Python封装代码的主要方法包括:使用函数、使用类、使用模块和包、使用装饰器。其中,使用类是封装代码的一个重要方式,它可以将属性和方法封装在一起,使代码更加模块化和易于维护。类的封装不仅仅是将代码组织在一起,还包括信息隐藏、接口暴露等技术。下面将详细介绍如何通过类来封装代码。
一、函数封装
函数是Python封装代码的基本单元。通过定义函数,可以将代码组织成一个个独立的模块,便于重用和维护。
1.定义函数
定义一个函数的基本语法如下:
def function_name(parameters):
"""
Docstring explaining the function.
"""
# Function body
return result
例如,定义一个计算两个数之和的函数:
def add(a, b):
"""
Return the sum of a and b.
"""
return a + b
2.调用函数
调用函数的方式非常简单,只需使用函数名和传递必要的参数:
result = add(5, 3)
print(result) # 输出8
二、类封装
类是Python面向对象编程的核心,通过类可以将属性和方法封装在一起,提供更高层次的抽象。
1.定义类
定义一个类的基本语法如下:
class ClassName:
"""
Docstring explaining the class.
"""
def __init__(self, parameters):
# Initialize attributes
self.attribute = parameters
def method(self, parameters):
# Method body
return result
例如,定义一个表示点的类:
class Point:
"""
A class to represent a point in 2D space.
"""
def __init__(self, x, y):
self.x = x
self.y = y
def move(self, dx, dy):
self.x += dx
self.y += dy
def __str__(self):
return f"Point({self.x}, {self.y})"
2.使用类
创建类的实例并调用其方法:
p = Point(2, 3)
print(p) # 输出Point(2, 3)
p.move(1, -1)
print(p) # 输出Point(3, 2)
三、模块和包
模块和包是Python封装代码的高级单元,通过将代码组织成模块和包,可以实现代码的模块化和重用。
1.创建模块
模块是一个包含Python代码的文件。创建一个模块非常简单,只需将代码保存到一个.py
文件中。例如,创建一个名为mymodule.py
的模块:
# mymodule.py
def greet(name):
return f"Hello, {name}!"
2.导入模块
可以使用import
语句导入模块:
import mymodule
print(mymodule.greet("Alice")) # 输出Hello, Alice!
3.创建包
包是一个包含多个模块的目录。创建包时,需要在目录中包含一个名为__init__.py
的文件。例如,创建一个名为mypackage
的包:
mypackage/
__init__.py
module1.py
module2.py
在__init__.py
中,可以定义包的初始化代码:
# __init__.py
from .module1 import *
from .module2 import *
可以使用import
语句导入包:
import mypackage
print(mypackage.module1.function1())
print(mypackage.module2.function2())
四、装饰器
装饰器是一种用于修改函数或类行为的高级封装技术。通过装饰器,可以在不修改原函数或类的情况下,添加新的功能。
1.定义装饰器
定义一个装饰器的基本语法如下:
def decorator(function):
def wrapper(*args, kwargs):
# Code before function call
result = function(*args, kwargs)
# Code after function call
return result
return wrapper
例如,定义一个计算函数执行时间的装饰器:
import time
def timer(function):
def wrapper(*args, kwargs):
start_time = time.time()
result = function(*args, kwargs)
end_time = time.time()
print(f"Execution time: {end_time - start_time:.4f} seconds")
return result
return wrapper
2.使用装饰器
使用装饰器的方式非常简单,只需在函数定义前加上@decorator
:
@timer
def slow_function():
time.sleep(2)
return "Finished"
print(slow_function())
五、综合实例
下面是一个综合实例,展示如何使用函数、类、模块、包和装饰器来封装代码。
