Python同时输入输出可以通过多线程、多进程、异步IO、队列等方式实现。在这些方法中,异步IO是最推荐的方式,因为它能够高效地管理输入输出操作,尤其是在处理大量I/O密集型任务时。以下是异步IO的详细描述:
在Python中,异步I/O操作可以通过asyncio
库来实现。asyncio
是一个用于编写并发代码的库,通过异步I/O的协程和事件循环,使得我们可以在一个线程内高效地处理多个I/O操作。在异步编程中,输入输出操作不会阻塞其他任务的执行,而是通过事件循环调度任务,从而提高程序的执行效率。
import asyncio
async def read_input():
while True:
user_input = await asyncio.to_thread(input, "Enter something: ")
print(f'You entered: {user_input}')
if user_input.lower() == 'exit':
break
async def main():
await read_input()
if __name__ == "__main__":
asyncio.run(main())
在上述示例中,read_input
函数是一个异步函数,通过await asyncio.to_thread(input, "Enter something: ")
来异步地读取用户输入,而不会阻塞程序的其他部分。asyncio.run(main())
用于运行事件循环,调度和执行异步任务。
一、多线程
多线程是通过创建多个线程来同时执行多个任务。在Python中,可以使用threading
模块来实现多线程。
1、创建线程
首先,我们需要创建一个线程并定义线程函数。
import threading
def thread_function(name):
print(f"Thread {name}: starting")
# 模拟I/O操作
time.sleep(2)
print(f"Thread {name}: finishing")
if __name__ == "__main__":
thread = threading.Thread(target=thread_function, args=(1,))
thread.start()
thread.join()
在上述示例中,我们创建了一个线程,并通过threading.Thread
创建线程对象,target
参数是线程函数,args
参数是传递给线程函数的参数。通过调用start()
方法启动线程,join()
方法等待线程结束。
2、多线程输入输出
在多线程中进行输入输出操作时,可以使用队列来实现线程之间的通信。queue
模块提供了线程安全的队列,可以用来在线程之间传递数据。
import threading
import queue
def producer(q):
while True:
item = input("Enter something: ")
q.put(item)
if item.lower() == 'exit':
break
def consumer(q):
while True:
item = q.get()
print(f'You entered: {item}')
if item.lower() == 'exit':
break
if __name__ == "__main__":
q = queue.Queue()
t1 = threading.Thread(target=producer, args=(q,))
t2 = threading.Thread(target=consumer, args=(q,))
t1.start()
t2.start()
t1.join()
t2.join()
在上述示例中,producer
函数负责读取用户输入并将其放入队列,consumer
函数负责从队列中取出数据并打印。通过使用队列,我们可以在线程之间进行数据传递,从而实现多线程的输入输出操作。
二、多进程
多进程是通过创建多个进程来同时执行多个任务。在Python中,可以使用multiprocessing
模块来实现多进程。
1、创建进程
首先,我们需要创建一个进程并定义进程函数。
import multiprocessing
def process_function(name):
print(f"Process {name}: starting")
# 模拟I/O操作
time.sleep(2)
print(f"Process {name}: finishing")
if __name__ == "__main__":
process = multiprocessing.Process(target=process_function, args=(1,))
process.start()
process.join()
在上述示例中,我们创建了一个进程,并通过multiprocessing.Process
创建进程对象,target
参数是进程函数,args
参数是传递给进程函数的参数。通过调用start()
方法启动进程,join()
方法等待进程结束。
2、多进程输入输出
在多进程中进行输入输出操作时,可以使用队列来实现进程之间的通信。multiprocessing.Queue
提供了进程安全的队列,可以用来在进程之间传递数据。
import multiprocessing
def producer(q):
while True:
item = input("Enter something: ")
q.put(item)
if item.lower() == 'exit':
break
def consumer(q):
while True:
item = q.get()
print(f'You entered: {item}')
if item.lower() == 'exit':
break
if __name__ == "__main__":
q = multiprocessing.Queue()
p1 = multiprocessing.Process(target=producer, args=(q,))
p2 = multiprocessing.Process(target=consumer, args=(q,))
p1.start()
p2.start()
p1.join()
p2.join()
在上述示例中,producer
函数负责读取用户输入并将其放入队列,consumer
函数负责从队列中取出数据并打印。通过使用队列,我们可以在进程之间进行数据传递,从而实现多进程的输入输出操作。
三、异步IO
异步I/O是通过事件循环来调度和执行异步任务。在Python中,可以使用asyncio
模块来实现异步I/O。
1、定义异步函数
首先,我们需要定义异步函数,并使用async
关键字定义协程,使用await
关键字等待异步操作。
import asyncio
async def async_function(name):
print(f"Async function {name}: starting")
