Python处理Word文档的方法包括:使用python-docx库、使用comtypes库、使用PyWin32库、以及基于模板生成文档。其中,python-docx库是最常用且功能齐全的库,它能够让你轻松地读取和写入Word文档。下面将详细介绍使用python-docx库处理Word文档的方法。
一、安装和基础操作
要使用python-docx库,你首先需要安装它。你可以使用pip命令来安装:
pip install python-docx
安装完成后,可以通过以下代码导入库并打开一个Word文档:
from docx import Document
打开一个已有的Word文档
doc = Document('example.docx')
创建一个新的Word文档
new_doc = Document()
二、读取Word文档内容
读取Word文档的内容是处理Word文档的基础。通过python-docx库,可以轻松地读取文档的各个部分,包括段落、表格和图片等。
读取段落
for paragraph in doc.paragraphs:
print(paragraph.text)
读取表格
for table in doc.tables:
for row in table.rows:
for cell in row.cells:
print(cell.text)
读取标题和其他内容
你还可以读取文档中的标题、页眉、页脚等内容:
# 读取标题
for paragraph in doc.paragraphs:
if paragraph.style.name.startswith('Heading'):
print(paragraph.text)
# 读取页眉
for section in doc.sections:
header = section.header
for paragraph in header.paragraphs:
print(paragraph.text)
三、修改Word文档内容
python-docx库不仅可以读取文档内容,还可以修改文档内容。你可以添加新段落、修改现有段落、插入表格和图片等。
添加段落
new_doc.add_paragraph('This is a new paragraph.')
添加标题
new_doc.add_heading('This is a new heading.', level=1)
插入表格
table = new_doc.add_table(rows=2, cols=2)
table.cell(0, 0).text = 'Cell 1'
table.cell(0, 1).text = 'Cell 2'
table.cell(1, 0).text = 'Cell 3'
table.cell(1, 1).text = 'Cell 4'
插入图片
new_doc.add_picture('image.png', width=Inches(1.25))
修改现有段落
for paragraph in doc.paragraphs:
if 'old text' in paragraph.text:
paragraph.text = paragraph.text.replace('old text', 'new text')
四、保存Word文档
完成所有修改后,你可以将文档保存到指定路径:
new_doc.save('new_example.docx')
五、复杂操作和高级功能
除了基本的读取和修改操作,python-docx库还提供了一些高级功能,如设置段落样式、添加页眉页脚、设置表格样式等。
设置段落样式
paragraph = new_doc.add_paragraph('This is a styled paragraph.')
paragraph.style = 'Title'
添加页眉和页脚
section = new_doc.sections[0]
header = section.header
header_paragraph = header.paragraphs[0]
header_paragraph.text = 'This is a header.'
footer = section.footer
footer_paragraph = footer.paragraphs[0]
footer_paragraph.text = 'This is a footer.'
设置表格样式
table.style = 'Table Grid'
六、处理复杂文档结构
对于一些复杂的文档结构,例如嵌套表格、分节符、多列布局等,python-docx库也有相应的功能支持。
处理分节符
# 添加分节符
new_section = new_doc.add_section(WD_SECTION.NEW_PAGE)
处理多列布局
# 设置多列布局
section = new_doc.sections[0]
section.page_width = Inches(8.5)
section.page_height = Inches(11)
section.left_margin = Inches(1)
section.right_margin = Inches(1)
设置为两列
section.columns[0].width = Inches(4)
section.columns[1].width = Inches(4)
七、综合应用实例
下面是一个综合应用实例,演示如何使用python-docx库创建一个包含段落、标题、表格和图片的Word文档,并设置样式和页眉页脚。
from docx import Document
from docx.shared import Inches
from docx.enum.section import WD_SECTION
创建一个新的Word文档
doc = Document()
添加标题
doc.add_heading('Python-docx Comprehensive Example', level=1)
添加段落
doc.add_paragraph('This is an example of using the python-docx library to create and modify Word documents.')
添加表格
table = doc.add_table(rows=3, cols=3)
table.style = 'Table Grid'
for row in range(3):
for col in range(3):
table.cell(row, col).text = f'Row {row+1}, Col {col+1}'
添加图片
doc.add_picture('image.png', width=Inches(2))
添加页眉和页脚
section = doc.sections[0]
header = section.header
header_paragraph = header.paragraphs[0]
header_paragraph.text = 'This is a header.'
footer = section.footer
footer_paragraph = footer.paragraphs[0]
footer_paragraph.text = 'This is a footer.'