1.创建模块和包
首先,创建一个名为geometry
的包,包含以下结构:
geometry/
__init__.py
shapes.py
utils.py
在shapes.py
中定义一些几何图形类:
# shapes.py
class Circle:
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14159 * self.radius 2
def perimeter(self):
return 2 * 3.14159 * self.radius
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
def perimeter(self):
return 2 * (self.width + self.height)
在utils.py
中定义一些实用函数:
# utils.py
def print_shape_info(shape):
print(f"Area: {shape.area()}")
print(f"Perimeter: {shape.perimeter()}")
在__init__.py
中导入这些模块:
# __init__.py
from .shapes import Circle, Rectangle
from .utils import print_shape_info
2.使用包
在另一个文件中导入并使用geometry
包:
import geometry
circle = geometry.Circle(5)
rectangle = geometry.Rectangle(4, 6)
geometry.print_shape_info(circle)
geometry.print_shape_info(rectangle)
3.添加装饰器
为Circle
和Rectangle
类的方法添加一个计算执行时间的装饰器:
# utils.py
import time
def timer(function):
def wrapper(*args, kwargs):
start_time = time.time()
result = function(*args, kwargs)
end_time = time.time()
print(f"Execution time for {function.__name__}: {end_time - start_time:.4f} seconds")
return result
return wrapper
shapes.py
from .utils import timer
class Circle:
def __init__(self, radius):
self.radius = radius
@timer
def area(self):
return 3.14159 * self.radius 2
@timer
def perimeter(self):
return 2 * 3.14159 * self.radius
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = self.height
@timer
def area(self):
return self.width * self.height
@timer
def perimeter(self):
return 2 * (self.width + self.height)
再次运行使用包的代码:
import geometry
circle = geometry.Circle(5)
rectangle = geometry.Rectangle(4, 6)
geometry.print_shape_info(circle)
geometry.print_shape_info(rectangle)
可以看到,每次调用area
和perimeter
方法时,都会输出方法的执行时间。
六、结论
通过以上内容,我们深入了解了Python封装代码的多种方法,包括函数、类、模块和包、装饰器等。每种方法都有其适用的场景和特点。在实际应用中,可以根据具体需求选择合适的封装方法,使代码更加模块化、易于维护和重用。通过合理的封装,可以提高代码的可读性、可扩展性和可靠性,为开发高质量的软件系统打下坚实的基础。
相关问答FAQs:
如何在Python中创建一个自定义模块?
创建自定义模块非常简单。您只需要将您的代码保存为一个以“.py”结尾的文件。例如,您可以创建一个名为my_module.py
的文件,并在其中编写您的函数和类。然后,在其他Python文件中,您可以通过import my_module
来导入这个模块,从而使用您定义的内容。
封装代码的好处是什么?
封装代码的主要好处在于提高代码的可重用性和可维护性。通过将相关功能组织到模块或类中,您可以减少代码重复,并使其更易于理解和修改。此外,封装还可以帮助您将复杂的实现细节隐藏起来,仅暴露必要的接口给使用者,从而提高代码的安全性。
在封装中使用类有什么优势?
使用类进行封装可以帮助您更好地组织代码。类允许您将数据和功能结合在一起,形成一个整体。这种结构使得管理大型代码库变得更加高效,尤其是在面向对象编程中。通过使用类,您可以创建实例,每个实例都有自己的状态和行为,这在处理复杂的数据时特别有用。
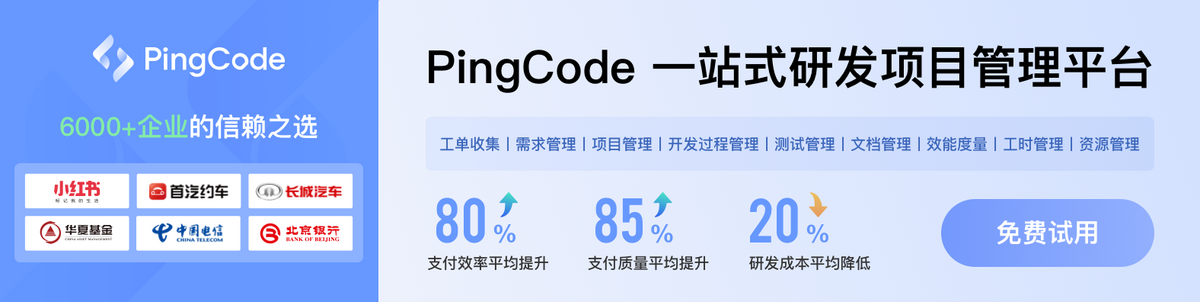