# 模拟I/O操作
await asyncio.sleep(2)
print(f"Async function {name}: finishing")
async def main():
await async_function(1)
if __name__ == "__main__":
asyncio.run(main())
在上述示例中,我们定义了一个异步函数,并通过await asyncio.sleep(2)
模拟异步I/O操作。asyncio.run(main())
用于运行事件循环,调度和执行异步任务。
2、异步IO输入输出
在异步I/O中进行输入输出操作时,可以使用asyncio
模块提供的异步I/O函数。
import asyncio
async def read_input():
while True:
user_input = await asyncio.to_thread(input, "Enter something: ")
print(f'You entered: {user_input}')
if user_input.lower() == 'exit':
break
async def main():
await read_input()
if __name__ == "__main__":
asyncio.run(main())
在上述示例中,read_input
函数是一个异步函数,通过await asyncio.to_thread(input, "Enter something: ")
来异步地读取用户输入,而不会阻塞程序的其他部分。asyncio.run(main())
用于运行事件循环,调度和执行异步任务。
四、队列
队列是一种先进先出的数据结构,可以用来在多个任务之间传递数据。在Python中,可以使用queue
模块和multiprocessing.Queue
来实现线程和进程之间的通信。
1、线程队列
在多线程中使用队列,可以通过queue.Queue
来实现线程之间的通信。
import threading
import queue
def producer(q):
while True:
item = input("Enter something: ")
q.put(item)
if item.lower() == 'exit':
break
def consumer(q):
while True:
item = q.get()
print(f'You entered: {item}')
if item.lower() == 'exit':
break
if __name__ == "__main__":
q = queue.Queue()
t1 = threading.Thread(target=producer, args=(q,))
t2 = threading.Thread(target=consumer, args=(q,))
t1.start()
t2.start()
t1.join()
t2.join()
在上述示例中,producer
函数负责读取用户输入并将其放入队列,consumer
函数负责从队列中取出数据并打印。通过使用队列,我们可以在线程之间进行数据传递,从而实现多线程的输入输出操作。
2、进程队列
在多进程中使用队列,可以通过multiprocessing.Queue
来实现进程之间的通信。
import multiprocessing
def producer(q):
while True:
item = input("Enter something: ")
q.put(item)
if item.lower() == 'exit':
break
def consumer(q):
while True:
item = q.get()
print(f'You entered: {item}')
if item.lower() == 'exit':
break
if __name__ == "__main__":
q = multiprocessing.Queue()
p1 = multiprocessing.Process(target=producer, args=(q,))
p2 = multiprocessing.Process(target=consumer, args=(q,))
p1.start()
p2.start()
p1.join()
p2.join()
在上述示例中,producer
函数负责读取用户输入并将其放入队列,consumer
函数负责从队列中取出数据并打印。通过使用队列,我们可以在进程之间进行数据传递,从而实现多进程的输入输出操作。
五、总结
在Python中,同时进行输入输出操作可以通过多线程、多进程、异步I/O和队列等方式来实现。每种方式都有其优点和适用场景:
- 多线程:适用于I/O密集型任务,可以通过线程之间的队列实现数据传递。
- 多进程:适用于CPU密集型任务,可以通过进程之间的队列实现数据传递。
- 异步I/O:适用于大量I/O密集型任务,通过事件循环调度任务,提高执行效率。
- 队列:可以在线程或进程之间传递数据,实现线程或进程之间的通信。
根据具体的需求选择合适的方式,可以有效地提高程序的执行效率和性能。
相关问答FAQs:
如何在Python中实现同时输入和输出?
在Python中,可以使用多线程或异步编程来同时进行输入和输出。通过使用threading
模块或asyncio
库,可以创建一个程序,允许用户在输入数据的同时输出结果。例如,您可以在一个线程中处理用户输入,而在另一个线程中处理输出,确保两者不会相互干扰。
在Python中,如何使用多线程进行输入和输出?
通过threading
模块,可以创建两个线程,一个用于接收用户输入,另一个用于显示输出。使用Queue
模块可以有效地在这两个线程之间传递数据。这样,输入和输出可以并行进行,提高程序的响应速度。
是否有示例代码展示如何在Python中实现同时输入输出的功能?
当然可以。以下是一个简单示例,展示如何使用多线程实现同时输入和输出:
import threading
import queue
import time
def input_thread(q):
while True:
user_input = input("请输入内容:")
q.put(user_input)
def output_thread(q):
while True:
if not q.empty():
output = q.get()
print(f"输出内容:{output}")
q = queue.Queue()
t1 = threading.Thread(target=input_thread, args=(q,))
t2 = threading.Thread(target=output_thread, args=(q,))
t1.start()
t2.start()
这个示例创建了两个线程,分别处理输入和输出,通过队列实现了两者之间的通信。
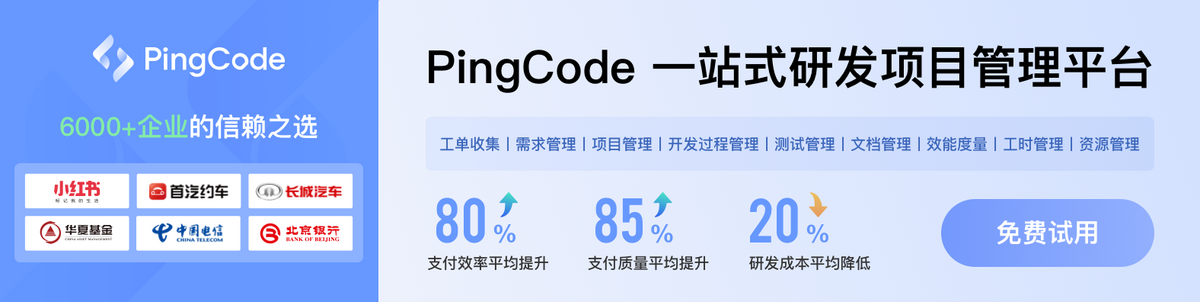