添加分节符和多列布局
new_section = doc.add_section(WD_SECTION.NEW_PAGE)
new_section.columns[0].width = Inches(4)
new_section.columns[1].width = Inches(4)
保存文档
doc.save('comprehensive_example.docx')
八、其他处理Word文档的库
除了python-docx库,还有其他一些库也可以用来处理Word文档,例如comtypes库和PyWin32库。
使用comtypes库
comtypes库可以通过调用微软的COM接口来操作Word文档。下面是一个简单的示例:
import comtypes.client
打开Word应用程序
word = comtypes.client.CreateObject('Word.Application')
word.Visible = True
打开一个文档
doc = word.Documents.Open('example.docx')
读取文档内容
for paragraph in doc.Paragraphs:
print(paragraph.Range.Text)
关闭文档和Word应用程序
doc.Close()
word.Quit()
使用PyWin32库
PyWin32库也是通过调用微软的COM接口来操作Word文档。下面是一个简单的示例:
import win32com.client
打开Word应用程序
word = win32com.client.Dispatch('Word.Application')
word.Visible = True
打开一个文档
doc = word.Documents.Open('example.docx')
读取文档内容
for paragraph in doc.Paragraphs:
print(paragraph.Range.Text)
关闭文档和Word应用程序
doc.Close()
word.Quit()
总结
Python处理Word文档的方法多种多样,最常用的是使用python-docx库。通过python-docx库,你可以轻松地读取、修改和保存Word文档。此外,comtypes库和PyWin32库也提供了处理Word文档的功能。无论你选择哪种方法,都可以根据具体需求灵活应用,完成各种复杂的文档处理任务。通过不断实践和学习,你将能够更加熟练地使用Python处理Word文档。
相关问答FAQs:
如何使用Python读取Word文档中的文本内容?
在Python中,读取Word文档通常可以通过python-docx
库来实现。首先,确保安装了该库,可以使用pip install python-docx
进行安装。接着,使用以下代码示例读取文档中的文本:
from docx import Document
doc = Document('your_file.docx')
for paragraph in doc.paragraphs:
print(paragraph.text)
这样就可以提取Word文档中的所有段落文本。
Python能否对Word文档进行格式化处理?
是的,Python不仅可以读取Word文档的文本内容,还可以对文本进行格式化处理。使用python-docx
库,可以设置字体、大小、颜色等属性。例如:
from docx import Document
from docx.shared import Pt
from docx.enum.text import WD_PARAGRAPH_ALIGNMENT
doc = Document()
para = doc.add_paragraph('Hello World!')
run = para.runs[0]
run.font.size = Pt(14) # 设置字体大小
para.alignment = WD_PARAGRAPH_ALIGNMENT.CENTER # 设置段落居中
doc.save('formatted.docx')
这段代码创建了一个新的Word文档,并将文本格式化为特定的字体大小和段落对齐方式。
如何在Python中添加图像到Word文档?
在处理Word文档时,添加图像也是一个常见需求。使用python-docx
库,可以轻松地将图片插入到文档中。以下是添加图像的示例代码:
from docx import Document
doc = Document()
doc.add_heading('Document Title', level=1)
doc.add_paragraph('Here is an image:')
doc.add_picture('image.png') # 替换为你的图片文件名
doc.save('document_with_image.docx')
通过上述代码,可以将指定的图像插入到Word文档中,丰富文档的内容。
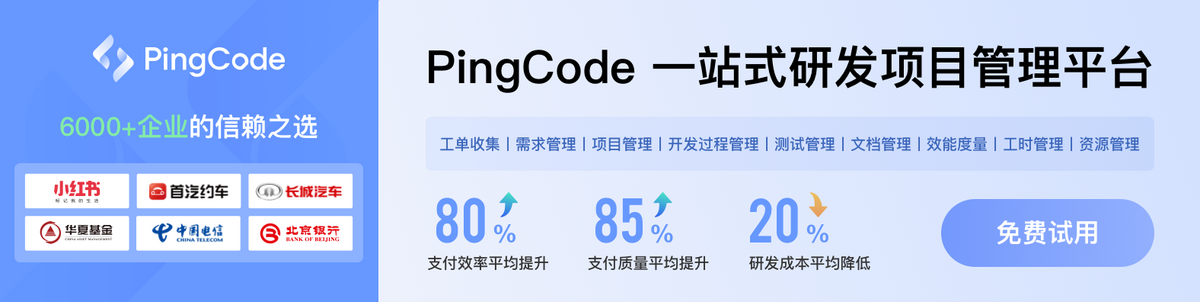