rstrip('\n') for line in lines]
with open('cleaned_logfile.log', 'w') as cleaned_file:
cleaned_file.writelines(cleaned_lines)
在这个例子中,逐行读取日志文件,并使用rstrip('\n')
方法删除每行末尾的换行符,然后写入新文件。
二十四、处理包含换行符的配置文件
在处理包含换行符的配置文件时,可以逐行读取配置文件,并删除每行末尾的换行符。
with open('config.ini', 'r') as file:
lines = file.readlines()
cleaned_lines = [line.rstrip('\n') for line in lines]
with open('cleaned_config.ini', 'w') as cleaned_file:
cleaned_file.writelines(cleaned_lines)
在这个例子中,逐行读取配置文件,并使用rstrip('\n')
方法删除每行末尾的换行符,然后写入新文件。
二十五、处理包含换行符的SQL数据
在处理包含换行符的SQL数据时,可以逐行读取SQL脚本文件,并删除每行末尾的换行符。
with open('script.sql', 'r') as file:
lines = file.readlines()
cleaned_lines = [line.rstrip('\n') for line in lines]
with open('cleaned_script.sql', 'w') as cleaned_file:
cleaned_file.writelines(cleaned_lines)
在这个例子中,逐行读取SQL脚本文件,并使用rstrip('\n')
方法删除每行末尾的换行符,然后写入新文件。
二十六、处理包含换行符的邮件数据
在处理包含换行符的邮件数据时,可以逐行读取邮件文件,并删除每行末尾的换行符。
with open('email.eml', 'r') as file:
lines = file.readlines()
cleaned_lines = [line.rstrip('\n') for line in lines]
with open('cleaned_email.eml', 'w') as cleaned_file:
cleaned_file.writelines(cleaned_lines)
在这个例子中,逐行读取邮件文件,并使用`rstrip('\n')
相关问答FAQs:
在Python中,如何去除字符串中的换行符?
可以使用str.replace()
或str.strip()
方法来删除字符串中的换行符。例如,使用string.replace('\n', '')
可以将换行符替换为空字符串,从而去掉它们。如果想删除字符串开头和结尾的换行符,可以使用string.strip()
方法。
在处理文件时,如何去掉每行末尾的换行符?
在读取文件内容时,可以使用readlines()
方法将每一行读取为列表。接着,可以通过列表推导式和strip()
方法去掉每行末尾的换行符。例如,使用[line.strip() for line in file.readlines()]
可以获得一个不包含换行符的行列表。
如何使用正则表达式删除字符串中的换行符?
正则表达式提供了强大的文本处理能力。可以使用re.sub()
函数来删除换行符。例如,使用import re
后,执行re.sub(r'\n', '', your_string)
可以将字符串中的所有换行符替换为空字符串,实现删除效果。
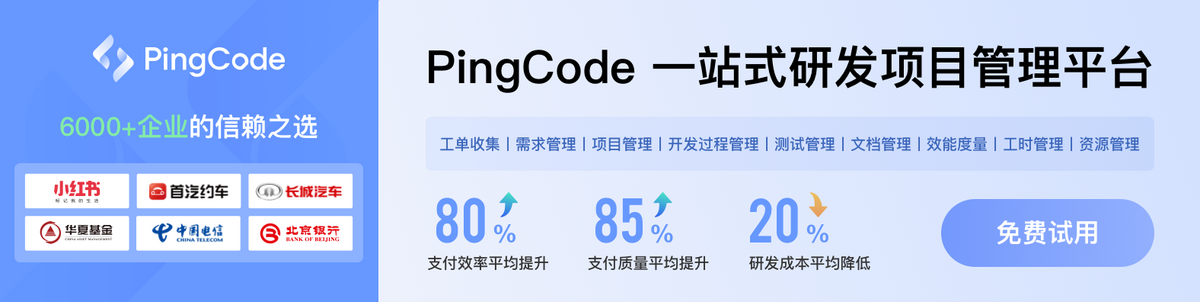